【C++】【设计模式】模板方法模式(Template Method Pattern)
定义
- 行为设计模式
- 一个抽象类公开定义了执行它的方法的方式/模板。它的子类可以按需要重写方法实现,但调用将以抽象类中定义的方式进行
结构
- 抽象类: 定义算法模版方法
- 具体子类:继承并实现具体的模版方法(有选择性的)
UML类图
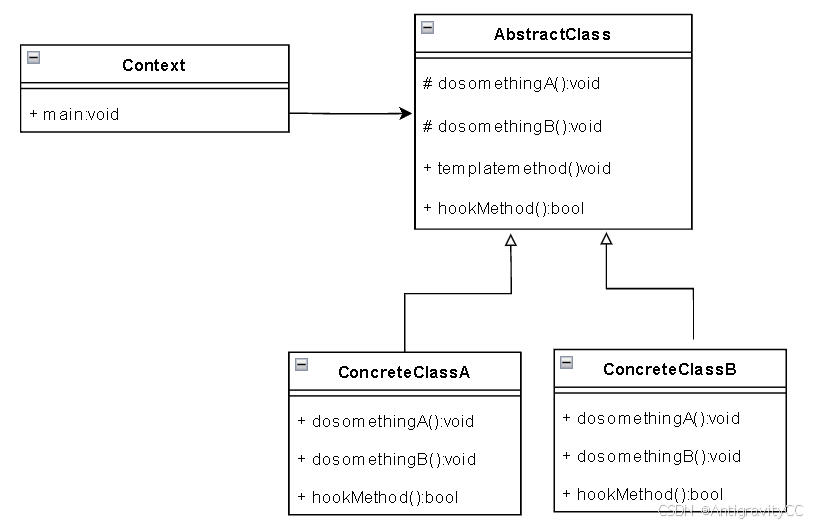
Demo源码
#include <iostream>
#include <memory>
using namespace std;
class CaffeineBeverage {
public:
virtual ~CaffeineBeverage() {}
// 模板方法,不允许子类修改
void prepareRecipe() {
boilWater();
brew();
pourInCup();
if (customerWantsCondiments()) {
addCondiments();
}
}
// 抽象方法,需要子类实现
virtual void brew() const = 0;
virtual void addCondiments() const = 0;
// 具体方法,不允许子类修改
void boilWater() const {
cout << "Boiling water" << endl;
}
void pourInCup() const {
cout << "Pouring into cup" << endl;
}
// 钩子方法,子类可以选择性的覆盖
virtual bool customerWantsCondiments() const {
return true;
}
};
class Coffee : public CaffeineBeverage {
public:
// 实现抽象方法
void brew() const override {
cout << "Dripping Coffee through filter" << endl;
}
void addCondiments() const override {
cout << "Adding Sugar and Milk" << endl;
}
// 重载钩子方法
bool customerWantsCondiments() const override {
char answer;
cout << "Would you like milk and sugar with your coffee (y/n)? " << endl;
cin >> answer;
return answer == 'y' || answer == 'Y';
}
};
class Tea : public CaffeineBeverage {
public:
// 实现抽象方法
void brew() const override {
cout << "Steeping the tea" << endl;
}
void addCondiments() const override {
cout << "Adding Lemon" << endl;
}
// 重载钩子方法
bool customerWantsCondiments() const override {
char answer;
cout << "Would you like lemon with your tea (y/n)? " << endl;
cin >> answer;
return answer == 'y' || answer == 'Y';
}
};
int main() {
auto coffee = make_unique<Coffee>();
coffee->prepareRecipe();
cout << endl;
auto tea = make_unique<Tea>();
tea->prepareRecipe();
cout << endl;
return 0;
}
分析总结
- 代码复用、符合开闭原则、不变部分稳定,易于拓展
- 增加类的数目
- 是多态的一种应用体现