php phar
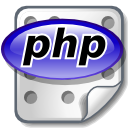
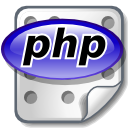
How to work with zip archives in PHP. Today we will continue PHP lessons. I guess you noticed that due your project become larger – the more and more files it contain. Sometimes it comes to the thousands of files. And then – Phar can help you. This utility allows us to pack a variety of files in the single library file. Thus, we can significantly reduce the number of include files in the project, and work with the entire library as with single file. It is also possible to have a packed (gzip/bzip2) version of the library. These principles already using in other languages, such as a DLL library for system languages (as example .Net: N# and VB.Net) or JAR files for Java. Read more.
如何使用PHP中的zip存档。 今天,我们将继续PHP课程。 我猜您已经注意到,由于您的项目越来越大–它包含的文件越来越多。 有时涉及数千个文件。 然后– Phar可以帮助您。 此实用程序使我们可以在单个库文件中打包各种文件。 因此,我们可以大大减少项目中包含文件的数量,并像处理单个文件一样使用整个库。 也可以具有库的压缩版本(gzip / bzip2)。 这些原则已在其他语言中使用,例如系统语言的DLL库(例如.Net:N#和VB.Net)或Java的JAR文件。 阅读更多。
Phar extension appear in PHP since version 5.2. And since 5.3 – this is part of PHP core. Firstly I can suggest you to check version of your PHP. And if you have PHP 5.2 – you will need to re-build PHP core with Phar, zlib and bzip2 extensions. If you have 5.3 – just ignore, Phar already installed by default.
从5.2版开始,Phar扩展出现在PHP中。 从5.3开始–这是PHP核心的一部分。 首先,我建议您检查PHP版本。 而且,如果您具有PHP 5.2,则需要使用Phar,zlib和bzip2扩展名重新构建PHP核心。 如果您有5.3,请忽略,默认情况下已安装Phar。
Make attention, by default, the PHAR archives have read-only access (so you will unable to create it). So, to have possibility to create own libraries (archives) you should to set phar.readonly = 0 in your php.ini and restart server.
请注意,默认情况下,PHAR归档文件具有只读访问权限(因此您将无法创建它)。 因此,要想创建自己的库(档案),您应该在php.ini中设置phar.readonly = 0并重新启动服务器。
In our lesson, I will tell you how you can create own libraries and use it. Here are samples and downloadable package:
在我们的课程中,我将告诉您如何创建和使用自己的库。 以下是示例和可下载的软件包:
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Ok, download the example files and lets start coding !
好的,下载示例文件并开始编码!
步骤1. PHP (Step 1. PHP)
Firstly, lets prepare 2 sample classes for us:
首先,让我们为我们准备2个示例类:
classes / SampleClass.php (classes/SampleClass.php)
<?
class SampleClass {
var $sName;
var $sVersion;
// constructor
function SampleClass() {
$this->sName = 'I am Sample class';
$this->sVersion = '1.0.0';
}
function getAnyContent() {
return '<h1>Hello World from Sample class</h1>';
}
function getContent2() {
return '<h2>Get content 2</h2>';
}
}
?>
<?
class SampleClass {
var $sName;
var $sVersion;
// constructor
function SampleClass() {
$this->sName = 'I am Sample class';
$this->sVersion = '1.0.0';
}
function getAnyContent() {
return '<h1>Hello World from Sample class</h1>';
}
function getContent2() {
return '<h2>Get content 2</h2>';
}
}
?>
classes / SampleClass2.php (classes/SampleClass2.php)
<?
class SampleClass2 extends SampleClass {
// constructor
function SampleClass2() {
$this->sName = 'I am Sample class 2';
$this->sVersion = '1.0.2';
}
function getAnyContent() {
return '<h1>Hello World from Sample class 2</h1>';
}
}
?>
<?
class SampleClass2 extends SampleClass {
// constructor
function SampleClass2() {
$this->sName = 'I am Sample class 2';
$this->sVersion = '1.0.2';
}
function getAnyContent() {
return '<h1>Hello World from Sample class 2</h1>';
}
}
?>
Very easy, isn`t it? And then, I`ll prepare single file (in same folder) which will load both classes:
很简单,不是吗? 然后,我将准备一个文件(在同一文件夹中),该文件将加载两个类:
classes / index.php (classes/index.php)
<?
require_once('SampleClass.php');
require_once('SampleClass2.php');
?>
<?
require_once('SampleClass.php');
require_once('SampleClass2.php');
?>
My goal will wrap whole folder ‘classes’ into single library file.
我的目标是将整个文件夹“类”包装到单个库文件中。
Now, lets prepare ‘lib’ folder (with access to writing) in your root folder. And lets prepare our main PHP file which will able to compile our library, and it will contain demonstration that our library works well:
现在,让我们在根文件夹中准备“ lib”文件夹(具有写权限)。 然后准备我们的主PHP文件,该文件将能够编译我们的库,其中将包含我们的库运行良好的演示:
index.php (index.php)
<?
$sLibraryPath = 'lib/SampleLibrary.phar';
// we will build library in case if it not exist
if (! file_exists($sLibraryPath)) {
ini_set("phar.readonly", 0); // Could be done in php.ini
$oPhar = new Phar($sLibraryPath); // creating new Phar
$oPhar->setDefaultStub('index.php', 'classes/index.php'); // pointing main file which require all classes
$oPhar->buildFromDirectory('classes/'); // creating our library using whole directory
$oPhar->compress(Phar::GZ); // plus - compressing it into gzip
}
// when library already compiled - we will using it
require_once('phar://'.$sLibraryPath.'.gz');
// demonstration of work
$oClass1 = new SampleClass();
echo $oClass1->getAnyContent();
echo '<pre>';
print_r($oClass1);
echo '</pre>';
$oClass2 = new SampleClass2();
echo $oClass2->getAnyContent();
echo $oClass2->getContent2();
echo '<pre>';
print_r($oClass2);
echo '</pre>';
?>
<?
$sLibraryPath = 'lib/SampleLibrary.phar';
// we will build library in case if it not exist
if (! file_exists($sLibraryPath)) {
ini_set("phar.readonly", 0); // Could be done in php.ini
$oPhar = new Phar($sLibraryPath); // creating new Phar
$oPhar->setDefaultStub('index.php', 'classes/index.php'); // pointing main file which require all classes
$oPhar->buildFromDirectory('classes/'); // creating our library using whole directory
$oPhar->compress(Phar::GZ); // plus - compressing it into gzip
}
// when library already compiled - we will using it
require_once('phar://'.$sLibraryPath.'.gz');
// demonstration of work
$oClass1 = new SampleClass();
echo $oClass1->getAnyContent();
echo '<pre>';
print_r($oClass1);
echo '</pre>';
$oClass2 = new SampleClass2();
echo $oClass2->getAnyContent();
echo $oClass2->getContent2();
echo '<pre>';
print_r($oClass2);
echo '</pre>';
?>
Here you can see result of our page:
在这里您可以看到我们页面的结果:
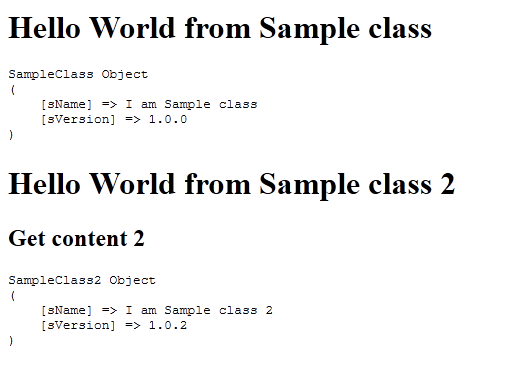
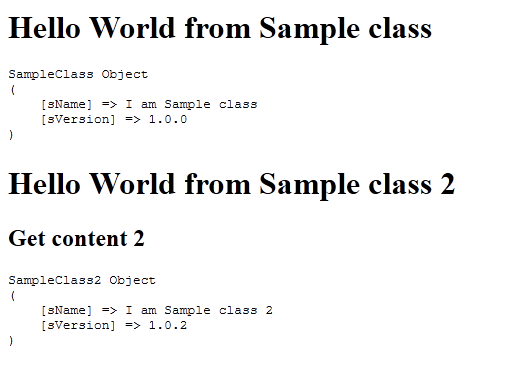
In beginning, I check, are compiled library (lib/SampleLibrary.phar) already exists or not. If exist – I don`t will compile it again, but if not exist – we will automatically compile (and pack) our library. Firstly lets check ‘readonly’ variable into Off state (or using php.ini or via ini_set function). Then, in constructor of Phar – pass name of our future library. After, I using ‘setDefaultStub’ function to make some auto-execute file which will executing when we including our library. In Phar extension it called ‘stab’. After, via ‘buildFromDirectory’ function I compiling our phar file into library, and via ‘compress’ function – pack it into gzip (SampleLibrary.phar.gz) version. Accessing to our classes will direct, without necessarity to extract our library (all via PHP Stream Wrapper).
首先,我检查是否已存在编译的库(lib / SampleLibrary.phar)。 如果存在-我不会再次编译,但是如果不存在-我们将自动编译(并打包)我们的库。 首先,让“ readonly”变量进入Off状态(或使用php.ini或通过ini_set函数)。 然后,在Phar的构造函数中-传递我们未来库的名称。 之后,我使用'setDefaultStub'函数制作一些自动执行文件,该文件将在包含库时执行。 在Phar扩展中,它称为“ stab”。 之后,通过“ buildFromDirectory”功能,将phar文件编译到库中,并通过“压缩”功能–将其打包到gzip(SampleLibrary.phar.gz)版本中。 访问我们的类将直接进行,而无需提取我们的库(全部通过PHP Stream Wrapper)。
You can use different ways (functions) to add files into library, this is possible via:
您可以使用不同的方式(函数)将文件添加到库中,这可以通过以下方式进行:
- via object properties 通过对象属性
- Phar::addFile() Phar :: addFile()
- Phar::addFromString() Phar :: addFromString()
- Phar::addEmptyDir() Phar :: addEmptyDir()
- Phar::buildFromDirectory() Phar :: buildFromDirectory()
- Phar::buildFromIterator() Phar :: buildFromIterator()
Ok, in first half or this file we created our library, then, we will use it, we will use ordinary ‘require_once’ function to include our result library (GZipped). Make attention to ‘phar://’, we should point that we going to attach Phar file (PHAR stream wrapper).
好的,在上半部分或该文件中,我们创建了我们的库,然后将使用它,我们将使用普通的'require_once'函数来包含我们的结果库(GZipped)。 注意“ phar://”,我们应该指出要附加Phar文件(PHAR流包装器)。
结论 (Conclusion)
In result, want to tell that here are several advantages of using Phar: its easy to use, you can pack multiple resource-files in single library, and, it have good performance. If you have any suggestions, or you just have to say – we are glad to hear it. Good luck in your projects!
结果,想告诉您使用Phar有以下几个优点:它易于使用,可以在单个库中打包多个资源文件,并且具有良好的性能。 如果您有任何建议,或者您只想说–我们很高兴听到它。 在您的项目中祝您好运!
翻译自: https://www.script-tutorials.com/phar-php-archiving-practice/
php phar