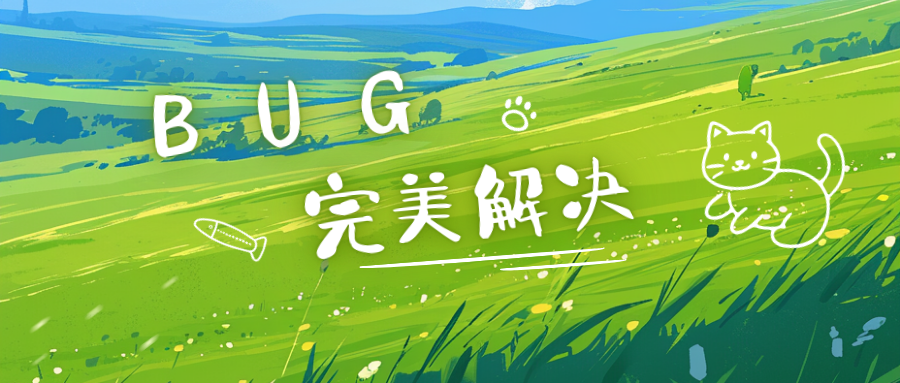
在Java多线程编程中,java.lang.ThreadDeath
异常是一个相对少见但重要的异常。它主要出现在线程被强制终止的情况下。本文将详细分析该异常的背景、可能的原因,提供错误和正确的代码示例,并列出相关的注意事项,帮助读者正确处理该异常。
一、分析问题背景
java.lang.ThreadDeath
是一个特殊的错误类型,继承自java.lang.Error
,并且是唯一一个被Thread.stop()
方法抛出的异常。尽管ThreadDeath
本质上是一个错误而非异常,但它可以被捕获。然而,由于其与线程强制终止密切相关,通常建议不要捕获它,而是允许线程自然终止。
这个异常通常出现在以下场景中:
- 在代码中显式调用了
Thread.stop()
方法来终止一个线程。 - 线程在执行过程中被强制中断,但没有提供适当的清理或终止逻辑。
场景示例:
Thread thread = new Thread(() -> {
while (true) {
// 模拟长时间运行的任务
System.out.println("Thread is running...");
}
});
thread.start();
// 停止线程
thread.stop(); // 这里将抛出ThreadDeath异常
二、可能出错的原因
导致java.lang.ThreadDeath
异常的原因主要包括以下几种:
- 使用
Thread.stop()
方法:该方法已被废弃,但在某些遗留系统或代码中可能仍在使用。该方法会强制终止线程,并抛出ThreadDeath
错误,导致线程中断时没有机会执行清理操作。 - 错误的线程终止方式:开发者试图通过不安全的方法强制停止线程,而不是使用推荐的线程中断机制(如
Thread.interrupt()
)。 - 缺乏对线程生命周期的正确管理:在多线程编程中,未正确管理线程的生命周期,可能导致线程在未预期的时间点被强制终止。
三、错误代码示例
以下是一个典型的错误代码示例,其中使用了Thread.stop()
方法:
public class ThreadDeathExample {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
while (true) {
// 模拟长时间运行的任务
System.out.println("Thread is running...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("Thread was interrupted.");
}
}
});
thread.start();
// 强制终止线程
try {
Thread.sleep(3000); // 让线程运行一段时间
thread.stop(); // 这里将抛出ThreadDeath异常
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
错误分析:
- 使用
Thread.stop()
方法是导致ThreadDeath
异常的直接原因。这会强制终止线程,并且会留下未处理的资源或不一致的状态。
四、正确代码示例
为了避免java.lang.ThreadDeath
异常,应使用更安全的方法来停止线程,通常推荐使用Thread.interrupt()
方法并在线程内部处理中断信号。以下是改进后的代码示例:
public class ThreadInterruptExample {
private static volatile boolean running = true;
public static void main(String[] args) {
Thread thread = new Thread(() -> {
while (running) {
// 模拟长时间运行的任务
System.out.println("Thread is running...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("Thread was interrupted.");
running = false; // 设置标志位以安全终止线程
}
}
System.out.println("Thread is stopping safely.");
});
thread.start();
// 请求终止线程
try {
Thread.sleep(3000); // 让线程运行一段时间
thread.interrupt(); // 安全地中断线程
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
代码改进说明:
- 使用
Thread.interrupt()
方法发送中断信号,而不是强制终止线程。 - 在线程内部使用
volatile
变量控制线程的运行状态,从而安全地终止线程。 - 在中断时适当处理
InterruptedException
并安全退出线程,避免资源泄露和数据不一致问题。
五、注意事项
在编写多线程代码时,注意以下几点可以有效避免java.lang.ThreadDeath
异常:
- 避免使用
Thread.stop()
:Thread.stop()
方法自JDK 1.2起已被废弃,不应在现代Java编程中使用。取而代之的是使用Thread.interrupt()
来安全地中断线程。 - 使用中断机制:通过使用中断机制(
Thread.interrupt()
和InterruptedException
),可以让线程有机会在中断时清理资源和处理未完成的任务。 - 正确管理线程生命周期:在多线程编程中,始终考虑线程的启动和终止条件,确保线程在生命周期结束时能够正常退出。
- 同步和资源管理:在使用线程时,确保对共享资源的访问是线程安全的,避免在终止线程时造成资源泄露或状态不一致。
通过遵循上述建议,您可以有效避免java.lang.ThreadDeath
异常,并编写更加健壮和安全的多线程应用程序。希望本文能够帮助您理解并解决这一异常问题。