wordpress后台样式
After developing WordPress plugins and themes for a while, you start thinking more and more about your theme performance and what problems could it cause for your customers. One of these problems is enqueuing scripts and styles. In this article we’ll cover the basics of enqueuing scripts and best practices for different use cases. You can check this article for a beginner overview on managing your WordPress assets.
开发了一段时间的WordPress插件和主题后,您开始越来越多地考虑主题性能以及它可能给客户带来什么问题。 这些问题之一是使脚本和样式排队。 在本文中,我们将介绍使脚本排队的基础以及针对不同用例的最佳实践。 您可以检查此文出于管理你的WordPress资产初学者概述 。
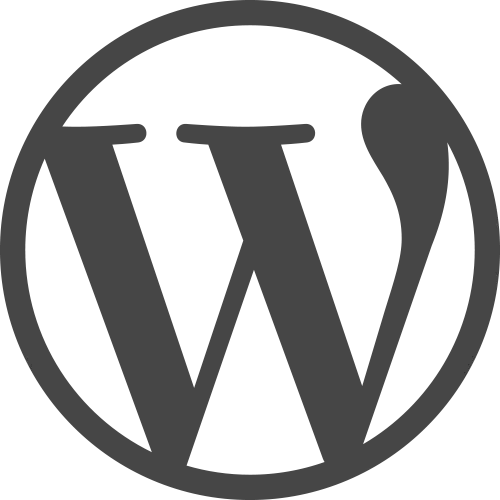
使脚本和样式入队 (Enqueuing Scripts and Styles)
If you need some CSS or JavaScript code to be injected into certain pages of your website, we tend to either add them inside the same markup file or add them using a direct URL to the file.
如果您需要将某些CSS或JavaScript代码注入网站的某些页面中,我们倾向于将它们添加到同一标记文件中,或者使用直接URL添加到文件中。
// ...
<script type="text/javascript">
// some JS code
</script>
<style type="text/css">
// some CSS code
</style>
//...
<script type="text/javascript" src="<?= plugins_url('assets/js/main.js') ?>"></script>
However, this is not the recommended way for adding scripts, and caching plugins won’t optimize and cache your assets to increase website performance. We need to use the admin_enqueue_scripts hook.
但是,这不是添加脚本的推荐方法,并且缓存插件不会优化和缓存您的资产以提高网站性能。 我们需要使用admin_enqueue_scripts钩子。
add_action( 'admin_enqueue_scripts', function($hook) {
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
} );
The first parameter for the wp_enqueue_script method is the script handler name, we should always prefix the handler to avoid any conflicts and make sure that our script will always be loaded.
wp_enqueue_script方法的第一个参数是脚本处理程序名称,我们应该始终为处理程序添加前缀,以避免任何冲突,并确保将始终加载脚本。
The current solution isn’t perfect, and our script is included in every back end page. We can use the $hook
variable passed to the callback function to test for a certain page.
当前的解决方案并不完美,我们的脚本包含在每个后端页面中。 我们可以使用传递给回调函数的$hook
变量来测试特定页面。
add_action( 'admin_enqueue_scripts', function($hook) {
if ( !in_array($hook, array("options-general.php", "post-new.php")) )
return;
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
} );
Now, our script will only be included inside the settings and new post pages. We can go further to only include our styles when the user is creating a new page.
现在,我们的脚本将仅包含在设置和新帖子页面中。 我们可以进一步扩展,仅在用户创建新页面时包括样式。
add_action( 'admin_enqueue_scripts', function($hook) {
if ( $hook == "post-new.php" && isset($_GET['post_type']) && $_GET['post_type'] == "page" )
{
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
}
} );
删除不必要的脚本 (Remove Unnecessary Scripts)
Most developers don’t care about enqueuing their scripts just when needed, this is why we’ll always end up getting unexpected errors and weird styling issues. When noticing that a script is throwing an error or causing conflicts, you can remove it before adding your own, this is often useful in debug mode.
大多数开发人员不在乎仅在需要时才排队他们的脚本,这就是为什么我们总是总会遇到意外错误和奇怪的样式问题的原因。 当注意到脚本引发错误或引起冲突时,可以在添加自己的脚本之前将其删除,这在调试模式下通常很有用。
add_action( 'admin_enqueue_scripts', function($hook) {
$unautorized_styles = [
'script1',
'another-script'
];
foreach ( $unautorized_styles as $handle )
{
wp_deregister_style( $handle );
}
// enqueue my scripts
} );
使用简码 (Working with Shortcodes)
Most plugins and themes provide a set of shortcodes to interact with the website, sometimes they need to inject scripts into the page. Sadly, we cannot test for front end pages as we did previously for the back end.
大多数插件和主题提供了一组与网站交互的短代码,有时它们需要将脚本注入页面。 遗憾的是,我们无法像以前那样测试前端页面。
An easy way to achieve this is to register a callback on the_content
filter and test if it contains your shortcode. If it returns yes, you can enqueue your scripts, otherwise do nothing.
一个简单的方法来实现这一目标是注册一个回调the_content
如果它包含您的简码过滤器和测试。 如果返回yes,则可以使脚本入队,否则不执行任何操作。
add_filter( 'the_content', function($content) {
if ( has_shortcode($content, "hello-shortcode") )
{
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/hello-shortcode.js') );
}
});
The above code works great, and will only enqueue our scripts for posts containing our shortcode. However, this solution has some serious performance issues on high load websites. We’ll create a more sophisticated solution for this problem.
上面的代码效果很好,并且只会在包含我们的短代码的帖子中加入我们的脚本。 但是,此解决方案在高负载网站上存在一些严重的性能问题。 我们将为这个问题创建一个更复杂的解决方案。
If you’re not already using Composer to load your classes, I advise you to start doing so. Our plugin composer.json
file would look like the following:
如果你还没有使用作曲家加载您的课,我劝你开始这样做。 我们的插件composer.json
文件如下所示:
// composer.json
{
"name": "Demo plugin",
"description": "",
"authors": [
{
"name": "Author name",
"email": "author.name@example.com"
}
],
"autoload": {
"psr-4": {
"ESP\\": "src/"
}
}
}
The src/shortcodes/HelloShortcode.php
class holds our shortcode definition.
在src/shortcodes/HelloShortcode.php
类持有我们的短代码的定义。
// src/shortcodes/HelloShortcode.php
namespace ESP\Shortcodes;
class HelloShortcode
{
static $loaded;
public $name;
public function __construct()
{
$this->name = "hello-shortcode";
}
public function run()
{
static::$loaded = true;
return "Hello shortcode!";
}
public function enqueueScripts()
{
if ( static::$loaded == false )
return;
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/hello-shortcode.js') );
}
}
The run
method will return the HTML markup for our shortcode, and will set the loaded
static attribute to true
when the shortcode is used.
run
方法将返回我们的简码HTML标记,并在使用简码时将已loaded
static属性设置为true
。
The enqueueScripts
method will enqueue our styles when the plugin is loaded. The remaining part will register our shortcode to be recognized inside the post content.
该enqueueScripts
加载插件时方法排队我们的风格。 其余部分将注册我们的简码,以便在帖子内容中被识别。
// plugin-file.php
require_once __DIR__."/vendor/autoload.php";
$shortcodes = [
'ESP\\Shortcodes\\HelloShortcode'
];
foreach ($shortcodes as $shortcode) {
$shortcode = new $shortcode;
add_shortcode($shortcode->name, [ $shortcode, 'run' ]);
}
add_action('wp_footer', function() use($shortcodes) {
foreach ($shortcodes as $shortcode) {
(new $shortcode)->enqueueScripts();
}
});
Surely we can avoid putting this code inside the main plugin file, but we’re going to keep things simple and readable.
当然,我们可以避免将这段代码放在主插件文件中,但是我们将使事情变得简单易读。
The $shortcodes
variable holds a list of available shortcodes. The first loop will register our shortcode and make the run
method the handler. Next, we’ll use the wp_footer
hook to enqueue our shortcode scripts before closing the body
tag.
在$shortcodes
变量保存可用短号码的列表。 第一个循环将注册我们的短代码,使run
方法的处理程序。 接下来,我们将使用wp_footer
钩在关闭之前,我们排队简码脚本body
标记。
You can now create a new page containing our shortcode and check if our script is loaded properly.
现在,您可以创建一个包含我们的简码的新页面,并检查脚本是否正确加载。
结论 (Conclusion)
This short article was a summary on how to enqueue your plugin and theme scripts, and the best way to avoid loading them inside every page on your website. If you have any question or comments, please post them below and I’ll do my best to answer them!
这篇简短的文章总结了如何使您的插件和主题脚本入队,以及避免将其加载到网站上每个页面中的最佳方法。 如果您有任何问题或意见,请在下面发布,我会尽力回答!
翻译自: https://www.sitepoint.com/enqueuing-scripts-styles-wordpress/
wordpress后台样式