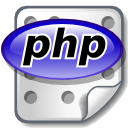
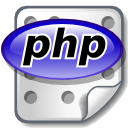
Akismet – spam protection. Today we will continue PHP lessons. And today we will talk about spam protection. I think every one have own website, and every one faced with appearing of unwanted content on the site (spam). What is spam? – this is (usually) any message which not relevant to this page – usually just an advertisement of something (and even with a backward link to another site). Yes, you can put the first line of defense – a captcha, but I think spammers are also ready for this and find ways to avoid the CAPTCHA (or, they even can solve its by self). In today’s tutorial I’ll show you how to create a second line of defense against spam – using web services – for example akismet.
Akismet –垃圾邮件防护。 今天,我们将继续PHP课程。 今天我们将讨论垃圾邮件防护。 我认为每个人都有自己的网站,每个人都面临网站上出现有害内容(垃圾邮件)的情况。 什么是垃圾邮件? –这是(通常)与该页面无关的任何消息–通常只是某物的广告(甚至带有指向另一个站点的反向链接)。 是的,您可以设置第一道防线-验证码,但我认为垃圾邮件发送者也已为此做好了准备,并设法避免使用验证码(或者甚至可以自行解决)。 在今天的教程中,我将向您展示如何使用Web服务(例如akismet)为垃圾邮件创建第二道防线。
Akismet is good automated spam protection web service for your website(s). Firstly – open this website, sign up here and obtain own Akismet API key. After, let’s go to Plugins & libraries page, and then, select PHP 5 class by Alex. We will use this class made by Alex for our website.
Akismet是为您的网站提供的出色的自动垃圾邮件防护Web服务。 首先–打开此网站,在此处注册并获取自己的Akismet API密钥。 之后,进入“ 插件和库”页面 ,然后选择AlexPHP 5类 。 我们将在我们的网站上使用Alex制作的此类。
Ok, here are online demo and downloadable package:
好的,这是在线演示和可下载的软件包:
现场演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Ok, download the example files and lets start coding !
好的,下载示例文件并开始编码!
步骤1. PHP (Step 1. PHP)
index.php (index.php)
<?
require_once ('classes/Akismet.class.php');
class MySpamProtection {
// variables
var $sMyAkismetKey;
var $sWebsiteUrl;
var $sAuthName;
var $sAuthEml;
var $sAuthUrl;
var $oAkismet;
// constructor
public function MySpamProtection() {
// set necessary values for variables
$this->sMyAkismetKey = '__YOUR_AKISMET_KEY__';
$this->sWebsiteUrl = '__YOUR_WEBSITE_URL__';
$this->sAuthName = '__YOUR_NAME__';
$this->sAuthEml = '';
$this->sAuthUrl = '';
// Akismet initialization
$this->oAkismet = new Akismet($this->sWebsiteUrl ,$this->sMyAkismetKey);
$this->oAkismet->setCommentAuthor($this->sAuthName);
$this->oAkismet->setCommentAuthorEmail($this->sAuthEml);
$this->oAkismet->setCommentAuthorURL($this->sAuthUrl);
}
public function isSpam($s) {
if (! $this->oAkismet) return false;
$this->oAkismet->setCommentContent($s);
return $this->oAkismet->isCommentSpam();
}
}
echo <<<EOF
<style type="text/css">
form div {
margin:10px;
}
form label {
width:90px;
float:left;
display:block;
}
</style>
<form action="" method="post">
<div><label for="author">Author</label><input id="author" name="author" type="text" value="" /></div>
<div><label for="comment">Comment</label><textarea id="comment" name="comment" cols="20" rows="4"></textarea></div>
<div><input name="submit" type="submit" value="Send" /></div>
</form>
EOF;
if ($_POST) {
// draw debug information
echo '<pre>';
print_r($_POST);
echo '</pre>';
// obtain sent info
$sPostAuthor = $_POST['author'];
$sCommentComment = $_POST['comment'];
// check for spam
$oMySpamProtection = new MySpamProtection();
$sAuthorCheck = ($oMySpamProtection->isSpam($sPostAuthor)) ? ' "Author" marked as Spam' : '"Author" not marked as Spam';
$sCommentCheck = ($oMySpamProtection->isSpam($sCommentComment)) ? ' "Comment" marked as Spam' : '"Comment" not marked as Spam';
echo $sAuthorCheck . '<br />' . $sCommentCheck;
}
?>
<?
require_once ('classes/Akismet.class.php');
class MySpamProtection {
// variables
var $sMyAkismetKey;
var $sWebsiteUrl;
var $sAuthName;
var $sAuthEml;
var $sAuthUrl;
var $oAkismet;
// constructor
public function MySpamProtection() {
// set necessary values for variables
$this->sMyAkismetKey = '__YOUR_AKISMET_KEY__';
$this->sWebsiteUrl = '__YOUR_WEBSITE_URL__';
$this->sAuthName = '__YOUR_NAME__';
$this->sAuthEml = '';
$this->sAuthUrl = '';
// Akismet initialization
$this->oAkismet = new Akismet($this->sWebsiteUrl ,$this->sMyAkismetKey);
$this->oAkismet->setCommentAuthor($this->sAuthName);
$this->oAkismet->setCommentAuthorEmail($this->sAuthEml);
$this->oAkismet->setCommentAuthorURL($this->sAuthUrl);
}
public function isSpam($s) {
if (! $this->oAkismet) return false;
$this->oAkismet->setCommentContent($s);
return $this->oAkismet->isCommentSpam();
}
}
echo <<<EOF
<style type="text/css">
form div {
margin:10px;
}
form label {
width:90px;
float:left;
display:block;
}
</style>
<form action="" method="post">
<div><label for="author">Author</label><input id="author" name="author" type="text" value="" /></div>
<div><label for="comment">Comment</label><textarea id="comment" name="comment" cols="20" rows="4"></textarea></div>
<div><input name="submit" type="submit" value="Send" /></div>
</form>
EOF;
if ($_POST) {
// draw debug information
echo '<pre>';
print_r($_POST);
echo '</pre>';
// obtain sent info
$sPostAuthor = $_POST['author'];
$sCommentComment = $_POST['comment'];
// check for spam
$oMySpamProtection = new MySpamProtection();
$sAuthorCheck = ($oMySpamProtection->isSpam($sPostAuthor)) ? ' "Author" marked as Spam' : '"Author" not marked as Spam';
$sCommentCheck = ($oMySpamProtection->isSpam($sCommentComment)) ? ' "Comment" marked as Spam' : '"Comment" not marked as Spam';
echo $sAuthorCheck . '<br />' . $sCommentCheck;
}
?>
Firstly – don`t forget to configure that class for you – apply your own __YOUR_AKISMET_KEY__, __YOUR_WEBSITE_URL__ and __YOUR_NAME__. And, you will able to use that service without problems. In this PHP code I prepared special own PHP class (MySpamProtection) which will using to check for spam. And, in second part of code – I drawing simple form, and, drawing response from Akismet plus some debug information to you.
首先-不要忘记为您配置该类-应用您自己的__YOUR_AKISMET_KEY __,__ YOUR_WEBSITE_URL__和__YOUR_NAME__。 并且,您将能够毫无问题地使用该服务。 在此PHP代码中,我准备了特殊PHP类(MySpamProtection),该类将用于检查垃圾邮件。 而且,在代码的第二部分–我绘制了简单的表单,并从Akismet绘制了响应,并向您提供了一些调试信息。
classes / Akismet.class.php (classes/Akismet.class.php)
This library I downloaded at ‘PHP 5 class by Alex’ page. Already available in our package.
我在“ PHP 5 class by Alex”页面上下载了该库。 我们的包装中已提供。
现场演示
结论 (Conclusion)
In result, now we have pretty easy class which you can use in your own projects which will help you to validate all incoming data. So you will protected from spam. Happy coding. Good luck in your projects!
结果,现在我们有了一个非常简单的类,您可以在自己的项目中使用该类,这将帮助您验证所有传入的数据。 因此,您将免受垃圾邮件的侵害。 快乐的编码。 在您的项目中祝您好运!
翻译自: https://www.script-tutorials.com/akismet-spam-protection/