Contents
Introduction
This is an intelligent parking simulation system based on QT, which involves the design and implementation of the parking lot interface. Each car can enter or leave the parking lot. In addition, the information such as license plate number, balance, and the duration of stay will be displayed. This is my undergraduate “Intelligent Transportation System” course project from September 2017 to November 2017.
Background
Usually, if there are some people who are responsible for managing and charging a fee in a parking system, this system is called semi-automatic parking management systems. If there are no such people, the system is called automatic parking management system.
The parking management system actually means a distributed control system, which consists of hardware and software. We have learned the relevant knowledge of this system, such as its functions, components, and main types of equipment. I hope to develop a simple Qt-based intelligent parking simulation system on the basis of theoretical knowledge learned from courses.
Environment
Qt is a cross-platform application development framework. Some of the well-known applications developed with Qt are KDE, Opera, Google Earth, Skype, VLC, Maya, or Mathematica. Qt was first publicly released in May 1995. It is dual-licensed. It can be used for creating open source applications as well as commercial ones. Qt toolkit is a very powerful toolkit. It is well established in the open-source community. Thousands of open source developers use Qt all over the world.
http://zetcode.com/gui/qt5/introduction/
Demo
UI Introduction:
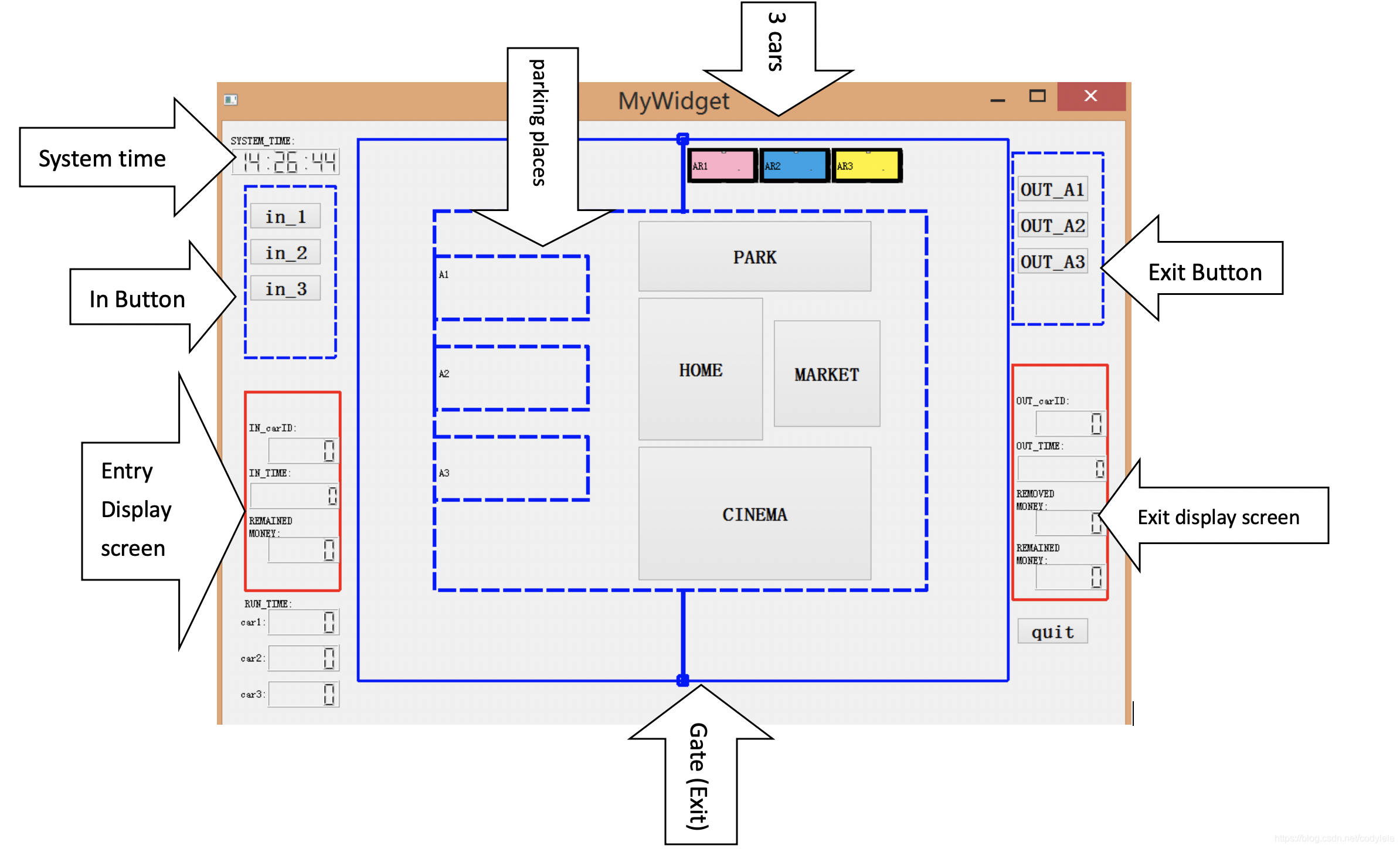
Car1 moves in:
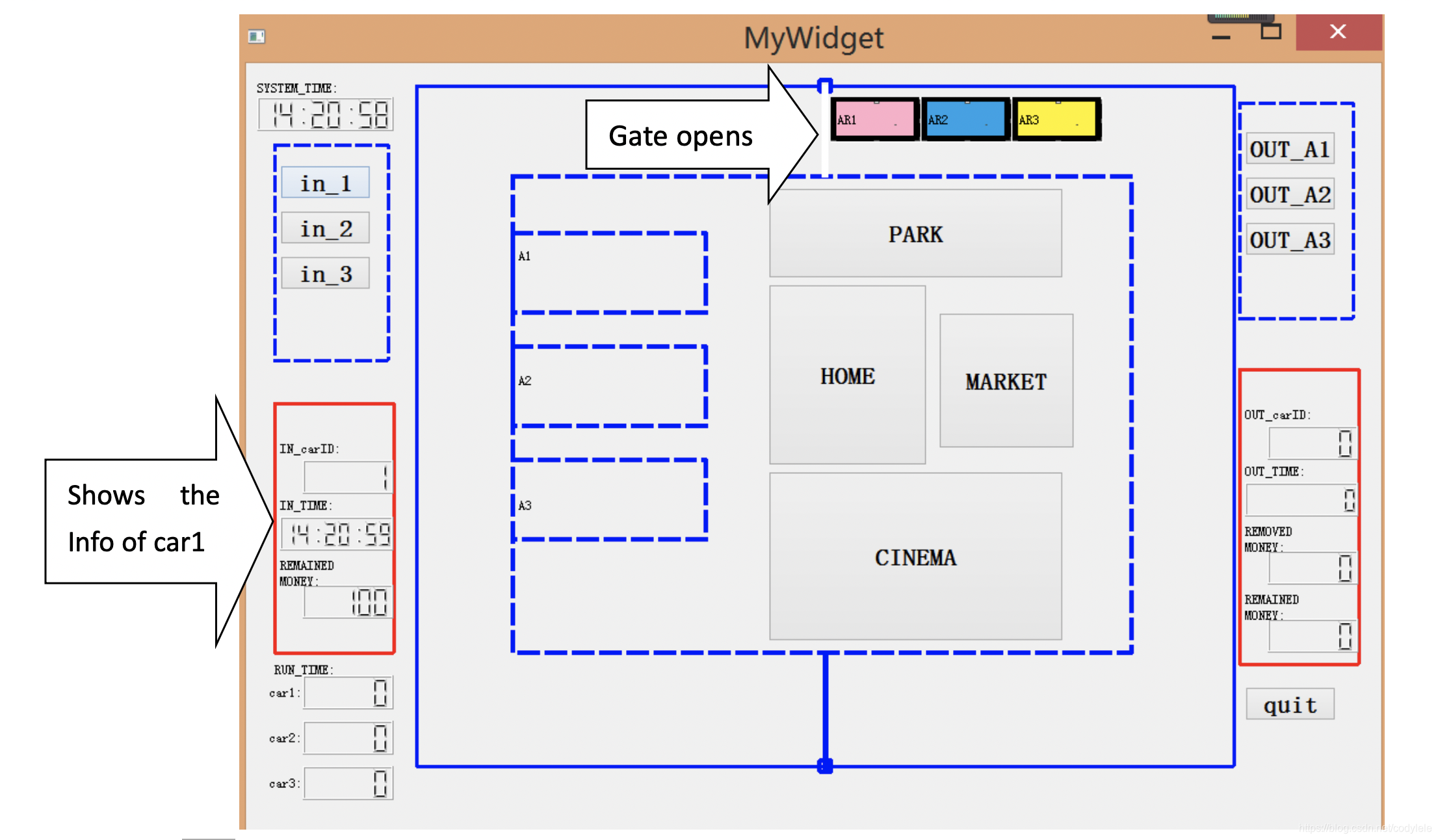
Car2 moves in:
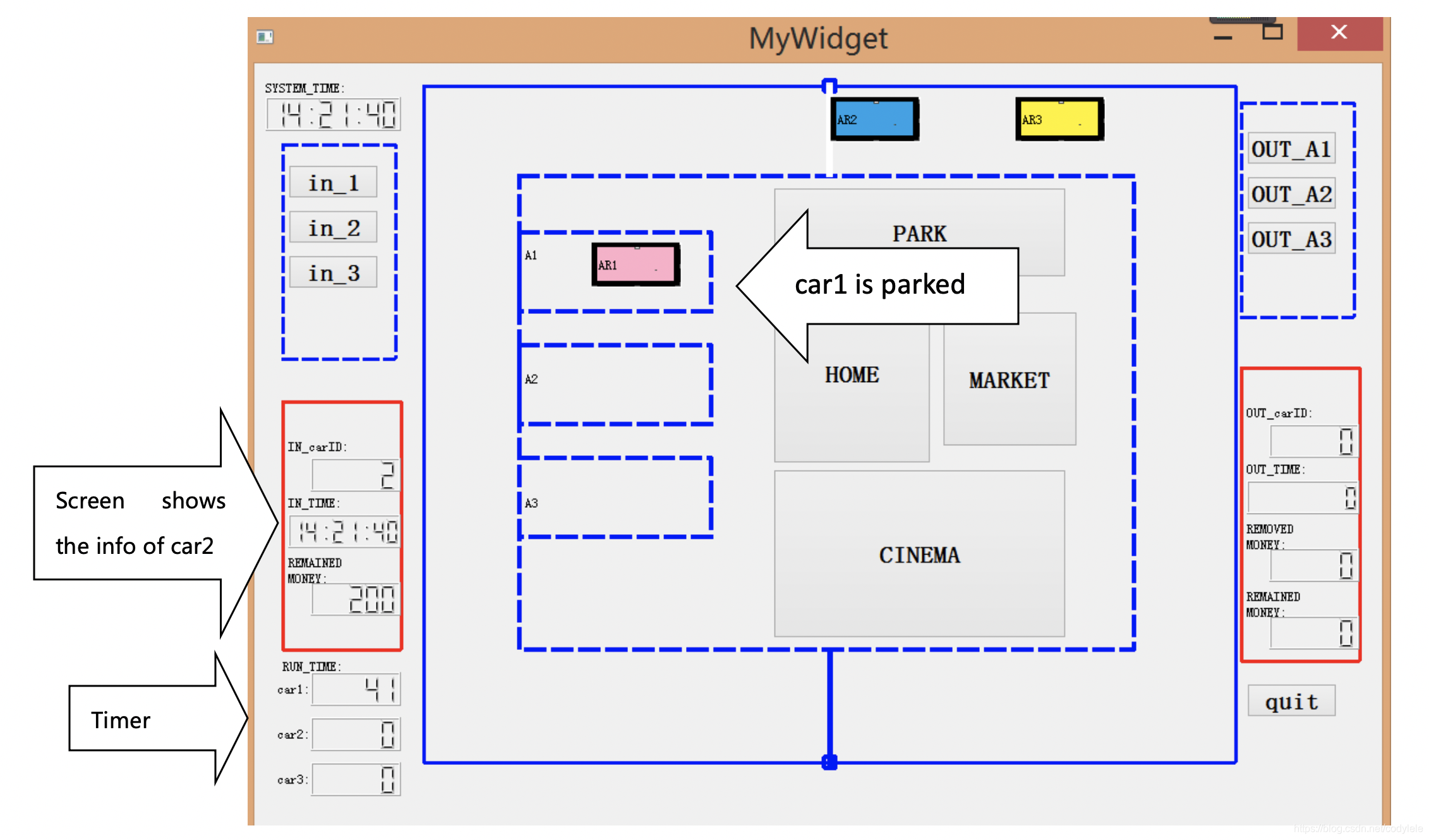
All cars move in:
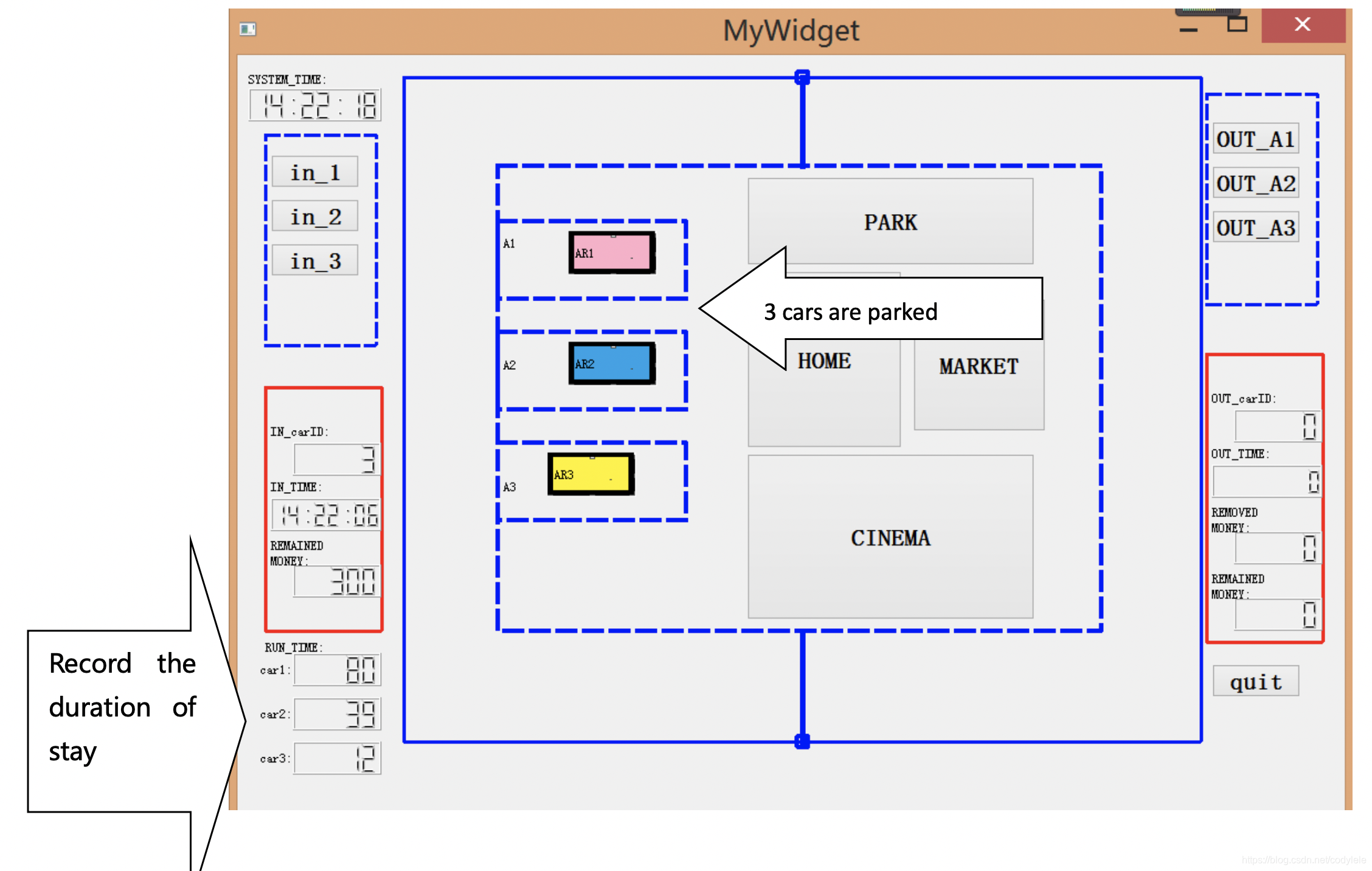
When car1 leaves the parking lot, the gate opens ( gate opens means steering gear is lifted, gate closes means steering gear is lowered )
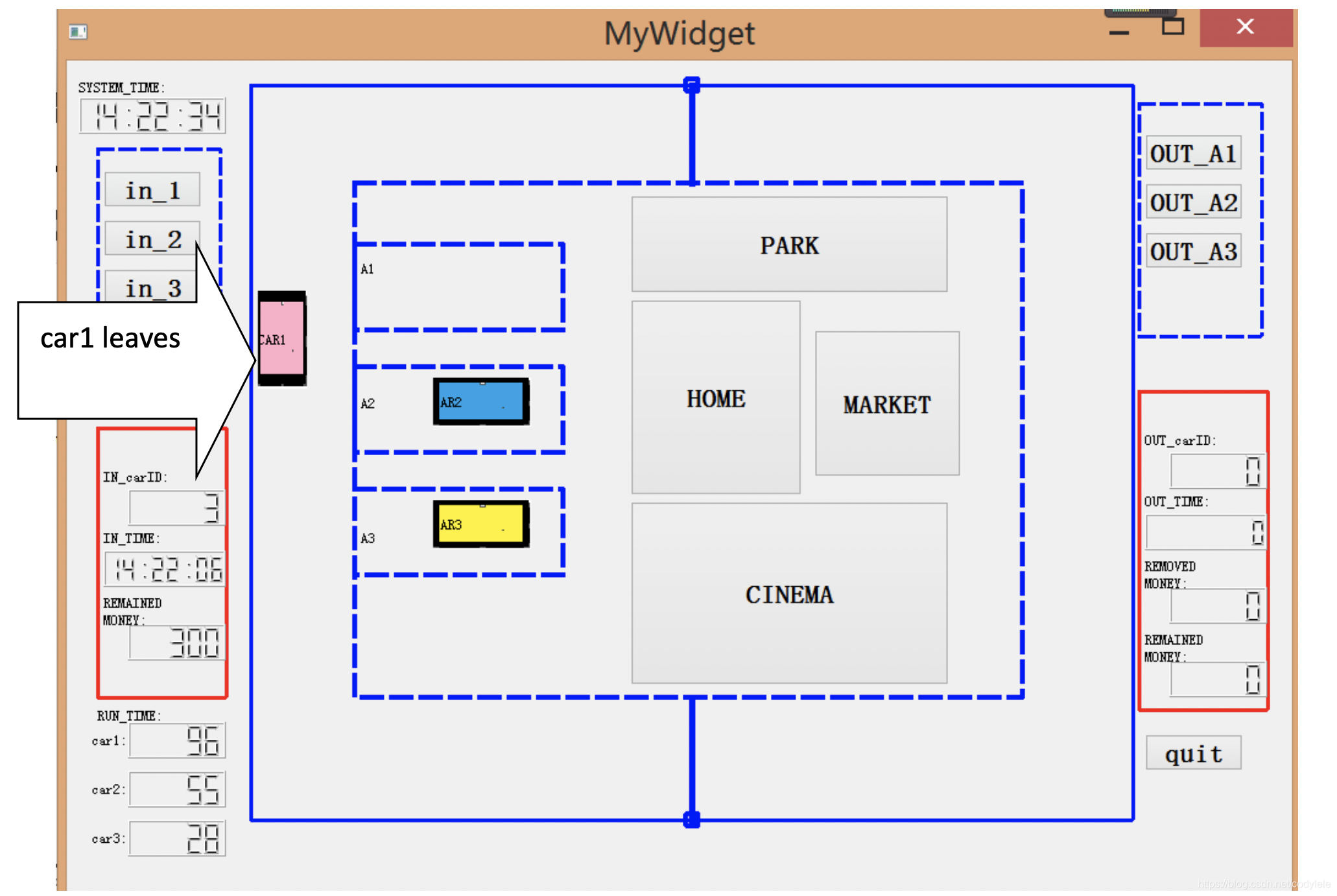
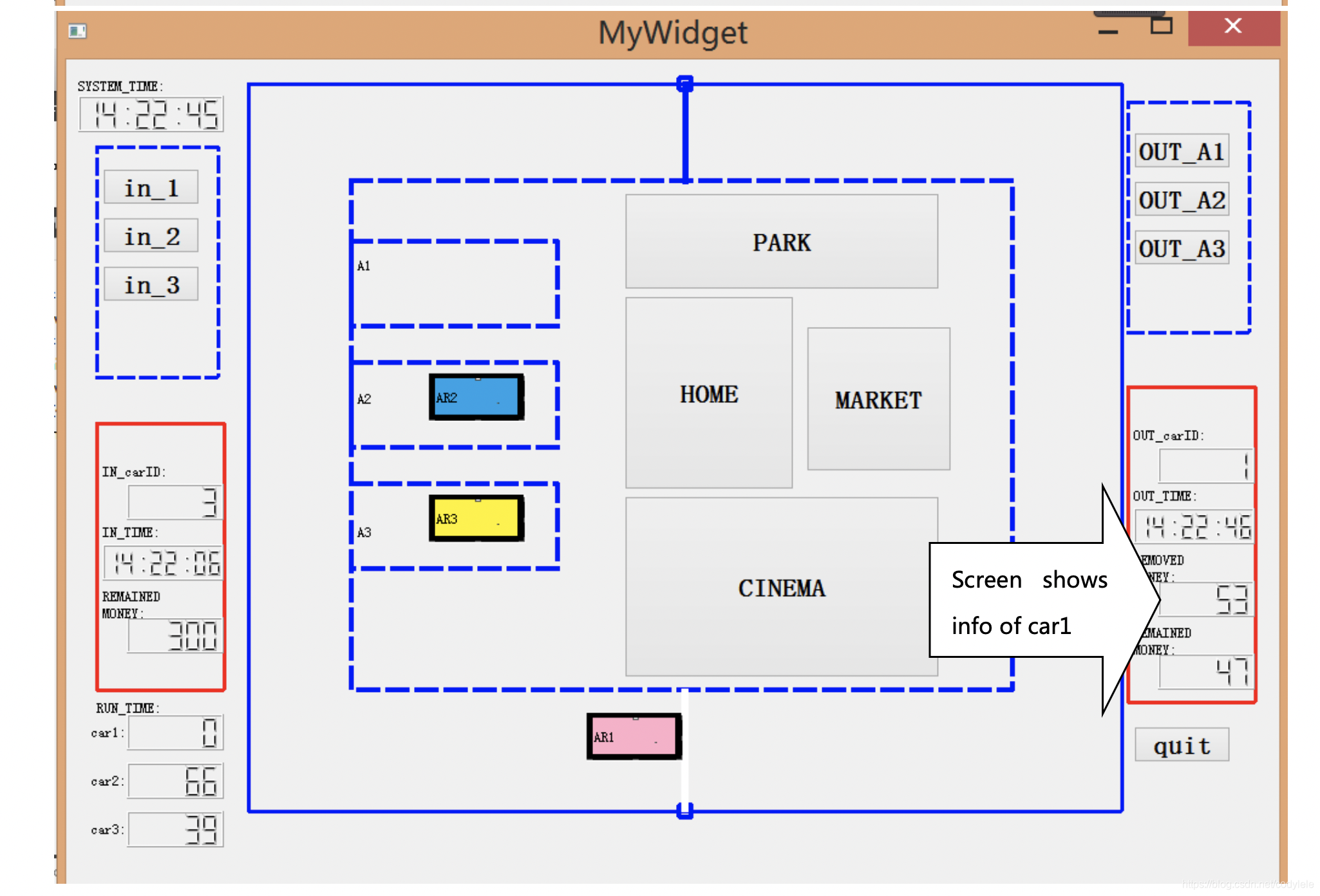
Car1 returns to the original place. Press In button, it can move in again:
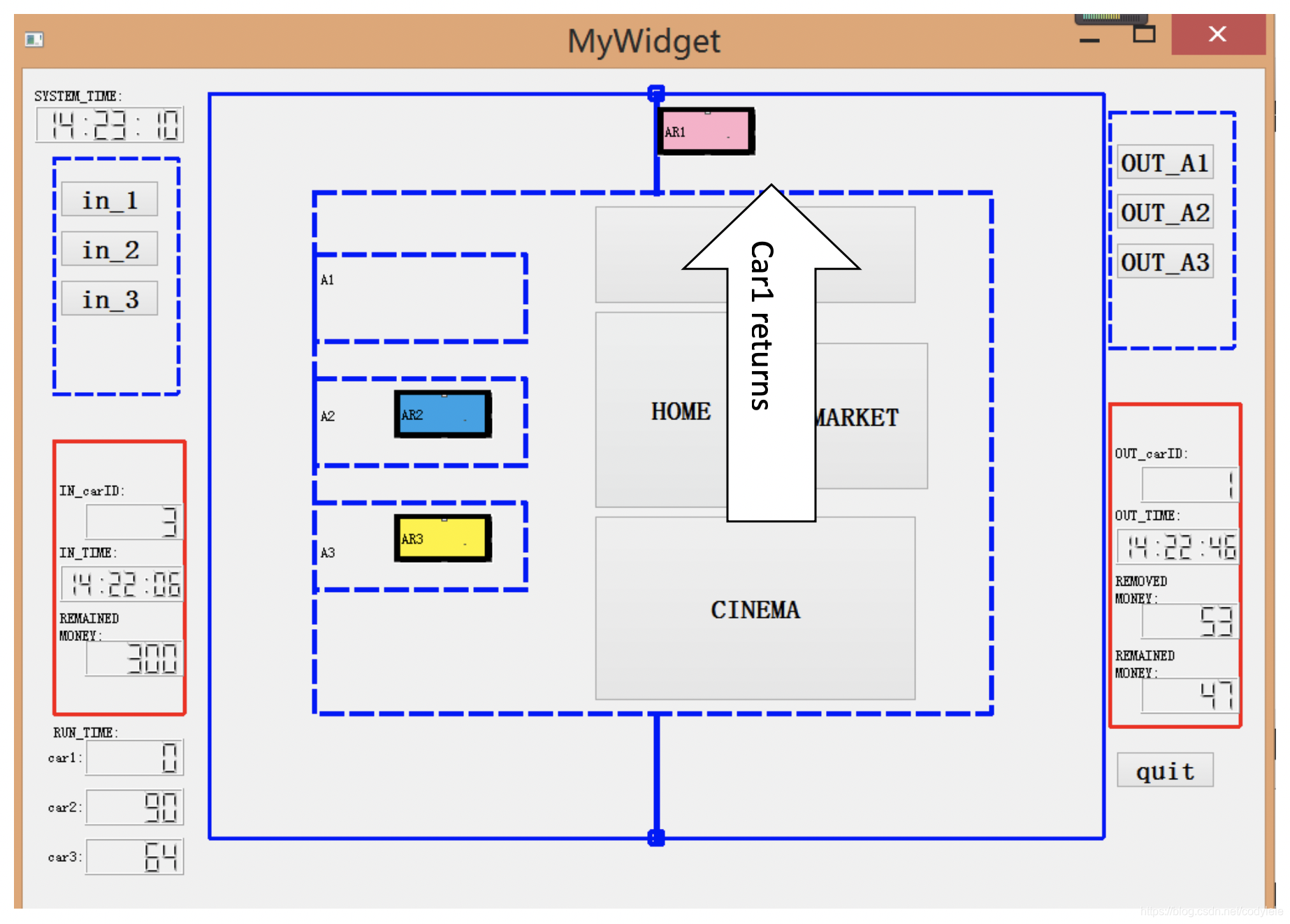
Design Summary
Requirements Analysis
UI Design
-
The upper left of the interface shows the system time.
-
The bottom left of the interface shows the timing of each car entering the parking lot.
-
The left-hand side of the interface displays the screen showing the information (such as time of entering, balance, the duration of stay) in detail when the car enters, and the right-hand side shows the information when the car enters.
-
In the two display screens introduced by 3, there are six buttons to control the car to enter or exit.
-
This system is designed based on the laboratory intelligent transportation simulation system.
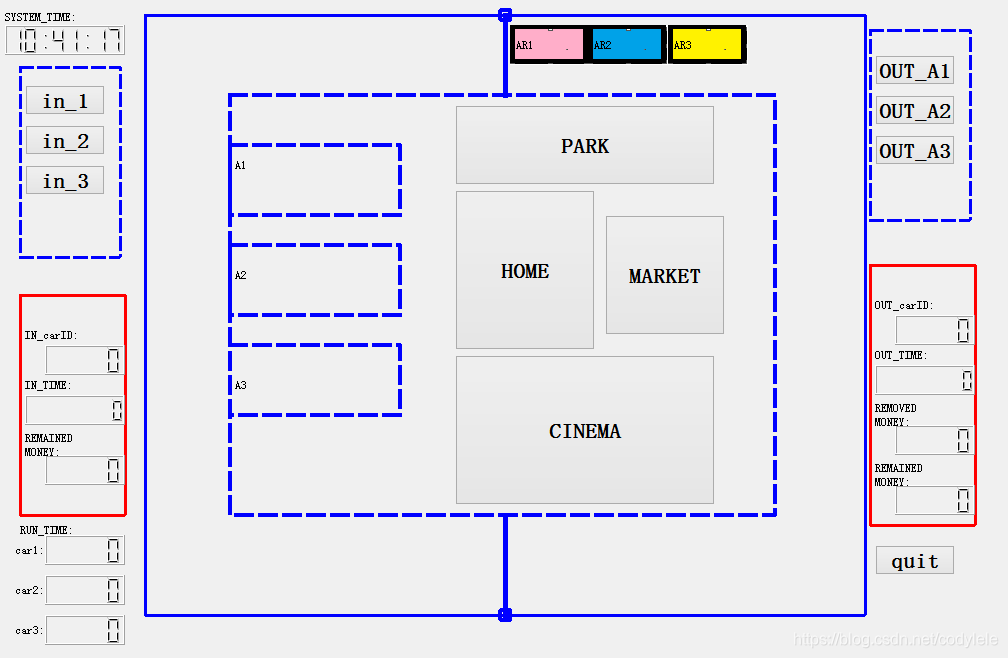
Car Control
-
Click the button IN to let the car to enter the parking lot. When the head of the car reaches the gate, the steering gear will be lifted, and the entry display screen shows the relevant information of the car (such as the balance, the entering time, etc.), records the duration of stay and shows it on the LCD display screen.
-
Click the Out button and the car starts to leave the parking place. When the head of the car reaches the exit gate, the timer stops timing, and the relevant information of the car is displayed (such as the amount of money deducted for the parking, the time of leaving the parking lot, etc.);
-
When the whole body of the car passes the steering gear, the gear will be lowered, and the car continues to move forward to the original place. To let the car re-entry, repeat the steps.
-
It realizes that all cars can enter the system one by one
Basic Idea
-
Plan routes for three cars (i.e. define 3 different functions to be called) and assign three cars three different timers to achieve their own timing.
-
A flag can be specially defined for the gate to close or open, that is, each car will set the flag as false or true according to its own requirements, so as to achieve the purpose of opening or closing the gate.
-
When the gate opens, screen function will be executed at the same time to display the relevant information.
Flowchart
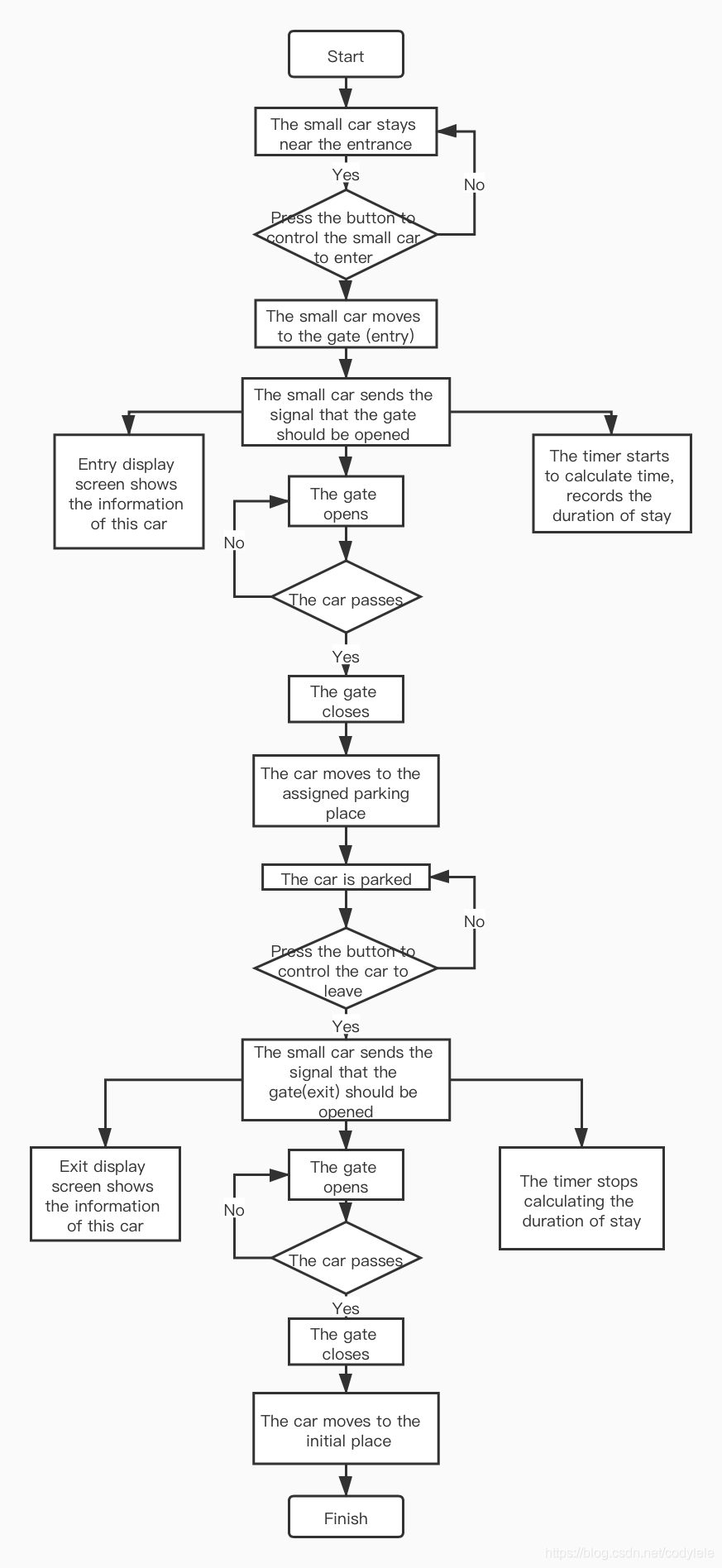
Code
1. Class (mywdget.h):
namespace Ui {
class MyWidget;
class Car;}
/*******************/
#ifndef MYCAR_H
#define MYCAR_H
class Car
{private:
int carID; //car id
float symoney; //get the remaining money
public:
Car(int _carID,float _symoney):carID(_carID) ,symoney(_symoney){}
float Get_Symoney()//get the remaining money
{ return symoney; }
int Get_CarId()//get car id
{return carID;}
void removemoney(float cou)
{ symoney= symoney-0.5*(cou); //the remaining money - 0.5 yuan/s * the duration of stay of this car}
~Car(){}};
#endif // MYCAR_H
/*********************/
class MyWidget : public QWidget
{ Q_OBJECT
public:
explicit MyWidget(QWidget *parent = 0);~MyWidget();
QPainter*paint =new QPainter(this);
QTimer*timer=new QTimer(this);//system timer
QTimer*timer1=new QTimer(this);//timer used by car1
QPixmap pix;//car1
int count=0;//records the staying time of car1
QTimer*timer2=new QTimer(this);//timer used by car2
QPixmap pix2;
int count2=0;
QTimer*timer3=new QTimer(this);//timer used by car3
QPixmap pix3;
int count3=0;
void back(); //car1 leaves parking lot
void back2();
void back3();
void displayup();//car1 entry display screen
void displaydown();//exit display screen
void displayup2();//car2 entry display screen
void displaydown2();//exit display screen
void displayup3();//car3 entry display screen
void displaydown3();//exit display screen
public slots ://all functions are slots
void carmovetoa1();//car moves to a1
void outa1();//car leaves a1
void displaytime();//records the duration of stay of each car
void carmovetoa2();
void outa2();
void displaytime2();
void carmovetoa3();
void outa3();
void displaytime3();
void XYtimerUpDate();//system clock
private:
Ui::MyWidget *ui;
QPushButton*b1,*in1,*in2,*in3,*a2,*a3,*park,*home,*cinema,*market,*a1;//各种按钮
QLabel*label,*carid1,*carid2,*intime,*outtime,*remainedmoney,*removedmoney,*la1,*la2,*la3,*car1,*car2,*car3,*sysclock,*runtime1,*runtime2,*runtime3,*remainedmoney2;
//some labels
QLCDNumber*lcarid1,*lcarid2,*lintime,*louttime,*clock,*lruntime1,*lruntime2,*lruntime3,*lremainedmoney,*lremovedmoney,*lremainedmoney2;
//some display screen
bool flag_in;// provide gate with this flag, when car moves in , the steering gear should be lifted
bool flag_out;//when car leaves , the steering gear should be lifted
protected:
void paintEvent(QPaintEvent*);
void delay(int time);//delay the speed of the car};
#endif // MYWIDGET_H
**2. Constructor(mywdget.cpp): **
Main Idea: Set the size of the interface, define and use buttons, labels and LCD display, design the interface (draw rectangles in the paint event function), and define the relationship between the signal and the slot (standard function). For example, when you click the mouse, that is, an event occurs, the click signal will be sent (emit is implemented), and the function in the slot will be executed.
connect(timer,SIGNAL(timeout()),this,SLOT(XYtimerUpDate()));
//The signal and corresponding slot function of the associated timer are used to display the system time
timer->start(1000);
//Timer starts to timing and 1000 ms = 1s
connect(b1,SIGNAL(clicked()),qApp,SLOT(quit()));//exit button
//Qapp is an inner pointer,point to an instance of QApllication
//take car1 as an example:
connect(in1,SIGNAL(clicked()),this,SLOT(carmovetoa1()));
//press the In button,car moves to a1
connect(a1,SIGNAL(clicked()),this,SLOT(outa1()));
//press the Out button,car leaves from a1
connect(timer1,SIGNAL(timeout()),this,SLOT(displaytime()));
//When the car enters the gate, it sends the signal that Timer1 starts timing (Timer - > start();), and when the car leaves the gate, it sends a signal that Timer1 stops timing (Timer - > stop();), and the displaytime() function is used to display the time difference between the two
timer1->setInterval(1000);//the interval of timer is 1s,count+1
//the definition of slots:
void carmovetoa1();//car moves to a1 , call displayup();function(entry diplay screen show the info of car)
void outa1();//car leaves a1 parking place,call void displaydown();function(exit display screen shows info of car)and void back(); function
void displaytime();//record the duration of stay
void XYtimerUpDate();//system clock, the timer will count every 1s automatically
3. Functions
void MyWidget::displayup()//entry display screen
void MyWidget::displaydown()//exit display screen
void MyWidget::back() //a1 leaves the parking lot,makes a detour around the parking lot and returns to the original designated location
4. Important Code Analysis
void MyWidget::carmovetoa1()//car moves to parking place
{
int i=0;
flag_in=true;//the steering gear has been lifted
this->repaint();//repaint function,loads the interface of gate opening and refresh the screen
displayup();//entry diplay screen shows the info of car
timer1->start();//starts timing to record the duration of stay
delay(1000);//the delay time that car waits for the steering gear to be lifted
for( i=car1->x();i>430;i--)//car body has passed the steering gear
{ car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(3);//to prevent the speed of the car from being too fast
}
delay(300);
flag_in=false;//close the gate
this->repaint();//repaint,loads the interface of gate closing and refresh the screen
for(i=car1->x();i>155;i--)//car continues to move
{ car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(3);
}
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->y();i<160;i++)//moves down
{car1->setGeometry(car1->x(),i,car1->width(),car1->height());
delay(3);
}
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->x();i<300;i++)//moves left
{ car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(6);
}
}
Car c1(1,100);
void MyWidget::displaytime()
{ count++;//the timer adds one at a time
lruntime1->display(count);//running time LCD shows the duration of stay of each car
}
void MyWidget::outa1()
{ //out a1
int i=0;
for(i=car1->x();i>155;i--)//the car leaves the parking place and moves to gate(exit)
{ car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(6);}
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->y();i<540;i++)
{car1->setGeometry(car1->x(),i,car1->width(),car1->height());
delay(3); }
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->x();i<430;i++)//car head reaches the gate
{car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(3);}
timer1->stop();//timer stops timing
delay(300);
flag_out=true;//the steering gear is lifted
this->repaint();
displaydown();//shows the info of the car
back();//after car leaves the gate (exit), it will move back to the original place
}
//the definition of functions
void MyWidget::back() //a1 leaves the parking lot
{ int i=0;
delay(1000);
for(i=car1->x();i<510;i++)
{ car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(3); }
delay(300);
flag_out=false;
this->repaint();
for(i=car1->x();i<800;i++)
{car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(1);}
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->y();i>30;i--)
{ car1->setGeometry(car1->x(),i,car1->width(),car1->height());
delay(1);}
car1->setGeometry(car1->x(),car1->y(),car1->height(),car1->width());
for(i=car1->x();i>510;i--)
{car1->setGeometry(i,car1->y(),car1->width(),car1->height());
delay(1);}
}
void MyWidget::displayup()//entry display screen
{ //Car c1(1,100);
QTime time1 = QTime::currentTime();//obtain the current system time
QString strTime1 = time1.toString("hh:mm:ss");// set the time format of system time
lintime->display(strTime1);//show the entering time in LCD
lremainedmoney->display(c1.Get_Symoney());//the remaining money of car1
lcarid1->display(c1.Get_CarId());//car id}
void MyWidget::displaydown()//exit display screen
{
QTime time1 = QTime::currentTime();//obtain the current system time
QString strTime1 = time1.toString("hh:mm:ss");//set the time format of system time
louttime->display(strTime1);//show the entering time in LCD
lremovedmoney->display(count*0.5);//the deducted money of car1
c1.removemoney(count);//deduct the money according to the duration of stay
lremainedmoney2->display(c1.Get_Symoney());//the remaining money of car1
lcarid2->display(c1.Get_CarId());//id号
count=0;//car passes the gate, count is set to 0
lruntime1->display(count);
}
Future Improvements
1.Since this system is designed based on the laboratory intelligent transportation simulation system, it could be combined with my undergraduate graduation project. It is possible to realize that when I press the button in this system, the real smart car in the laboratory ITS can also be controlled.
2. If event B is triggered after event A occurs, event A will be paused and A will wait for the end of event B to continue.
3. The car can only be parked in its assigned parking place, for example, car 1 can only be parked in a1 parking place.
Conclusion
In this project, a simple Qt-based intelligent parking simulation system has been established. It is a bit challenging project for me since I have never learned QT before. I have paid much attention to the following four aspects:
- Signal slot:
The signal slot plays an important role in this project, which can be achieved only after class Qobject has been imported, and many slots and signals defined in the library can be directly used by us, but in this project, I also have to define many slots by myself. - Interface design:
It was a bit difficult to design the interface from scratch, I added buttons, labels, and display screens step by step. After I design the interface, I didn’t know how to paint the interface outside the drawing function, to solve this problem, I searched many materials and learned that repaint function can be used to achieve the function of controlling the real-time interface in the slot. - Timer:
When I started to work on the timer, it showed no information on the display screen however I defined it, thus I felt a little frustrated. Later I asked my classmates around who knew how to use QT, and finally understood the meaning of timer under the explanation of them, and realized the system clock; - Information display of three cars:
Finally, the class car was defined, the balance, card number, and deduction fee of the three cars needed to be assigned to their initial values respectively.
In short, in this project, I understand that no pain, no gains, and correct guidance are also needed. And thanks for those who have help me a lot! Thanks for reading!
Reference:
QT Creator Quick Start Tutorial
24-Hour QT Programming