/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode swapPairs(ListNode head) {
if(head == null || head.next == null){
return head;
}
ListNode dummy = new ListNode(0);
dummy.next = head;
ListNode current = dummy;
while(current.next!= null && current.next.next != null){
ListNode first = current.next;
ListNode second = current.next.next;
current.next = second;
first.next = second.next;
second.next = first;
current = current.next.next;
}
return dummy.next;
}
}
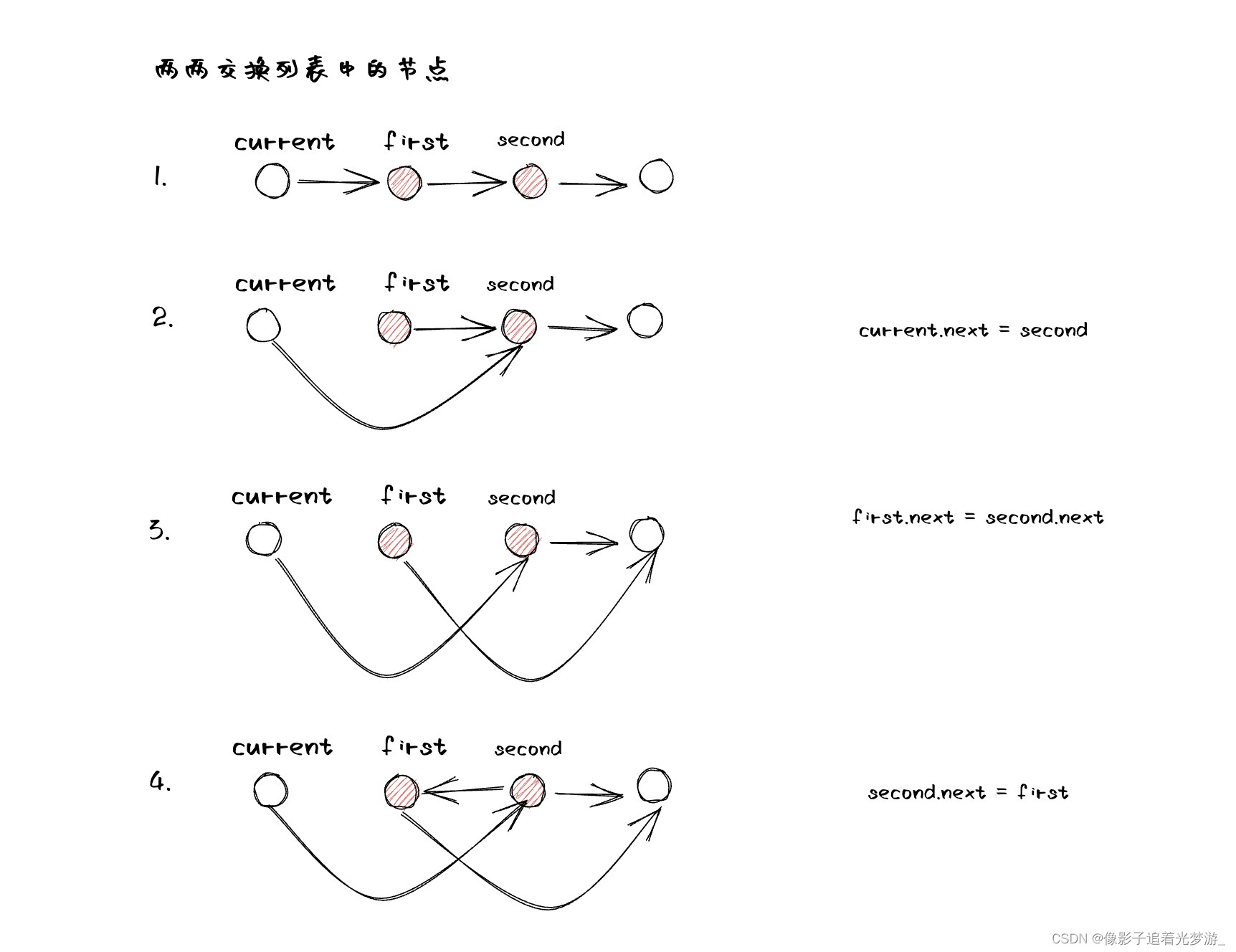
19.删除链表的倒数第N个节点
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode removeNthFromEnd(ListNode head, int n) {
if(head == null){
return head;
}
int length = 1;
ListNode current = head;
while(current.next!= null){
length++;
current = current.next;
}
System.out.println(length);
if(length == n){
return head.next;
}
ListNode p = head;
for(int i = 1; i <= length - n -1; i++){
p = p.next;
}
p.next = p.next.next;
return head;
}
}
-
如果头结点
head
为空,直接返回,因为一个空链表没有节点可以删除。 -
用
current
指针遍历链表,一边遍历一边计数,得到链表的总长度length
。 -
如果链表的长度恰好等于
n
,说明要删除的是头结点。因此,返回head.next
即可,此时原链表的第二个节点成为了新链表的头结点。 -
如果要删除的不是头结点,那么再次从头结点开始,遍历链表。这次只遍历到“第(length - n - 1)个节点”,这个节点就是要删除节点的前一个节点。将这个节点的
next
指向next.next
,就相当于删除了下一个节点。 -
最后返回头结点
head
。
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
// 两个指针分别从头节点headA headB 开始遍历
// 当一个指针到达链表末尾时 就将它已到另一个链表的头部继续遍历
// 这样两个指针会相遇在相交节点
if(headA == null || headB == null){
return null;
}
ListNode pA = headA;
ListNode pB = headB;
while(pA != pB){
if(pA == null){
pA = headB;
} else {
pA = pA.next;
}
if(pB == null){
pB = headA;
} else {
pB = pB.next;
}
}
return pA;
}
}
/**
* Definition for singly-linked list.
* class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
public class Solution {
public ListNode detectCycle(ListNode head) {
// 检查边界
if(head == null || head.next == null){
return null;
}
// 初始化慢指针和快指针
ListNode slow = head;
ListNode fast = head;
// floyd算法 找到相遇点
while( fast != null && fast.next != null){
// 慢指针移动一步
slow = slow.next;
// 快指针移动两步
fast = fast.next.next;
// 如果慢指针和快指针相遇,说明存在环
if(slow == fast){
break;
}
}
// 如果不存在环,返回null
if( fast == null || fast.next == null){
return null;
}
// 将慢指针移回链表头部, 寻找环的入口
// 当慢指针和快指针再次相遇时,相遇点即为环的入口
slow = head;
while(slow != fast){
slow = slow.next;
fast = fast.next;
}
// 返回环的入口节点
return slow;
}
}