函数式编程 返回函数
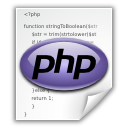
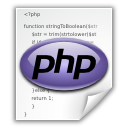
Functional Programming – How to Write Functional Code in PHP Functional programming can be defined in simple terms as a programming paradigm that do not change the state of a program instead it uses pure functions. A pure function is a function that has the ability to accept a value and return another value without changing the input supplied to it. It is characterized by its ability to support functions that are of high order. This implies that these functions take and return other functions. Also functional programming supports systems that are elaborated in nature, that is, they can prevent the issue of null pointers that is a problem in object oriented programming. A programming paradigm that is functional has the following attributes: do not alter the states which make parallelism easier, deals mostly with a function which is the smallest unit hence enhances readability of code, has deterministic functions that enable stability of a program.
函数式编程–如何在PHP中编写函数式代码函数式编程可以简单地定义为一种编程范例,它不更改程序的状态,而是使用纯函数。 纯函数是一种能够接受一个值并返回另一个值而无需更改提供给它的输入的函数。 它的特点是能够支持高级功能。 这意味着这些函数采用并返回其他函数。 函数式编程还支持本质上精心设计的系统,也就是说,它们可以防止在面向对象编程中出现问题的空指针问题。 具有功能的编程范例具有以下属性:不更改使并行变得更容易的状态,主要处理最小单元的功能,从而提高代码的可读性,具有确定性功能,可确保程序的稳定性。
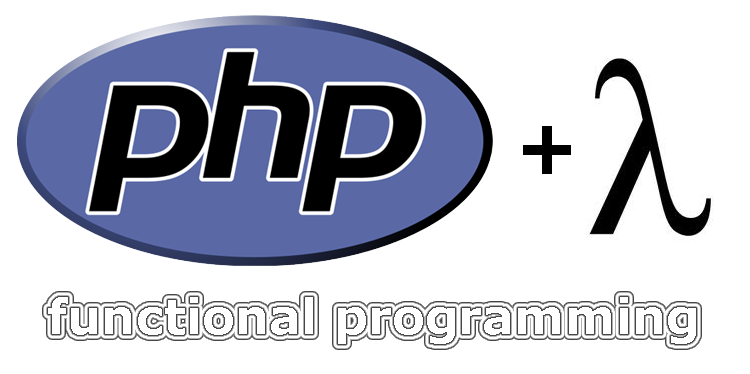
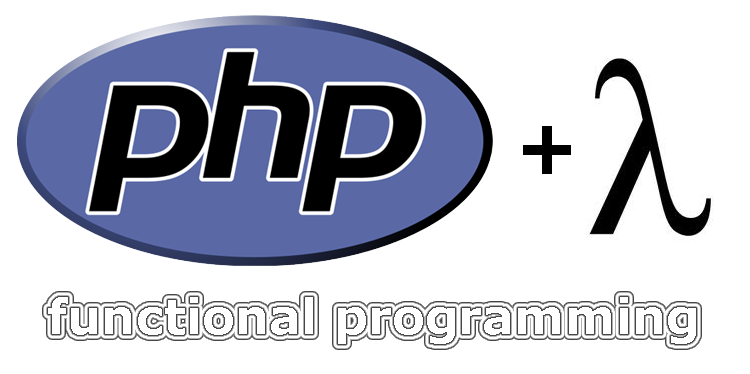
PHP如何实现函数式编程 (How PHP implements functional programming)
PHP has the following characteristics that make it support functional programming. They include:
PHP具有以下特性,使其支持函数式编程。 它们包括:
匿名函数或lambda函数 (Anonymous functions or lambda functions)
They are functions that use internal closure class during their implementation. In PHP 5.3.x they use the invoke() function. In PHP 5.4.x an anonymous function can be defined inside a class but to access the properties and methods of that class it uses variables like $this. Basically they are classes that do not contain a name. The values that are seen by anonymous functions are those that were bound during function definition.
它们是在实现过程中使用内部闭包类的函数。 在PHP 5.3.x中,它们使用invoke()函数。 在PHP 5.4.x中,可以在类内部定义匿名函数,但是要访问该类的属性和方法,它使用$ this之类的变量。 基本上,它们是不包含名称的类。 匿名函数看到的值是在函数定义期间绑定的值。
关闭 (Closures)
By definition a closure is similar to an anonymous function but the difference is that a closure encloses some parts of its surroundings (external scope). Since PHP uses early binding by default the values at the external scope can be seen by implementing late binding. This is done by passing by reference the variables outside the scope which is implemented applying the keyword use. For example
根据定义,闭包类似于匿名函数,但不同之处在于闭包将其周围环境的某些部分(外部范围)包围起来。 由于PHP默认使用早期绑定,因此可以通过实现后期绑定来查看外部作用域的值。 这是通过将引用传递给范围外的变量来完成的,该范围是使用关键字use实现的。 例如
$variable = 10;
// Create a closure using a variable outside of the scope of the anonymous function.
$closure = function($arg) use ($variable) {
Return $arg + $variable;
};
$variable = 10;
// Create a closure using a variable outside of the scope of the anonymous function.
$closure = function($arg) use ($variable) {
Return $arg + $variable;
};
部分函数和计算 (Partial functions and currying)
In PHP a partial function is derived from a general function that takes many variables in that a partial function has the capability of fixing most of the variables in a general function. It can be implemented using closures as follows
在PHP中,部分函数是从具有许多变量的常规函数派生而来的,因为部分函数具有将大多数变量固定在常规函数中的能力。 可以使用以下闭包来实现
$general_function = function ($a, $b) {
Return $a * $a - $b * $b;
};
$partial_function = function ($a) use ($general_function) {
Return $general_function ($a, 5);
};
Currying is a technique whereby a function that takes one argument can be created from a function that takes multiple arguments. Example:
$add2 = curry($add, 2); //returns a new function reference, to add(), bound with 2 as the first argument
$add2 (8); // return 10
$general_function = function ($a, $b) {
Return $a * $a - $b * $b;
};
$partial_function = function ($a) use ($general_function) {
Return $general_function ($a, 5);
};
Currying is a technique whereby a function that takes one argument can be created from a function that takes multiple arguments. Example:
$add2 = curry($add, 2); //returns a new function reference, to add(), bound with 2 as the first argument
$add2 (8); // return 10
Curry is not a PHP function but it can be created.
Curry不是PHP函数,但可以创建。
高阶函数 (Higher order functions)
Higher order functions are functions that a capable of accepting functions as their input parameters. The input function’s parameters must accept the same number of parameters and the outputted function takes the same number of parameters. Higher order functions are implemented by closures and lambdas.
高阶函数是能够接受函数作为其输入参数的函数。 输入函数的参数必须接受相同数量的参数,而输出函数采用相同数量的参数。 高阶函数由闭包和lambda实现。
纯函数,不变的数据 (Pure functions, immutable data)
It is not easy to implement this in PHP but to make it possible one has to avoid state and mutable data.PHP implements pure functions by using variables such as global or static but these variables do not alter the inputs and outputs.PHP also implements immutable data by defining its variables as constants.
在PHP中实现此功能并非易事,但要避免状态和可变数据成为可能.PHP通过使用诸如global或static之类的变量来实现纯函数,但这些变量不会改变输入和输出.PHP还实现不可变通过将其变量定义为常量来获取数据。
递归 (Recursion)
It is a technique in programming whereby the output of a problem relies on the outputs of other smaller problems but the smaller problems must be from the same problem. Recursion is used so as to maintain the state and prevent data mutation. Recursion is not implemented in a structured way rather by function calls.
这是一种编程技术,问题的输出依赖于其他较小问题的输出,但是较小问题必须来自同一问题。 使用递归以保持状态并防止数据突变。 递归不是通过结构化的方式而是通过函数调用来实现的。
优点 (Advantages)
Functional programming has many advantages as far as PHP is concerned but not all advantages apply to PHP since its not a programming language that is functional in its design. Some of the advantages include:
就PHP而言,函数式编程具有许多优点,但并非所有优点都适用于PHP,因为它不是在设计中起作用的编程语言。 一些优点包括:
- Referencing: anonymous functions are used to reference factions that kept for future use. 引用:匿名函数用于引用保留以备将来使用的派系。
- Secure programs: this is achieved by using immutable data and maintaining the same state whereby a program is divided functions to make it manageable. 安全程序:这是通过使用不变数据并保持相同状态来实现的,程序被划分功能以使其易于管理。
- Code reuse: this is achieved through recursion whereby we use the results of an existing problem to solve another problem. 代码重用:这是通过递归实现的,通过递归,我们可以使用现有问题的结果来解决另一个问题。
缺点 (Disadvantages)
- The cost of programming: recursion comes with some internal overheads such as space and time that brings about efficiency problems. 编程的代价:递归会带来一些内部开销,例如空间和时间,从而带来效率问题。
- It is not easy to learn functional programming as compared to imperative since it has tools that gives light to solve old problems. 与命令式编程相比,学习函数式编程并不容易,因为它具有可以解决旧问题的工具。
In conclusion functional programming is just not only a programming paradigm since it takes into consideration the composition of functions and their arrangements without using specific steps explicitly. This calls for languages such as PHP to implement functional programming so as to solve commercial problems.
总之,函数式编程不仅是编程范例,因为它考虑了功能的组成及其安排,而没有明确地使用特定步骤。 这需要诸如PHP之类的语言来实现功能编程,从而解决商业问题。
翻译自: https://www.script-tutorials.com/functional-programming-php/
函数式编程 返回函数