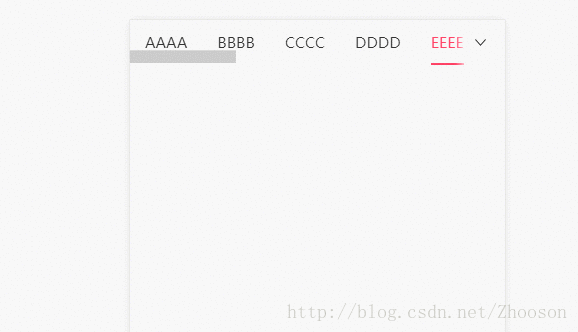
<template>
<div>
<div class="topic-list-inner">
<div class="nav" ref="nav">
<div class="box" v-for="(item,index) in list" @click="queryTopic(item,index)">
<div class="item" :class="{active:navActiveIndex==index}">{{item.title}}</div>
</div>
</div>
<div class="nav-right-arrow rotateUp" @click="openTagModal(list)">
<img src="./images/drop-down.png" alt="" class="drop-down" :class="{reverse:showModal}">
</div>
<modal-tag v-if="showModal" @close="showModal = false" :query="queryTopic"
:my-tag="selectTag" :active-index="navActiveIndex"
></modal-tag>
</div>
</div>
</template>
<script>
import modalTag from 'components/navModal/navModal.vue';
import AutoScroll from 'assets/script/autoScroll'
let autoScrollInstance = null
export default {
name: 'navScroll',
data() {
return {
list: [
{title: 'AAAA', id: 1},
{title: 'BBBB', id: 2},
{title: 'CCCC', id: 3},
{title: 'DDDD', id: 4},
{title: 'EEEE', id: 5},
{title: 'FFFF', id: 6},
{title: 'HHHH', id: 7},
{title: 'MMMM', id: 8},
{title: 'RRRR', id: 9},
{title: 'QQQQ', id: 10},
{title: 'UUUU', id: 11},
{title: 'TTTT', id: 12},
{title: 'NNNN', id: 13},
{title: 'OOOO', id: 14},
{title: 'PPPP', id: 15},
{title: 'ZZZZ', id: 16},
],
navActiveIndex: 0,
showModal: false,
selectTag: null,
}
},
methods: {
queryTopic(data, index) {
this.navActiveIndex = index;
if (autoScrollInstance) {
autoScrollInstance.scrollTo(this.$refs.nav.childNodes[index])
}
},
openTagModal(tag) {
event.stopPropagation()
this.showModal = true
this.selectTag = tag;
},
},
components: {
'modal-tag': modalTag,
},
mounted() {
this.$nextTick(() => {
autoScrollInstance = new AutoScroll(this.$refs.nav, {spaceBetween: 0})
})
}
}
</script>
<style lang="scss" scoped>
.topic-list-inner {
width: 100%;
position: fixed;
top: 0;
left: 0;
background: #fff;
}
.nav {
display: flex;
width: 7rem;
overflow-x: auto;
overflow-y: hidden;
.box { white-space: nowrap;
font-size: 0.3rem;
padding: 0 0.3rem;
height: 0.9rem;
line-height: 0.9rem;
color: #333333;
.item { height: 100%;
&.active { color: #fe3e62;
border-bottom: 1.5px solid #fe3e62;
}
}
}
}
.nav-right-arrow {
position: fixed;
right: 0;
top: 0;
width: 1rem;
height: 0.9rem;
background-image: linear-gradient(to right, rgba(#fff, 0), #fff 30%, #fff);
display: flex;
align-items: center;
justify-content: center;
.drop-down { width: 0.22rem;
transform: rotate(0deg);
transition: all 0.5s;
&.reverse { transform: rotate(-180deg);
}
}
}
</style>
<template>
<transition name="modal">
<div class="modal-mask" @click="$emit('close')">
<div class="modal-wrapper">
<div class="modal-container cf">
<div v-for='(list,index) in myTag' class="list-title"
@click="query(list,index)"
:class="{active:activeIndex===index}">
{{list.title}}
</div>
</div>
</div>
</div>
</transition>
</template>
<script type="text/babel">
export default {
props: ['myTag', 'activeIndex', 'query'],
data() {
return {
navActiveIndex: 0,
}
},
mounted() {
},
methods: {}
}
</script>
<style scoped lang="scss">
.modal-mask {
position: fixed;
z-index: 9998;
top: 0;
left: 0;
width: 100%;
height: 100%;
transition: opacity .3s ease;
}
.modal-wrapper {
padding-top: 0.91rem;
}
.modal-container {
width: 100%;
margin: 0 auto;
background-color: #fff;
transition: all .3s ease;
padding: 0 0.1rem 0.4rem 0.22rem;
font-family: Helvetica, Arial, sans-serif;
.list-title { color: #666;
border-radius: 4px;
font-size: 0.26rem;
border: 1px solid #999;
height: 0.57rem;
width: 1.93rem;
text-align: center;
float: left;
line-height: 0.57rem;
margin: 0.4rem 0.2rem 0 0.2rem;
&.active { color: #fe3e62;
border: 1.5px solid #fe3e62;
}
}
}
.myTagClose {
color: #fff;
font-size: 0.6rem;
margin: 0.4rem auto 0 auto;
height: 1rem;
width: 1rem;
text-align: center;
}
.modal-default-button {
float: right;
}
.modal-enter {
opacity: 0;
}
.modal-leave-active {
transition: all .8s cubic-bezier(1.0, 0.5, 0.8, 1.0);
}
.modal-enter .modal-container,
.modal-leave-active .modal-container {
transform: translateY(-0.9rem);
opacity: 0;
}
</style>
export default function autoScroll(dom,options){
var options=options||{};
var defaults={
spaceBetween:15,
duration:600,
};
var settings = $.extend( {}, defaults, options );
var $container = $(dom);
var $item = $container.children();
$item.css({
margin:"0 0"
});
$item.first().css({
"margin-left":"0"
});
$item.last().css({
"margin-right":"0"
});
var _this=this;
var cW = $container.outerWidth();
$item.on('click',function(){
scrollTo(this);
});
this.scrollTo=function(dom){
scrollTo(dom);
};
function scrollTo(dom){
var itemPL=$(dom).position().left;
var containerSl=$container.scrollLeft();
var itemW=$(dom).outerWidth();
var containerW=$container.outerWidth();
$container.animate({scrollLeft: itemPL+settings.spaceBetween+containerSl-containerW/2+itemW/2}, settings.duration);
}
}