element-plus Dialog 对话框改手机预览组件,方便后台管理系统部分手机预览功能样式,手机内主体部分使用插槽,可自行编写html结构,也可使用iframe引入页面
效果预览:
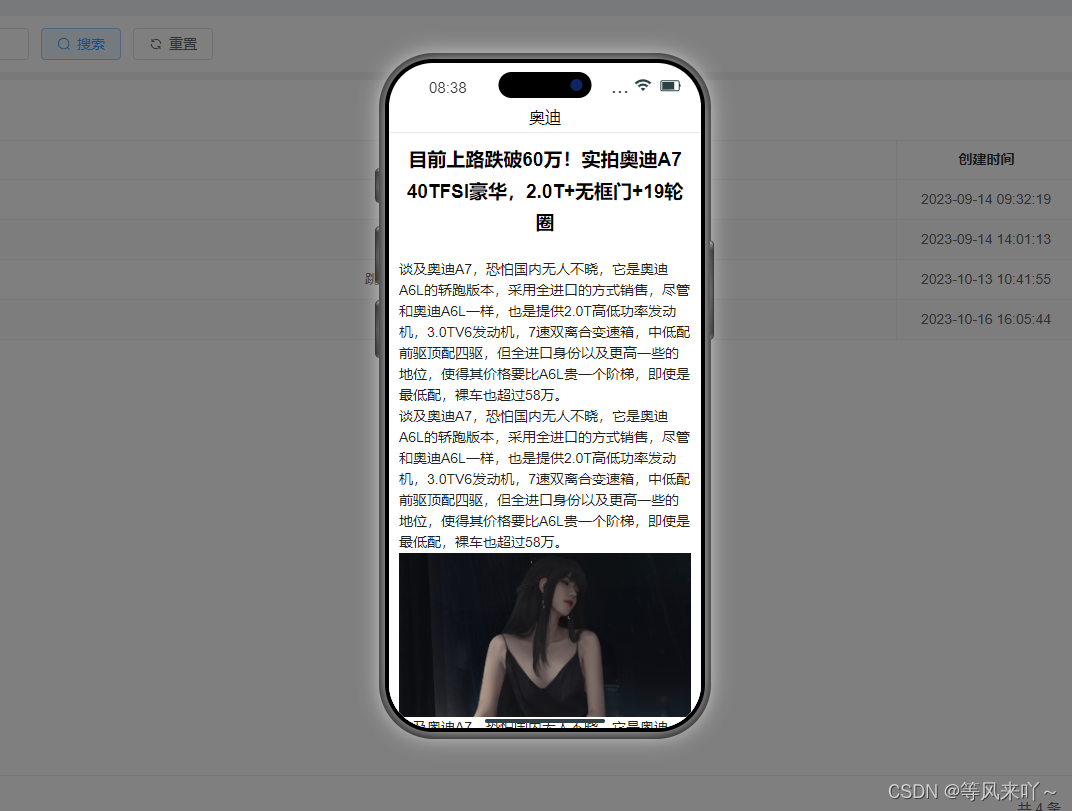
使用:
//引入
import Phone from "@/components/Phone/index.vue";
//html
<Phone v-model:phoneStatus="组件显示状态" :phone-title="手机导航标题名称">
<template #conts>
<!-- 这里插槽手机body内容可以自己编写html -->
<div class="page_conts" v-html="lookForm.content" />
</template>
</Phone>
组件完整代码(图标用svg可以根据项目图标自行更换):
<script setup lang="ts">
defineOptions({
name: "Phone"
});
const emits = defineEmits(["update:phoneStatus"]);
const props = defineProps({
/* 是否显示 */
phoneStatus: {
type: Boolean,
required: true,
default() {
return false;
}
},
/* 标题 */
phoneTitle: {
type: String,
required: true,
default() {
return "";
}
}
});
/* 关闭回调 */
function phoneClose() {
emits("update:phoneStatus", false);
}
/* 手机时间 */
function showData() {
const now = new Date();
const hours = now.getHours();
const minutes = now.getMinutes();
const currentTime = `${hours < 10 ? "0" + hours : hours}:${minutes}`;
return currentTime;
}
</script>
<template>
<div>
<div class="look_box">
<el-dialog
modal-class="look_box"
:model-value="props.phoneStatus"
title="Tips"
width="332px"
:append-to-body="false"
@close="phoneClose"
>
<div class="phone">
<div class="phone_shadow">
<div class="nav">
<div style="font-size: 15px; padding-top: 5px; padding-left: 5px">
{{ showData() }}
</div>
<div class="icons">
<span style="font-size: 22px; margin-right: 5px">...</span>
<span style="margin-right: 7px">
<svg
t="1701753286800"
class="icon"
viewBox="0 0 1024 1024"
version="1.1"
xmlns="http://www.w3.org/2000/svg"
p-id="1539"
width="18"
height="18"
>
<path
d="M42.666667 384l85.333333 85.333333c212.053333-212.053333 555.946667-212.053333 768 0l85.333333-85.333333C722.133333 124.8 301.866667 124.8 42.666667 384z m341.333333 341.333333l128 128 128-128c-70.613333-70.613333-185.386667-70.613333-256 0z m-170.666667-170.666666l85.333334 85.333333c117.76-117.76 308.906667-117.76 426.666666 0l85.333334-85.333333c-164.906667-164.906667-432.426667-164.906667-597.333334 0z"
p-id="1540"
fill="#2d4247"
/>
</svg>
</span>
<span>
<svg
t="1701754878961"
class="icon"
viewBox="0 0 1024 1024"
version="1.1"
xmlns="http://www.w3.org/2000/svg"
p-id="6536"
width="23"
height="23"
>
<path
d="M144.700101 684.994006l535.580045 0L680.280146 359.237781 144.700101 359.237781 144.700101 684.994006 144.700101 684.994006zM918.373823 440.680675l0-81.442894c0-44.791136-36.649711-81.437777-81.437777-81.437777l-692.235944 0c-44.791136 0-81.437777 36.646642-81.437777 81.437777L63.262324 684.994006c0 44.791136 36.646642 81.442894 81.437777 81.442894l692.235944 0c44.788066 0 81.437777-36.650735 81.437777-81.442894l0-81.437777c22.396079 0 40.7194-18.322297 40.7194-40.7194l0-81.436754C959.093223 459.003995 940.769902 440.680675 918.373823 440.680675L918.373823 440.680675zM877.655446 481.400075l0 81.436754L877.655446 684.994006c0 22.395056-18.323321 40.718377-40.7194 40.718377l-692.235944 0c-22.396079 0-40.7194-18.323321-40.7194-40.718377L103.980701 359.237781c0-22.396079 18.323321-40.7194 40.7194-40.7194l692.235944 0c22.396079 0 40.7194 18.323321 40.7194 40.7194L877.655446 481.400075 877.655446 481.400075zM877.655446 481.400075"
fill="#2d4247"
p-id="6537"
/>
</svg>
</span>
</div>
</div>
<div class="nav_s" style="background-color: #fff">
<div class="span_title">{{ props.phoneTitle }}</div>
</div>
<!-- 内容区域 -->
<div class="phone_conts" style="background-color: #f3f4f6">
<slot name="conts">
<!-- 页面头部 -->
</slot>
</div>
<div class="bottom_bar" />
</div>
<div class="volume" />
<div class="bang">
<div class="yuan" />
</div>
<div class="power1" />
<div class="power2" />
<div class="power3" />
</div>
</el-dialog>
</div>
</div>
</template>
<style lang="scss" scoped>
/* 手机样式 */
:deep(.look_box .el-dialog__header) {
display: none;
}
:deep(.look_box .el-dialog) {
border-radius: 0px !important;
}
:deep(.look_box .el-dialog__body) {
padding: 0 !important;
}
:deep(.look_box .el-dialog) {
background: transparent !important;
box-shadow: none;
}
:deep(.look_box .el-dialog__body) {
border: none !important;
}
.html_conts img {
width: 100%;
height: auto;
vertical-align: bottom;
padding: 5px 0;
}
.look_box {
.look_box_cont {
height: 500px;
}
}
.phone {
position: relative;
width: 332px;
height: 686px;
border: 15px solid #000;
border-radius: 55px;
box-sizing: border-box;
z-index: 1;
}
.phone_shadow {
content: "";
position: absolute;
width: 320px;
height: 673px;
box-shadow: 0px 0px 24px #fff;
border-radius: 48px;
left: -9px;
top: -9px;
background: #fff;
border: 4px solid #000;
box-sizing: border-box;
overflow: hidden;
z-index: 8;
.nav {
height: 36px;
color: #333333;
font-size: 16px;
display: flex;
align-items: center;
justify-content: space-between;
box-sizing: border-box;
padding-right: 19px;
padding-left: 35px;
margin-top: 4px;
.data {
}
.icons {
display: flex;
align-items: center;
}
}
.nav_s {
height: 30px;
display: flex;
align-items: center;
justify-content: space-between;
position: relative;
background-color: #f4f4f5;
box-sizing: border-box;
padding: 0 10px;
font-size: 16px;
border-bottom: 1px solid #ececec;
.span_title {
position: absolute;
font-size: 16px;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
color: #000;
-webkit-line-clamp: 1;
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-box-orient: vertical;
}
.left {
color: #000;
font-size: 20px;
}
.right {
color: #83878d;
.one {
margin-right: 5px;
}
}
}
.phone_conts {
height: 597px;
overflow-y: scroll;
box-sizing: border-box;
color: #000;
.titles {
font-size: 16px;
font-weight: 700;
line-height: 30px;
}
.data {
color: #666769;
border-bottom: 1px dashed #c6c6c6;
line-height: 26px;
}
.html_conts {
margin-top: 20px;
color: #666769;
line-height: 30px;
word-break: break-all;
}
}
.bottom_bar {
position: absolute;
width: 120px;
height: 4px;
background-color: #2d4247;
position: absolute;
left: 50%;
transform: translateX(-50%);
bottom: 5px;
border-radius: 5px;
}
}
.phone_conts::-webkit-scrollbar {
display: none;
}
.volume {
width: 5px;
height: 100px;
border: #000 1px solid;
background: linear-gradient(#8c8c8c, #000 9%, #222, #000 94%, #353535 100%);
position: absolute;
right: -18px;
top: 172px;
z-index: -1;
border-radius: 0 20px 20px 0;
}
.power1 {
width: 5px;
height: 35px;
border: #000 1px solid;
background: linear-gradient(#8c8c8c, #000 9%, #222, #000 94%, #353535 100%);
position: absolute;
left: -19px;
top: 100px;
z-index: -1;
border-radius: 20px 0 0 20px;
}
.power2 {
width: 5px;
height: 58px;
border: #000 1px solid;
background: linear-gradient(#8c8c8c, #000 9%, #222, #000 94%, #353535 100%);
position: absolute;
left: -19px;
top: 158px;
z-index: -1;
border-radius: 20px 0 0 20px;
}
.power3 {
width: 5px;
height: 58px;
border: #000 1px solid;
background: linear-gradient(#8c8c8c, #000 9%, #222, #000 94%, #353535 100%);
position: absolute;
left: -19px;
top: 232px;
z-index: -1;
border-radius: 20px 0 0 20px;
}
.receiver {
position: absolute;
top: 4px;
left: 50%;
transform: translateX(-50%);
background: #000000;
border: #484848 1px solid;
width: 50px;
height: 8px;
z-index: 9;
border-radius: 20px;
}
.bang {
width: 93px;
height: 26px;
background-color: #000;
position: absolute;
left: 50%;
transform: translateX(-50%);
border-radius: 13px;
top: 4px;
z-index: 9;
}
.yuan {
width: 12px;
height: 12px;
background-color: #0f2563;
box-sizing: border-box;
position: absolute;
top: 7px;
right: 9px;
border-radius: 50%;
}
.look_my_phone .phone_conts {
overflow: hidden !important;
padding: 0 !important;
}
</style>