176-File类与IO流-IO流概述与流的分类
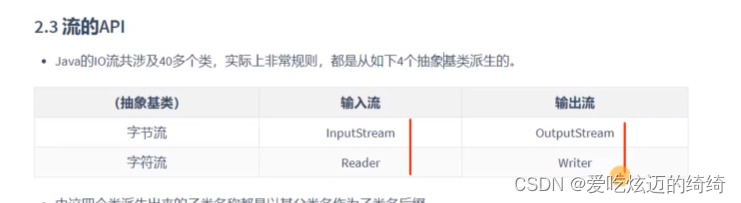
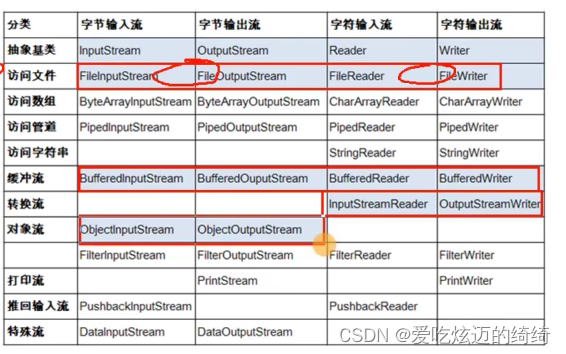
177-File类与IO流-使用FileReader和FileWriter读取、写出文本数据
package cd_one.code18.exer2;
import org.junit.Test;
import java.io.*;
public class FileReaderWriterTest {
@Test
public void test() throws IOException {
File file1 = new File("hello.txt");
FileReader fileReader = new FileReader(file1);
// System.out.println(((char)fileReader.read()));
int data;
while ((data = fileReader.read()) != -1) {
System.out.print((char) (data));
}
fileReader.close();
}
@Test
public void test2() {
FileReader fileReader = null;
try {
//1.创建File类的对象,对应着hello.txt文件
File file1 = new File("hello.txt");
fileReader = new FileReader(file1);
//2.创建输入型的字符流,用于读取数据
// System.out.println(((char)fileReader.read()));
//3.读取数据,并显示在控制台上
int data;
while ((data = fileReader.read()) != -1) {
System.out.print((char) (data));
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
//4.流资源的关闭操作(必须要关闭,否则内存泄漏)
try {
if (fileReader != null) {
fileReader.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void test3() {
FileReader fileReader = null;
try {
//1.创建File类的对象,对应着hello.txt文件
File file1 = new File("hello.txt");
fileReader = new FileReader(file1);
//2.创建输入型的字符流,用于读取数据
// System.out.println(((char)fileReader.read()));
//3.读取数据,并显示在控制台上
char[] cbutter = new char[5];
int len;
while ((len = fileReader.read(cbutter)) != -1) {
//错误的写法:
// for (int i = 0; i < cbutter.length; i++) {
// System.out.print(cbutter[i]);
// }
for (int i = 0; i < len; i++) {
System.out.print(cbutter[i]);
}
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
//4.流资源的关闭操作(必须要关闭,否则内存泄漏)
try {
if (fileReader != null) {
fileReader.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void test4() {
FileWriter fw = null;
try {
//1.chuanjian File类的对象,支出写出的文件的名称
File file = new File("info.txt");
//2.创建输出流
// fw = new FileWriter(file);
fw = new FileWriter(file, true);//现有的文件下追加内容
//3.写出具体的过程
fw.write("I love U!\n");
fw.write("You love him!\n");
fw.write("太惨了...");
System.out.println("输出成功!");
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.关闭资源
try {
if (fw != null) {
fw.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void test5() throws IOException {
//1.穿件File类的对象
FileReader fr = null;
FileWriter fw = null;
try {
File srcFile = new File("hello.txt");
File destFile = new File("hello_copy.txt");
//2.创建输入流、输出流
fr = new FileReader(srcFile);
fw = new FileWriter(srcFile);
//3.数据的读入和写出的过程
char[] cbutter = new char[5];
int len;
while ((len = fr.read(cbutter)) != -1) {
fw.write(cbutter, 0, len);//正确的
// fw.write(cbutter);//错误的
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
//方式2:
// try {
// fw.close();
//
// } catch (IOException e) {
// e.printStackTrace();
// }finally {
// try {
// fr.close();
// } catch (IOException e) {
// e.printStackTrace();
// }
// }
//4.关闭流资源
try {
if (fw != null) {
fw.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fr != null) {
fr.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
178-File类与IO流-FileInputStream和FileOutputStream的使用
package cd_one.code18.exer3;
import org.junit.Test;
import java.io.*;
public class FileStreamTest {
@Test
public void test() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
//1.创建相关的File类的对象
File srcFile = new File("2.jpg");
File destFile = new File("2_copy.jpg");
//2.创建相关的字节流
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile,true);
//3.数据的读入和写出
byte[] butter = new byte[1024];//1kb
int len;
while ((len = fis.read(butter)) != -1) {
fos.write(butter, 0, len);
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
//4.关闭资源
}
@Test
public void test1() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
//1.创建相关的File类的对象
File srcFile = new File("hello1.txt");
File destFile = new File("hello1_copy.txt");
//2.创建相关的字节流
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile,true);
//3.数据的读入和写出
byte[] butter = new byte[1024];//1kb
int len;
while ((len = fis.read(butter)) != -1) {
fos.write(butter, 0, len);
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
//4.关闭资源
}
@Test
public void test2() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
//1.创建相关的File类的对象
File srcFile = new File("hello.txt");
//2.创建相关的字节流
fis = new FileInputStream(srcFile);
//3.数据的读入和写出
byte[] butter = new byte[1024];//1kb
int len;
while ((len = fis.read(butter)) != -1) {
String str = new String(butter,0,len);
System.out.println(str);
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
//4.关闭资源
}
179-File类与IO流-处理流之1:缓冲流的使用
package cd_one.code18.exer4;
import org.junit.Test;
import java.io.*;
public class BufferedStreamTest {
@Test
public void test() throws Exception {
//使用BufferedInputStream 、 BufferedOutputStream复制一个图片
//创建相关的File类的对象
File srcFile = new File("2.jpg");
File destFile = new File("2_copy.jpg");
//2.创建相关的字节流
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
BufferedInputStream bis = new BufferedInputStream(fis);
BufferedOutputStream bos = new BufferedOutputStream(fos);
//3.数据的读入和写出
byte[] butter = new byte[1024];
int len;
while((len = bis.read(butter)) != -1){
bos.write(butter,0,len);
}
System.out.println("复制成功");
//4.关闭资源
//外层的流的关闭
bos.close();
bis.close();
//内层的流的关闭
fos.close();
fis.close();
}
}
package cd_one.code18.exer4;
import org.junit.Test;
import java.io.*;
public class CopyFileTest {
@Test
public void testSpendTime(){
long start = System.currentTimeMillis();
String src = "D:\\A新放\\70-标准流.mp4";
String dest = "D:\\A新放\\73-标准流.mp4";
copyFileWithFileSteam(src,dest);
copyFileWithBufferedSteam(src,dest);
long end = System.currentTimeMillis();
System.out.println("花费的时间为:" + (end - start));
}
/**
* 使用BufferedInputStream、BufferedOutputStream来复制这个文件
*/
public void copyFileWithBufferedSteam(String src, String dest) {
FileInputStream fis = null;
FileOutputStream fos = null;
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File(src);
File destFile = new File(dest);
//2.创建相关的字节流
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
//3.数据的读入和写出
byte[] butter = new byte[1024];
int len;
while ((len = bis.read(butter)) != -1) {
bos.write(butter, 0, len);
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.关闭资源
//外层的流的关闭
try {
if (bos != null) {
bos.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bis != null) {
bis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/**
* 使用FileInputStream、FileOutputStream复制文件
*/
public void copyFileWithFileSteam(String src, String dest) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
//1.创建相关的File类的对象
File srcFile = new File(src);
File destFile = new File(dest);
//2.创建相关的字节流
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
//3.数据的读入和写出
byte[] butter = new byte[1024];//1kb
int len;
while ((len = fis.read(butter)) != -1) {
fos.write(butter, 0, len);
}
System.out.println("复制成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
package cd_one.code18.exer4;
import org.junit.Test;
import java.io.*;
/**
* 测试BufferedReader和BufferedWriter
*/
public class BufferedReadWriterTest {
/**
* 使用BufferedReader和BufferedWriter将dbcp——ytf-8.txt中的内容显示在控制台上。
*/
@Test
public void test() throws IOException {
File file = new File("dbcp_utf-8.txt");
BufferedReader br = new BufferedReader(new FileReader(new File("dbcp_utf-8.txt")));
//读取的过程
// char[] cButter = new char[1024];
// int len;
// while((len = br.read(cButter)) != -1){
for (int i = 0; i < len; i++) {
System.out.println(cButter[i]);
}
// //方法2:
String str = new String(cButter,0,len);
System.out.println(str);
// }
//方式2:readLine()
String data;
while((data = br.readLine()) != null){
System.out.print(data+"\n");
}
br.close();
}
/**
* 使用BufferedReader和BufferedWriter实现文本文件的复制
*/
@Test
public void test2() throws IOException {
//1。造文件,造流
File file1 = new File("dbcp_utf-8.txt");
File file2 = new File("dbcp_utf-8_copy.txt");
BufferedReader br = new BufferedReader(new FileReader(file1));
BufferedWriter bw = new BufferedWriter(new FileWriter(file2));
//2.文件读写
String data;
while((data = br.readLine()) != null){
bw.write(data);
bw.newLine();//表示换行操作
bw.flush();//刷新的方法。每当调用此方法时,就会主动的将内存中的数据写到磁盘文件中。
}
System.out.println("复制成功");
// 3.关闭资源
// br.close();
// bw.close();
}
}
180-File类与IO流-处理流之2:转换流的使用及各种字符集的讲解
package cd_one.code18.exer4;
import org.junit.Test;
import java.io.*;
public class InputStreamReaderTest {
@Test
public void test() throws IOException{
//1.创建File对象
File file1 = new File("dbcp_utf-8.txt");
//2.创建流对象
FileInputStream fis = new FileInputStream(file1);
InputStreamReader isr = new InputStreamReader(fis,"gbk");
//3.读入操作
char[] cButter = new char[1024];
int len;
while((len = isr.read(cButter)) != -1){
String str = new String(cButter,0,len);
System.out.println(str);
}
isr.close();
}
@Test
public void test2() throws IOException {
//1.创建File对象
File file1 = new File("dbcp_gbk.txt");
File file2 = new File("dbcp_gbk_to_utf-8.txt");
//2.创建流对象
FileInputStream fis = new FileInputStream(file1);
InputStreamReader isr = new InputStreamReader(fis,"GBK");
FileOutputStream fos = new FileOutputStream(file2);
OutputStreamWriter osw = new OutputStreamWriter(fos,"utf8");
//3.读入操作
char[] cButter = new char[1024];
int len;
while((len = isr.read(cButter)) != -1){
String str = new String(cButter,0,len);
System.out.println(str);
}
System.out.println("操作完成");
isr.close();
osw.close();
}
}
181File类与IO流-处理流之3:对象流的使用及对象的序列化机制
package cd_one.code18.exer5;
import org.junit.Test;
import java.io.*;
public class ObjectInputOutputStreamTest {
/**
*
* 序列化过程:使用ObjectOutputStream流实现,将内存中的java对象保存在文件中或者通过网络传输出去
*
*/
@Test
public void test() throws IOException {
//1.
File file = new File("object.txt");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(file));
//2.写出数据即为序列化的过程
oos.writeUTF("江山如此多娇,引得无数英雄尽折腰。");
oos.flush();
oos.writeObject("轻轻的我走了,正如我轻轻地来");
oos.flush();
oos.close();
}
@Test
public void test2() throws IOException, ClassNotFoundException {
//1.
File file = new File("object.txt");
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
//2.读取文件中的对象
String str1 = ois.readUTF();
System.out.println(str1);
String str2 = (String) ois.readObject();
System.out.println(str2);
//3.
ois.close();
}
//演示自定类的序列化和反序列化的过程
@Test
public void test3() throws IOException {
//1.
File file = new File("object1.dat");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(file));
//2.写出数据即为序列化的过程
Person p1 = new Person("Tom",12);
oos.writeObject(p1);
oos.flush();
//3
oos.close();
}
//演示自定类的反序列化的过程
@Test
public void test4() throws IOException, ClassNotFoundException {
//1.
File file = new File("object1.dat");
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
//2.读取文件中的对象
Person person = (Person) ois.readObject();
System.out.println(person);
//Person{name='Tom', age=12}
//3.
ois.close();
}
}
package cd_one.code18.exer5;
import org.junit.Test;
import java.io.*;
public class ObjectInputOutputStreamTest {
/**
*
* 序列化过程:使用ObjectOutputStream流实现,将内存中的java对象保存在文件中或者通过网络传输出去
*
*/
@Test
public void test() throws IOException {
//1.
File file = new File("object.txt");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(file));
//2.写出数据即为序列化的过程
oos.writeUTF("江山如此多娇,引得无数英雄尽折腰。");
oos.flush();
oos.writeObject("轻轻的我走了,正如我轻轻地来");
oos.flush();
Person p2 = new Person("Jerry",23,1001,new Account(2000));//Account
oos.writeObject(p2);
oos.flush();
oos.close();
}
@Test
public void test2() throws IOException, ClassNotFoundException {
//1.
File file = new File("object.txt");
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
//2.读取文件中的对象
String str1 = ois.readUTF();
System.out.println(str1);
String str2 = (String) ois.readObject();
System.out.println(str2);
//3.
ois.close();
}
//演示自定类的序列化和反序列化的过程
@Test
public void test3() throws IOException {
//1.
File file = new File("object1.dat");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(file));
//2.写出数据即为序列化的过程
Person p1 = new Person("Tom",12);
oos.writeObject(p1);
oos.flush();
Person p2 = new Person("Jerry",23,1,new Account(2000));
oos.writeObject(p2);
oos.flush();
//3
oos.close();
}
//演示自定类的反序列化的过程
@Test
public void test4() throws IOException, ClassNotFoundException {
//1.
File file = new File("object1.dat");
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
//2.读取文件中的对象
Person person = (Person) ois.readObject();
System.out.println(person);
//Person{name='Tom', age=12}
Person person1 = (Person) ois.readObject();
System.out.println(person1);
// Person{name='Jerry', age=23, id=1, account=Account{balance=2000.0}}
//3.
ois.close();
}
}
182File类与IO流-其它流的使用_第15章复习与企业真题