#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
struct Teacher
{
char * name;
char ** Students;
};
void allocateSpace(struct Teacher *** teachers)
{
struct Teacher ** pArray = malloc(sizeof(struct Teacher *) * 3);
for (int i = 0; i < 3; i++)
{
pArray[i] = malloc(sizeof(struct Teacher));
pArray[i]->name = malloc(sizeof(char) * 64);
sprintf(pArray[i]->name, "Teacher_%d", i + 1);
pArray[i]->Students = malloc(sizeof(char *) * 4);
for (int j = 0; j < 4; j++)
{
pArray[i]->Students[j] = malloc(sizeof(char) * 64);
sprintf(pArray[i]->Students[j], "%s_Student_%d", pArray[i]->name, j + 1);
}
}
*teachers = pArray;
}
void showArray(struct Teacher ** pArray, int len)
{
for (int i = 0; i < len; i++)
{
printf("%s\n", pArray[i]->name);
for (int j = 0; j < 4; j++)
{
printf(" %s\n", pArray[i]->Students[j]);
}
}
}
void freeSpace(struct Teacher ** pArray, int len)
{
for (int i = 0; i < len; i++)
{
if (pArray[i]->name != NULL)
{
free(pArray[i]->name);
pArray[i]->name = NULL;
}
for (int j = 0; j < 4; j++)
{
if (pArray[i]->Students[j] != NULL)
{
free(pArray[i]->Students[j]);
pArray[i]->Students[j] = NULL;
}
}
free(pArray[i]->Students);
pArray[i]->Students = NULL;
free(pArray[i]);
pArray[i] = NULL;
}
free(pArray);
pArray = NULL;
}
void test01()
{
struct Teacher ** pArray = NULL;
allocateSpace(&pArray);
showArray(pArray, 3);
freeSpace(pArray, 3);
pArray = NULL;
}
int main() {
test01();
system("pause");
return EXIT_SUCCESS;
}
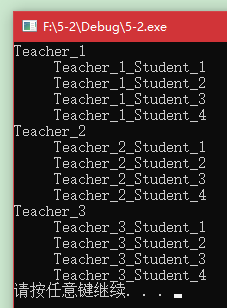
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
struct Tearcher
{
char *name;
char **student;
};
struct Tearcher ** allocateSpace()
{
struct Tearcher** pArray = malloc(sizeof(struct Tearcher*) * 3);
for (int i = 0; i < 3; i++)
{
pArray[i] = malloc(sizeof(struct Tearcher));
pArray[i]->name = malloc(sizeof(char) * 64);
sprintf(pArray[i]->name, "Teacher_%d", i + 1);
pArray[i]->student = malloc(sizeof(char*) * 4);
for (int j = 0; j < 4; j++)
{
pArray[i]->student[j] = malloc(sizeof(char) * 64);
sprintf(pArray[i]->student[j], "Teacher_%d_Student_%d", i+1, j+1);
}
}
return pArray;
}
void printArray(struct Tearcher ** pArray, int len)
{
for (int i = 0; i < len; i++)
{
printf("老师姓名: %s\n", pArray[i]->name);
for (int j = 0; j < 4; j++)
{
printf("学生姓名: %s\n", pArray[i]->student[j]);
}
printf("\n");
}
}
void freeSpace(struct Tearcher ** pArray, int len)
{
for (int i = 0; i < len; i++)
{
if (pArray[i]->name != NULL)
{
free(pArray[i]->name);
pArray[i]->name = NULL;
}
}
for (int i = 0; i < len; i++)
{
for (int j = 0; j < 4; j++)
{
if (pArray[i]->student[j] != NULL)
{
free(pArray[i]->student[j]);
pArray[i]->student[j] = NULL;
}
}
if (pArray[i]->student != NULL)
{
free(pArray[i]->student);
pArray[i]->student = NULL;
}
}
if (pArray != NULL)
{
free(pArray);
pArray = NULL;
}
}
void test01()
{
struct Tearcher ** pArray = NULL;
pArray = allocateSpace();
printArray(pArray, 3);
freeSpace(pArray, 3);
pArray = NULL;
}
int main() {
test01();
system("pause");
return 0;
}
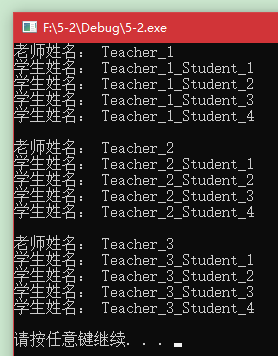