js模块和es6模块
ES模块简介 (Introduction to ES Modules)
ES Modules is the ECMAScript standard for working with modules.
ES模块是用于模块的ECMAScript标准。
While Node.js has been using the CommonJS standard for years, the browser never had a module system, as every major decision such as a module system must be first standardized by ECMAScript and then implemented by the browser.
尽管Node.js多年来一直使用CommonJS标准,但浏览器从未拥有模块系统,因为每个主要决策(例如模块系统)都必须首先由ECMAScript标准化,然后由浏览器实现。
This standardization process completed with ES6 and browsers started implementing this standard trying to keep everything well aligned, working all in the same way, and now ES Modules are supported in Chrome, Safari, Edge and Firefox (since version 60).
这个由ES6完成的标准化过程和浏览器开始实施该标准,以使所有内容保持一致,并以相同的方式工作,现在Chrome,Safari,Edge和Firefox(版本60开始)都支持ES模块。
Modules are very cool, because they let you encapsulate all sorts of functionality, and expose this functionality to other JavaScript files, as libraries.
模块非常酷,因为它们使您可以封装各种功能,并将该功能作为库公开给其他JavaScript文件。
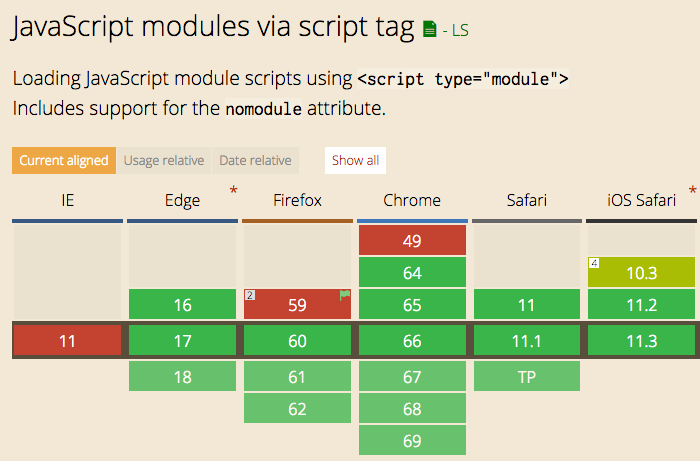
ES模块语法 (The ES Modules Syntax)
The syntax to import a module is:
导入模块的语法为:
import package from 'module-name'
while CommonJS uses
而CommonJS使用
const package = require('module-name')
A module is a JavaScript file that exports one or more values (objects, functions or variables), using the export
keyword. For example, this module exports a function that returns a string uppercase:
模块是一个JavaScript文件,该文件使用export
关键字导出一个或多个值(对象,函数或变量)。 例如,此模块导出一个返回大写字符串的函数:
uppercase.js
uppercase.js
export default str => str.toUpperCase()
In this example, the module defines a single, default export, so it can be an anonymous function. Otherwise it would need a name to distinguish it from other exports.
在此示例中,模块定义了一个默认的export ,因此它可以是匿名函数。 否则,将需要一个名称来区别于其他出口。
Now, any other JavaScript module can import the functionality offered by uppercase.js by importing it.
现在, 任何其他JavaScript模块都可以通过导入来导入uppercase.js提供的功能。
An HTML page can add a module by using a <script>
tag with the special type="module"
attribute:
HTML页面可以通过使用具有特殊type="module"
属性的<script>
标记来添加模块:
<script type="module" src="index.js"></script>
Note: this module import behaves like a
defer
script load. See efficiently load JavaScript with defer and async注意:此模块导入的行为类似于
defer
脚本加载。 通过延迟和异步查看有效加载JavaScript
It’s important to note that any script loaded with type="module"
is loaded in strict mode.
重要的是要注意,任何用type="module"
加载的脚本都是在严格模式下加载的。
In this example, the uppercase.js
module defines a default export, so when we import it, we can assign it a name we prefer:
在此示例中, uppercase.js
模块定义了默认的export ,因此在导入时,可以为其指定一个我们更喜欢的名称:
import toUpperCase from './uppercase.js'
and we can use it:
我们可以使用它:
toUpperCase('test') //'TEST'
You can also use an absolute path for the module import, to reference modules defined on another domain:
您还可以使用绝对路径进行模块导入,以引用在另一个域上定义的模块:
import toUpperCase from 'https://flavio-es-modules-example.glitch.me/uppercase.js'
This is also valid import syntax:
这也是有效的导入语法:
import { toUpperCase } from '/uppercase.js'
import { toUpperCase } from '../uppercase.js'
This is not:
这不是:
import { toUpperCase } from 'uppercase.js'
import { toUpperCase } from 'utils/uppercase.js'
It’s either absolute, or has a ./
or /
before the name.
它可以是绝对的,也可以在名称前带有./
或/
。
其他导入/导出选项 (Other import/export options)
We saw this example above:
我们在上面看到了这个例子:
export default str => str.toUpperCase()
This creates one default export. In a file however you can export more than one thing, by using this syntax:
这将创建一个默认导出。 但是,在文件中,可以使用以下语法导出多个内容:
const a = 1
const b = 2
const c = 3
export { a, b, c }
Another module can import all those exports using
另一个模块可以使用
import * from 'module'
You can import just a few of those exports, using the destructuring assignment:
您可以使用解构分配来导入其中一些导出:
import { a } from 'module'
import { a, b } from 'module'
You can rename any import, for convenience, using as
:
您可以重命名任何进口,为方便起见,使用as
:
import { a, b as two } from 'module'
You can import the default export, and any non-default export by name, like in this common React import:
您可以导入默认导出,也可以按名称导入任何非默认导出,例如在此常见的React导入中:
import React, { Component } from 'react'
You can see an ES Modules example here: https://glitch.com/edit/#!/flavio-es-modules-example?path=index.html
您可以在此处看到ES模块示例: https : //glitch.com/edit/#!/ flavio-es-modules-example ?path=index.html
CORS (CORS)
Modules are fetched using CORS. This means that if you reference scripts from other domains, they must have a valid CORS header that allows cross-site loading (like Access-Control-Allow-Origin: *
)
使用CORS提取模块。 这意味着,如果您引用其他域中的脚本,则它们必须具有允许跨站点加载的有效CORS标头(例如Access-Control-Allow-Origin: *
)。
不支持模块的浏览器呢? (What about browsers that do not support modules?)
Use a combination of type="module"
and nomodule
:
使用type="module"
和nomodule
:
<script type="module" src="module.js"></script>
<script nomodule src="fallback.js"></script>
结论 (Conclusion)
ES Modules are one of the biggest features introduced in modern browsers. They are part of ES6 but the road to implement them has been long.
ES模块是现代浏览器中引入的最大功能之一。 它们是ES6的一部分,但是实现它们的路很长。
We can now use them! But we must also remember that having more than a few modules is going to have a performance hit on our pages, as it’s one more step that the browser must perform at runtime.
我们现在可以使用它们了! 但是我们还必须记住,拥有多个模块将对我们的页面产生性能影响,因为这是浏览器在运行时必须执行的又一步。
Webpack is probably going to still be a huge player even if ES Modules land in the browser, but having such a feature directly built in the language is huge for a unification of how modules work client-side and on Node.js as well.
即使ES模块位于浏览器中, Webpack仍将扮演重要角色,但是直接用该语言构建这样的功能对于统一模块在客户端以及在Node.js上的工作方式来说是巨大的。
js模块和es6模块