一、效果图如下
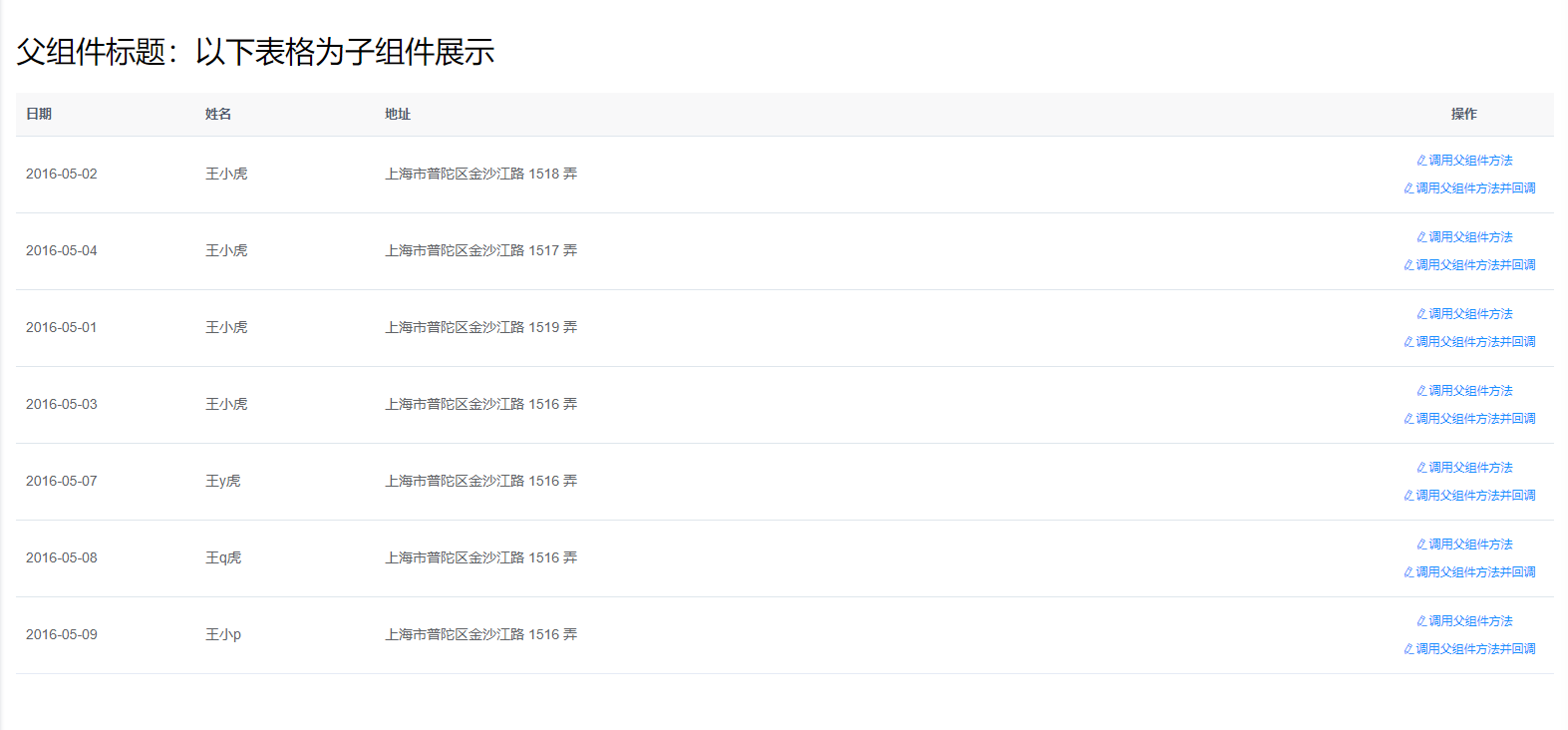
二、首先要实现拖拽行,需要安装sortableJs插件,执行命令:npm install sortablejs --save
三、共两个代码文件,一个父组件Parent.vue,一个子组件Child.vue,如下所示
a.Parent.vue,主要提供了子组件标签使用,传递到子组件的数据tableData,子组件可调用的方法。
<template>
<div class="app-container">
<h1>父组件标题:以下表格为子组件展示</h1>
<!--需要将tableData数据传递到子组件就需要在这里设置属性绑定
子组件需要调父组件方法,父组件需要在这里绑定,类似parentMethod,可以设置多个,属性名自定义-->
<el-child :tableData="tableData" @haveCallBack="haveCallBack" @parentMethod="parentMethod"></el-child>
</div>
</template>
<script>
import Child from "./Child";
export default {
name: "Parent",
components: {"el-child": Child},
data() {
return {
tableData: [
{
date: "2016-05-02",
name: "王小虎",
address: "上海市普陀区金沙江路 1518 弄",
},
{
date: "2016-05-04",
name: "王小虎",
address: "上海市普陀区金沙江路 1517 弄",
},
{
date: "2016-05-01",
name: "王小虎",
address: "上海市普陀区金沙江路 1519 弄",
},
{
date: "2016-05-03",
name: "王小虎",
address: "上海市普陀区金沙江路 1516 弄",
},
{
date: "2016-05-07",
name: "王y虎",
address: "上海市普陀区金沙江路 1516 弄",
},
{
date: "2016-05-08",
name: "王q虎",
address: "上海市普陀区金沙江路 1516 弄",
},
{
date: "2016-05-09",
name: "王小p",
address: "上海市普陀区金沙江路 1516 弄",
},
]
};
},
methods: {
parentMethod(row) {
console.log("父组件方法已调用,子组件传递日期打印:" + row.date);
},
haveCallBack(row, callback) {
console.log("父组件方法已调用,子组件传递日期打印:" + row.date);
callback(row.date);
}
}
}
</script>
b.Child.vue,主要显示表格数据,处理表格拖拽,以及调用父组件方法并获取方法返回值。
<template>
<div>
<!--这里row-key必须要加唯一的prop,不加拖拽位置会不对-->
<el-table :data="tableData" class="t1" row-key="date" style="width: 100%" :default-sort="{prop:'id',order:'descending'}">
<!--前端编号显示列,default-sort设置通过该列将数据倒序展示-->
<el-table-column align="center" label="编号" prop="id" type="index" width="80px" sortable/>
<el-table-column label="日期" prop="date" width="180"></el-table-column>
<el-table-column label="姓名" prop="name" width="180"></el-table-column>
<el-table-column label="地址" prop="address"></el-table-column>
<el-table-column align="center" class-name="small-padding" label="操作" width="180px">
<template slot-scope="scope">
<el-button v-hasPermi="['minigame:level:edit']" icon="el-icon-edit"
size="mini"
type="text"
@click="callParentMethod(scope.row)">调用父组件方法
</el-button>
<el-button v-hasPermi="['minigame:level:edit']" icon="el-icon-edit"
size="mini"
type="text"
@click="haveCallBack(scope.row)">调用父组件方法并回调
</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
import Sortable from "sortablejs"; // 引入拖拽js 这个是实现拖拽功能的核心,可以通過npm 安裝,文檔鏈接:http://www.sortablejs.com/options.html
export default {
name: "Child",
components: {},
//父组件传过来的数据属性名
props: ['tableData'],
data() {
return {};
},
mounted() {
//调用拖拽行方法
this.rowDrop()
},
methods: {
callParentMethod(row) {
//在子组件调用父组件的方法,需要传参就在后面带
this.$emit('parentMethod', row)
},
haveCallBack(row) {
this.$emit('haveCallBack', row, rsp => {
console.log("回调到子组件,打印日期:" + rsp)
})
},
//拖拽行方法
rowDrop() {
const tbody = document.querySelector('.t1 tbody')
Sortable.create(tbody, {
disabled: false, // 是否开启拖拽
ghostClass: 'sortable-ghost', //拖拽样式
animation: 150, // 拖拽延时,效果更好看
group: { // 是否开启跨表拖拽
pull: false,
put: false
},
onEnd: (e) => { // 这里主要进行数据的处理,拖拽实际并不会改变绑定数据的顺序,这里需要自己做数据的顺序更改
let arr = this.tableData; // 获取表数据
arr.splice(e.newIndex, 0, arr.splice(e.oldIndex, 1)[0]); // 数据处理
this.$nextTick(function () {
this.tableData = arr;
});
}
});
}
}
}
</script>
<style scoped>
.sortable-ghost {
opacity: 0.4;
background: #00a7d0;
}
</style>