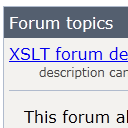
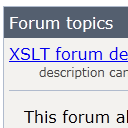
Animated forum with XSLT and AJAX. Today is a special day. Today our XSLT lessons will show some really interesting (attractive) results which you can apply in practice. We will make a forum. Initially we will display only the forum topics, and load posts dynamically (by mouse clicking on the topics) using ajax technology. I think you may have seen similar forums on the Internet, so I will tell you how to do it your own.
带有XSLT和AJAX的动画论坛。 今天是个特别的日子。 今天,我们的XSLT课程将显示一些非常有趣的(有吸引力的)结果,您可以在实践中应用它们。 我们将举办一个论坛。 最初,我们将仅显示论坛主题,并使用ajax技术动态加载帖子(通过单击主题)。 我想您可能已经在Internet上看到过类似的论坛,所以我将告诉您如何自己做。
Here are samples and downloadable package:
以下是示例和可下载的软件包:
论坛演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Ok, download the source files and lets start coding !
好的,下载源文件并开始编码!
步骤1.源XML (Step 1. Source XML)
Firstly lets define our structure of forum:
首先让我们定义论坛的结构:
forum.xml (forum.xml)
<?xml version="1.0" encoding="UTF-8"?>
<forum>
<topic title="XSLT forum demonstration" date="15.04.2011" description="description can be here">
<post date="15.04.2011" author="Diego">
<description><![CDATA[
This forum able to display any HTML entities
]]></description>
</post>
<post date="15.04.2011" author="Andrew">
<description><![CDATA[
As example <b>bold text</b>, <i>italic</i>, <u>underline</u>, <a href="http://www.google.com">links</a> etc
]]></description>
</post>
<post date="15.04.2011" author="James">
<description><![CDATA[
<p>Some HTML description 3, <a href="http://www.google.com">links</a> etc
]]></description>
</post>
<post date="15.04.2011" author="John">
<description><![CDATA[
Hello guys here
]]></description>
</post>
<post date="15.04.2011" author="Robert">
<description><![CDATA[
Wonderful forum, isn`t it?
]]></description>
</post>
</topic>
<topic title="sample forum topic 2" date="16.04.2011" description="description of topic 2">
<post date="16.04.2011" author="Michael">
<description><![CDATA[
sample record 1
]]></description>
</post>
<post date="16.04.2011" author="William">
<description><![CDATA[
sample record 2
]]></description>
</post>
<post date="16.04.2011" author="David">
<description><![CDATA[
sample record 3
]]></description>
</post>
</topic>
<topic title="sample forum topic 3" date="17.04.2011" description="description of topic 3">
<post date="17.04.2011" author="Charles">
<description><![CDATA[
sample record 3.1
]]></description>
</post>
<post date="17.04.2011" author="Joseph">
<description><![CDATA[
sample record 3.2
]]></description>
</post>
</topic>
</forum>
<?xml version="1.0" encoding="UTF-8"?>
<forum>
<topic title="XSLT forum demonstration" date="15.04.2011" description="description can be here">
<post date="15.04.2011" author="Diego">
<description><![CDATA[
This forum able to display any HTML entities
]]></description>
</post>
<post date="15.04.2011" author="Andrew">
<description><![CDATA[
As example <b>bold text</b>, <i>italic</i>, <u>underline</u>, <a href="http://www.google.com">links</a> etc
]]></description>
</post>
<post date="15.04.2011" author="James">
<description><![CDATA[
<p>Some HTML description 3, <a href="http://www.google.com">links</a> etc
]]></description>
</post>
<post date="15.04.2011" author="John">
<description><![CDATA[
Hello guys here
]]></description>
</post>
<post date="15.04.2011" author="Robert">
<description><![CDATA[
Wonderful forum, isn`t it?
]]></description>
</post>
</topic>
<topic title="sample forum topic 2" date="16.04.2011" description="description of topic 2">
<post date="16.04.2011" author="Michael">
<description><![CDATA[
sample record 1
]]></description>
</post>
<post date="16.04.2011" author="William">
<description><![CDATA[
sample record 2
]]></description>
</post>
<post date="16.04.2011" author="David">
<description><![CDATA[
sample record 3
]]></description>
</post>
</topic>
<topic title="sample forum topic 3" date="17.04.2011" description="description of topic 3">
<post date="17.04.2011" author="Charles">
<description><![CDATA[
sample record 3.1
]]></description>
</post>
<post date="17.04.2011" author="Joseph">
<description><![CDATA[
sample record 3.2
]]></description>
</post>
</topic>
</forum>
Forum contain several topics, each topic can contain variable amount of posts. Each post have name of Author, date of posting, and, text itself (Description) can contain HTML tags.
论坛包含几个主题,每个主题可以包含不同数量的帖子。 每个帖子都具有作者姓名,发布日期以及文本本身(描述)可以包含HTML标记。
步骤2. PHP (Step 2. PHP)
Here are easy PHP file which we using for xsl transformation:
这是我们用于xsl转换的简单PHP文件:
index.php (index.php)
<?php
if (version_compare(phpversion(), "5.3.0", ">=") == 1)
error_reporting(E_ALL & ~E_NOTICE & ~E_DEPRECATED);
else
error_reporting(E_ALL & ~E_NOTICE);
// create DomDocument and load our forum
$xml = new DOMDocument;
$xml->load('forum.xml');
$xsl = new DOMDocument;
switch ($_POST['action']) {
case 'posts':
$xsl->load('xslt/posts.xslt'); // importing xslt
$proc = new XSLTProcessor; // creating xslt processor
$proc->importStyleSheet($xsl); // attaching xsl rules
$proc->setParameter('', 'topic_id', (int)$_POST['i']); // we will set param for XSL
break;
default:
$xsl->load('xslt/forum.xslt'); // importing xslt
$proc = new XSLTProcessor; // creating xslt processor
$proc->importStyleSheet($xsl); // attaching xsl rules
break;
}
echo $proc->transformToXML($xml); // output
?>
<?php
if (version_compare(phpversion(), "5.3.0", ">=") == 1)
error_reporting(E_ALL & ~E_NOTICE & ~E_DEPRECATED);
else
error_reporting(E_ALL & ~E_NOTICE);
// create DomDocument and load our forum
$xml = new DOMDocument;
$xml->load('forum.xml');
$xsl = new DOMDocument;
switch ($_POST['action']) {
case 'posts':
$xsl->load('xslt/posts.xslt'); // importing xslt
$proc = new XSLTProcessor; // creating xslt processor
$proc->importStyleSheet($xsl); // attaching xsl rules
$proc->setParameter('', 'topic_id', (int)$_POST['i']); // we will set param for XSL
break;
default:
$xsl->load('xslt/forum.xslt'); // importing xslt
$proc = new XSLTProcessor; // creating xslt processor
$proc->importStyleSheet($xsl); // attaching xsl rules
break;
}
echo $proc->transformToXML($xml); // output
?>
Make attention to switch-case. For ajax response (when we clicking to our topics) I using first switch case (when action = posts). To determine which messages we will display (filter) I will pass ID of topic (topic_id) to our XSL script.
注意开关盒。 对于ajax响应(当我们单击主题时),我使用第一个切换条件(当action = posts时)。 为了确定我们将显示(过滤)的消息,我将主题ID(topic_id)传递给我们的XSL脚本。
步骤3. CSS (Step 3. CSS)
Here are used CSS file:
这是使用过CSS文件:
css / styles.css (css/styles.css)
body{background:#eee;font-family:Verdana, Helvetica, Arial, sans-serif;margin:0;padding:0}
.main{background:#FFF;width:698px;min-height:500px;overflow:hidden;border:1px #000 solid;margin:3.5em auto 2em;padding:1em 2em 2em}
p{margin:0;padding:0}
.forum{border:1px solid #B0BBCD}
.forum .header{color:#fff;background-color:#545F6F;padding:5px}
.forum .topic{border-bottom:2px solid #B0BBCD;background-color:#F3F2EF;padding:5px}
.forum .topic .desc{margin-left:30px;font-size:12px;color:#444}
.forum .topic .posts{border-top:1px solid #B0BBCD;display:none;margin-top:10px;padding:10px}
.forum .topic .posts .post{border-bottom:1px solid #B0BBCD;margin:5px}
.forum .post .date{font-size:10px;color:#444;text-align:right}
body{background:#eee;font-family:Verdana, Helvetica, Arial, sans-serif;margin:0;padding:0}
.main{background:#FFF;width:698px;min-height:500px;overflow:hidden;border:1px #000 solid;margin:3.5em auto 2em;padding:1em 2em 2em}
p{margin:0;padding:0}
.forum{border:1px solid #B0BBCD}
.forum .header{color:#fff;background-color:#545F6F;padding:5px}
.forum .topic{border-bottom:2px solid #B0BBCD;background-color:#F3F2EF;padding:5px}
.forum .topic .desc{margin-left:30px;font-size:12px;color:#444}
.forum .topic .posts{border-top:1px solid #B0BBCD;display:none;margin-top:10px;padding:10px}
.forum .topic .posts .post{border-bottom:1px solid #B0BBCD;margin:5px}
.forum .post .date{font-size:10px;color:#444;text-align:right}
Here are several styles of our forum – styles for topics, posts etc.
这是我们论坛的几种样式-主题,帖子等的样式。
步骤4. JS (Step 4. JS)
Here are used JS files:
以下是使用过的JS文件:
js / jquery.min.js (js/jquery.min.js)
This is just jQuery library, available in package.
这只是jQuery库,可在包中使用。
js / main.js (js/main.js)
function loadPosts(obj, i) {
$('.topic#'+i+' .posts').load('index.php',
{action: 'posts', i: i},
function() {
$(this).fadeIn('slow');
obj.unbind('click');
obj.click(function(e) {
$('.topic#'+i+' .posts').slideToggle('slow');
});
}
);
}
$(function(){
$('.topic').click(function() {
loadPosts( $(this), $(this).attr('id') );
});
});
function loadPosts(obj, i) {
$('.topic#'+i+' .posts').load('index.php',
{action: 'posts', i: i},
function() {
$(this).fadeIn('slow');
obj.unbind('click');
obj.click(function(e) {
$('.topic#'+i+' .posts').slideToggle('slow');
});
}
);
}
$(function(){
$('.topic').click(function() {
loadPosts( $(this), $(this).attr('id') );
});
});
When page loaded – I linking onClick events to our forum topics. After, when we clicking to Topic element, I using $.load to load posts of this Topic. And, unbind old onClick functional (we don`t will need to load this content twice), and adding new onClick event, which will toggle slide of these posts.
页面加载后–我将onClick事件链接到我们的论坛主题。 之后,当我们单击“主题”元素时,我使用$ .load加载该主题的帖子。 并且,取消绑定旧的onClick功能(我们不需要两次加载此内容),并添加新的onClick事件,这将切换这些帖子的幻灯片。
步骤5. XSLT (Step 5. XSLT)
And, the delicacy – used XSLT rules:
而且,美味佳肴–使用了XSLT规则:
xslt /论坛.xslt (xslt/forum.xslt)
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="html" doctype-system="http://www.w3.org/TR/html4/strict.dtd" doctype-public="-//W3C//DTD HTML 4.01//EN" indent="yes" />
<xsl:template match="/">
<html xml:lang="en">
<head>
<script src="js/jquery.min.js" type="text/javascript">></script>
<script src="js/main.js" type="text/javascript"></script>
<link media="all" href="css/styles.css" type="text/css" rel="stylesheet"/>
<title>Our XSLT-based ajaxy forum</title>
</head>
<body>
<div class="main">
<h2>Our XSLT-based ajaxy forum</h2>
<div class="forum">
<div class="header">Forum topics</div>
<xsl:for-each select="forum/topic">
<div class="topic">
<xsl:attribute name="id">
<xsl:value-of select="position()"/>
</xsl:attribute>
<a class="top_link" href="javascript:void(0)">
<xsl:value-of select="@title"/> (<xsl:value-of select="count(child::*)"/>)
</a>
<div class="desc">
<xsl:value-of select="@description"/>
</div>
<div class="posts"></div>
</div>
</xsl:for-each>
</div>
</div>
<xsl:comment>Copyright: AndrewP</xsl:comment>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="html" doctype-system="http://www.w3.org/TR/html4/strict.dtd" doctype-public="-//W3C//DTD HTML 4.01//EN" indent="yes" />
<xsl:template match="/">
<html xml:lang="en">
<head>
<script src="js/jquery.min.js" type="text/javascript">></script>
<script src="js/main.js" type="text/javascript"></script>
<link media="all" href="css/styles.css" type="text/css" rel="stylesheet"/>
<title>Our XSLT-based ajaxy forum</title>
</head>
<body>
<div class="main">
<h2>Our XSLT-based ajaxy forum</h2>
<div class="forum">
<div class="header">Forum topics</div>
<xsl:for-each select="forum/topic">
<div class="topic">
<xsl:attribute name="id">
<xsl:value-of select="position()"/>
</xsl:attribute>
<a class="top_link" href="javascript:void(0)">
<xsl:value-of select="@title"/> (<xsl:value-of select="count(child::*)"/>)
</a>
<div class="desc">
<xsl:value-of select="@description"/>
</div>
<div class="posts"></div>
</div>
</xsl:for-each>
</div>
</div>
<xsl:comment>Copyright: AndrewP</xsl:comment>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
As you can see, here we generating our main forum, and drawing only topics now. We will load posts ajaxy fr each Topic using jQuery, nice, isn`t is?
如您所见,这里我们生成了主要论坛,现在仅绘制主题。 我们将使用jQuery在每个主题上加载ajaxy帖子,不错,不是吗?
xslt / posts.xslt (xslt/posts.xslt)
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:for-each select="forum/topic[position() = $topic_id]/*">
<div class="post">
<xsl:attribute name="id">
<xsl:value-of select="position()"/>
</xsl:attribute>
<xsl:value-of select="description" disable-output-escaping="yes"/>
<div class="date">
posted at <xsl:value-of select="@date"/> by <xsl:value-of select="@author"/>
</div>
</div>
</xsl:for-each>
</xsl:template>
</xsl:stylesheet>
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:for-each select="forum/topic[position() = $topic_id]/*">
<div class="post">
<xsl:attribute name="id">
<xsl:value-of select="position()"/>
</xsl:attribute>
<xsl:value-of select="description" disable-output-escaping="yes"/>
<div class="date">
posted at <xsl:value-of select="@date"/> by <xsl:value-of select="@author"/>
</div>
</div>
</xsl:for-each>
</xsl:template>
</xsl:stylesheet>
Second XSL file we will use to generate list of posts for requested Topic. Hope that you don`t forget that we will request it ajaxy? We already passed $topic_id variable into this XSL above (in PHP code). So, we just should display all child ‘posts’ of our Topic.
第二个XSL文件,我们将使用它为请求的主题生成帖子列表。 希望您别忘了我们会要求它ajaxy吗? 我们已经在上面的XSL中(通过PHP代码)传递了$ topic_id变量。 因此,我们只应显示主题的所有子级“帖子”。
现场演示
结论 (Conclusion)
I hope that today’s article was very interesting, and I hope that interest to XSL language has not dried up :-) Good luck!
我希望今天的文章非常有趣,也希望对XSL语言的兴趣不会枯竭:-)祝您好运!
翻译自: https://www.script-tutorials.com/creating-ajaxy-forums-using-xslt/