开发环境
开发语言为Java,开发环境Eclipse或者IDEA都可以。数据库采用:MySQL。运行主程序,或者执行打开JAR文件即可以运行本程序。
系统框架
利用JDK自带的SWING框架开发,不需要安装第三方JAR包。MySQL数据库,纯窗体模式,直接运行Main文件即可以。同时带有详细得设计文档
主要功能
主要角色
系统主要分为:企业用户和求职者两大类
主要功能点
普通用户
1 注册:注册成为系统中的合法用户
2 登陆系统
3 完善简历:
基本资料:姓名、邮箱、性别、状态、电话、住址
职业意向:期望行业,期望职位、期望地点、期望薪水
工作经历:工作地点、工作职位
教育经历:毕业学校、专业名称、学历
语言能力:普通话、英语、法语、粤语、日语、法语、德语
自我评价
附加信息
擅长技能
4 搜索职位:根据工作城市、职位、薪水等条件搜索符合条件的职位信息
5 投递简历
企业用户
1 发布职位信息
2 赛选简历
3 完善公司信息。公司信息包括:
企业名称、企业所在地
企业性质
企业规模
联系人
联系人电话
系统实现效果
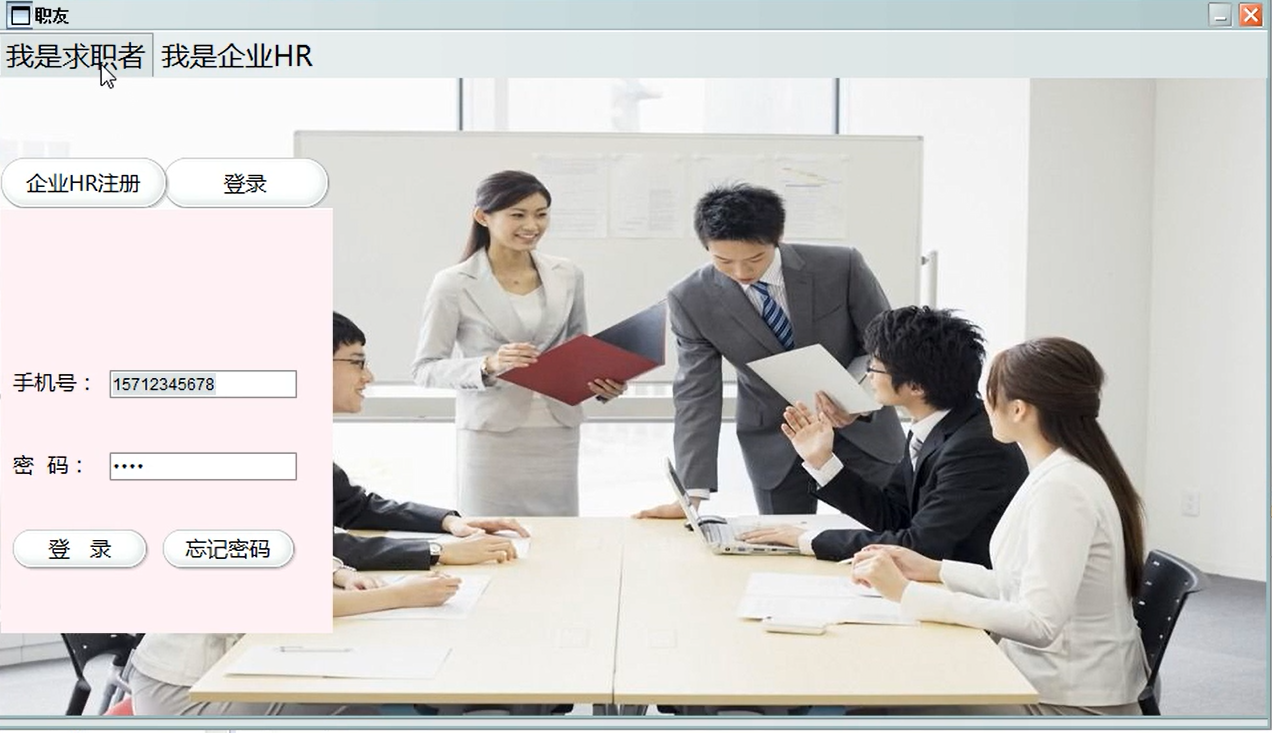
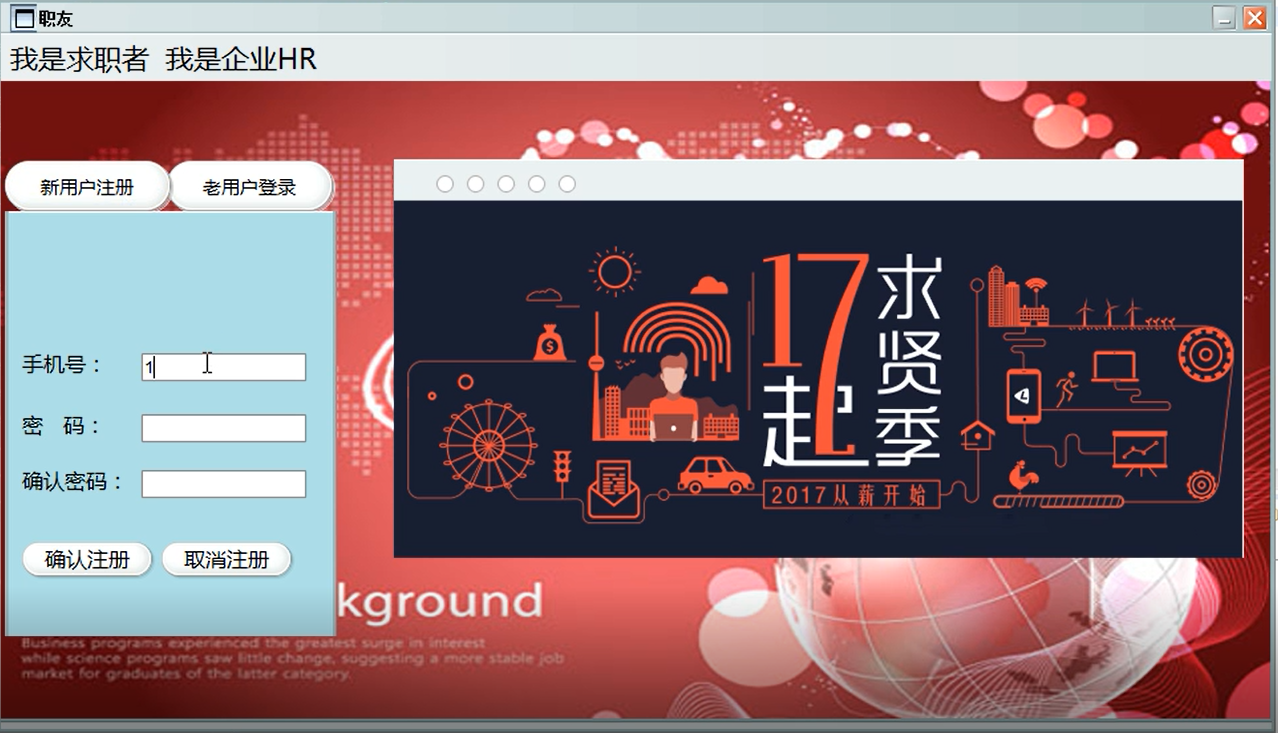
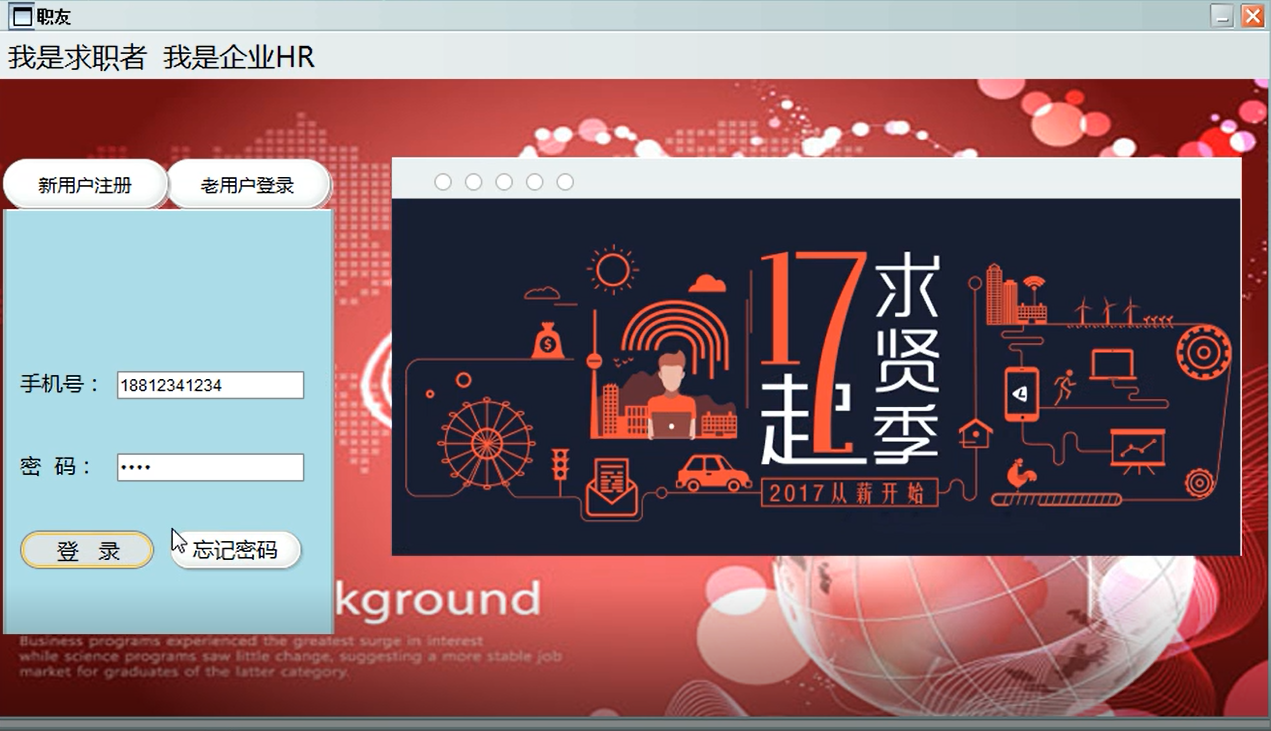
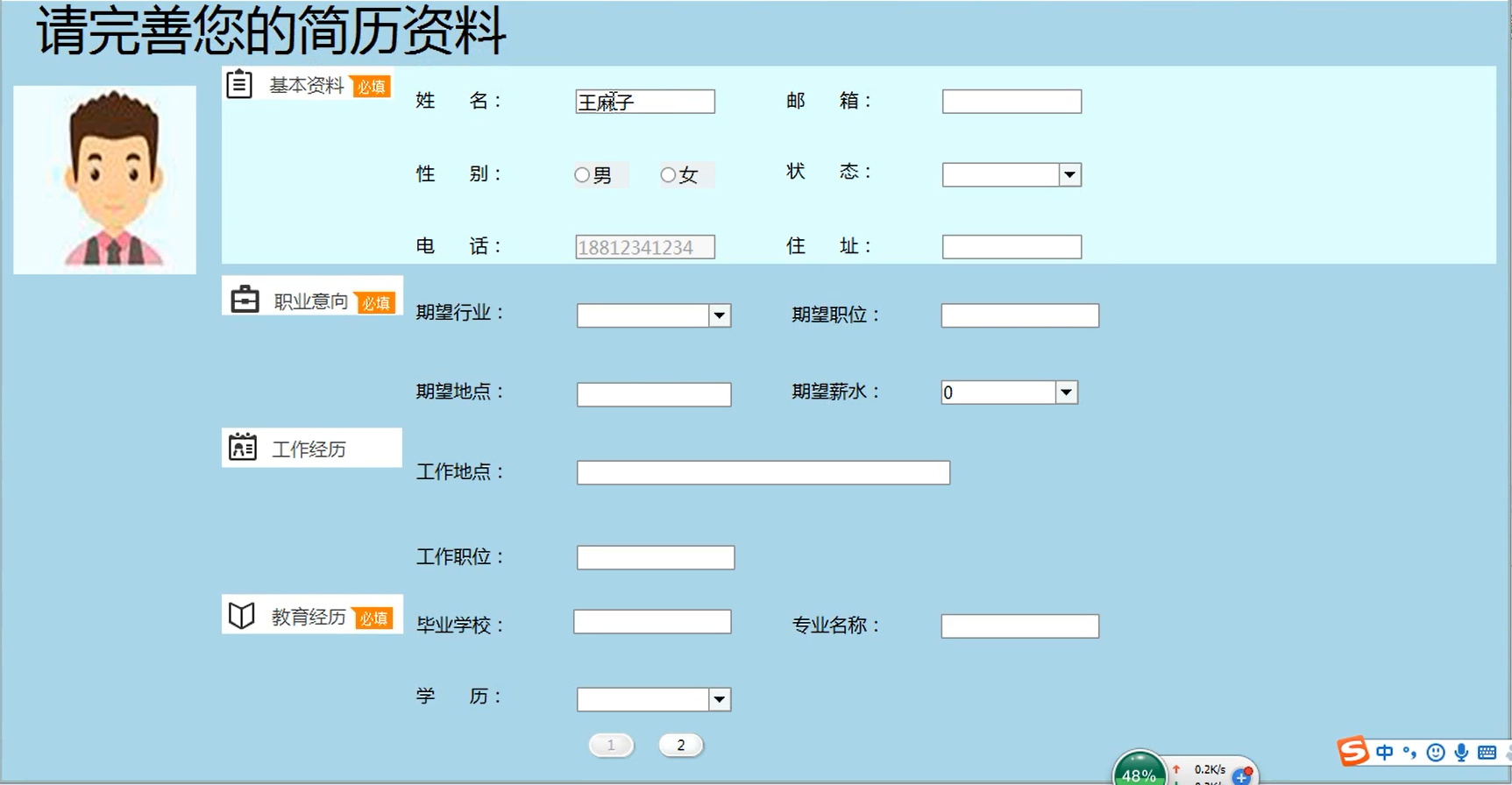
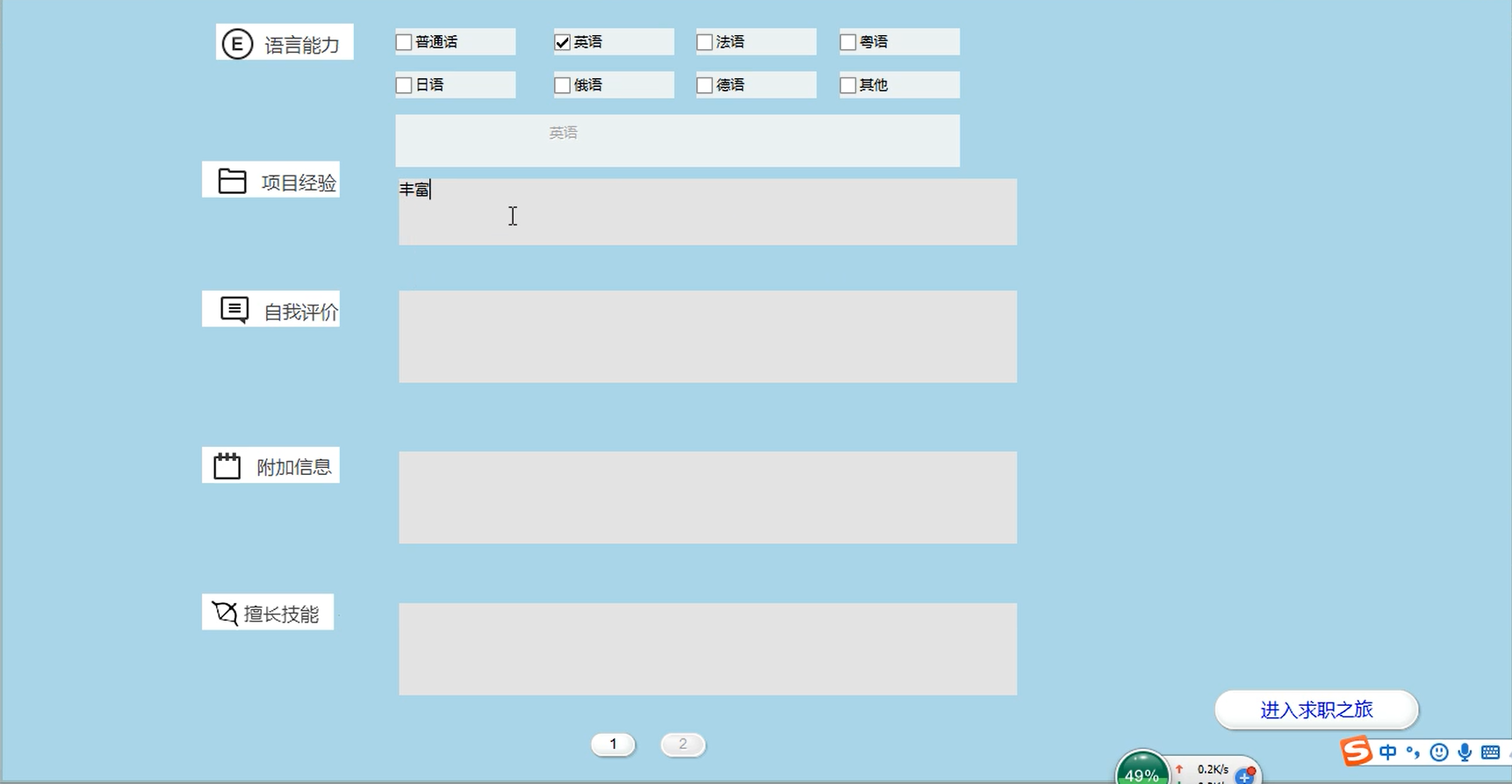
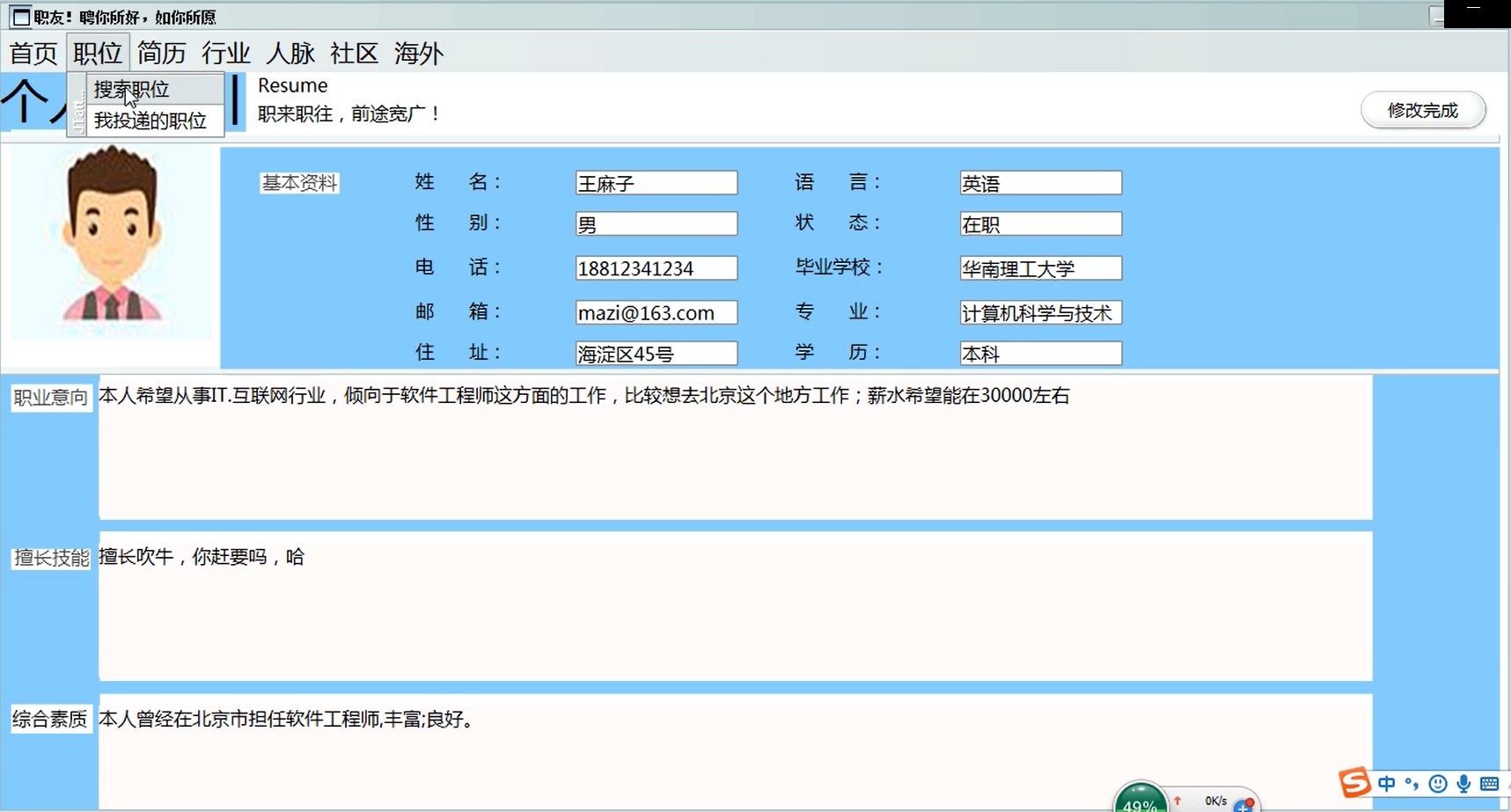
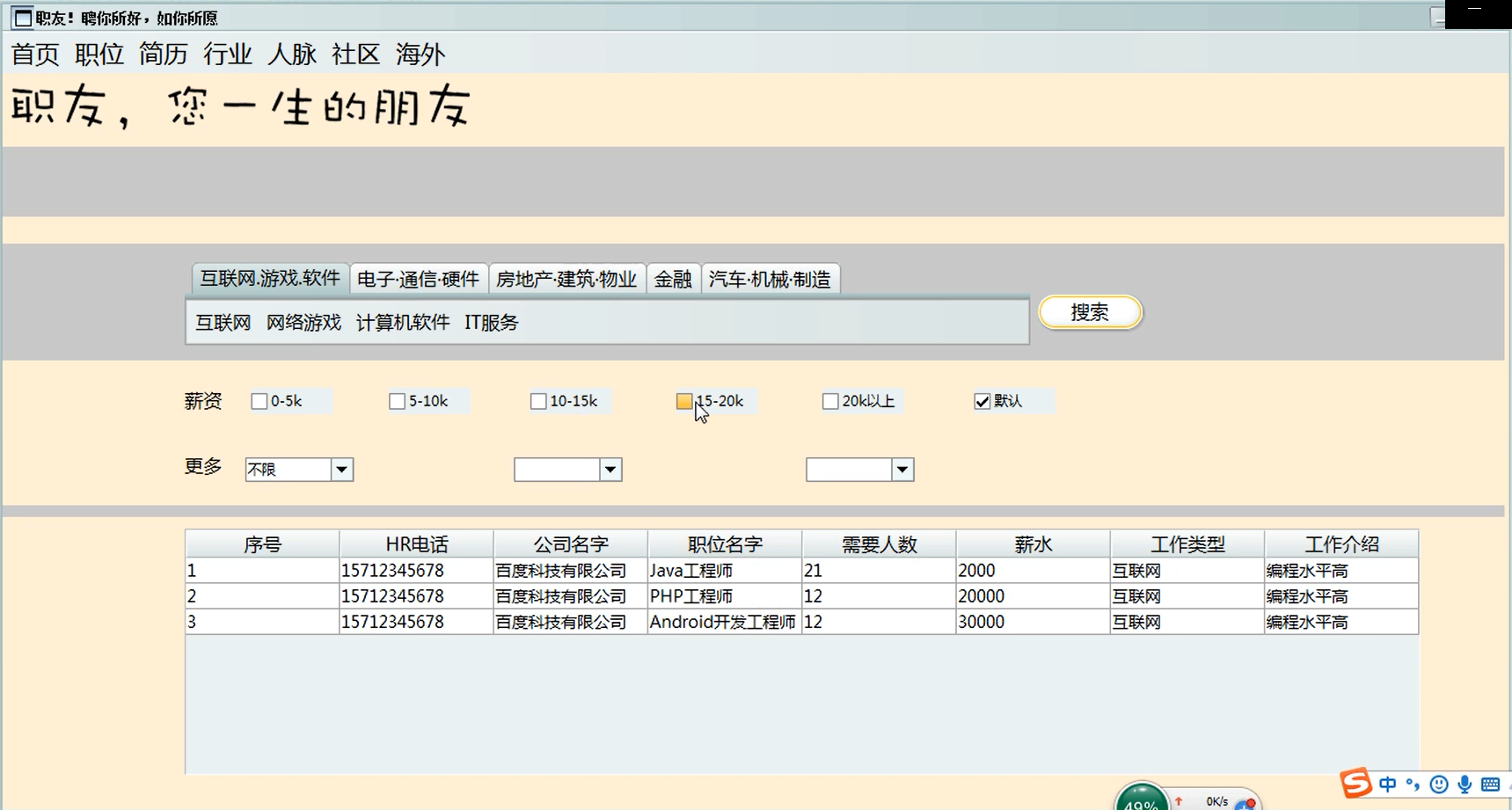
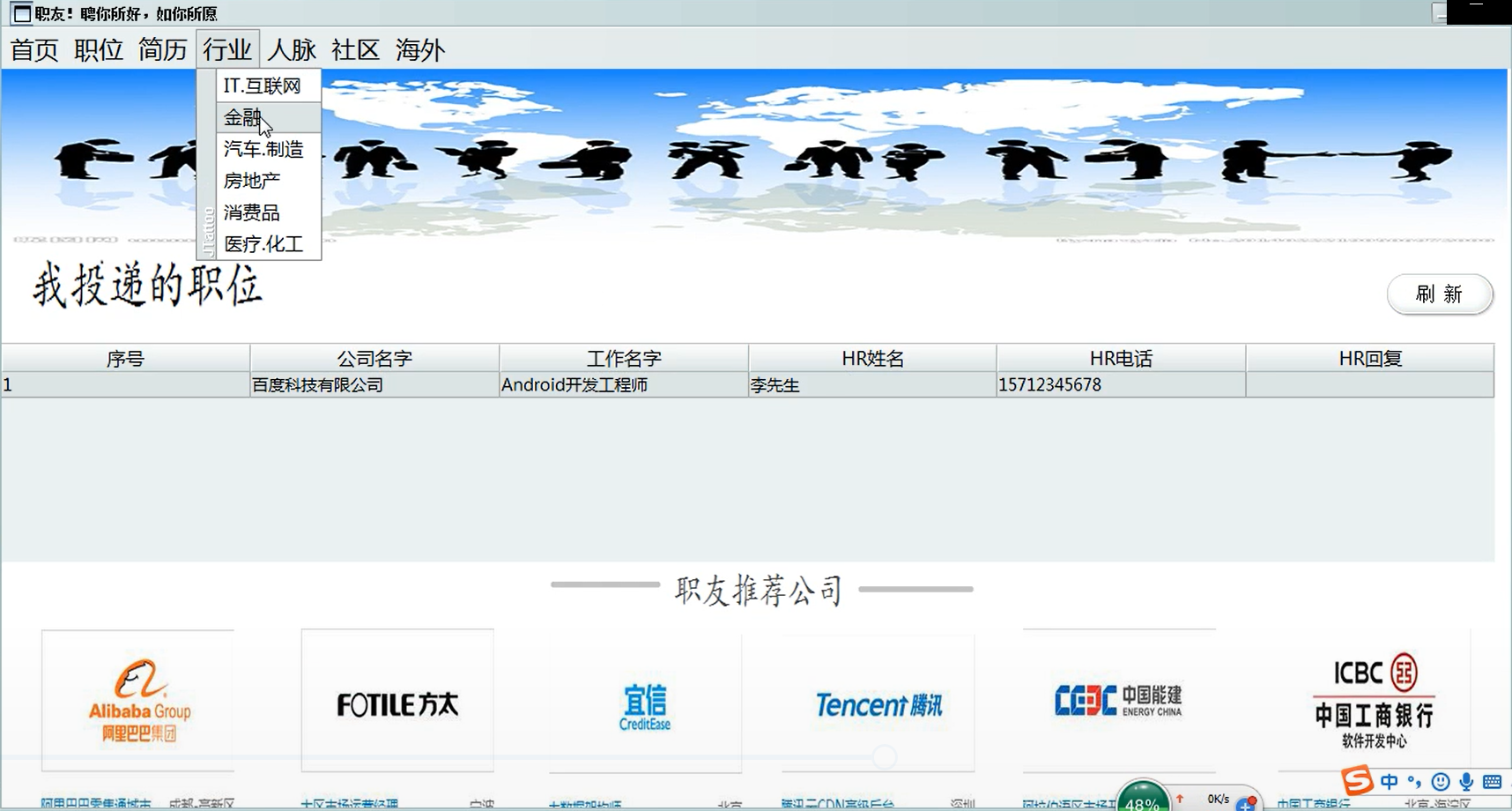
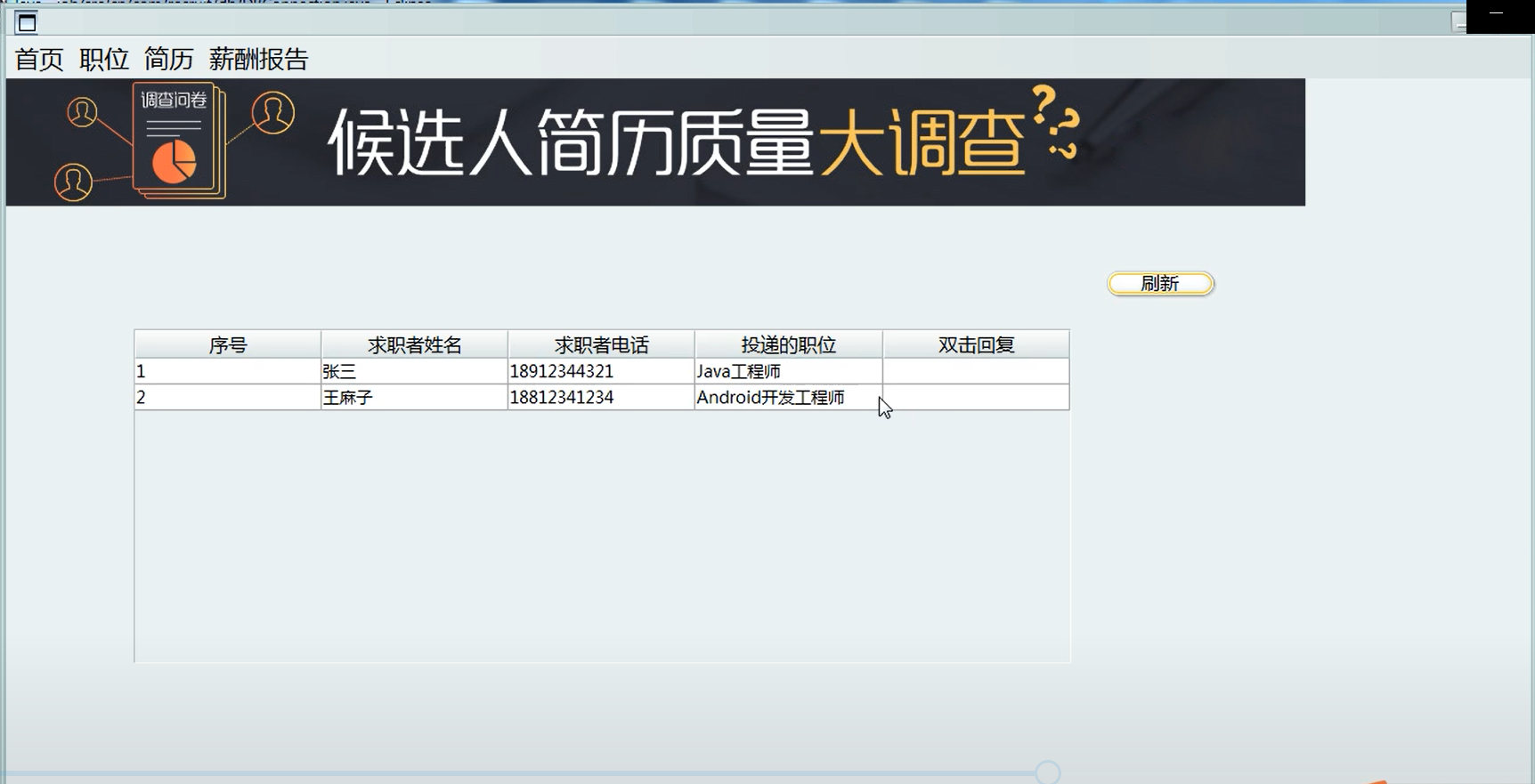
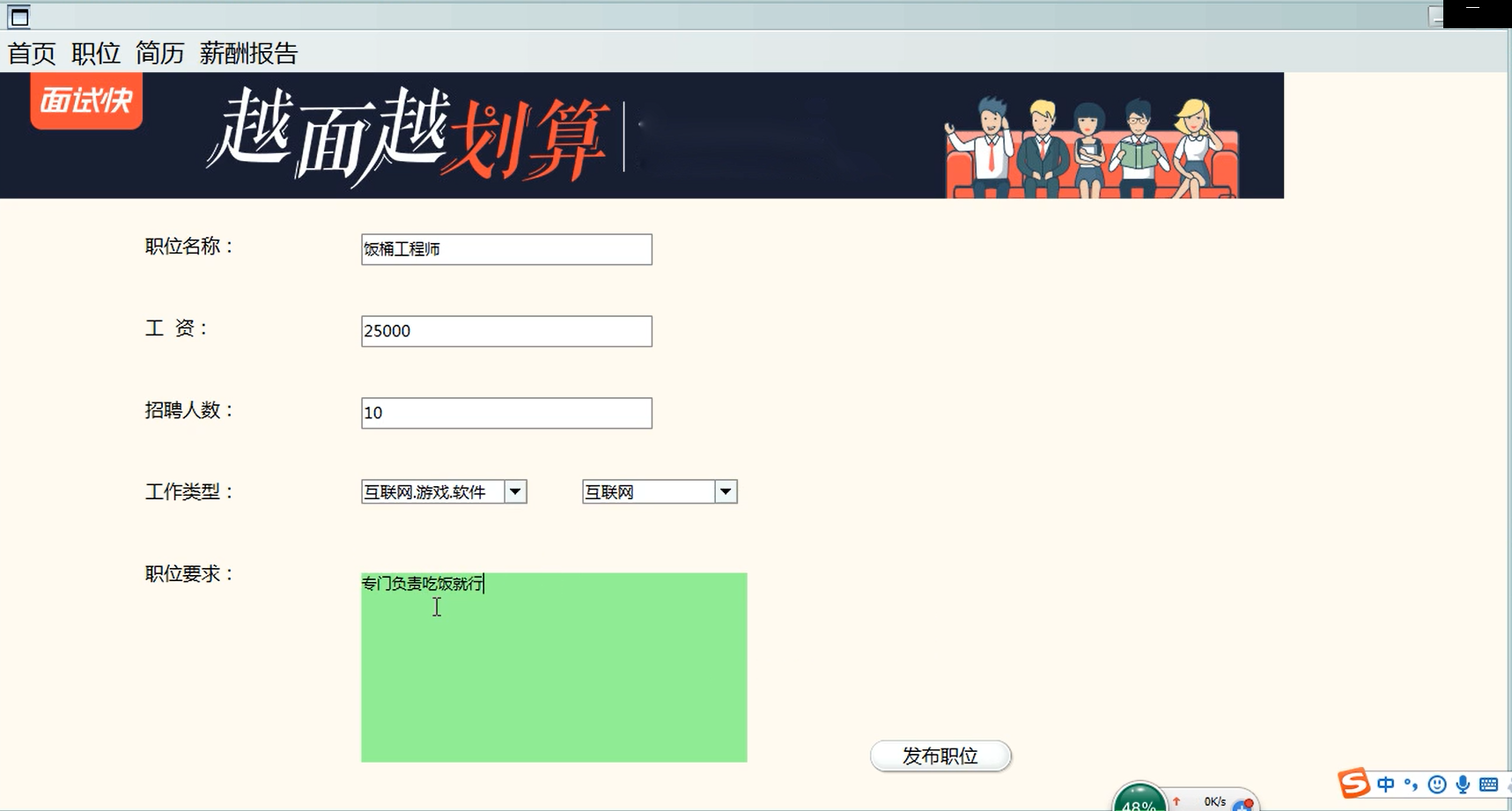
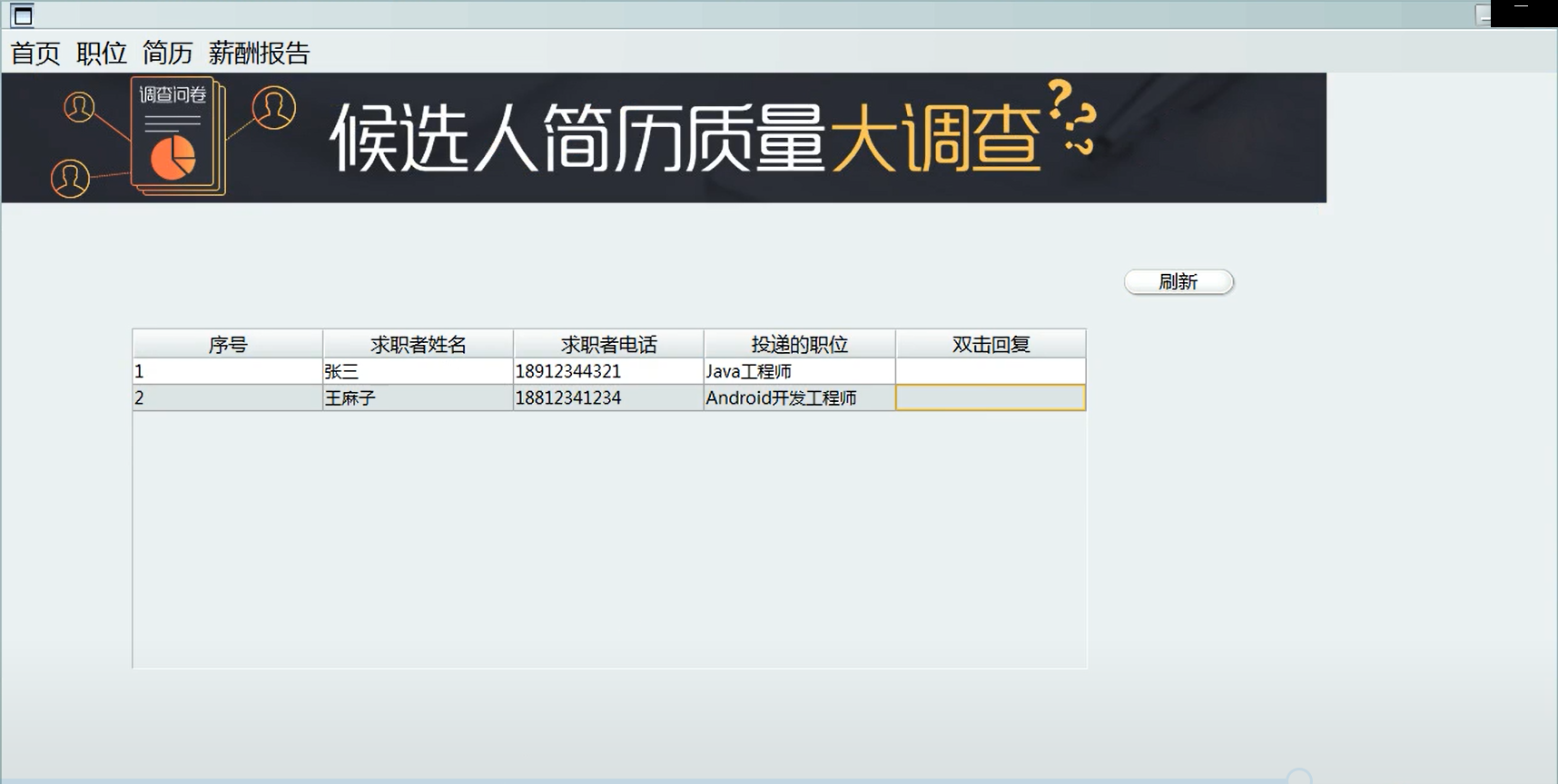
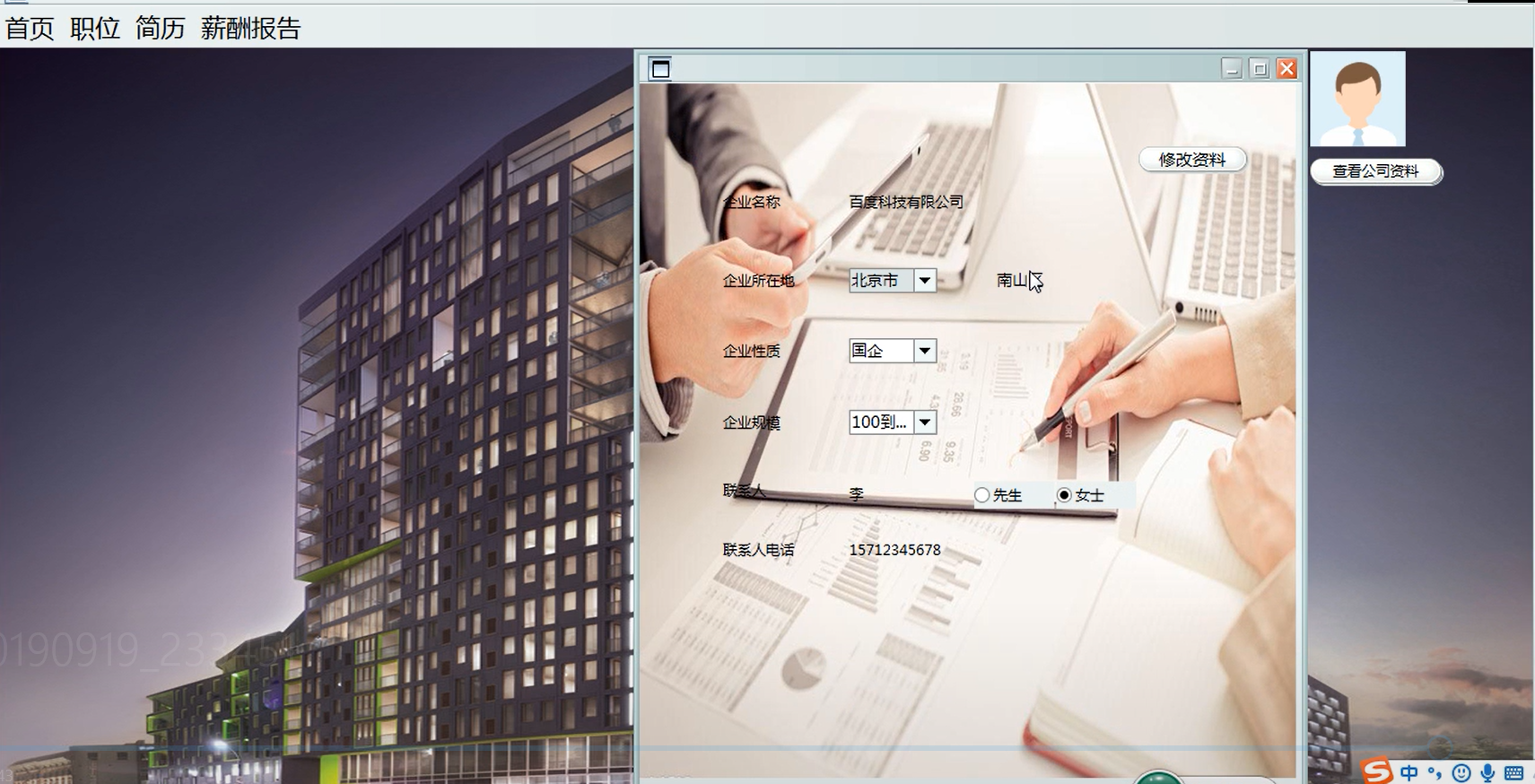
关键代码
package cn.com.recruit.ui.win;
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JDesktopPane;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import cn.com.recruit.ui.win.inter.candidate.CandidateWindow;
import cn.com.recruit.ui.win.inter.company.CompanyWindow;
import java.awt.Toolkit;
public class LoginWindow extends JFrame {
private JPanel contentPane;
private CandidateWindow log;
private CompanyWindow companySignin;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
javax.swing.UIManager.setLookAndFeel("com.jtattoo.plaf.mint.MintLookAndFeel");
// javax.swing.UIManager.setLookAndFeel("com.jtattoo.plaf.aluminium.AluminiumLookAndFeel");
// javax.swing.UIManager.setLookAndFeel("com.jtattoo.plaf.aero.AeroLookAndFeel");
// javax.swing.UIManager.setLookAndFeel("com.birosoft.liquid.LiquidLookAndFeel");
LoginWindow frame = new LoginWindow();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public LoginWindow() {
setFont(new Font("微软雅黑", Font.PLAIN, 14));
setIconImage(Toolkit.getDefaultToolkit().getImage(LoginWindow.class.getResource("/com/birosoft/liquid/icons/maximize.png")));
setResizable(false);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 963, 553);
setTitle("\u804C\u53CB");
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JDesktopPane desktopPane = new JDesktopPane();
desktopPane.setBounds(0, 0, 980, 520);
contentPane.add(desktopPane);
log = new CandidateWindow();
log.setSize(980, 511);
log.setLocation(-21, -28);
log.setVisible(true);
desktopPane.add(log);
companySignin = new CompanyWindow();
companySignin.setVisible(false);
companySignin.setBounds(-21, -28,980, 511);
desktopPane.add(companySignin);
JMenu menu_Hr = new JMenu("\u6211\u662F\u6C42\u804C\u8005");
menu_Hr.setFont(new Font("微软雅黑", Font.PLAIN, 21));
menu_Hr.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
log.setVisible(true);
companySignin.setVisible(false);
}
});
menuBar.add(menu_Hr);
JMenu menu_candidate = new JMenu("\u6211\u662F\u4F01\u4E1AHR");
menu_candidate.setFont(new Font("微软雅黑", Font.PLAIN, 21));
menu_candidate.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
log.setVisible(false);
companySignin.setVisible(true);
}
});
menuBar.add(menu_candidate);
}
}
public class DBConnection {
private static String url;
private static String userName;
private static String password;
//我把这里改成了单例模式,其他地方用dbcon 是直接用类点出来。
private static DBConnection dbcon = new DBConnection();
private DBConnection(){}
public DBConnection(int test){}
static {
try {
// 加载配置文件
Properties props = new Properties();
InputStream in = DBConnection.class.getClassLoader().getResourceAsStream("db.properties");
props.load(in);
// 读取连接信息
String driverName = props.getProperty("driverName");
url = props.getProperty("url");
userName = props.getProperty("userName");
password = props.getProperty("password");
// 加载驱动
Class.forName(driverName);
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 获取连接
*/
public Connection getConnection() throws Exception {
Connection conn = null;
// 获取连接
conn = DriverManager.getConnection(url, userName, password);
return conn;
}
public void close(Connection conn, Statement stmt, ResultSet rs) {
try {
if (rs != null)
rs.close();
if (stmt != null)
stmt.close();
if (conn != null)
conn.close();
} catch (Exception e) {
}
}
public static DBConnection getdbcon(){
return dbcon;
}
}