Working with dates can be complicated. No matter the technology, developers do feel the pain.
处理日期可能会很复杂 。 无论采用哪种技术,开发人员都会感到痛苦。
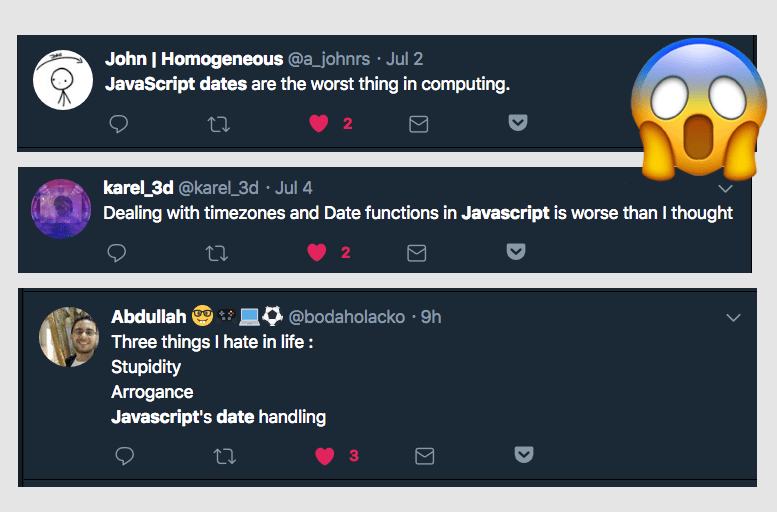
JavaScript offers us a date handling functionality through a powerful built-in object: Date
.
JavaScript通过强大的内置对象Date
为我们提供了日期处理功能。
Tip: you might want to use a library when working with dates. Moment.js and date-fns are two of the most popular ones.
日期对象 (The Date object)
A Date object instance represents a single point in time.
Date对象实例表示单个时间点。
Despite being named Date
, it also handles time.
尽管被命名为Date
,它也可以处理时间 。
初始化日期对象 (Initialize the Date object)
We initialize a Date object by using
我们通过使用以下方法初始化Date对象
new Date()
This creates a Date object pointing to the current moment in time.
这将创建一个指向当前时间的Date对象。
Internally, dates are expressed in milliseconds since Jan 1st 1970 (UTC). This date is important because as far as computers are concerned, that’s where it all began.
在内部,日期以毫秒为单位,自1970年1月1日(UTC)起。 这个日期很重要,因为就计算机而言,这就是一切的开始。
You might be familiar with the UNIX timestamp: that represents the number of seconds that passed since that famous date.
您可能对UNIX时间戳很熟悉:它代表自该著名日期以来经过的秒数 。
Important: the UNIX timestamp reasons in seconds. JavaScript dates reason in milliseconds.
要点:UNIX时间戳记以秒为单位。 JavaScript日期原因(以毫秒为单位)。
If we have a UNIX timestamp, we can instantiate a JavaScript Date object by using
如果我们有UNIX时间戳,则可以通过使用实例化JavaScript Date对象
const timestamp = 1530826365
new Date(timestamp * 1000)
unless the timestamp was generated by JS, in which case it’s already in the correct scale.
除非时间戳是由JS生成的,否则时间戳已经是正确的比例。
Make sure you pass a number (a string will get you an “invalid date” result - use parseInt()
in doubt)
确保您传递一个数字(字符串将为您提供“无效日期”结果-怀疑使用parseInt()
)
If we pass 0 we’d get a Date object that represents the time at Jan 1st 1970 (UTC):
如果传递0,则将获得一个Date对象,该对象代表1970年1月1日(UTC)的时间:
new Date(0)
If we pass a string rather than a number, then the Date object uses the parse
method to determine which date you are passing. Examples:
如果我们传递的是字符串而不是数字,则Date对象将使用parse
方法来确定传递的日期。 例子:
new Date('2018-07-22')
new Date('2018-07') //July 1st 2018, 00:00:00
new Date('2018') //Jan 1st 2018, 00:00:00
new Date('07/22/2018')
new Date('2018/07/22')
new Date('2018/7/22')
new Date('July 22, 2018')
new Date('July 22, 2018 07:22:13')
new Date('2018-07-22 07:22:13')
new Date('2018-07-22T07:22:13')
new Date('25 March 2018')
new Date('25 Mar 2018')
new Date('25 March, 2018')
new Date('March 25, 2018')
new Date('March 25 2018')
new Date('March 2018') //Mar 1st 2018, 00:00:00
new Date('2018 March') //Mar 1st 2018, 00:00:00
new Date('2018 MARCH') //Mar 1st 2018, 00:00:00
new Date('2018 march') //Mar 1st 2018, 00:00:00
There’s lots of flexibility here. You can add, or omit, the leading zero in months or days.
这里有很多灵活性。 您可以在几个月或几天内添加或省略前导零。
Be careful with the month/day position, or you might end up with the month being misinterpreted as the day.
请注意月/日的位置,否则可能会导致月份被误解为日期。
You can also use Date.parse
:
您也可以使用Date.parse
:
Date.parse('2018-07-22')
Date.parse('2018-07') //July 1st 2018, 00:00:00
Date.parse('2018') //Jan 1st 2018, 00:00:00
Date.parse('07/22/2018')
Date.parse('2018/07/22')
Date.parse('2018/7/22')
Date.parse('July 22, 2018')
Date.parse('July 22, 2018 07:22:13')
Date.parse('2018-07-22 07:22:13')
Date.parse('2018-07-22T07:22:13')
Date.parse
will return a timestamp (in milliseconds) rather than a Date object.
Date.parse
将返回时间戳(以毫秒为单位),而不是Date对象。
You can also pass a set of ordered values that represent each part of a date: the year, the month (starting from 0), the day, the hour, the minutes, seconds and milliseconds:
您还可以传递一组代表日期各部分的有序值:年,月(从0开始),日,时,分,秒和毫秒:
new Date(2018, 6, 22, 7, 22, 13, 0)
new Date(2018, 6, 22)
The minimum should be 3 parameters, but most JavaScript engines also interpret less than these:
最小值应为3个参数,但大多数JavaScript引擎的解释也少于这些:
new Date(2018, 6) //Sun Jul 01 2018 00:00:00 GMT+0200 (Central European Summer Time)
new Date(2018) //Thu Jan 01 1970 01:00:02 GMT+0100 (Central European Standard Time)
In any of these cases, the resulting date is relative to the timezone of your computer. This means that two different computers might output a different value for the same date object.
在任何这些情况下,结果日期都是相对于计算机时区的。 这意味着两台不同的计算机可能为同一日期对象输出不同的值 。
JavaScript, without any information about the timezone, will consider the date as UTC, and will automatically perform a conversion to the current computer timezone.
没有任何时区信息JavaScript会将日期视为UTC,并将自动执行到当前计算机时区的转换。
So, summarizing, you can create a new Date object in 4 ways
综上所述,您可以通过4种方式创建新的Date对象
passing no parameters, creates a Date object that represents “now”
不传递任何参数 ,创建一个表示“现在”的Date对象
passing a number, which represents the milliseconds from 1 Jan 1970 00:00 GMT
传递一个数字 ,该数字表示格林尼治标准时间1970年1月1日00:00以来的毫秒数
passing a string, which represents a date
传递一个字符串 ,它代表一个日期
passing a set of parameters, which represent the different parts of a date
传递一组参数 ,这些参数代表日期的不同部分
时区 (Timezones)
When initializing a date you can pass a timezone, so the date is not assumed UTC and then converted to your local timezone.
初始化日期时,您可以传递时区,因此不假定该日期为世界标准时间,然后将其转换为本地时区。
You can specify a timezone by adding it in +HOURS format, or by adding the timezone name wrapped in parentheses:
您可以通过以+ HOURS格式添加时区或通过添加用括号括起来的时区名称来指定时区:
new Date('July 22, 2018 07:22:13 +0700')
new Date('July 22, 2018 07:22:13 (CET)')
If you specify a wrong timezone name in the parentheses, JavaScript will default to UTC without complaining.
如果您在括号中指定了错误的时区名称,则JavaScript将默认为UTC,而不会抱怨。
If you specify a wrong numeric format, JavaScript will complain with an “Invalid Date” error.
如果您指定了错误的数字格式,JavaScript会抱怨“ Invalid Date”错误。
日期转换和格式 (Date conversions and formatting)
Given a Date object, there are lots of methods that will generate a string from that date:
给定一个Date对象,有很多方法可以从该日期开始生成一个字符串:
const date = new Date('July 22, 2018 07:22:13')
date.toString() // "Sun Jul 22 2018 07:22:13 GMT+0200 (Central European Summer Time)"
date.toTimeString() //"07:22:13 GMT+0200 (Central European Summer Time)"
date.toUTCString() //"Sun, 22 Jul 2018 05:22:13 GMT"
date.toDateString() //"Sun Jul 22 2018"
date.toISOString() //"2018-07-22T05:22:13.000Z" (ISO 8601 format)
date.toLocaleString() //"22/07/2018, 07:22:13"
date.toLocaleTimeString() //"07:22:13"
date.getTime() //1532236933000
date.getTime() //1532236933000
Date对象的获取方法 (The Date object getter methods)
A Date object offers several methods to check its value. These all depends on the current timezone of the computer:
Date对象提供了几种检查其值的方法。 这些都取决于计算机的当前时区:
const date = new Date('July 22, 2018 07:22:13')
date.getDate() //22
date.getDay() //0 (0 means sunday, 1 means monday..)
date.getFullYear() //2018
date.getMonth() //6 (starts from 0)
date.getHours() //7
date.getMinutes() //22
date.getSeconds() //13
date.getMilliseconds() //0 (not specified)
date.getTime() //1532236933000
date.getTimezoneOffset() //-120 (will vary depending on where you are and when you check - this is CET during the summer). Returns the timezone difference expressed in minutes
There are equivalent UTC versions of these methods, that return the UTC value rather than the values adapted to your current timezone:
这些方法有等效的UTC版本,它们返回UTC值,而不是适合您当前时区的值:
date.getUTCDate() //22
date.getUTCDay() //0 (0 means sunday, 1 means monday..)
date.getUTCFullYear() //2018
date.getUTCMonth() //6 (starts from 0)
date.getUTCHours() //5 (not 7 like above)
date.getUTCMinutes() //22
date.getUTCSeconds() //13
date.getUTCMilliseconds() //0 (not specified)
编辑日期 (Editing a date)
A Date object offers several methods to edit a date value:
Date对象提供了几种编辑日期值的方法:
const date = new Date('July 22, 2018 07:22:13')
date.setDate(newValue)
date.setFullYear(newValue) //note: avoid setYear(), it's deprecated
date.setMonth(newValue)
date.setHours(newValue)
date.setMinutes(newValue)
date.setSeconds(newValue)
date.setMilliseconds(newValue)
date.setTime(newValue)
setDate
andsetMonth
start numbering from 0, so for example March is month 2.
setDate
和setMonth
从0开始编号,例如3月是月份2。
Example:
例:
const date = new Date('July 22, 2018 07:22:13')
date.setDate(23)
date //July 23, 2018 07:22:13
Fun fact: those methods “overlap”, so if you, for example, set date.setHours(48)
, it will increment the day as well.
有趣的事实:这些方法“重叠”,因此,例如,如果您设置date.setHours(48)
,它也会增加日期。
Good to know: you can add more than one parameter to setHours()
to also set minutes, seconds and milliseconds: setHours(0, 0, 0, 0)
- the same applies to setMinutes
and setSeconds
.
提提您:您可以在setHours()
添加多个参数来设置分钟,秒和毫秒: setHours(0, 0, 0, 0)
-同样适用于setMinutes
和setSeconds
。
As for get, also set methods have an UTC equivalent:
至于get ,set方法也具有UTC等效项:
const date = new Date('July 22, 2018 07:22:13')
date.setUTCDate(newValue)
date.setUTCDay(newValue)
date.setUTCFullYear(newValue)
date.setUTCMonth(newValue)
date.setUTCHours(newValue)
date.setUTCMinutes(newValue)
date.setUTCSeconds(newValue)
date.setUTCMilliseconds(newValue)
获取当前时间戳 (Get the current timestamp)
If you want to get the current timestamp in milliseconds, you can use the shorthand
如果要以毫秒为单位获取当前时间戳,则可以使用速记
Date.now()
instead of
代替
new Date().getTime()
JavaScript努力工作正常 (JavaScript tries hard to work fine)
Pay attention. If you overflow a month with the days count, there will be no error, and the date will go to the next month:
请注意。 如果您用天数计满了一个月,则不会有错误,并且日期将转到下个月:
new Date(2018, 6, 40) //Thu Aug 09 2018 00:00:00 GMT+0200 (Central European Summer Time)
The same goes for months, hours, minutes, seconds and milliseconds.
几个月,几小时,几分钟,几秒钟和几毫秒都一样。
根据地区设置日期格式 (Format dates according to the locale)
The Internationalization API, well supported in modern browsers (notable exception: UC Browser), allows you to translate dates.
国际化API在现代浏览器中得到了很好的支持 (值得注意的例外:UC浏览器),它允许您翻译日期。
It’s exposed by the Intl
object, which also helps localizing numbers, strings and currencies.
它由Intl
对象公开,该对象还有助于本地化数字,字符串和货币。
We’re interested in Intl.DateTimeFormat()
.
我们对Intl.DateTimeFormat()
感兴趣。
Here’s how to use it.
这是使用方法。
Format a date according to the computer default locale:
根据计算机的默认语言环境设置日期格式:
// "12/22/2017"
const date = new Date('July 22, 2018 07:22:13')
new Intl.DateTimeFormat().format(date) //"22/07/2018" in my locale
Format a date according to a different locale:
根据其他区域设置日期格式:
new Intl.DateTimeFormat('en-US').format(date) //"7/22/2018"
Intl.DateTimeFormat
method takes an optional parameter that lets you customize the output. To also display hours, minutes and seconds:
Intl.DateTimeFormat
方法采用一个可选参数,使您可以自定义输出。 要同时显示小时,分钟和秒:
const options = {
year: 'numeric',
month: 'numeric',
day: 'numeric',
hour: 'numeric',
minute: 'numeric',
second: 'numeric'
}
new Intl.DateTimeFormat('en-US', options).format(date) //"7/22/2018, 7:22:13 AM"
new Intl.DateTimeFormat('it-IT', options2).format(date) //"22/7/2018, 07:22:13"
Here’s a reference of all the properties you can use.
比较两个日期 (Compare two dates)
You can calculate the difference between two dates using Date.getTime()
:
您可以使用Date.getTime()
计算两个日期之间的差:
const date1 = new Date('July 10, 2018 07:22:13')
const date2 = new Date('July 22, 2018 07:22:13')
const diff = date2.getTime() - date1.getTime() //difference in milliseconds
In the same way you can check if two dates are equal:
您可以用相同的方式检查两个日期是否相等:
const date1 = new Date('July 10, 2018 07:22:13')
const date2 = new Date('July 10, 2018 07:22:13')
if (date2.getTime() === date1.getTime()) {
//dates are equal
}
Keep in mind that getTime() returns the number of milliseconds, so you need to factor in time in the comparison. July 10, 2018 07:22:13
is not equal to new July 10, 2018
. In this case you can use setHours(0, 0, 0, 0)
to reset the time.
请记住,getTime()返回毫秒数,因此您需要在比较中考虑时间。 July 10, 2018 07:22:13
不等于新的July 10, 2018
。 在这种情况下,您可以使用setHours(0, 0, 0, 0)
重置时间。