0. Generate matrices A, with random Gaussian entries, B, a Toeplitz matrix, where A ∈Rn×m and B ∈Rm×m, for n = 200, m = 500.
import numpy as np
from scipy.linalg import toeplitz
#0__construct A snd B
n = 10
m = 15
A = np.random.randn(n,m)
print(A)
r = np.random.randint(0,10,size = m)
c = np.random.randint(0,10,size = m)
B = toeplitz(r,c)
print(B)
为了操作方便,所以先用n = 10,m = 15,打印了A和B,利用了numpy里的randn和randint,randn时标准正态分布,randint是离散分部的整数值。toeplitz(r,c)是scipy里的一个函数,可用来生成toeplitz矩阵。如果r[0]和c[0]不同则得到矩阵的(0,0)位使用r[0];
得到的矩阵如下:
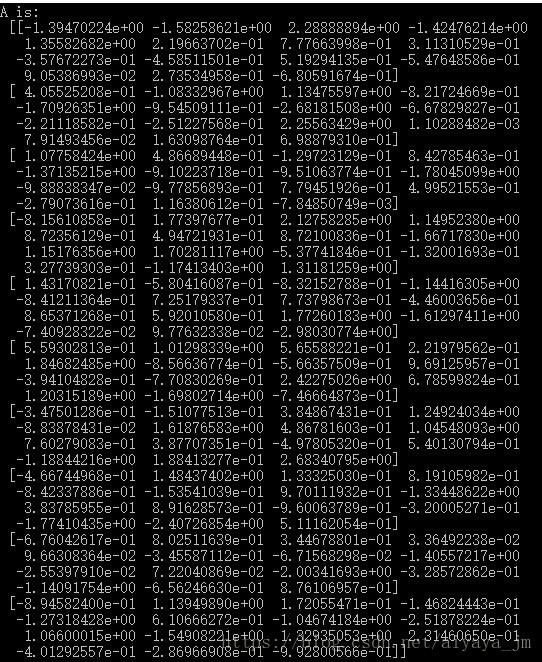