C#中HttpWebRequest、WebClient、HttpClient 、HttpClientFactory、 Flurl的使用总结
三者的区别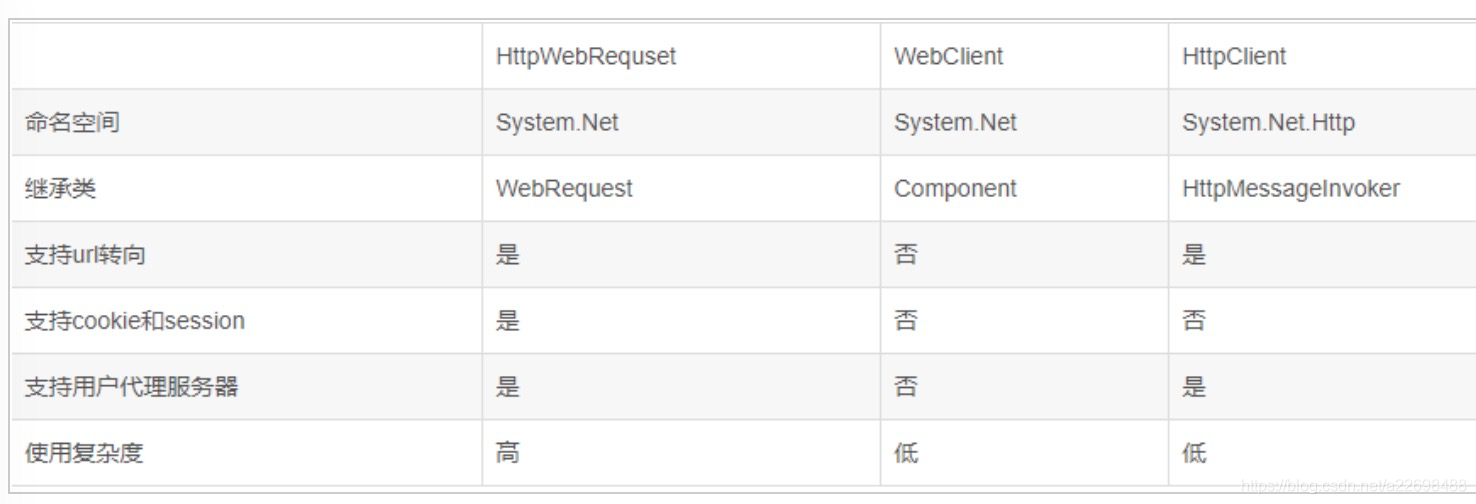
HttpWebRequest
命名空间: System.Net,这是.NET创建者最初开发用于使用HTTP请求的标准类。使用HttpWebRequest可以让开发者控制请求/响应流程的各个方面,如 timeouts, cookies, headers, protocols。另一个好处是HttpWebRequest类不会阻塞UI线程。例如,当您从响应很慢的API服务器下载大文件时,您的应用程序的UI不会停止响应。HttpWebRequest通常和WebResponse一起使用,一个发送请求,一个获取数据。HttpWebRquest更为底层一些,能够对整个访问过程有个直观的认识,但同时也更加复杂一些。在framework中,
大量并发 HttpWebRequest 需要设置一个最大连接数
,- 如果第一次访问很慢,取消代理检查
- .Net中并发连接限制数量默认是2,哪怕你开100条线程同时进行请求,有效的连接数仍是2条,解决办法就是在App.config中修改配置.
<system.net>
<defaultProxy enabled="false">
<proxy/>
<bypasslist/>
<module/>
</defaultProxy>
<connectionManagement>
<!-- address:要限制的地址 maxconnection:最大连接数 -->
<add address="*" maxconnection="512"/>
</connectionManagement>
ServicePointManager.DefaultConnectionLimit = 200;
WebClient.Proxy = null; 或 HttpWebRequest.Proxy = null;
//POST方法
public static string HttpPost(string Url, string postDataStr)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
Encoding encoding = Encoding.UTF8;
byte[] postData = encoding.GetBytes(postDataStr);
request.ContentLength = postData.Length;
Stream myRequestStream = request.GetRequestStream();
myRequestStream.Write(postData, 0, postData.Length);
myRequestStream.Close();
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream myResponseStream = response.GetResponseStream();
StreamReader myStreamReader = new StreamReader(myResponseStream, encoding);
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
return retString;
}
//GET方法
public static string HttpGet(string Url, string postDataStr)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url + (postDataStr == "" ? "" : "?") + postDataStr);
request.Method = "GET";
request.ContentType = "text/html;charset=UTF-8";
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream myResponseStream = response.GetResponseStream();
StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.GetEncoding("utf-8"));
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
return retString;
}
WebClient
命名空间System.Net,WebClient是一种更高级别的抽象,是HttpWebRequest为了简化最常见任务而创建的,使用过程中你会发现他缺少基本的header,timeoust的设置,不过这些可以通过继承httpwebrequest来实现。相对来说,WebClient比WebRequest更加简单,它相当于封装了request和response方法,不过需要说明的是,Webclient和WebRequest继承的是不同类,两者在继承上没有任何关系。使用WebClient可能比HttpWebRequest直接使用更慢(大约几毫秒),但却更为简单,减少了很多细节,代码量也比较少。
public class WebClientHelper
{
public static string DownloadString(string url)
{
WebClient wc = new WebClient();
//wc.BaseAddress = url; //设置根目录
wc.Encoding = Encoding.UTF8; //设置按照何种编码访问,如果不加此行,获取到的字符串中文将是乱码
string str = wc.DownloadString(url);
return str;
}
public static string DownloadStreamString(string url)
{
WebClient wc = new WebClient();
wc.Headers.Add("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/76.0.3809.132 Safari/537.36");
Stream objStream = wc.OpenRead(url);
StreamReader _read = new StreamReader(objStream, Encoding.UTF8); //新建一个读取流,用指定的编码读取,此处是utf-8
string str = _read.ReadToEnd();
objStream.Close();
_read.Close();
return str;
}
public static void DownloadFile(string url, string filename)
{
WebClient wc = new WebClient();
wc.DownloadFile(url, filename); //下载文件
}
public static void DownloadData(string url, string filename)
{
WebClient wc = new WebClient();
byte [] bytes = wc.DownloadData(url); //下载到字节数组
FileStream fs = new FileStream(filename, FileMode.Create);
fs.Write(bytes, 0, bytes.Length);
fs.Flush();
fs.Close();
}
public static void DownloadFileAsync(string url, string filename)
{
WebClient wc = new WebClient();
wc.DownloadFileCompleted += DownCompletedEventHandler;
wc.DownloadFileAsync(new Uri(url), filename);
Console.WriteLine("下载中。。。");
}
private static void DownCompletedEventHandler(object sender, AsyncCompletedEventArgs e)
{
Console.WriteLine(sender.ToString()); //触发事件的对象
Console.WriteLine(e.UserState);
Console.WriteLine(e.Cancelled);
Console.WriteLine("异步下载完成!");
}
public static void DownloadFileAsync2(string url, string filename)
{
WebClient wc = new WebClient();
wc.DownloadFileCompleted += (sender, e) =>
{
Console.WriteLine("下载完成!");
Console.WriteLine(sender.ToString());
Console.WriteLine(e.UserState);
Console.WriteLine(e.Cancelled);
};
wc.DownloadFileAsync(new Uri(url), filename);
Console.WriteLine("下载中。。。");
}
}
HttpClient
HttpClient是.NET4.5引入的一个HTTP客户端库,其命名空间为 System.Net.Http ,.NET 4.5之前我们可能使用WebClient和HttpWebRequest来达到相同目的。HttpClient利用了最新的面向任务模式,使得处理异步请求非常容易。它适合用于多次请求操作,一般设置好默认头部后,可以进行重复多次的请求,基本上用一个实例可以提交任何的HTTP请求。HttpClient有预热机制,第一次进行访问时比较慢,所以不应该用到HttpClient就new一个出来,应该使用单例或其他方式获取HttpClient的实例
public class HttpClientHelper
{
private static readonly object LockObj = new object();
private static HttpClient client = null;
public HttpClientHelper() {
GetInstance();
}
public static HttpClient GetInstance()
{
if (client == null)
{
lock (LockObj)
{
if (client == null)
{
client = new HttpClient();
}
}
}
return client;
}
public async Task<string> PostAsync(string url, string strJson)//post异步请求方法
{
try
{
HttpContent content = new StringContent(strJson);
content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/json");
//由HttpClient发出异步Post请求
HttpResponseMessage res = await client.PostAsync(url, content);
if (res.StatusCode == System.Net.HttpStatusCode.OK)
{
string str = res.Content.ReadAsStringAsync().Result;
return str;
}
else
return null;
}
catch (Exception ex)
{
return null;
}
}
public string Post(string url, string strJson)//post同步请求方法
{
try
{
HttpContent content = new StringContent(strJson);
content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/json");
//client.DefaultRequestHeaders.Connection.Add("keep-alive");
//由HttpClient发出Post请求
Task<HttpResponseMessage> res = client.PostAsync(url, content);
if (res.Result.StatusCode == System.Net.HttpStatusCode.OK)
{
string str = res.Result.Content.ReadAsStringAsync().Result;
return str;
}
else
return null;
}
catch (Exception ex)
{
return null;
}
}
public string Get(string url)
{
try
{
var responseString = client.GetStringAsync(url);
return responseString.Result;
}
catch (Exception ex)
{
return null;
}
}
}
HttpClient有预热机制,第一次请求比较慢;可以通过初始化前发送一次head请求解决:
_httpClient = new HttpClient() { BaseAddress = new Uri(BASE_ADDRESS) };
//帮HttpClient热身
_httpClient.SendAsync(new HttpRequestMessage {
Method = new HttpMethod("HEAD"),
RequestUri = new Uri(BASE_ADDRESS + "/") })
.Result.EnsureSuccessStatusCode();
HttpClient 日常使用及坑点:
我们先来用个简单的例子做下测试,看为什么不要每次RPC请求都实例化一个HttpClient:
public class Program
{
static void Main(string[] args)
{
HttpAsync();
Console.WriteLine("Hello World!");
Console.Read();
}
public static async void HttpAsync()
{
for (int i = 0; i < 10; i++)
{
using (var client = new HttpClient())
{
var result = await client.GetAsync("http://www.baidu.com");
Console.WriteLine($"{i}:{result.StatusCode}");
}
}
}
}
通过netstat
查看下TCP连接情况:
默认在windows下,
TIME_WAIT
状态将会使系统将会保持该连接240s
。
这里也就引出了我上面说的载过的一次坑:在高并发的情况下,连接来不及释放,socket被耗尽,耗尽之后就会出现喜闻乐见的一个错误:
#使用jemter压测复现错误信息:
Unable to connect to the remote serverSystem.Net.Sockets.SocketException: Only one usage of each socket address (protocol/network address/port) is normally permitted.
复用了HttpClient,每次RPC请求的时候,实际上还节约了创建通道的时间,在性能压测的时候也是很明显的提升。
因为是复用的HttpClient,那么一些公共的设置就没办法灵活的调整了,如请求头的自定义。
因为HttpClient请求每个url时,会缓存该url对应的主机ip
,从而会导致DNS更新失效(TTL失效了)
HttpClientFactory优势:
HttpClientFactory 是
ASP.NET CORE 2.1
中新增加的功能。
Factory,顾名思义HttpClientFactory就是HttpClient的工厂,内部已经帮我们处理好了对HttpClient的管理,不需要我们人工进行对象释放,同时,支持自定义请求头,支持DNS更新等等等。
HttpClientFactory使用方法:
从微软源码分析,HttpClient继承自HttpMessageInvoker,而HttpMessageInvoker实质就是HttpClientHandler。
HttpClientFactory 创建的HttpClient,也即是HttpClientHandler,只是这些个HttpClient被放到了“池子”中,工厂每次在create的时候会自动判断是新建还是复用。(默认生命周期为2min)
。
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
//other codes
services.AddHttpClient("client_1",config=> //这里指定的name=client_1,可以方便我们后期服用该实例
{
config.BaseAddress= new Uri("http://client_1.com");
config.DefaultRequestHeaders.Add("header_1","header_1");
});
services.AddHttpClient("client_2",config=>
{
config.BaseAddress= new Uri("http://client_2.com");
config.DefaultRequestHeaders.Add("header_2","header_2");
});
services.AddHttpClient();
//other codes
services.AddMvc().AddFluentValidation();
}
}
这里直接以controller为例,其他地方自行DI
public class TestController : ControllerBase
{
private readonly IHttpClientFactory _httpClient;
public TestController(IHttpClientFactory httpClient)
{
_httpClient = httpClient;
}
public async Task<ActionResult> Test()
{
var client = _httpClient.CreateClient("client_1"); //复用在Startup中定义的client_1的httpclient
var result = await client.GetStringAsync("/page1.html");
var client2 = _httpClient.CreateClient(); //新建一个HttpClient
var result2 = await client.GetStringAsync("http://www.site.com/XXX.html");
return null;
}
}