import java.util.UUID;
import java.util.concurrent.LinkedBlockingQueue;
public class LinkedBlockingQueueTest {
public static void main(String[] args) {
try {
LinkedBlockingQueue<String> linkedBlockingQueue = new LinkedBlockingQueue<String>(10);
new Thread(){
@Override
public void run() {
try {
while (true){
String msg = Thread.currentThread().getName() + " --> "+ UUID.randomUUID().toString();
boolean result = false;
// add 增加时,如果队列满了,就报错
result = linkedBlockingQueue.add(msg);
System.out.println(result+" --> add增加后的数量:"+linkedBlockingQueue.size());
msg = Thread.currentThread().getName()+" --> "+UUID.randomUUID().toString();
// put 增加时,如果队列满了,就阻塞
linkedBlockingQueue.put(msg);
System.out.println("put增加后的数量:"+linkedBlockingQueue.size());
// put 增加时,如果队列满了,不报错也不阻塞,只返回false
result=linkedBlockingQueue.offer(msg);
System.out.println(result+" --> offer增加后的数量:"+linkedBlockingQueue.size());
String d = null;
Thread.sleep(1000);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}.start();
new Thread(){
@Override
public void run() {
try {
String msg = null;
while (true){
// peek 获取后,只是窥探,并不把数据从队列中移除
msg = linkedBlockingQueue.peek();
System.out.println("peek获取后的数量:"+linkedBlockingQueue.size());
// poll 获取后,会把元素从队列中移除,队列为0时,不报错也不阻塞
msg = linkedBlockingQueue.poll();
System.out.println("poll获取后的数量:"+linkedBlockingQueue.size());
// poll 获取后,会把元素从队列中移除,队列为0时,不报错也不阻塞
msg = linkedBlockingQueue.take();
System.out.println("take获取后的数量:"+linkedBlockingQueue.size());
String d = null;
Thread.sleep(2000);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}.start();
}catch (Exception e){
e.printStackTrace();
}
}
}
LinkedBlockingQueue
最新推荐文章于 2024-10-09 08:50:17 发布
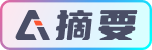