概念:
- map中所有元素都是pair类型
- pair中第一个元素为key(键值),起到索引作用,第二个元素为value(实值),起存储值的作用
- 所有元素都会根据key值进行自动排序,底层采用的是二叉树数据结构
优点:
- 根据key值能够快速找到对应value值
- map中的key值都是唯一的
常用操作:
- insert 插入元素
- at 通过键值访问元素
- [] 访问或插入元素
- empty 检查容器是否为空
- size 返回容器元素个数
- erase 擦除指定元素,可通过键值或迭代器
- clear 清除所有元素
#include <iostream>
#include <string>
#include <map>
void printMap(std::map<int, std::string>&m)
{
for (auto it = m.begin(); it != m.end(); ++it)
std::cout << "key = " << it->first << " " << "value = " << it->second << std::endl;
std::cout << std::endl;
}
int main()
{
std::map<int, std::string> m;
//插入方式
//insert方式
//第一种
m.insert(std::pair<int, std::string>(1, "xiaoZhao"));
//第二种
m.insert(std::make_pair(2, "xiaoQian"));
//第三种
m.insert(std::map<int, std::string>::value_type(3, "xiaoSun"));
//[]方式,[]既可用于访问也可用于插入,若map中不存在该键值,则进行插入;若存在,则对其实值进行赋值修改
//第四种
m[4] = "xiaoLi";
printMap(m);
if (m.empty()) {
std::cout << "容器为空" << std::endl << std::endl;
}
else {
std::cout << "容器大小:" << m.size() << std::endl << std::endl;
}
//通过at访问指定key值元素
std::cout << "key值为3的value = " << m.at(3) << std::endl << std::endl;
//通过find查找key是否存在,若存在则返回该键值的元素的迭代器,若不存在则返回end()迭代器
if (m.find(2) != m.end()) {
std::cout << "查找到该键: " << "key = " << m.find(2)->first << " value = " << m.find(2)->second << std::endl;
}
else {
std::cout << "不存在该键" << std::endl;
}
std::cout << std::endl;
std::cout << "===删除操作===" << std::endl;
//删除操作
//删除某一迭代器位置的元素
m.erase(m.begin());
printMap(m);
//按照key值删除元素
m.erase(2);
printMap(m);
//清空所有元素
m.erase(m.begin(), m.end());
m.clear();
std::cout << "容器大小:" << m.size() << std::endl;
system("pause");
return 0;
}
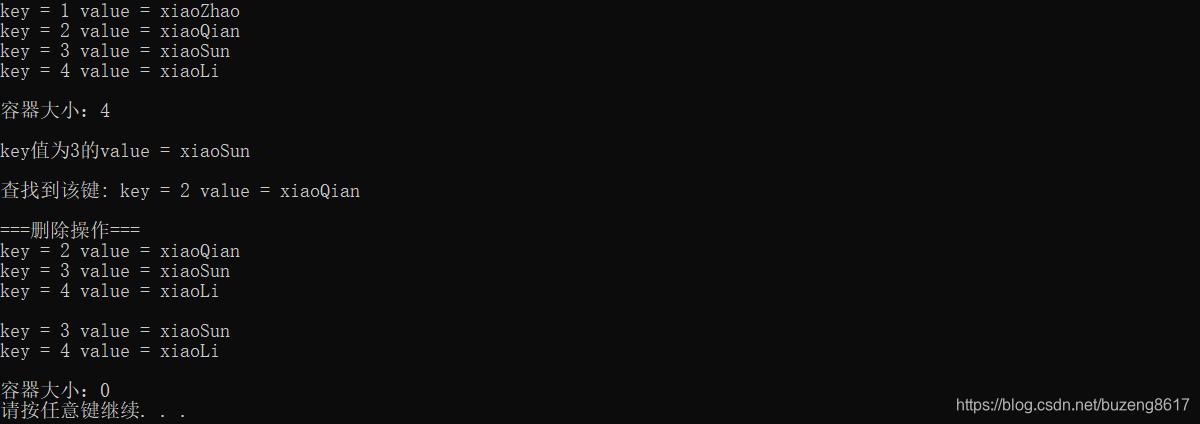