处理 JSON (使用MessageConverter)
1. 加入 jar 包
jackson-annotations-2.6.0-xh.jar
jackson-core-2.6.0-xh.jar
jackson-databind-2.6.0-xh.jar
2. 前端页面
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="scripts/jquery-1.9.1.min.js"></script>
<script type="text/javascript">
$(function() {
$("#testJson").click(function(){
var url = this.href;
var args = {};
$.post(url, args, function(data){
for(var i=0; i<data.length; i++){
alert("id: "+data[i].id+"lastName: "+data[i].lastName);
}
})
return false;
})
})
</script>
</head>
<body>
<a href="emps">List All Employees</a>
<br>
<a href="testJson" id= "testJson">Test Json</a>
</body>
</html>
3. 后端处理
@Controller
public class SpringMVCTest {
@Autowired
private EmployeeDao employeeDao;
@ResponseBody
@RequestMapping("/testJson")
public Collection<Employee> testJson(){
return employeeDao.getAll();
}
}
使用 MessageConverter
1. 简介
使用 HttpMessageConverter<T> 将请求信息转化并绑定到处理方法的入参中或将响应结果转为对应类型的响应信息,Spring 提供了两种途径:
- 使用 @RequestBody / @ResponseBody 对处理方法进行标注
- 使用 HttpEntity<T> / ResponseEntity<T> 作为处理方法的入参或者返回值
当控制处理方法使用到 @RequestBody / @ResponseBody 或者 HttpEntity<T> / ResponseEntity<T>时,Spring 首先根据请求头或响应头的 Accept 属性选择匹配的 HrrpMessageConverter,进而根据参数类型或泛型类型的过滤得到匹配的 HttpMessageConverter,若找不到可用的 HttpMessageConverter 将报错。
@RequestBody 和 ResponseBody 不需要成对出现
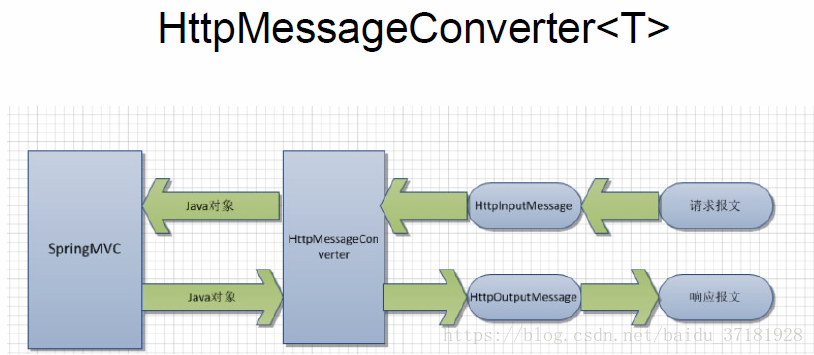
2. HttpMessageConverter原理
public interface HttpInputMessage extends HttpMessage {
InputStream getBody() throws IOException;
}
public interface HttpOutputMessage extends HttpMessage {
OutputStream getBody() throws IOException;
}
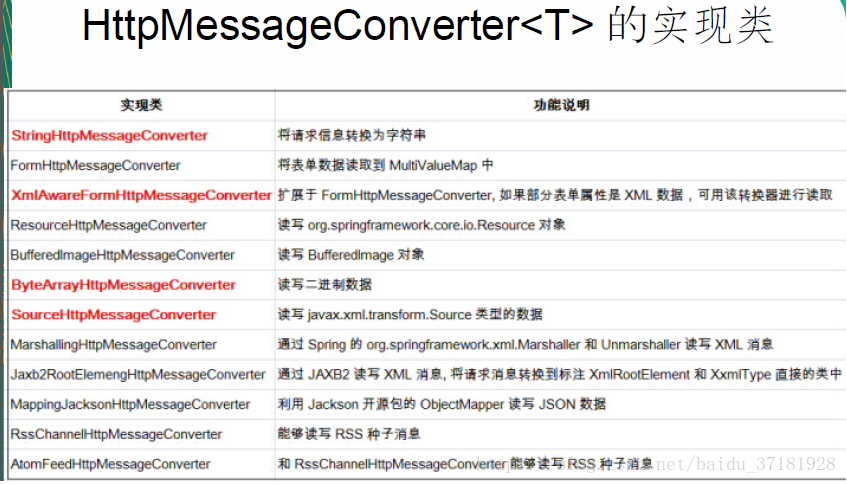
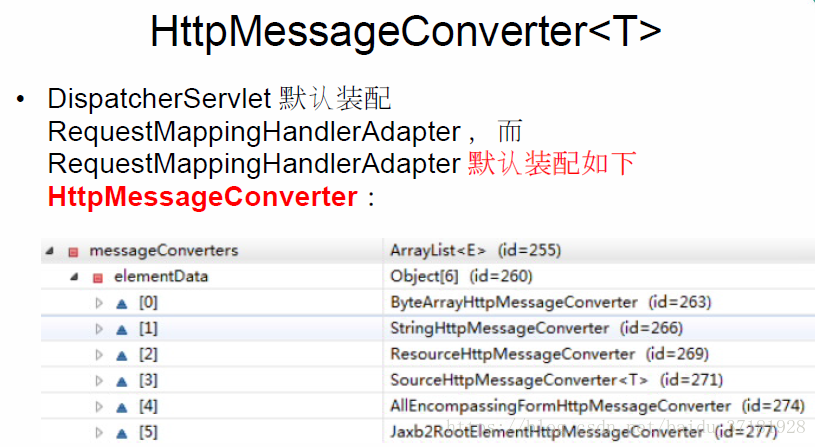
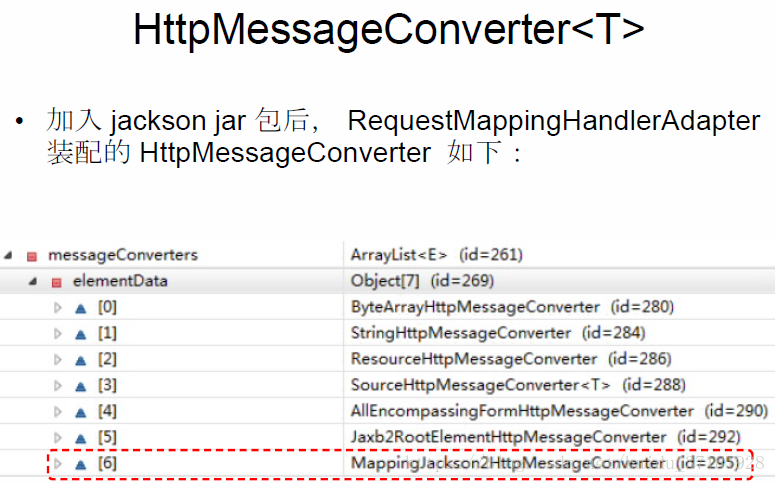
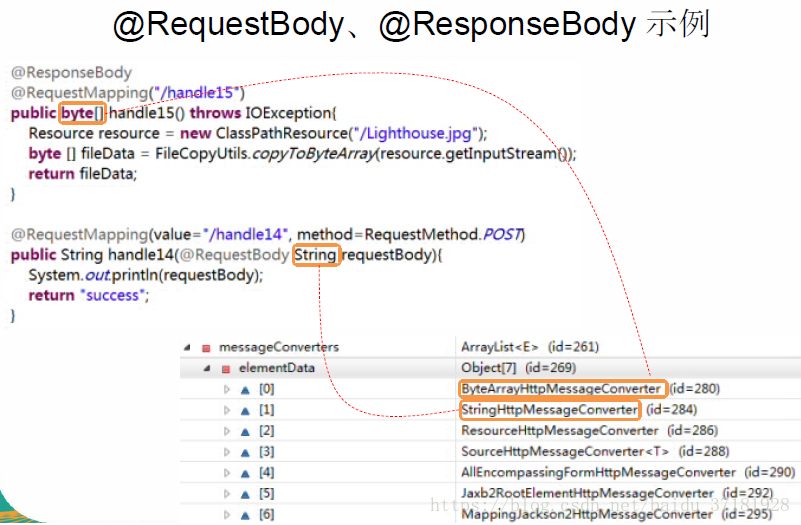
3. 文件上传实例
<form action="testHttpMessageConverter" method="post" enctype="multipart/form-data">
File:<input type="file" name="file"><br>
desc:<input type="text" name="desc"><br>
<input type="submit" value="Submit">
</form>
@ResponseBody
@RequestMapping("/testHttpMessageConverter")
public String testHttpMessageConverter(@RequestBody String body) {
System.out.println(body);
return "hello httpMessageConverter -" + new Date();
}
显示效果 选择一个写了三行a的文本文件
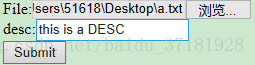
控制台输出
-----------------------------7e2f93a20830
Content-Disposition: form-data; name="file"; filename="C:\Users\51618\Desktop\a.txt"
Content-Type: text/plain
aaaaaaaaaa
aaaaaaaaaa
aaaaaaaaaa
-----------------------------7e2f93a20830
Content-Disposition: form-data; name="desc"
this is a DESC
-----------------------------7e2f93a20830--
4. 使用ResponseEntity实例
<a href="testResponseEntity">Test ResResponseEntity</a>
@RequestMapping("/testResponseEntity")
public ResponseEntity<byte[]> testResResponseEntity(HttpSession session) throws IOException{
byte[] body = null;
ServletContext servletContext = session.getServletContext();
InputStream in = servletContext.getResourceAsStream("/files/abc.txt");
body = new byte[in.available()];
in.read(body);
HttpHeaders headers = new HttpHeaders();
headers.add("anqi","anqi");
HttpStatus statusCode = HttpStatus.OK;
ResponseEntity<byte[]> response = new ResponseEntity<byte[]>(body, headers, statusCode);
return response;
}
显示结果
http://localhost:8080/Springmvc-2/testResponseEntity
hello
this is a file
yes txt