-
线程方法
方法 说明 setPriority(int newPriority) 更改线程的优先级 static void sleep(long millis) 在指定的毫秒数内让当前正在执行的线程休眠 void join() 等待该线程终止 static void yield() 暂停当前正在执行的线程对象,并执行其它线程 void interrupt() 中断线程,别用这个方式 boolean isAlive() 测试线程是否处于活动状态 -
停止线程
- 不推荐使用JDK提供的stop()、destroy()方法【已废弃】
- 推荐线程自己停止下来
- 建议使用一个标志位进行终止变量,当flag=false,则终止线程运行
//测试stop //1.建议线程正常停止 ---> 利用次数,不建议死循环 //2.建议使用标志位 ---> 设置一个标志位 //3.不要使用stop或者destroy等过时或者jdk不建议使用的方法 public class TestStop implements Runnable{ //1.设置一个标志位 private boolean flag = true; @Override public void run(){ int i = 0; while(flag){ System.out.println("run...Thread" + i++); } } //2.设置一个公开的方法停止线程,转换标志位 public void stop(){ this.flag = false; } public static void main(String[] args){ TestStop testStop = new TestStop(); new Thread(testStop).start(); for(int i = 0; i < 1000; i++){ System.out.println("main...Thread" + i); if(i==900){ //调用stop方法切换标志位,让线程停止 testStop.stop(); System.out.println("线程停止了"); } } } }
-
线程休眠
- sleep(时间)指定当前线程阻塞的豪秒数
- sleep存在异常InterruptedException
- sleep时间达到后线程进入就绪状态
- sleep可以模拟网络延时,倒计时等
- 每一个对象都有一个锁,sleep不会释放锁
//模拟倒计时 public class TestSleep{ public void tenDown() throws InterruptedException{ int num = 10; while(true){ Thread.sleep(1000); System.out.println(num--); if(num <= 0){ break; } } } public static void main(String[] args){ TestSleep testSleep = new TestSleep(); try{ testSleep.tenDown(); }catch(InterruptedException e){ e.printStackTrace(); } } }
-
线程礼让
- 让当前正在执行的线程暂停,但不阻塞
- 将线程从运行状态转为就绪状态
- 让cpu重新调度,礼让不一定成功
//测试礼让线程:不一定成功 public class TestYield{ public static void main(String[] args){ MyYield myYield = new MyYield(); new Thread(myYield,"A").start(); new Thread(myYield,"B").start(); } } class MyYield implements Runnable{ @Override public void run(){ System.out.println(Thread.currentThread().getName()+"线程开始执行"); Thread.yield(); //礼让 System.out.println(Thread.currentThread().getName()+"线程停止执行"); } }
-
Join
- join合并线程,待此线程执行完成后,再执行其他线程,其他线程阻塞
- 可以想象成插队
//测试join方法,插队 public class TestJoin implements Runnable{ @Override public void run(){ for(int i = 0; i < 1000; i++){ System.out.println("线程vip来了" + i); } } public static void main(String[] args) throws InterruptedException{ //启动线程 TestJoin testJoin = new TestJoin(); Thread thread = new Thread(testJoin); thread.start(); //主线程 for(int i = 0; i < 1000; i++){ if(i == 200){ thread.join(); //插队 } System.out.println("main方法" + i); } } }
-
观测线程状态
- NEW:尚未启动的线程处于此状态
- RUNNABLE:在Java虚拟机中执行的线程处于此状态
- BLOCKED:被阻塞等待监视器锁定的线程处于此状态
- WAITING:正在等待另一个线程执行特定动作的线程处于此状态
- TIMED_WAITING:正在等待另一个线程执行动作达到指定等待时间的线程处于此状态
- TERMINATED:已退出的线程处于此状态
- 一个线程可以在给定时间点处于一个状态,这些状态是不反映任何操作系统线程状态的虚拟机状态
//观察测试线程的状态 public class TestState{ public static void main(String[] args) throws InterruptedException{ Thread thread = new Thread(()->{ for(int i = 0; i < 5; i++){ try{ Thread.sleep(100); }catch(InterruptedException e) { e.printStackTrace(); } } System.out.println("---------"); }); //观察状态 Thread.State state = thread.getState(); System.out.println(state); //NEW //观察启动后 thread.start(); //启动线程 state = thread.getState(); System.out.println(state); //RUNNABLE while(state != Thread.State.TERMINATED){ //只要线程不终止,就一直输出状态 Thread.sleep(100); state = thread.getState(); //更新线程状态 System.out.println(state); //输出状态 } } } /* NEW RUNNABLE TIMED_WAITING TIMED_WAITING TIMED_WAITING TIMED_WAITING TIMED_WAITING --------- TERMINATED */
-
线程优先级
-
Java提供一个线程调度器来监控程序中启动后进入就绪状态的所有的线程,线程调度器按照优先级决定应该调度哪个线程来执行
-
线程的优先级用数字来表示,范围从1~10
- Thread.MIN_PRORITY = 1;
- Thread.MAX_PRORITY = 10;
- Thread.NORM_PRORITY = 5;
-
使用一下方式改变或获得优先级
- getPriority()
- setPriority(int xxx)
-
优先级低只是意味着获得调度的概率低,并不是优先级低就不会被调用了,还是得看cpu的调度
//测试线程的优先级 public class TestPriority extends Thread{ public static void main(String[] args) { //主线程默认优先级 System.out.println(Thread.currentThread().getName() + "--->" + Thread.currentThread().getPriority()); MyPriority myPriority = new MyPriority(); Thread t1 = new Thread(myPriority); Thread t2 = new Thread(myPriority); Thread t3 = new Thread(myPriority); Thread t4 = new Thread(myPriority); Thread t5 = new Thread(myPriority); Thread t6 = new Thread(myPriority); //先设置优先级,,再启动 t1.start(); t2.setPriority(1); t2.start(); t3.setPriority(4); t3.start(); t4.setPriority(Thread.MAX_PRIORITY); //MAX_PRIORITY=10 t4.start(); /* t5.setPriority(-1); //报错 t5.start(); t6.setPriority(11); //报错 t6.start(); */ } } class MyPriority implements Runnable{ @Override public void run() { System.out.println(Thread.currentThread().getName() + "--->" + Thread.currentThread().getPriority()); } }
-
-
守护(daemon)线程
- 线程分为用户线程和守护线程
- 虚拟机必须确保用户线程执行完毕
- 虚拟机不用等待守护线程执行完毕
//测试守护线程 //上帝守护你 public class TestDaemon{ public static void main(String[] args){ God god = new God(); You you = new You(); Thread thread = new Thread(god); thread.setDaemon(true); //默认是false表示是用户线程,正常的线程都是用户线程 thread.start(); //上帝守护线程启动 new Thread(you).start(); //你 } } //上帝 class God implements Runnable{ @Override public void run(){ while(true){ //人的一生36500天 System.out.println("上帝保佑着你"); } } } //你 class You implements Runnable{ @Override public void run(){ for(int i = 0; i < 36500; i++){ //人的一生36500天 System.out.println("你这一生都很开心的活着"); } System.out.println("=====goodbye====="); } }
线程状态
最新推荐文章于 2023-07-10 10:30:00 发布
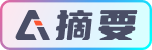