用于将文件夹下文件全部复制的测试类
优势:
- 无需导入第三方插件,不受jdk版本限制,
- 不破坏原有的路径结构,不复制空的文件夹
用法描述:
- 找到对应的版本变更文件
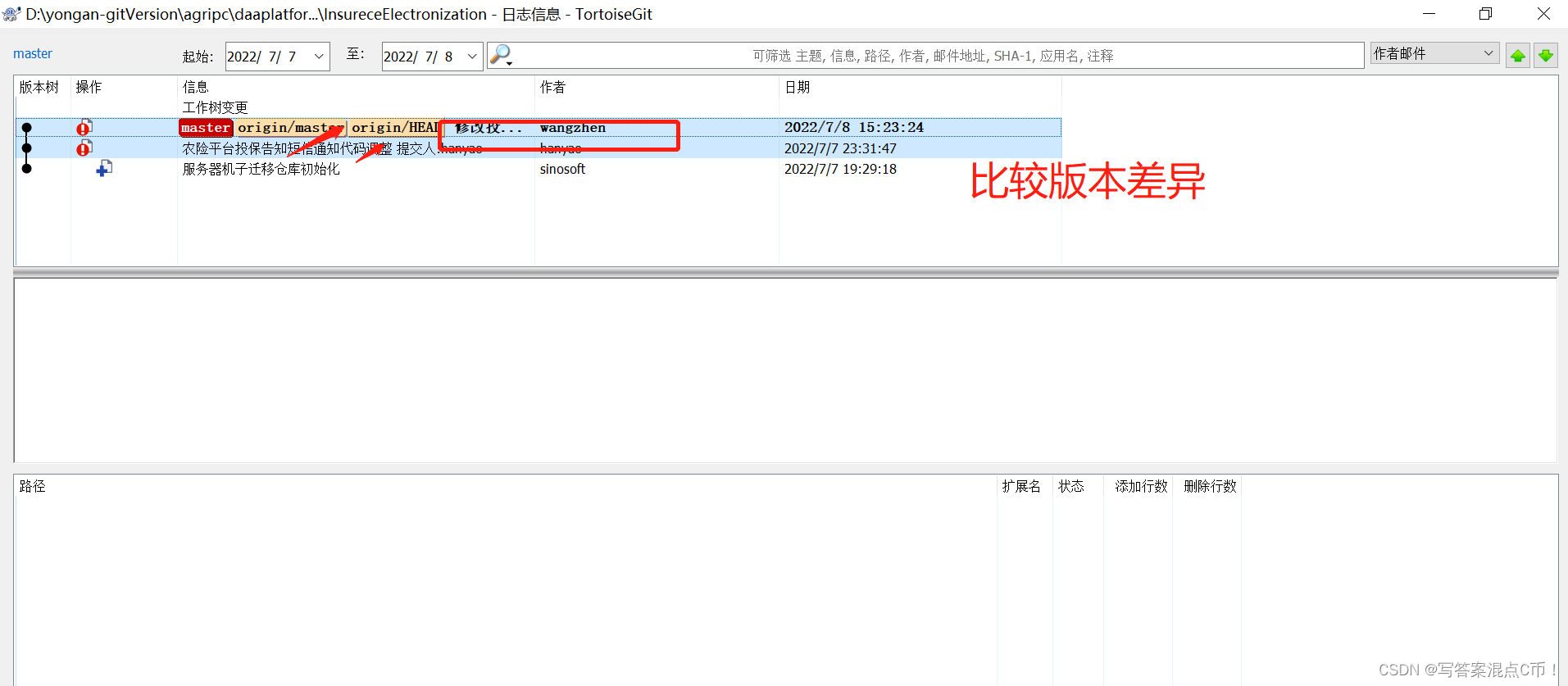
- 点击比较版本差异
- 查看变更文件列表
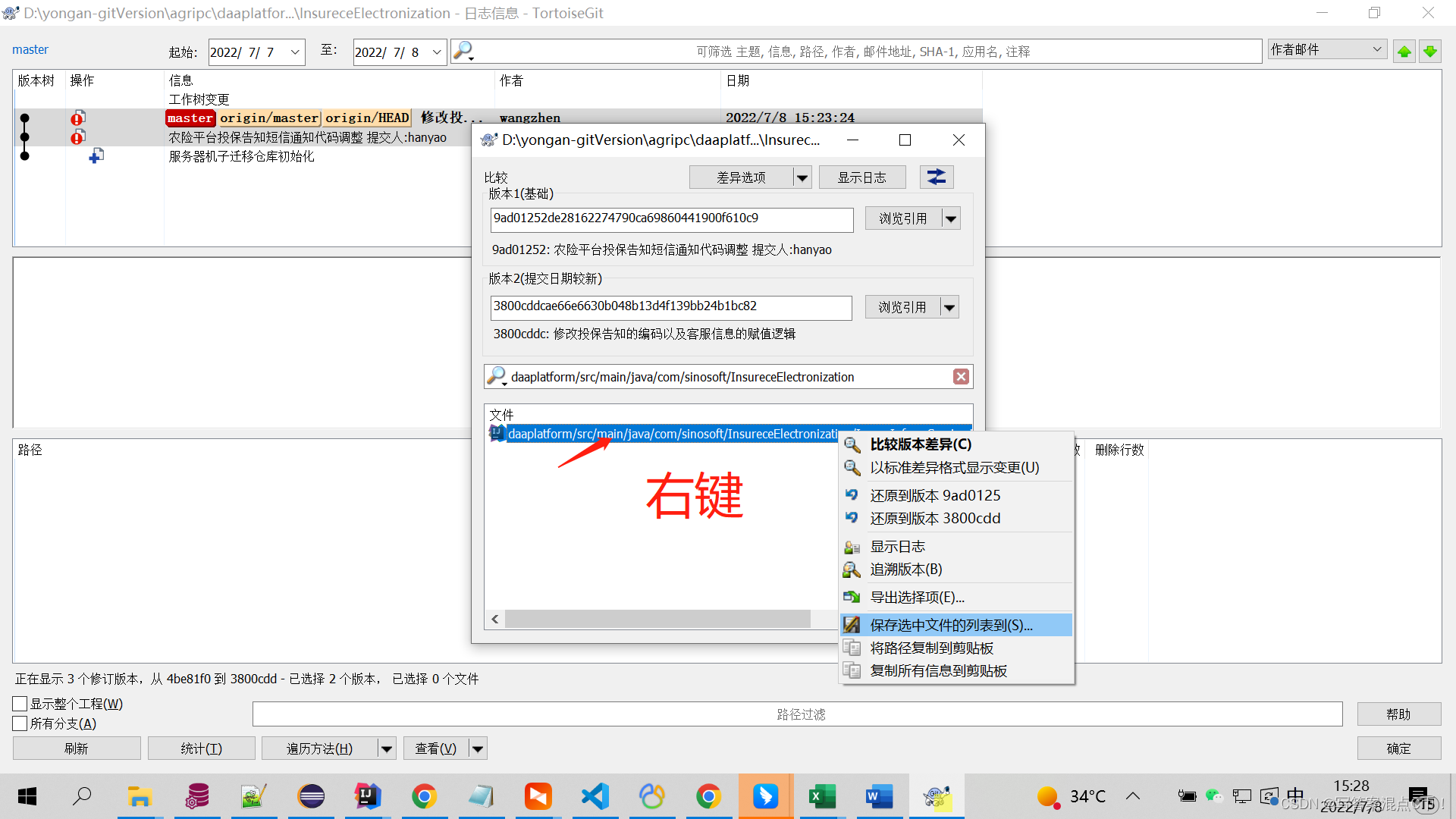
- 选中“将路径复制到剪切版”保存并新建文件。
- 将下图中的readTxtFile 后的路径改为新建文件的路径
- 根据代码配置即可
package com.sinsoft.test;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class CopyFile {
public static String[] filterFile = { ".jks", ".js", ".jsp", ".class",
".java", ".xml", ".properties", ".sql",".jar",".wsdd" };
private static long total = 0l;
private final static String orgPathPre = "D:/changan-midAgri/src/main/webapp/";
static String destDirPath="D:/package/daaplatform/";
public static void main(String[] args) {
try {
getFile(readTxtFile("D:/changan-midAgri/变更的文件信息-20220112"));
} catch (Exception e) {
e.printStackTrace();
System.out.println(e.getMessage());
}
}
private static void getFile(List<String> fileInfoList) throws IOException, Exception {
ArrayList<String> tFileCopyFailString = new ArrayList<String>();
for (String fileInfo : fileInfoList) {
if ("".equals(fileInfo) || fileInfo == null) {
continue;
}
if (new File(fileInfo).isDirectory()) {
continue;
}
String destFileStr = fileInfo.replace(orgPathPre, "");
System.out.println("替换后的文件路径"+destFileStr);
String df = new SimpleDateFormat("YYYYMMdd").format(new Date());
File des = null;
String destPathString=destDirPath+df+File.separator+destFileStr;
{
System.out.println("目的路径"+destPathString);
File destFile = new File(destPathString);
if (!destFile.exists()) {
destFile.getParentFile().mkdirs();
destFile.createNewFile();
}
des = new File(destPathString);
}
System.out.println("源文件路径:"+fileInfo+";生成文件路径:"+destPathString);
try {
copyFile(new File(fileInfo),des);
} catch (Exception e) {
e.printStackTrace();
System.out.println("复制文件:"+fileInfo+"失败");
tFileCopyFailString.add(fileInfo);
continue;
}
}
System.out.println("下载失败文件个数:"+tFileCopyFailString.size());
for (String tFailPath : tFileCopyFailString) {
System.out.println(tFailPath);
}
}
public static List<String> readTxtFile(String filePath) {
List<String> txtContent = new ArrayList<String>();
try {
String encoding = "UTF-8";
File file = new File(filePath);
if (file.isFile() && file.exists()) {
InputStreamReader read = new InputStreamReader(
new FileInputStream(file), encoding);
BufferedReader bufferedReader = new BufferedReader(read);
String lineTxt = null;
while ((lineTxt = bufferedReader.readLine()) != null) {
String trim = lineTxt.trim();
txtContent.add(trim.replace("\\", "\\\\"));
}
read.close();
} else {
System.out.println("找不到指定的文件");
}
} catch (Exception e) {
System.out.println("读取文件内容出错");
e.printStackTrace();
}
return txtContent;
}
public void copyFolder(File srcFolder, File destFolder,
String[] filterFile, String fname) throws Exception {
File[] files = srcFolder.listFiles();
destFolder.mkdirs();
for (File file : files) {
if (file.isFile()) {
String vl=file.getName().substring(file.getName().lastIndexOf("\\")+1,file.getName().length()).replace(".class", "");
if (vl.equals(fname.replace(".class", ""))||vl.indexOf(fname.replace(".class", "")+"$")==0) {
System.out.println("test:"+file.getName());
String pathname = destFolder + File.separator
+ file.getName();
for (String suff : filterFile) {
if (pathname.endsWith(suff)) {
File dest = new File(pathname);
File destPar = dest.getParentFile();
destPar.mkdirs();
if (!dest.exists()) {
dest.createNewFile();
}
if ((file.getParent()
.substring(file.getParent().length() - 4,
file.getParent().length() - 1)
.equals(dest.getParent().substring(
dest.getParent().length() - 4,
dest.getParent().length() - 1)))||file.getParent().contains("conf")) {
if (file.length() == 0) {
throw new IOException("文件不允许为空"
+ ",需要处理的文件为:" + file.getParent()
+ "\\" + file.getName());
}
copyFile(file, dest);
}
}
}
}
} else {
copyFolder(file, destFolder, filterFile, fname);
}
}
}
private static void copyFile(File src, File dest) throws Exception {
BufferedInputStream reader = null;
BufferedOutputStream writer = null;
try {
reader = new BufferedInputStream(new FileInputStream(src));
writer = new BufferedOutputStream(new FileOutputStream(dest));
byte[] buff = new byte[reader.available()];
while ((reader.read(buff)) != -1) {
writer.write(buff);
}
total += 1;
} catch (Exception e) {
throw e;
} finally {
writer.flush();
writer.close();
reader.close();
String temp = "\ncopy:\n" + src + "\tsize:" + src.length()
+ "\nto:\n" + dest + "\tsize:" + dest.length()
+ "\n complate\n totoal:" + total;
System.out.println(temp);
}
}
private static boolean deleteDir(File dir) {
if (dir.isDirectory()) {
String[] children = dir.list();
for (int i = 0; i < children.length; i++) {
boolean success = deleteDir(new File(dir, children[i]));
if (!success) {
return false;
}
}
}
return dir.delete();
}
public static void copyFile(String oldPath, String newPath) throws IOException {
File oldFile = new File(oldPath);
File file = new File(newPath);
FileInputStream in = new FileInputStream(oldFile);
FileOutputStream out = new FileOutputStream(file);;
byte[] buffer=new byte[2097152];
int readByte = 0;
while((readByte = in.read(buffer)) != -1){
out.write(buffer, 0, readByte);
}
in.close();
out.close();
}
}