栈的介绍
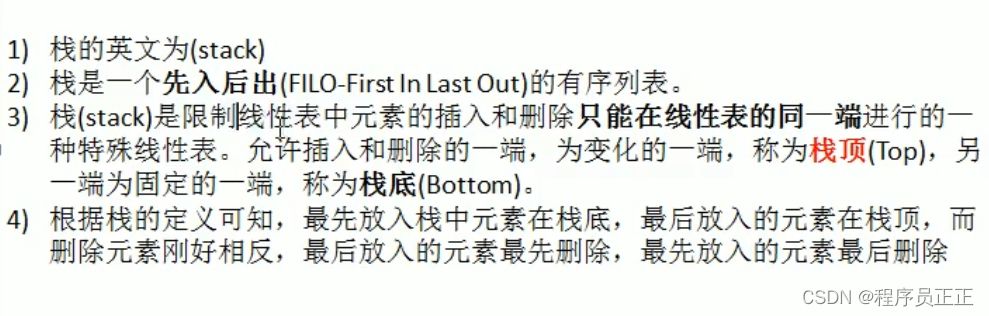
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-l2mMhRzC-1646979355507)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220309211915242.png)]](https://i-blog.csdnimg.cn/blog_migrate/71733bea257ad50bc3fa013dcda6a820.png)
栈的应用场景
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-io3OoRLe-1646979355509)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220309212210377.png)]](https://i-blog.csdnimg.cn/blog_migrate/c68a1aa5ac130765e6067581c9b29234.png)
数组模拟栈的思路分析
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-fkRExP2L-1646979355509)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220309212705303.png)]](https://i-blog.csdnimg.cn/blog_migrate/34e11b50505cbab888917f682e62da1b.png)
代码实现
package com.xz.stack;
import java.util.Scanner;
public class ArrayStackDemo {
public static void main(String[] args) {
ArrayStack stack = new ArrayStack(4);
String key = "";
boolean loop = true;
Scanner scanner = new Scanner(System.in);
while (loop) {
System.out.println("show: 表示显示栈");
System.out.println("exit: 退出程序");
System.out.println("push: 表示添加数据到栈(入栈)");
System.out.println("pop: 表示从栈取出数据(出栈)");
System.out.println("请输入你的选择");
key = scanner.next();
switch (key) {
case "show":
stack.list();
break;
case "push":
System.out.println("请输入一个数");
int value = scanner.nextInt();
stack.push(value);
break;
case "pop":
try {
int res = stack.pop();
System.out.printf("出栈的数据是 %d\n", res);
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "exit":
scanner.close();
loop = false;
break;
default:
break;
}
}
System.out.println("程序退出~~~");
}
}
class ArrayStack {
private int maxSize;
private int[] stack;
private int top = -1;
public ArrayStack(int maxSize) {
this.maxSize = maxSize;
stack = new int[this.maxSize];
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
public void push(int value) {
if (isFull()) {
System.out.println("栈满");
return;
}
top++;
stack[top] = value;
}
public int pop() {
if (isEmpty()) {
throw new RuntimeException("栈空,没有数据~");
}
int value = stack[top];
top--;
return value;
}
public void list() {
if (isEmpty()) {
System.out.println("栈空,没有数据~~");
return;
}
for (int i = top; i >= 0; i--) {
System.out.printf("stack[%d]=%d\n", i, stack[i]);
}
}
}
关于栈的三种表达式
前缀表达式(波兰表达式)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-zoLrTlXk-1646979355510)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310110146657.png)]](https://i-blog.csdnimg.cn/blog_migrate/aeca49cb51b97a77c8cf1b53a5887061.png)
中缀表达式
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-GrZktYP1-1646979355510)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310110433571.png)]](https://i-blog.csdnimg.cn/blog_migrate/e4ef23ceb9ebffabd482e25a95c777fb.png)
后缀表达式
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7sxOC4n8-1646979355511)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310110525264.png)]](https://i-blog.csdnimg.cn/blog_migrate/a8d671ecda06c33dd8ec9539f2078845.png)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-gBlb4Bjl-1646979355511)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310110724472.png)]](https://i-blog.csdnimg.cn/blog_migrate/c9ea177cdd93d0fb23fa8e479a448cf3.png)
逆波兰表达式完成计算器
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-p5cER4tH-1646979355512)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310110845330.png)]](https://i-blog.csdnimg.cn/blog_migrate/75d2131288997590d02277f98dff2d2a.png)
中缀表达式转后缀表达式
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-8ZCURXlj-1646979355513)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310172303023.png)]](https://i-blog.csdnimg.cn/blog_migrate/c3f61b56e59b21ecf4781373093e6b26.png)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-sPbKQzVn-1646979355514)(C:\Users\许正\AppData\Roaming\Typora\typora-user-images\image-20220310172437603.png)]](https://i-blog.csdnimg.cn/blog_migrate/07782550319cdd72168cdf287edacd95.png)
完整版逆波兰计算器代码实现
package com.atguigu.reversepolishcal;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Stack;
import java.util.regex.Pattern;
public class ReversePolishMultiCalc {
static final String SYMBOL = "\\+|-|\\*|/|\\(|\\)";
static final String LEFT = "(";
static final String RIGHT = ")";
static final String ADD = "+";
static final String MINUS= "-";
static final String TIMES = "*";
static final String DIVISION = "/";
static final int LEVEL_01 = 1;
static final int LEVEL_02 = 2;
static final int LEVEL_HIGH = Integer.MAX_VALUE;
static Stack<String> stack = new Stack<>();
static List<String> data = Collections.synchronizedList(new ArrayList<String>());
public static String replaceAllBlank(String s ){
return s.replaceAll("\\s+","");
}
public static boolean isNumber(String s){
Pattern pattern = Pattern.compile("^[-\\+]?[.\\d]*$");
return pattern.matcher(s).matches();
}
public static boolean isSymbol(String s){
return s.matches(SYMBOL);
}
public static int calcLevel(String s){
if("+".equals(s) || "-".equals(s)){
return LEVEL_01;
} else if("*".equals(s) || "/".equals(s)){
return LEVEL_02;
}
return LEVEL_HIGH;
}
public static List<String> doMatch (String s) throws Exception{
if(s == null || "".equals(s.trim())) throw new RuntimeException("data is empty");
if(!isNumber(s.charAt(0)+"")) throw new RuntimeException("data illeagle,start not with a number");
s = replaceAllBlank(s);
String each;
int start = 0;
for (int i = 0; i < s.length(); i++) {
if(isSymbol(s.charAt(i)+"")){
each = s.charAt(i)+"";
if(stack.isEmpty() || LEFT.equals(each)
|| ((calcLevel(each) > calcLevel(stack.peek())) && calcLevel(each) < LEVEL_HIGH)){
stack.push(each);
}else if( !stack.isEmpty() && calcLevel(each) <= calcLevel(stack.peek())){
while (!stack.isEmpty() && calcLevel(each) <= calcLevel(stack.peek()) ){
if(calcLevel(stack.peek()) == LEVEL_HIGH){
break;
}
data.add(stack.pop());
}
stack.push(each);
}else if(RIGHT.equals(each)){
while (!stack.isEmpty() && LEVEL_HIGH >= calcLevel(stack.peek())){
if(LEVEL_HIGH == calcLevel(stack.peek())){
stack.pop();
break;
}
data.add(stack.pop());
}
}
start = i ;
}else if( i == s.length()-1 || isSymbol(s.charAt(i+1)+"") ){
each = start == 0 ? s.substring(start,i+1) : s.substring(start+1,i+1);
if(isNumber(each)) {
data.add(each);
continue;
}
throw new RuntimeException("data not match number");
}
}
Collections.reverse(stack);
data.addAll(new ArrayList<>(stack));
System.out.println(data);
return data;
}
public static Double doCalc(List<String> list){
Double d = 0d;
if(list == null || list.isEmpty()){
return null;
}
if (list.size() == 1){
System.out.println(list);
d = Double.valueOf(list.get(0));
return d;
}
ArrayList<String> list1 = new ArrayList<>();
for (int i = 0; i < list.size(); i++) {
list1.add(list.get(i));
if(isSymbol(list.get(i))){
Double d1 = doTheMath(list.get(i - 2), list.get(i - 1), list.get(i));
list1.remove(i);
list1.remove(i-1);
list1.set(i-2,d1+"");
list1.addAll(list.subList(i+1,list.size()));
break;
}
}
doCalc(list1);
return d;
}
public static Double doTheMath(String s1,String s2,String symbol){
Double result ;
switch (symbol){
case ADD : result = Double.valueOf(s1) + Double.valueOf(s2); break;
case MINUS : result = Double.valueOf(s1) - Double.valueOf(s2); break;
case TIMES : result = Double.valueOf(s1) * Double.valueOf(s2); break;
case DIVISION : result = Double.valueOf(s1) / Double.valueOf(s2); break;
default : result = null;
}
return result;
}
public static void main(String[] args) {
String math = "12.8 + (2 - 3.55)*4+10/5.0";
try {
doCalc(doMatch(math));
} catch (Exception e) {
e.printStackTrace();
}
}
}