目录结构如下:
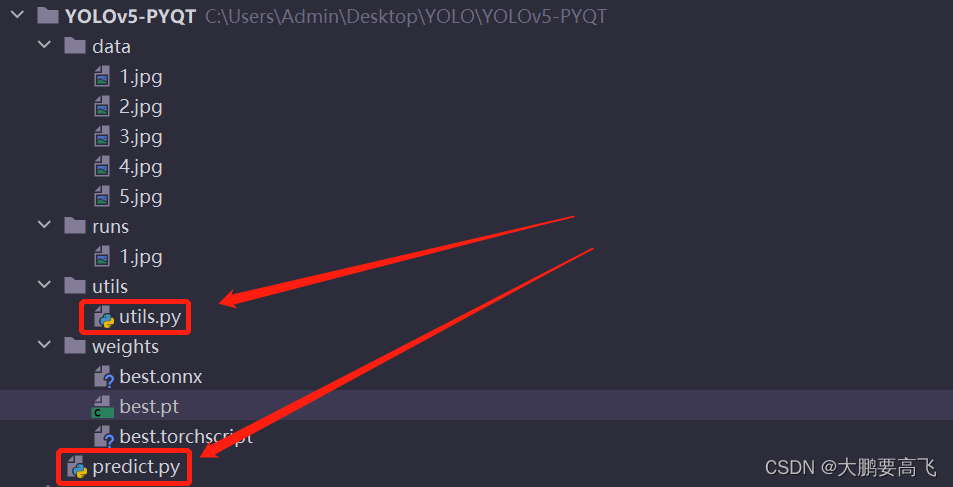
以加载torchscript模型文件为例,如下为Predict.py代码,utils.py中代码则从原始YOLOV5代码中拷贝,即Predict.py中用到的代码块,其中对部分用不到的代码进行了删减:
import cv2
import numpy as np
import torch
from utils.utils import letterbox, non_max_suppression, Annotator, scale_boxes, process_mask, colors
from pathlib import Path
image_path = './data/1.jpg'
output_path = './runs/'
onnx_model_path = './weights/best.torchscript'
names = ['crack']
device = torch.device('cuda:0')
model = torch.jit.load(onnx_model_path, map_location=device)
model.half()
img0 = cv2.imread(image_path)
img = letterbox(img0, new_shape=(416, 416), color=(114, 114, 114), auto=True, scaleFill=False, scaleup=True, stride=32)[0]
img = img.transpose((2, 0, 1))[::-1]
img = np.expand_dims(img, 0)
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).float().to(device)
img = img.half()
img /= 255
pred, proto = model(img)
pred = non_max_suppression(pred, conf_thres=0.25, iou_thres=0.45, nm=32)
for i, det in enumerate(pred):
annotator = Annotator(img0, line_width=3, example=str(names))
if len(det):
masks = process_mask(proto[i], det[:, 6:], det[:, :4], img.shape[2:], upsample=True)
det[:, :4] = scale_boxes(img.shape[2:], det[:, :4], img0.shape).round()
annotator.masks(
masks,
colors=[colors(x, True) for x in det[:, 5]],
im_gpu=img[0])
for j, (*xyxy, conf, cls) in enumerate(reversed(det[:, :6])):
c = int(cls)
label = f'{names[c]} {conf:.2f}'
annotator.box_label(xyxy, label, color=colors(c, True))
im0 = annotator.result()
save_image_name = Path(image_path).name
cv2.imwrite(output_path + save_image_name, im0)
if __name__ == '__main__':
print('hello')