react 安装
How do you install React?
你如何安装React?
React is a library, so saying install might sound a bit weird. Maybe setup is a better word, but you get the concept.
React是一个库,所以说安装听起来有点奇怪。 也许设置是一个更好的词,但是您可以理解。
There are various ways to setup React so that it can be used on your app or site.
有多种方法可以设置React,以便可以在您的应用程序或网站上使用它。
直接在网页中加载React (Load React directly in the web page)
The simplest one is to add the React JavaScript file into the page directly. This is best when your React app will interact with the elements present on a single page, and not actually controls the whole navigation aspect.
最简单的方法是将React JavaScript文件直接添加到页面中。 当您的React应用程序将与单个页面上存在的元素进行交互,而不实际控制整个导航方面时,这是最好的方法。
In this case, you add 2 script tags to the end of the body
tag:
在这种情况下,您可以在body
标签的末尾添加2个脚本标签:
<html>
...
<body>
...
<script
src="https://cdnjs.cloudflare.com/ajax/libs/react/16.7.0-alpha.2/umd/react.development.js"
crossorigin
></script>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.7.0-alpha.2/umd/react-dom.production.min.js"
crossorigin
></script>
</body>
</html>
The
16.7.0-alpha.2
version in the links points to the latest Alpha of 16.7 (at the time of writing), which has Hooks available. Please change it to the latest version of React that is available.链接中的
16.7.0-alpha.2
版本指向最新的Alpha 16.7(在撰写本文时),该版本具有可用的Hooks。 请将其更改为可用的最新版本的React。
Here we loaded both React and React DOM. Why 2 libraries? Because React is 100% independent from the browser and can be used outside it (for example on Mobile devices with React Native). Hence the need for React DOM, to add the wrappers for the browser.
在这里,我们加载了React和React DOM。 为什么要2个库? 因为React是100%独立于浏览器的,并且可以在浏览器之外使用(例如,在具有React Native的移动设备上)。 因此,需要React DOM为浏览器添加包装器。
After those tags you can load your JavaScript files that use React, or even inline JavaScript in a script
tag:
在这些标签之后,您可以加载使用ReactJavaScript文件,甚至在script
标签中内嵌JavaScript:
<script src="app.js"></script>
<!-- or -->
<script>
//my app
</script>
To use JSX you need an extra step: load Babel
<script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script>
and load your scripts with the special text/babel
MIME type:
并使用特殊的text/babel
MIME类型加载脚本:
<script src="app.js" type="text/babel"></script>
Now you can add JSX in your app.js file:
现在,您可以在您的app.js文件中添加JSX:
const Button = () => {
return <button>Click me!</button>
}
ReactDOM.render(<Button />, document.getElementById('root'))
Check out this simple Glitch example: https://glitch.com/edit/#!/react-example-inline-jsx?path=script.js
看看这个简单的Glitch示例: https ://glitch.com/edit/#!/react-example-inline-jsx ? path = script.js
Starting in this way with script tags is good for building prototypes and enables a quick start without having to set up a complex workflow.
从这种方式开始,使用脚本标签非常适合构建原型,并且无需设置复杂的工作流程即可快速入门。
使用create-react-app (Use create-react-app)
create-react-app
is a project aimed at getting you up to speed with React in no time, and any React app that needs to outgrow a single page will find that create-react-app
meets that need.
create-react-app
是一个旨在立即使您熟悉React的项目,任何需要超出单个页面的React应用都会发现create-react-app
可以满足需求。
You start by using npx
, which is an easy way to download and execute Node.js commands without installing them. npx
comes with npm
(since version 5.2) and if you don’t have npm installed already, do it now from https://nodejs.org (npm is installed with Node).
首先使用npx
,这是一种无需安装即可下载和执行Node.js命令的简便方法。 npx
随附npm
(自5.2版开始),如果尚未安装npm,请立即从https://nodejs.org进行安装(npm与Node一起安装)。
If you are unsure which version of npm you have, run npm -v
to check if you need to update.
如果不确定您具有哪个版本的npm,请运行npm -v
来检查是否需要更新。
Tip: check out my OSX terminal tutorial at https://flaviocopes.com/macos-terminal/ if you’re unfamiliar with using the terminal, applies to Linux as well - I’m sorry but I don’t have a tutorial for Windows at the moment, but Google is your friend.
提示:如果您不熟悉该终端,请访问https://flaviocopes.com/macos-terminal/查看我的OSX终端指南,该指南也适用于Linux-很抱歉,但我没有针对该终端的指南目前是Windows,但Google是您的朋友。
When you run npx create-react-app <app-name>
, npx
is going to download the most recent create-react-app
release, run it, and then remove it from your system. This is great because you will never have an outdated version on your system, and every time you run it, you’re getting the latest and greatest code available.
当运行npx create-react-app <app-name>
, npx
将下载最新的create-react-app
版本,运行它,然后将其从系统中删除。 这很棒,因为您的系统上永远不会有过时的版本,并且每次运行它时,您都可以获得最新,最好的代码。
Let’s start then:
让我们开始:
npx create-react-app todolist
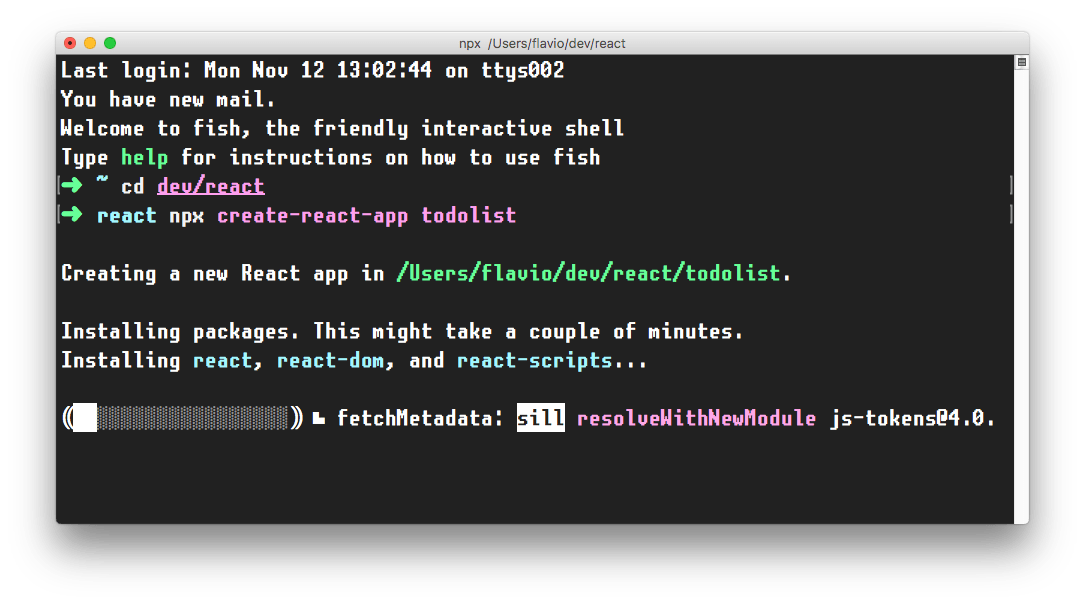
This is when it finished running:
这是它完成运行的时间:
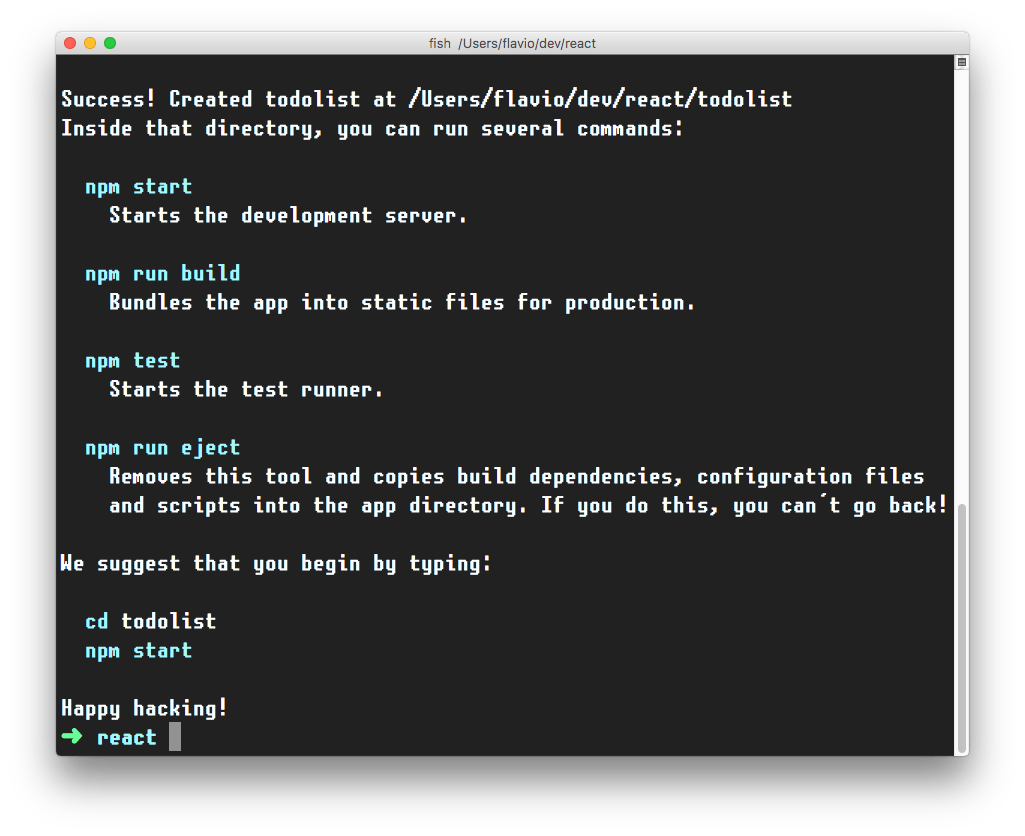
create-react-app
created a files structure in the folder you told (todolist
in this case), and initialized a Git repository.
create-react-app
在您指定的文件夹(在本例中为todolist
中创建了一个文件结构,并初始化了一个Git存储库。
It also added a few commands in the package.json
file, so you can immediately start the app by going into the folder and run npm start
.
它还在package.json
文件中添加了一些命令,因此您可以通过进入文件夹并运行npm start
立即启动应用npm start
。
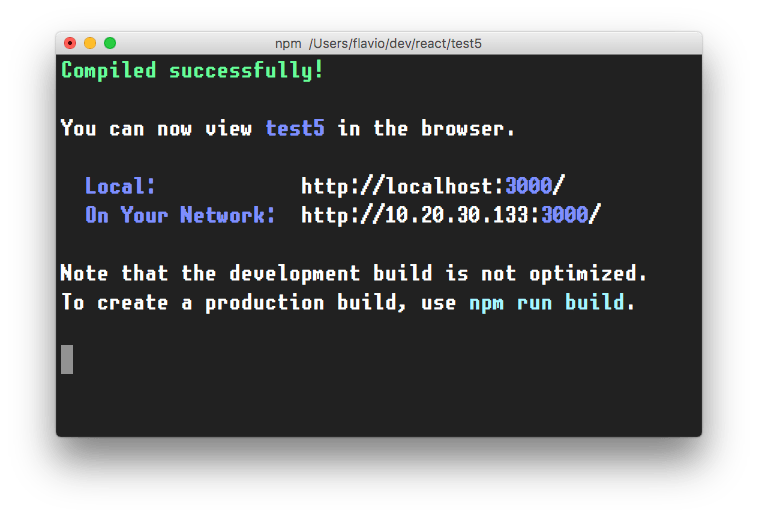
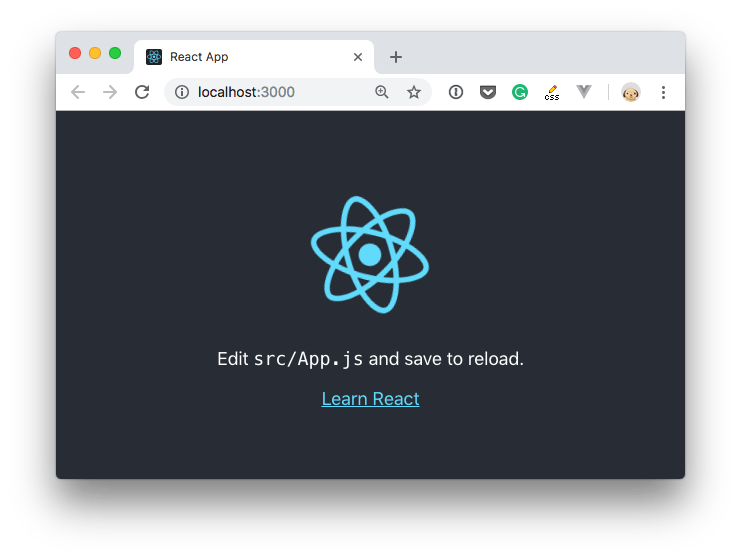
In addition to npm start
, create-react-app
added a few other commands:
除了npm start
, create-react-app
还添加了一些其他命令:
npm run build
: to build the React application files in thebuild
folder, ready to be deployed to a servernpm run build
:在build
文件夹中构建React应用程序文件,准备将其部署到服务器上npm test
: to run the testing suite using Jestnpm test
:使用Jest运行测试套件npm eject
: to eject fromcreate-react-app
npm eject
:从create-react-app
弹出
Ejecting is the act of deciding that create-react-app
has done enough for you, but you want to do more than what it allows.
退出是决定create-react-app
对您已经足够完成的动作,但是您想要做的事情超出其允许的范围。
Since create-react-app
is a set of common denominator conventions and a limited amount of options, it’s probable that at some point your needs will demand something unique that outgrows the capabilities of create-react-app
.
由于create-react-app
是一组通用的分母约定,并且选项数量有限,因此在某些时候,您的需求可能会需要一些独特的东西,而这些东西已经超出了create-react-app
。
When you eject, you lose the ability of automatic updates but you gain more flexibility in the Babel and Webpack configuration.
弹出时,您将失去自动更新的能力,但在Babel和Webpack配置中获得了更大的灵活性。
When you eject the action is irreversible. You will get 2 new folders in your application directory, config
and scripts
. Those contain the configurations - and now you can start editing them.
当您弹出时,动作是不可逆的。 您将在应用程序目录, config
和scripts
获得2个新文件夹。 这些包含配置-现在您可以开始编辑它们。
If you already have a React app installed using an older version of React, first check the version by adding
console.log(React.version)
in your app, then you can update by runningyarn add react@16.7
, and yarn will prompt you to update (choose the latest version available). Repeat foryarn add react-dom@16.7
(change “16.7” with whatever is the newest version of React at the moment)如果您已经使用较旧版本的React安装了React应用,请先通过在应用中添加
console.log(React.version)
来检查版本,然后通过运行yarn add react@16.7
进行更新,yarn会提示您更新(选择可用的最新版本)。 对yarn add react-dom@16.7
重复上述步骤,yarn add react-dom@16.7
(将当前最新版本的“ 16.7”更改为“ 16.7”)
CodeSandbox (CodeSandbox)
An easy way to have the create-react-app
structure, without installing it, is to go to https://codesandbox.io/s and choose “React”.
无需安装create-react-app
结构的简单方法是转到https://codesandbox.io/s并选择“ React”。
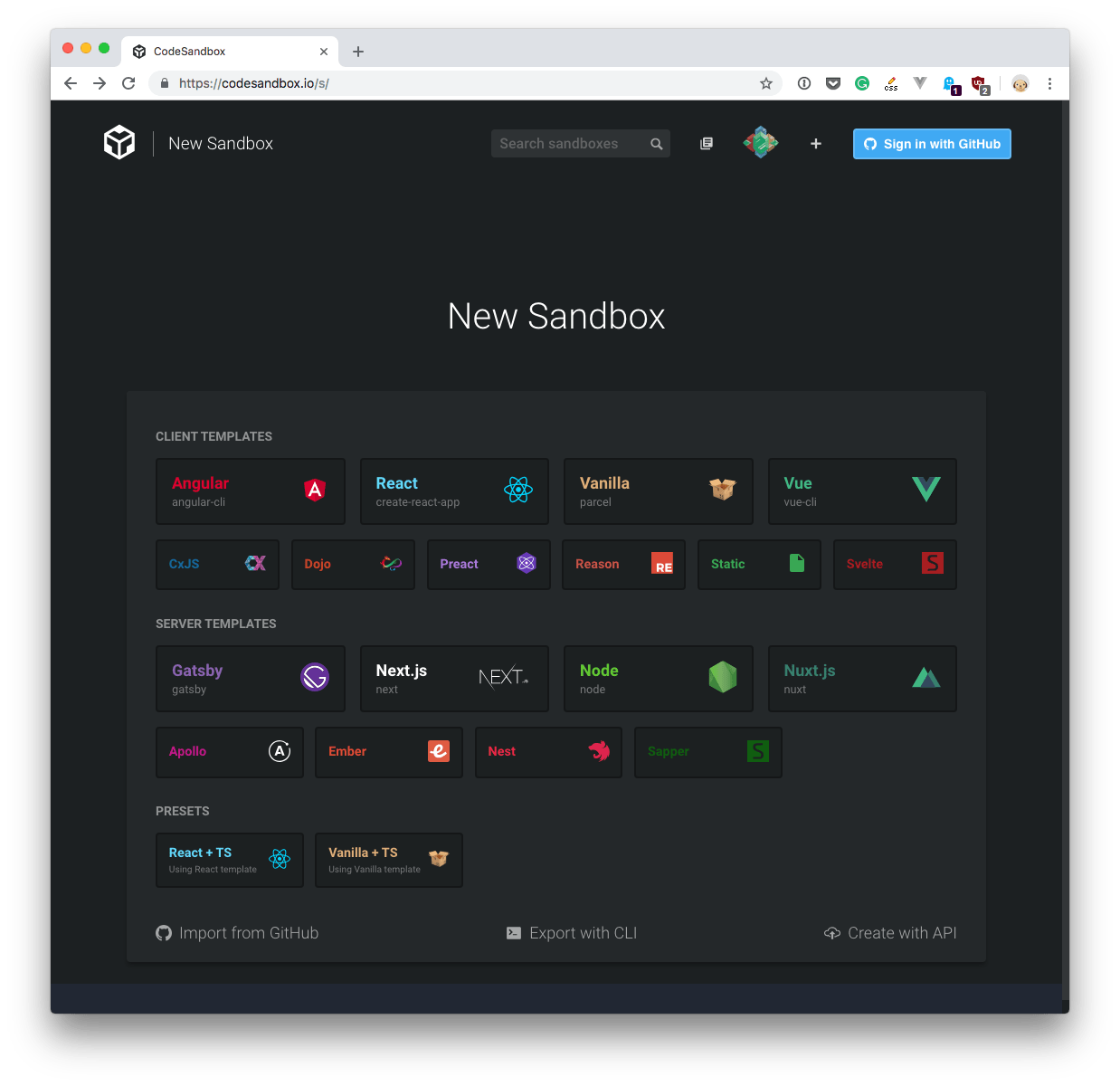
CodeSandbox is a great way to start a React project without having to install it locally.
CodeSandbox是启动React项目的好方法,而无需在本地安装它。
码笔 (Codepen)
Another great solution is Codepen.
另一个很棒的解决方案是Codepen 。
You can use this Codepen starter project which already comes pre-configured with React, with support for Hooks: https://codepen.io/flaviocopes/pen/VqeaxB
您可以使用已经预先配置了React并支持Hooks的Codepen入门项目: https ://codepen.io/flaviocopes/pen/VqeaxB
Codepen “pens” are great for quick projects with one JavaScript file, while “projects” are great for projects with multiple files, like the ones we’ll use the most when building React apps.
Codepen“笔”非常适合具有一个JavaScript文件的快速项目,而“ projects”非常适合具有多个文件的项目,例如在构建React应用程序时我们将使用最多的项目。
One thing to note is that in Codepen, due to how it works internally, you don’t use the regular ES Modules import
syntax, but rather to import for example useState
, you use
需要注意的一件事是,在Codepen中,由于其内部工作方式,您不使用常规的ES Modules import
语法,而是例如使用useState
来导入
const { useState } = React
and not
并不是
import { useState } from 'react'
react 安装