代码内容
// 递归解决老鼠找迷宫
public class Labyrinth{
public static void main(String[] args) {
// 先画出迷宫地图,规定0是可以走的,1是墙不能走
int[][] map = new int[8][7];//8行7列
for (int i = 0;i< 8; i++) {
for (int j =0;j<7 ;j++ ) {
map[i][j] = 0;//将所有位置都变成0
}
}
// 把最上最下变成墙
for (int i=0; i<7; i++) {
map[0][i] = 1;
map[7][i] = 1;
}
// 把最左最右变成墙
for (int i=0; i<8; i++) {
map[i][0] = 1;
map[i][6] = 1;
}
// 制造路障
map[3][1]=1;
map[3][2]=1;
// 输出原始地图
System.out.println("\n======迷宫地图======\n");
for (int i = 0;i< 8; i++) {
for (int j =0;j<7 ;j++ ) {
System.out.print(map[i][j] + " ");
}
System.out.println();
}
T t1 = new T();
t1.findWay(map,1,1);//传入地图和初始位置为1,1
// 输出路线地图
System.out.println("\n======行走的地图======\n");
for (int i = 0;i< 8; i++) {
for (int j =0;j<7 ;j++ ) {
System.out.print(map[i][j] + " ");
}
System.out.println();
}
}
}
class T{
/*
定规则
1、0是可以走的,1是墙不能走,2是能走且走得通的,3是走过但是走不通
2、目的地的位置坐标为map[6][5],当map[6][5]=2的时候表示走通了
*/
public boolean findWay(int[][] map,int i ,int j){//接收地图和起始位置
if (map[6][5]==2) {//如果目标位置的值为2,就说明走通了
return true;
}else{
if (map[i][j] == 0) {
map[i][j]=2;//假设是能走通的,接着继续走,约定按照下下,右,上,左的方式去试探,这个先后不同,路线结果会不同
if (findWay(map,i+1,j)) {//下
return true;
}else if (findWay(map,i,j+1)) {//右
return true;
}else if (findWay(map,i-1,j)) {//上
return true;
}else if (findWay(map,i,j-1)) {//左
return true;
}else{
map[i][j]=3;//如果上下左右都走不通,说明这条路走不通,假设就不成立,标记当前为3
return false;
}
}else{
return false;//如果当前位置不为0,那就为1,2,3,其中2在上面内部也检测了,这里就为1或3,都是走不通的,返回false
}
}
}
}
运行效果
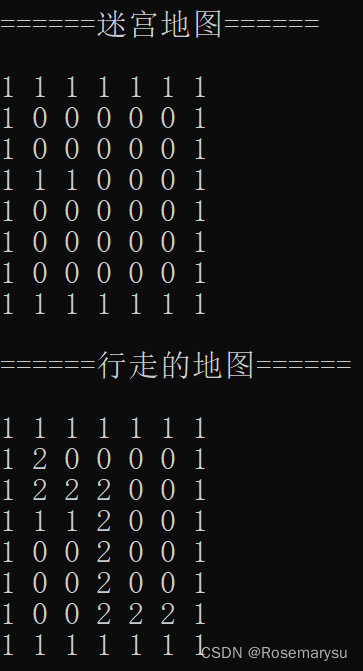