maven写的登录 注册 原生代码
一级目录
先导入bean实体类。
下面展示一些 内联代码片
。
// A code block
var foo = 'bar';
// An highlighted block
package com.briup.estore.entity;
import java.io.Serializable;
/**
* 顾客类
*/
public class Customer implements Serializable{
private static final long serialVersionUID = 1L;
private Integer id;
private String name;
private String password;
private String zipCode;
private String address;
private String telephone;
private String email;
public Customer() {}
public Customer(String name, String password, String zipCode, String address, String telephone, String email) {
this.name = name;
this.password = password;
this.zipCode = zipCode;
this.address = address;
this.telephone = telephone;
this.email = email;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getZipCode() {
return zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getTelephone() {
return telephone;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "Customer [id=" + id + ", name=" + name + ", password=" + password + ", zipCode=" + zipCode
+ ", address=" + address + ", telephone=" + telephone + ", email=" + email + "]";
}
}
web层(servlet filter listener)
先写 servlet层
下面展示一些 内联代码片
。
// A code block
var foo = 'bar';
// An highlighted block
package com.briup.estore.web.servlet;
import com.briup.estore.entity.Customer;
import com.briup.estore.service.ICustomerService;
import com.briup.estore.service.impl.CustomerServiceImpl;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
/**
* @Auther: vanse(lc))
* @Date: 2022/6/27-06-27-11:10
* @Description:ecom.briup.estore.web.servlet
*/
@WebServlet("/customerLogin")
public class CustomerLoginServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 1.接收参数
String name = req.getParameter("name");
String password = req.getParameter("password");
// 2.调用service 并传参
ICustomerService customerService = new CustomerServiceImpl();
// 3.调用login方法
HttpSession session = req.getSession();
try {
Customer customer = customerService.login(name,password);
// 4.响应 成功 -> 首页 失败->登录
// 将登录成功的用户存到session
session.setAttribute("customer",customer);
resp.sendRedirect("index.jsp");
}catch (Exception e){
e.printStackTrace(); // 打印异常
// 请求转发 request.setAttribute
session.setAttribute("error",e.getMessage());
resp.sendRedirect("login.jsp");
}
}
}
图片
图片: 在这里插入图片描述 {
if(sqlSessionFactory==null) {
InputStream inputStream = null;
try {
inputStream = Resources.getResourceAsStream("mybatis-config.xml");
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
return sqlSessionFactory;
}
public static SqlSession openSession() {
return openSession(false);
}
public static SqlSession openSession(boolean autoCommit) {
return getSqlSessionFactory().openSession(autoCommit);
}
}
下面展示一些 内联代码片
。
// maven 依赖
// An highlighted block
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.briup</groupId>
<artifactId>estore-qidi</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<!-- 引入servlet3.1的依赖 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- 引入jstl的依赖,jsp页面中会使用到
<c:foreach>
-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- 引入Mybatis的依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.6</version>
</dependency>
<dependency>
<groupId>com.oracle.ojdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>19.3.0.0</version>
</dependency>
<!--
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.38</version>
</dependency>
-->
<!-- 日志框架log4j的依赖 -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<!--测试单元-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<!--集成沙箱支付-->
<dependency>
<groupId>com.alipay.sdk</groupId>
<artifactId>alipay-sdk-java</artifactId>
<version>4.8.10.ALL</version>
</dependency>
<!--json ajax 异步传输 前后端交互数据-->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.11.3</version>
</dependency>
<!--工具集-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.3</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
</dependencies>
</project>
下面展示一些 内联代码片
。
// service
// An highlighted block
package com.briup.estore.service.impl;
import com.briup.estore.dao.CustomerDao;
import com.briup.estore.entity.Customer;
import com.briup.estore.service.ICustomerService;
import com.briup.estore.utils.MD5Util;
import com.briup.estore.utils.MyBatisSqlSessionFactory;
import org.apache.ibatis.session.SqlSession;
import javax.management.RuntimeErrorException;
public class CustomerServiceImpl implements ICustomerService {
SqlSession session = MyBatisSqlSessionFactory.openSession();
CustomerDao customerDao = session.getMapper(CustomerDao.class);
/**
* 注册用户
* @param customer 顾客对象 页面表单传值
*/
@Override
public void register(Customer customer) {
String name =customer.getName();
String email = customer.getEmail();
String telephone = customer.getTelephone();
// 1.判断名字是否为空 空就抛出异常 字符串 == 判断地址 "000"
if (name==null||"".equals(name)){
// 终止程序运行
throw new RuntimeException("用户名不能为空");
}
if (email==null||"".equals(email)){
// 终止程序运行
throw new RuntimeException("邮箱不能为空");
}
if (telephone == null||"".equals(telephone)){
throw new RuntimeException("手机号码不能为空");
}
//2 判断数据库是否有这个名
// 根据从数据库查的对象
Customer customerFromDB= customerDao.findByName(name);
if (customerFromDB!=null){
// 数据库有该名字了
throw new RuntimeException("用户名已占用");
}
// 3.正常注册
// 密码加密 明文转密文
customer.setPassword(MD5Util.encode(customer.getPassword()));
customerDao.register(customer);
session.commit();
}
/**
* 登录方法
* @param name 用户名
* @param password 密码
* @return 返回成功的登录对象
*/
@Override
public Customer login(String name, String password) {
// 根据用户名查对象 如果查不到 账户名错了
Customer customerFromDB=customerDao.findByName(name);
if (customerFromDB==null){
//查不到用户
throw new RuntimeException("用户名有误");
}
// 如果查到了 比对密码 此时比对的是密文
if (!MD5Util.encode(password).equals(customerFromDB.getPassword())){
// 名字对 密码错
throw new RuntimeException("密码有误");
}
// 此时说明登录成功 select * from es_customer
// where name=#{name} and password=#{password}
return customerFromDB;
}
}
下面展示一些 内联代码片
。
// serive 接口
// An highlighted block
package com.briup.estore.service;
import com.briup.estore.entity.Customer;
public interface ICustomerService {
void register(Customer customer); // 注册方法
Customer login(String name, String password); // 登录方法
}
下面展示一些 内联代码片
。
// 注册的servlet
// An highlighted block
package com.briup.estore.web.servlet;
import com.briup.estore.entity.Customer;
import com.briup.estore.service.ICustomerService;
import com.briup.estore.service.impl.CustomerServiceImpl;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/userRegister")
public class CustomerRegisterServlet extends HttpServlet {
// doPost 一般: 插入数据(三个或者以上的参数 post)
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
super.doGet(req, resp);
}
// doPost 一般: 插入数据(三个或者以上的参数 post)
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("UTF-8");
resp.setCharacterEncoding("UTF-8");
// 1.接收参数 6 BeanUtil
String name = req.getParameter("name");
String password = req.getParameter("password");
String zipCode = req.getParameter("zipCode");
String address = req.getParameter("address");
String telephone = req.getParameter("telephone");
String email = req.getParameter("email");
// 2.将参数封装成Customer对象
Customer customer= new Customer(name,password,zipCode,address,telephone,email);
// 3.调用service做业务校验 并传递参数 (左边是接口 右边是实现类)
ICustomerService customerService= new CustomerServiceImpl();
// 4.根据业务执行 根据servcie是否异常做响应
try {
customerService.register(customer);
// 4.1 成功了 跳转到登录页面
resp.sendRedirect("login.jsp");
}catch (Exception e){
e.printStackTrace(); // 打印异常
// 4.2 失败了 继续注册 并携带错误信息
// e.getMessage => new RuntimeException(msg);
req.getSession().setAttribute("error",e.getMessage());
resp.sendRedirect("register.jsp");
}
}
}
图片: 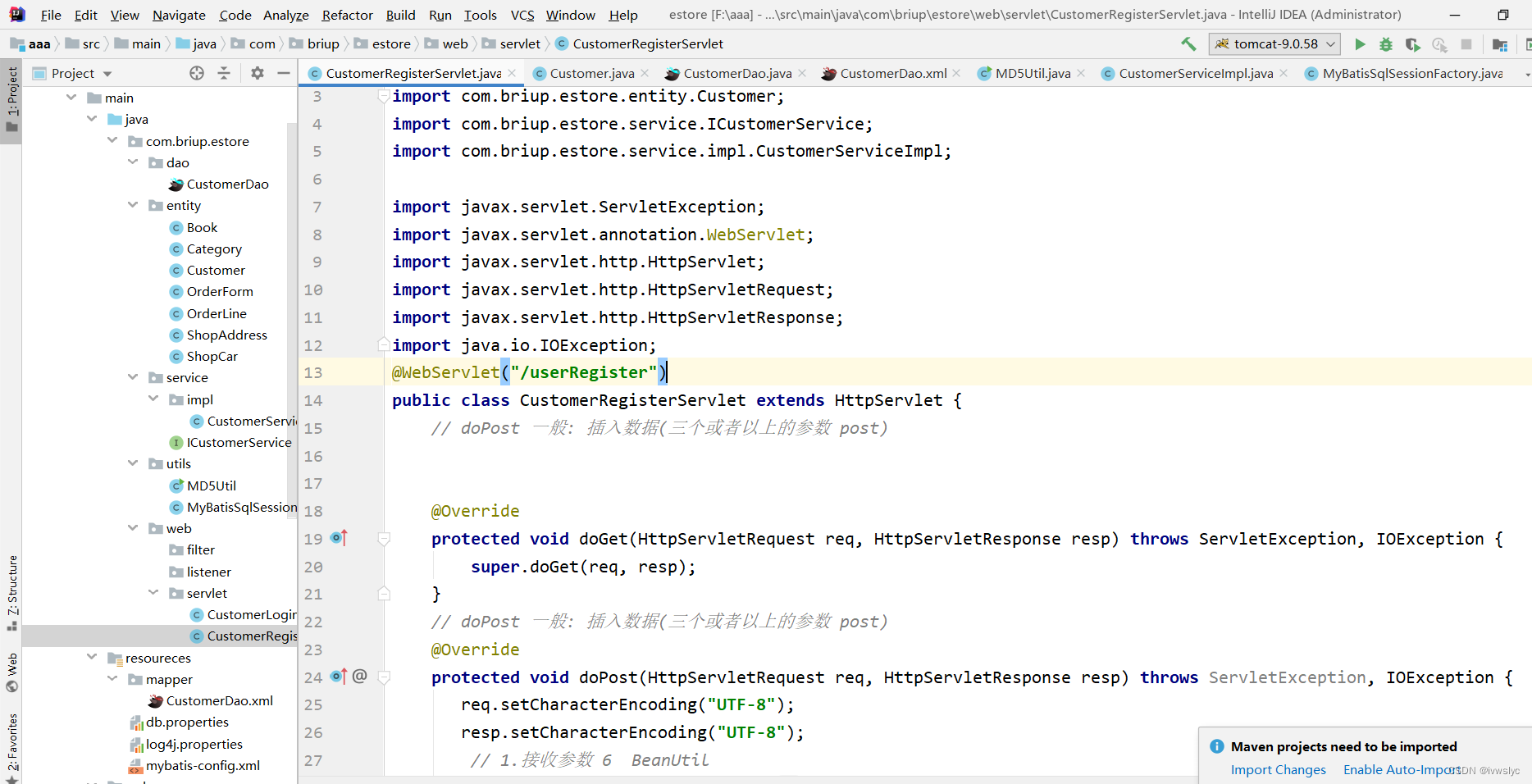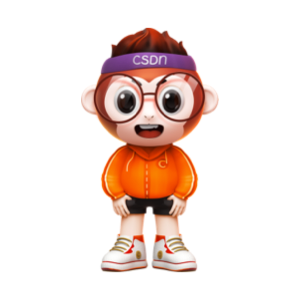
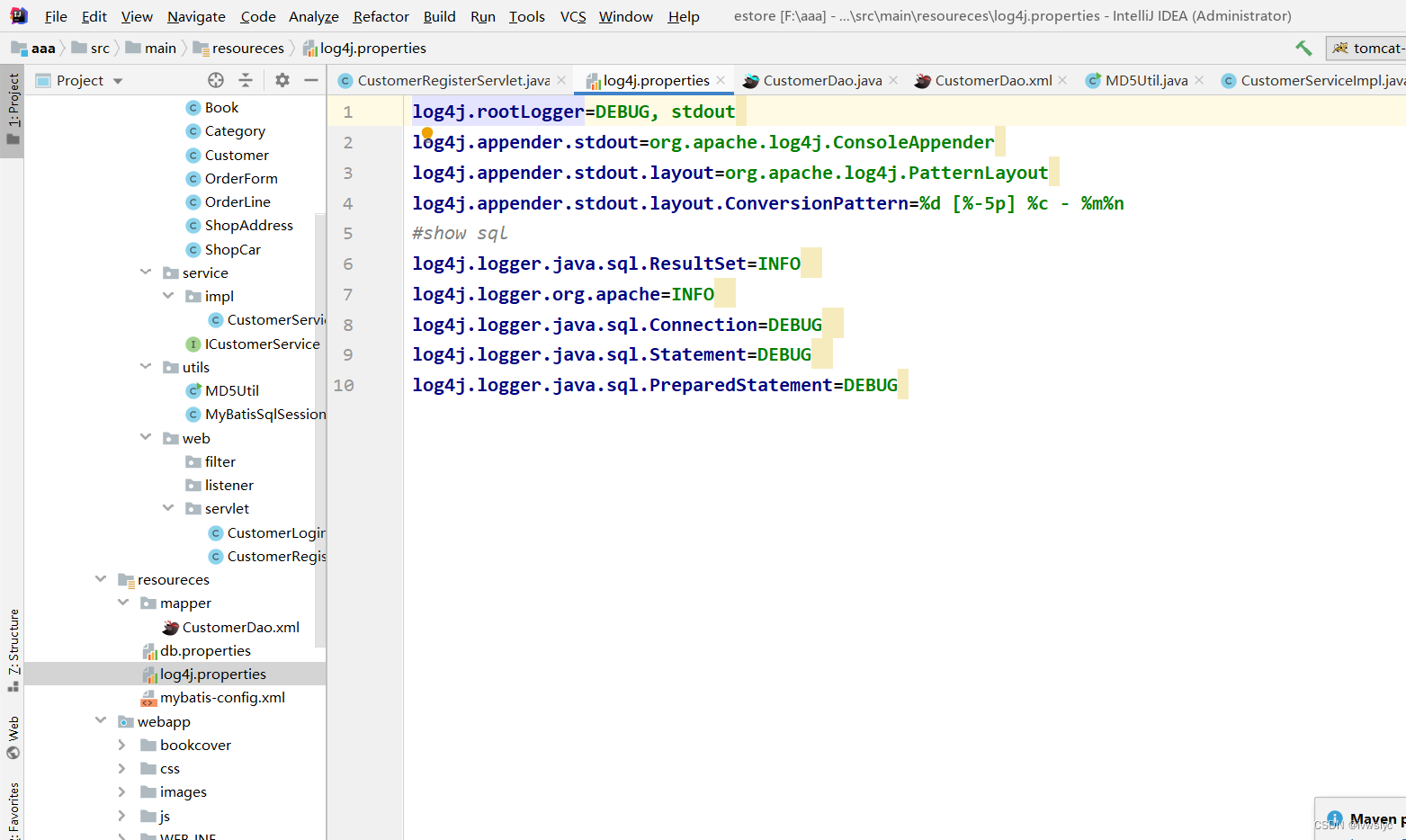
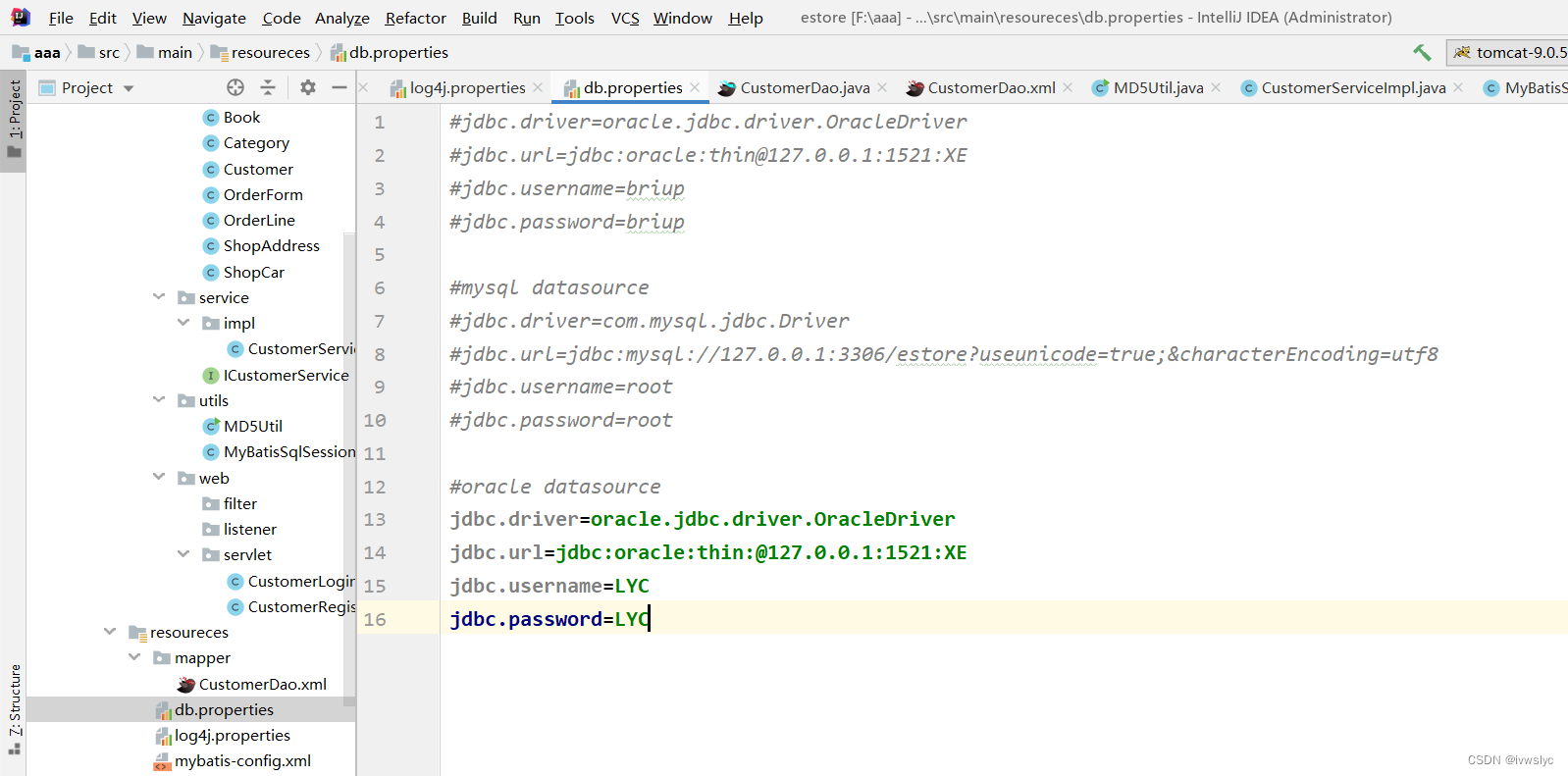
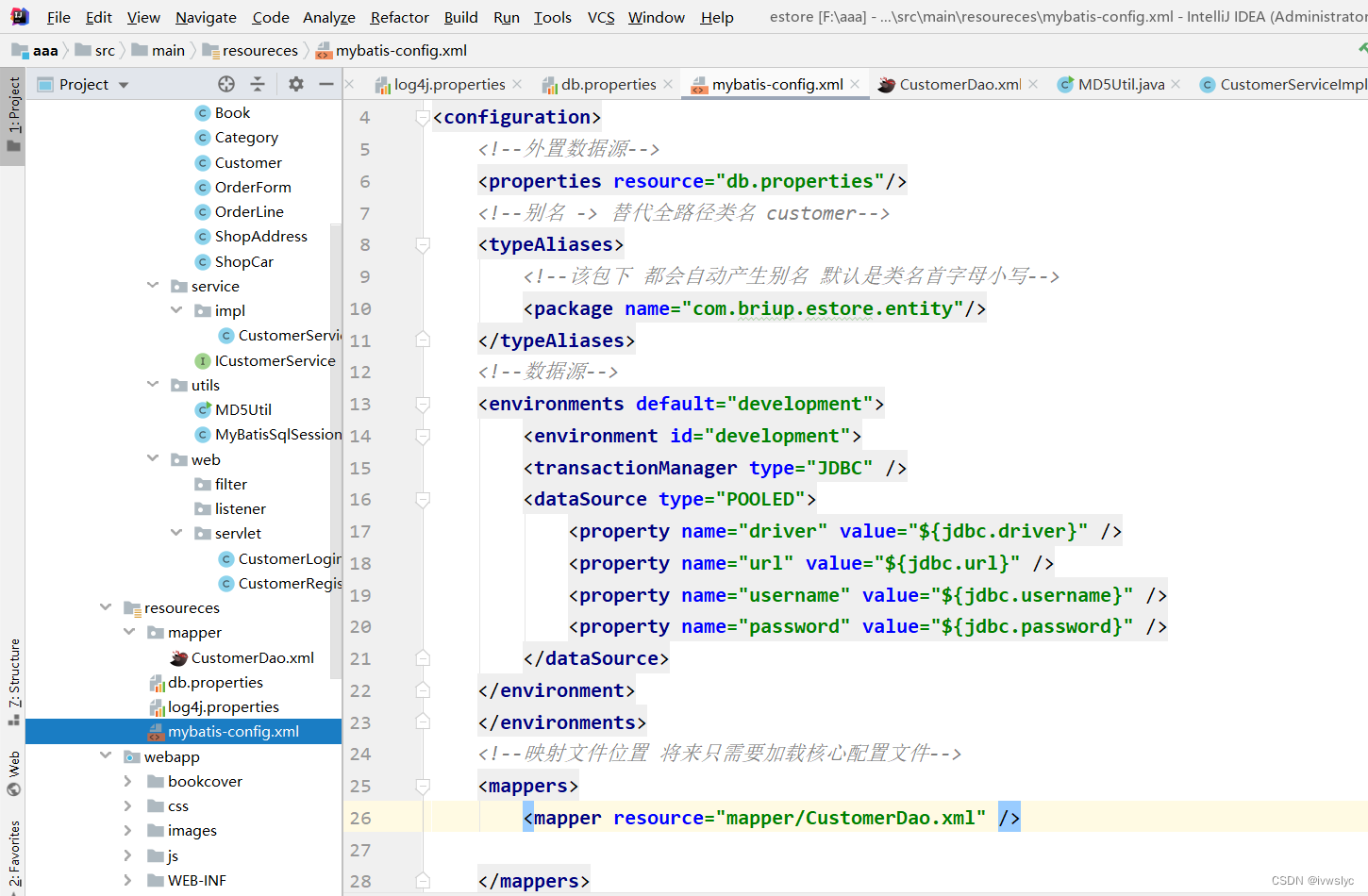