#!/usr/bin/env python
# coding: utf-8
# # —— 基于电商产品评论数据情感分析 ——
# ### 1.案例简介
#
# 1、利用文本挖掘技术,对碎片化、非结构化的电商网站评论数据进行清洗与处理,转化为结构化数据。
# 2、参考知网发布的情感分析用词语集,统计评论数据的正负情感指数,然后进行情感分析,通过词云图直观查看正负评论的关键词。
# 3、比较“机器挖掘的正负情感”与“人工打标签的正负情感”,精度达到88%。
# 4、采用LDA主题模型提取评论关键信息,以了解用户的需求、意见、购买原因、产品的优缺点等。
#
# ### 2.框架
#
# 工具准备
#
# 一、导入数据
# 二、数据预处理
# (一)去重
# (二)数据清洗
# (三)分词、词性标注、去除停用词、词云图
# 三、模型构建
# (一)决策树
# (二)情感分析
# (三)基于LDA模型的主题分析
# ## 工具准备
# In[ ]:
import os
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from matplotlib.pylab import style #自定义图表风格
style.use('ggplot')
from IPython.core.interactiveshell import InteractiveShell
InteractiveShell.ast_node_interactivity = "all"
plt.rcParams['font.sans-serif'] = ['Simhei'] # 解决中文乱码问题
import re
import jieba.posseg as psg
import itertools
#conda install -c anaconda gensim
from gensim import corpora,models #主题挖掘,提取关键信息
# pip install wordcloud
from wordcloud import WordCloud,ImageColorGenerator
from collections import Counter
from sklearn import tree
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.metrics import classification_report
from sklearn.metrics import accuracy_score
import graphviz
# #### 注意
#
# 以下方法,是为了帮助我们直观查看对象处理的结果。是辅助代码,非必要代码!
# .head()
# print()
# len()
# .shape
# .unique()
# ## 一、导入数据
# In[ ]:
raw_data=pd.read_csv('data/reviews.csv')
raw_data.head()
# In[ ]:
raw_data.info()
# In[ ]:
raw_data.columns
# In[ ]:
#取值分布
for cate in ['creationTime', 'nickname', 'referenceName', 'content_type']:
raw_data[cate].value_counts()
# ## 二、数据预处理
# ### (一)去重
#
# 删除系统自动为客户做出的评论。
# In[ ]:
reviews=raw_data.copy()
reviews=reviews[['content', 'content_type']]
print('去重之前:',reviews.shape[0])
reviews=reviews.drop_duplicates()
print('去重之后:',reviews.shape[0])
# ### (二)数据清洗
# In[ ]:
# 清洗之前
content=reviews['content']
for i in range(5,10):
print(content[i])
print('-----------')
# In[ ]:
#清洗之后,将数字、字母、京东美的电热水器字样都删除
info=re.compile('[0-9a-zA-Z]|京东|美的|电热水器|热水器|')
content=content.apply(lambda x: info.sub('',x)) #替换所有匹配项
for i in range(5,10):
print(content[i])
print('-----------')
# ### (三)分词、词性标注、去除停用词、词云图
# (1)分词
# 目标
#
# 输入:
# - content、content_type
# - 共有1974条评论句子
# 输出:
# - 构造DF,包含: 分词、对应词性、分词所在原句子的id、分词所在原句子的content_type
# - 共有6万多行
#
# 非结构化数据——>结构化数据
# 
# In[ ]:
#分词,由元组组成的list
seg_content=content.apply( lambda s: [(x.word,x.flag) for x in psg.cut(s)] )
seg_content.shape
len(seg_content)
print(seg_content[0])
# In[ ]:
#统计评论词数
n_word=seg_content.apply(lambda s: len(s))
len(n_word)
n_word.head(6)
# In[ ]:
#得到各分词在第几条评论
n_content=[ [x+1]*y for x,y in zip(list(seg_content.index),list(n_word))] #[x+1]*y,表示复制y份,由list组成的list
# n_content=[ [x+1]*y for x,y in [(0,32)]]
index_content_long=sum(n_content,[]) #表示去掉[],拉平,返回list
len(index_content_long)
# n_content
# index_content_long
# list(zip(list(seg_content.index),list(n_word)))
# In[ ]:
sum([[2,2],[3,3,3]],[])
# In[ ]:
#分词及词性,去掉[],拉平
seg_content.head()
seg_content_long=sum(seg_content,[])
seg_content_long
type(seg_content_long)
len(seg_content_long)
# In[ ]:
seg_content_long[0]
# In[ ]:
#得到加长版的分词、词性
word_long=[x[0] for x in seg_content_long]
nature_long=[x[1] for x in seg_content_long]
len(word_long)
len(nature_long)
# In[ ]:
#content_type拉长
n_content_type=[ [x]*y for x,y in zip(list(reviews['content_type']),list(n_word))] #[x+1]*y,表示复制y份
content_type_long=sum(n_content_type,[]) #表示去掉[],拉平
# n_content_type
# content_type_long
len(content_type_long)
# In[ ]:
review_long=pd.DataFrame({'index_content':index_content_long,
'word':word_long,
基于电商产品评论数据情感分析
最新推荐文章于 2024-07-12 14:01:57 发布
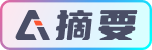