1.抽象接口的实现
public abstract class Pool {
public String propertiesName = "connection.properties";
private static Pool instance = null;
protected int maxConnect = 100;
protected int normalConnect = 10;
protected String driverName = null;
protected String username = null;
protected String password = null;
protected Driver driver = null;
protected String url = null;
protected Pool() {
initDbInfo();
loadDrivers(driverName);
}
protected void initDbInfo() {
InputStream inputStream = Pool.class.getClassLoader().getResourceAsStream(propertiesName);
Objects.requireNonNull(inputStream, "输入流数据为空");
Properties properties = new Properties();
try {
properties.load(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
this.driverName = properties.getProperty("driverName");
this.maxConnect = Integer.parseInt(properties.getProperty("maxConnect"));
this.normalConnect = Integer.parseInt(properties.getProperty("normalConnect"));
this.username = properties.getProperty("username");
this.password = properties.getProperty("password");
this.url = properties.getProperty("url");
}
private void loadDrivers(String driverName) {
String driverClassName = driverName;
try {
driver = (Driver) Class.forName(driverClassName).newInstance();
DriverManager.registerDriver(driver);
System.out.println("系统成功注册驱动程序");
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
} finally {
System.out.println("系统注册驱动异常");
}
}
public static synchronized Pool getInstance() throws InstantiationException, IllegalAccessException, ClassNotFoundException {
if (instance == null) {
instance.initDbInfo();
instance = (Pool) Class.forName("factory.Pool").newInstance();
}
return instance;
}
public abstract Connection getConnection();
public abstract Connection getConnection(long time);
public abstract void freeConnection(Connection connection);
public abstract int getNum();
public abstract int getNumActive();
public synchronized void realse() {
try {
DriverManager.deregisterDriver(driver);
System.out.println("连接驱动释放成功" + driver.getClass().getName());
} catch (SQLException e) {
e.printStackTrace();
System.out.println("连接释放异常");
}
}
}
2. 利用抽象接口创建数据库连接池
public final class DBConnectionPool extends Pool {
private int checkedOut;
private Vector<Connection> freeConnection = new Vector<Connection>();
private static int num = 0;
private static int numActive = 0;
private static DBConnectionPool pool = null;
public static synchronized DBConnectionPool getInstance() {
if (pool == null) {
pool = new DBConnectionPool();
}
return pool;
}
private DBConnectionPool() {
initDbInfo();
for (int i = 0; i < normalConnect; i++) {
Connection connection = newConnection();
if (connection != null) {
freeConnection.addElement(connection);
num++;
}
}
}
public synchronized Connection getConnection() {
Connection connection = null;
if (freeConnection.size() > 0) {
num--;
connection = freeConnection.firstElement();
freeConnection.removeElementAt(0);
try {
if (connection.isClosed()) {
System.out.println("从连接池中取出的是一个无效的连接");
connection = getConnection();
}
} catch (SQLException e) {
e.printStackTrace();
System.out.println("删除一个无效的连接");
}
} else if (maxConnect == 0 || checkedOut < maxConnect) {
connection = newConnection();
}
if (connection != null) {
checkedOut++;
}
numActive++;
return connection;
}
public synchronized Connection getConnection(long time) {
long startTime = new Date().getTime();
Connection connection = null;
while ((connection = getConnection()) == null) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
if (new Date().getTime() - startTime >= time) {
return null;
}
}
return connection;
}
public void freeConnection(Connection connection) {
freeConnection.add(connection);
num++;
numActive--;
checkedOut--;
notifyAll();
}
public int getNum() {
return num;
}
public int getNumActive() {
return numActive;
}
private Connection newConnection() {
Connection connection = null;
try {
if (username == null) {
connection = DriverManager.getConnection(url);
} else {
connection = DriverManager.getConnection(url, username, password);
}
System.out.println("连接池创建一个新的连接");
} catch (SQLException e) {
e.printStackTrace();
System.out.println("创建连接失败");
} finally {
}
return connection;
}
public synchronized void realse() {
Enumeration allConnection = freeConnection.elements();
while (allConnection.hasMoreElements()) {
Connection connection = null;
connection = (Connection) allConnection.nextElement();
try {
connection.close();
num--;
} catch (SQLException e) {
System.out.println("无法关闭连接池中的连接");
}
freeConnection.removeAllElements();
numActive = 0;
}
super.realse();
}
}
3.配置文件 : connection.properties
url=jdbc:mysql://localhost:3306/MUSICDB
username=root
password=数据库的密码
maxConnect=100
normalConnect =10
driverName=com.mysql.cj.jdbc.Driver
4.使用测试:
Pool pool = DBConnectionPool.getInstance();
Connection connection = pool.getConnection(2000);
Objects.requireNonNull(connection , "获取连接对象为空");
System.out.println("当前连接池中空闲的连接对象 = " + pool.getNum());
System.out.println("正在使用的连接的数量 = " + pool.getNumActive());
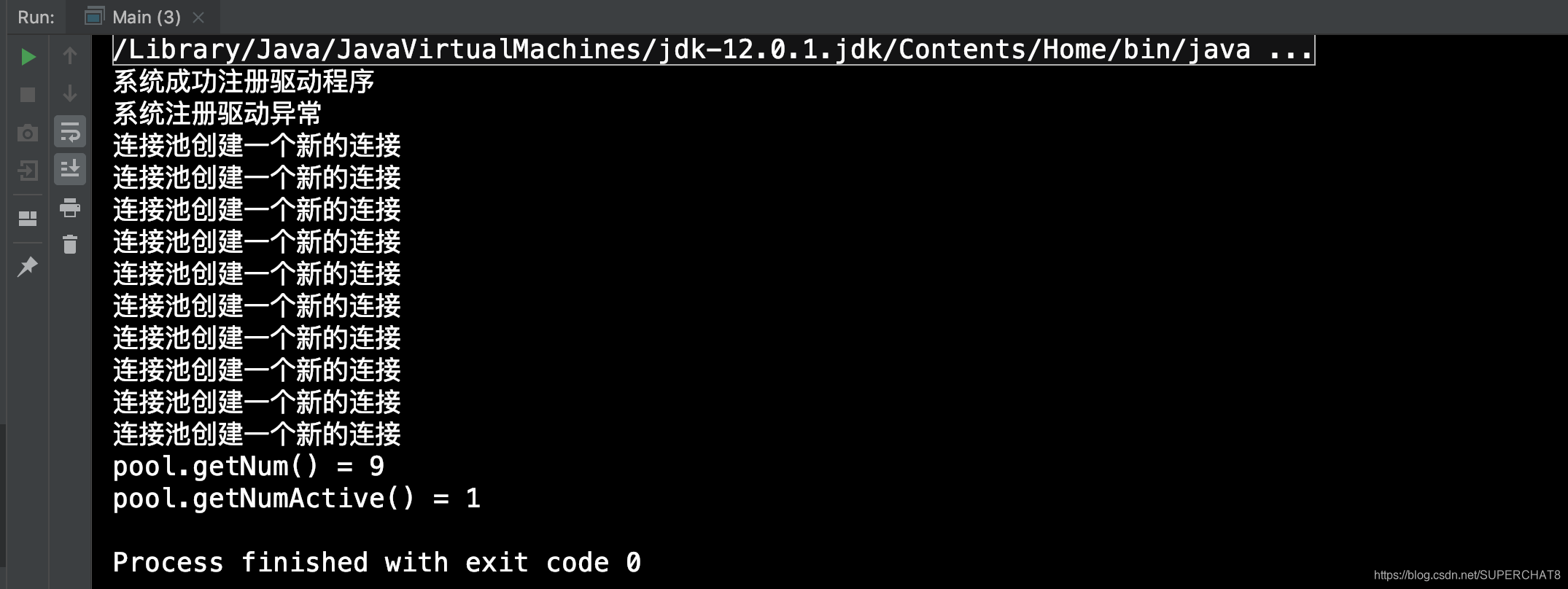