Note: When is_training is True the moving_mean and moving_variance need to be updated, by default the update_ops are placed in tf.GraphKeys.UPDATE_OPS so they need to be added as a dependency to the train_op, example:
update_ops = tf.get_collection(tf.GraphKeys.UPDATE_OPS)
with tf.control_dependencies(update_ops):
optimize_op = optimizer.minimize(optimize_target, global_step=self.global_step)
moving_mean and moving_variance 在做 val 和 test 时都需要,所以尽管训练时用到的是每个 batch 的 mean 和 variance,没有用到 moving_mean and moving_variance ,在训练时仍需要被计算。使用control_dependencies
语句的原因是在sess.run
中可以不写update_ops
,只需写sesss.run(optimize_op)
, updates_op
就会被被调用。
2,在使用 batch_norm
的偏置时,卷积可以不使用 bias_add
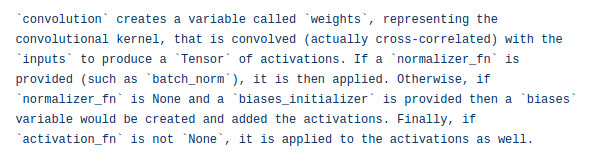
3,在使用 saver = tf.train.Saver()
必须在图构建完成之后申明,否则将不能保存模型参数。即应写为:
Mymodel()
saver = tf.train.Saver()
sess = tf.Session()
sess.run(tf.global_variables_initializer())
而不是:
saver = tf.train.Saver()
Mymodel()
sess = tf.Session()
sess.run(tf.global_variables_initializer())
博主在自己训练 u-net 时,采用了第二种方法,导致在使用训练的 u-net 做测试时会出现参数没有初始化
的报错信息。
4, tensorflow 中 Tensor 与 Variable 的区别: Tensor 不需要初始化,Variable需要初始化。区分两者的方法:Variable 会通过tf.Variable
或tf.get_variable
来显式定义。
A = tf.zeros([10]) # A is a Tensor
B = tf.Variable([111, 11, 11]) # B is a Variable
sess.run(A) # OK. Will return the values in A
sess.run(B) # Error.