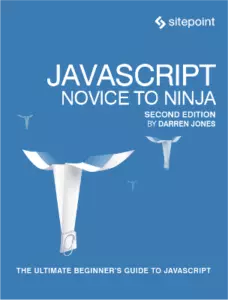
The following is a short extract from our new book, JavaScript: Novice to Ninja, 2nd Edition, written by Darren Jones. It’s the ultimate beginner’s guide to JavaScript. SitePoint Premium members get access with their membership, or you can buy a copy in stores worldwide.
以下是Darren Jones撰写的新书《 JavaScript:Ninja的新手》(第二版)的摘录。 这是JavaScript的终极入门指南。 SitePoint Premium成员可以使用其成员身份进行访问,或者您可以在世界各地的商店中购买副本。
It is a tradition when learning programming languages to start with a “Hello, world!” program. This is a simple program that outputs the phrase “Hello world!” to announce your arrival to the world of programming. We’re going to stick to this tradition and write this type of program in JavaScript. It will be a single statement that logs the phrase “Hello, world!” to the console.
学习编程语言以“你好,世界!”开始是一种传统。 程序。 这是一个简单的程序,输出短语“ Hello world!” 宣布您的编程世界。 我们将坚持这一传统,并使用JavaScript编写此类程序。 这将是一个记录短语“ Hello,world!”的语句。 到控制台。
To get started, you’ll need to open up your preferred console (either the Node REPL, browser console, or ES6 Console on the web). Once the console has opened, all you need to do is enter the following code:
首先,您需要打开首选控制台(Node REPL,浏览器控制台或Web上的ES6控制台 )。 打开控制台后,您只需输入以下代码:
console.log('Hello world!');
Then press Enter. if all went to plan you should see an output of “Hello, world!” displayed; similar to the screenshot below.
然后按Enter 。 如果一切都按计划进行,您应该会看到“ Hello,world!”的输出。 显示; 类似于下面的截图。
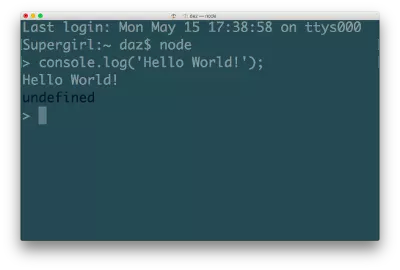
Congratulations, you’ve just written your first JavaScript program! It might not look like much, but a wise person once said that every ninja programmer’s journey begins with a single line of code (or something like that, anyway!).
恭喜,您已经编写了第一个JavaScript程序! 看起来可能并不多,但是一个聪明的人曾经说过,每个忍者程序员的旅程都始于单行代码(或者类似的东西!)。
浏览器中JavaScript (JavaScript in the Browser)
JavaScript is an interpreted language and needs a host environment to run. Because of its origins, the main environment that JavaScript runs in is the browser, although it can be run in other environments; for example, our first program that we just wrote ran in the Node REPL. Node can also be used to run JavaScript on a server. By far the most common use of JavaScript is still to make web pages interactive. Because of this, we should have a look at what makes up a web page before we go any further.
JavaScript是一种解释型语言,需要宿主环境才能运行。 由于其起源,JavaScript所运行的主要环境是浏览器,尽管它可以在其他环境中运行。 例如,我们刚编写的第一个程序在Node REPL中运行。 Node也可以用于在服务器上运行JavaScript。 到目前为止,JavaScript最普遍的用途仍然是使网页具有交互性。 因此,在继续之前,我们应该先看一下组成网页的内容。
网络的三层 (Three Layers of the Web)
Nearly all web pages are made up of three key ingredients ― HTML, CSS and JavaScript. HTML is used to mark up the content. CSS is the presentation layer, and JavaScript adds the interactivity.
几乎所有网页都由三个关键要素组成-HTML,CSS和JavaScript。 HTML用于标记内容。 CSS是表示层,而JavaScript添加了交互性。
Each layer builds on the last. A web page should be able to function with just the HTML layer ― in fact, many websites celebrate “naked day” when they remove the CSS layer from their site. A website using just the HTML layer will be in its purest form and look very old school, but should still be fully functional.
每层都建立在最后一层上。 网页应仅能与HTML层一起使用-实际上,许多网站在从其网站上删除CSS层时都会庆祝“ 裸日 ”。 仅使用HTML层的网站将是最纯净的形式,看起来非常老套,但仍应具有完整的功能。
保持这些层分开 (Keep These Layers Separate)
It is widely considered best practice to separate the concerns of each layer, so each layer is only responsible for one thing. Putting them altogether can lead to very complicated pages where all of the code is mixed up together in one file, causing “tag soup” or “code spaghetti”. This used to be the standard way of producing a website and there are still plenty of examples on the web that do this.
分离每一层关注点的最佳实践被广泛认为,因此每一层仅负责一件事。 将它们放在一起会导致非常复杂的页面,其中所有代码都混合在一起在一个文件中,从而导致“标签汤”或“代码意大利面条”。 这曾经是制作网站的标准方法,但网上仍然有很多示例可以做到这一点。
简洁JavaScript (Unobtrusive JavaScript)
When JavaScript was initially used, it was designed to be inserted directly into the HTML code, as can be seen in this example that will display a message when a button is clicked:
最初使用JavaScript时,它被设计为直接插入HTML代码中,如在本示例中可以看到的那样,当单击按钮时将显示一条消息:
<button id='button' href='#' onclick='alert("Hello World")'>Click Me</a>
This made it difficult to see what was happening, as the JavaScript code was mixed up with the HTML. It also meant the code was tightly coupled to the HTML, so any changes in the HTML required the JavaScript code to also be changed to stop it breaking.
由于JavaScript代码与HTML混合在一起,因此很难看清发生了什么。 这也意味着代码与HTML紧密耦合,因此HTML中的任何更改都需要更改JavaScript代码以阻止其中断。
It’s possible to keep the JavaScript code away from the rest of the HTML by placing it inside its own <script>
tags. The following code will achieve the same result as that above:
通过将JavaScript代码放在自己的<script>
标记中,可以使JavaScript代码远离HTML的其余部分。 以下代码将实现与上述相同的结果:
<script>
const btn = document.getElementById('button');
btn.addEventListener('click', function() {
alert('Hello World!');
});
</script>
This is better because all the JavaScript is in one place, between the two script tags, instead of mixed with the HTML tags.
这样比较好,因为所有JavaScript都位于两个脚本标签之间的一个位置,而不是与HTML标签混合。
We can go one step further and keep the JavaScript code completely separate from the HTML and CSS in its own file. This can be linked to using the src
attribute in the script
tag to specify the file to link to:
我们可以更进一步,将JavaScript代码与HTML和CSS完全隔离在自己的文件中。 这可以链接到使用script
标记中的src
属性来指定要链接到的文件:
<script src='main.js'></script>
The JavaScript code would then be placed in a file called main.js
inside the same directory as the HTML document. This concept of keeping the JavaScript code completely separate is one of the core principles of unobtrusive JavaScript.
然后,将JavaScript代码放在与HTML文档相同目录下的main.js
文件中。 保持JavaScript代码完全独立的这一概念是不干扰JavaScript的核心原则之一。
In a similar way, the CSS should also be kept in a separate file, so the only code in a web page is the actual HTML with links to the CSS and JavaScript files. This is generally considered best practice and is the approach we’ll be using in the book.
同样,CSS也应保存在单独的文件中,因此网页中唯一的代码是带有指向CSS和JavaScript文件链接的实际HTML。 这通常被认为是最佳实践,也是我们在本书中将使用的方法。
自闭合标签 (Self-Closing Tags)
If you’ve used XML or XHTML, you might have come across self-closing tags such as this script tag:
如果您使用过XML或XHTML,则可能会遇到自闭标签,例如以下脚本标签:
<script src='main.js' />
These will fail to work in HTML5, so should be avoided.
这些将无法在HTML5中使用,因此应避免使用。
You may see some legacy code that uses the language attribute:
您可能会看到一些使用language属性的旧代码:
<script src='main.js' language='javascript'></script>
This is unnecessary in HTML5, but it will still work.
这在HTML5中是不必要的,但仍然可以使用。
优雅降级和逐步增强 (Graceful Degradation and Progressive Enhancement)
Graceful degradation is the process of building a website so it works best in a modern browser that uses JavaScript, but still works to a reasonable standard in older browsers, or if JavaScript or some of its features are unavailable. An example of this are programs that are broadcast in high definition (HD) ― they work best on HD televisions but still work on a standard TV; it’s just the picture will be of a lesser quality. The programs will even work on a black-and-white television.
正常降级是网站建设的过程,因此它在使用JavaScript的现代浏览器中效果最佳,但在较旧的浏览器中,或者在JavaScript或其某些功能不可用的情况下,仍然可以达到合理的标准。 例如,以高清(HD)广播的节目-它们在高清电视上效果最好,但在标准电视上仍然可以播放; 只是图片质量较差。 这些程序甚至可以在黑白电视上运行。
Progressive enhancement is the process of building a web page from the ground up with a base level of functionality, then adding extra enhancements if they are available in the browser. This should feel natural if you follow the principle of three layers, with the JavaScript layer enhancing the web page rather than being an essential element that the page cannot exist without. An example might be the phone companies who offer a basic level of phone calls, but provide extra services such as call-waiting and caller ID if your telephone supports it.
渐进增强是指从头开始构建具有基本功能的网页,然后在浏览器中可用的情况下添加其他增强的过程。 如果您遵循三层原则,那么JavaScript层可以增强Web页面,而不是页面不可缺少的基本元素,那么这应该很自然。 例如,电话公司可以提供基本级别的电话,但是如果您的电话支持的话,它们可以提供额外的服务,例如呼叫等待和呼叫者ID。
Whenever you add JavaScript to a web page, you should always think about the approach you want to take. Do you want to start with lots of amazing effects that push the boundaries, then make sure the experience degrades gracefully for those who might not have the latest and greatest browsers? Or do you want to start off building a functional website that works across most browsers, then enhance the experience using JavaScript? The two approaches are similar, but subtly different.
每当将JavaScript添加到网页时,都应始终考虑要采用的方法。 您是否要从许多令人惊叹的效果开始突破,然后确保对于那些可能没有最新最好的浏览器的用户而言,体验会优雅地下降? 还是要开始构建可在大多数浏览器上使用的功能网站,然后使用JavaScript增强体验? 两种方法是相似的,但是有细微的不同。
您的第二个JavaScript程序 (Your Second JavaScript Program)
We’re going to finish the chapter with a second JavaScript program that will run in the browser. This example is more complicated than the previous one and includes a lot of concepts that will be covered in later chapters in more depth, so don’t worry if you don’t understand everything at this stage! The idea is to show you what JavaScript is capable of, and introduce some of the important concepts that will be covered in the upcoming chapters.
我们将使用将在浏览器中运行的第二个JavaScript程序来结束本章。 这个示例比上一个示例更加复杂,并且包含许多概念,这些概念将在后面的章节中更深入地介绍,因此,如果您在此阶段不了解所有内容,请不要担心! 这样做的目的是向您展示JavaScript的功能,并介绍一些将在接下来的章节中介绍的重要概念。
We’ll follow the practice of unobtrusive JavaScript mentioned earlier and keep our JavaScript code in a separate file. Start by creating a folder called rainbow
. Inside that folder create a file called rainbow.html
and another called main.js
.
我们将遵循前面提到的不干扰JavaScript的做法,并将我们JavaScript代码保存在单独的文件中。 首先创建一个名为rainbow
的文件夹。 该文件夹内创建一个名为rainbow.html
和另一个名为main.js
。
Let’s start with the HTML. Open up rainbow.html
and enter the following code:
让我们从HTML开始。 打开rainbow.html
并输入以下代码:
<head>
<meta charset='utf-8'>
<title>I Can Click A Rainbow</title>
</head>
<body>
<button id='button'>click me</button>
<script src='main.js'></script>
</body>
</html>
This file is a fairly standard HTML5 page that contains a button with an ID of button
. The ID attribute is very useful for JavaScript to use as a hook to access different elements of the page. At the bottom is a script
tag that links to our JavaScript file.
该文件是一个相当标准HTML5页面,其中包含ID为button
。 ID属性对于JavaScript用作挂钩来访问页面的不同元素非常有用。 底部是一个script
标记,该标记链接到我们JavaScript文件。
Now for the JavaScript. Open up main.js
and enter the following code:
现在使用JavaScript。 打开main.js
并输入以下代码:
const btn = document.getElementById('button');
const rainbow = ['red','orange','yellow','green','blue','rebeccapurple','violet'];
function change() {
document.body.style.background = rainbow[Math.floor(7*Math.random())];
}
btn.addEventListener('click', change);
Our first task in the JavaScript code is to create a variable called btn
(we cover variables in Chapter 2).
JavaScript代码中的第一个任务是创建一个名为btn
的变量(我们将在第2章中介绍变量)。
We then use the document.getElementById
function to find the HTML element with the ID of btn
(Finding HTML elements is covered in Chapter 6). This is then assigned to the btn
variable.
然后,我们使用document.getElementById
函数查找ID为btn
HTML元素(第6章介绍了查找HTML元素)。 然后将其分配给btn
变量。
We now create another variable called rainbow
. An array containing a list of strings of different colors is then assigned to the rainbow
variable (we cover strings and variables in Chapter 2 and arrays in Chapter 3).
现在,我们创建另一个名为rainbow
变量。 然后,将包含不同颜色字符串列表的数组分配给rainbow
变量(我们在第2章介绍了字符串和变量,在第3章介绍了数组)。
Then we create a function called change
(we cover functions in Chapter 4). This sets the background color of the body element to one of the colors of the rainbow (changing the style of a page will be covered in Chapter 6). This involves selecting a random number using the built-in Math
object (covered in Chapter 5) and selecting the corresponding color from the rainbow
array.
然后,我们创建一个名为change
的函数(我们将在第4章中介绍这些函数)。 这会将body元素的背景色设置为彩虹色之一(更改页面样式将在第6章中介绍)。 这涉及到使用内置的Math
对象(在第5章中介绍过)选择一个随机数,并从rainbow
数组中选择相应的颜色。
Last of all, we create an event handler, which checks for when the button is clicked on. When this happens it calls the change
function that we just defined (event handlers are covered in Chapter 7).
最后,我们创建一个事件处理程序 ,该事件处理程序将检查单击按钮的时间。 发生这种情况时,它将调用我们刚定义的change
函数(第7章介绍了事件处理程序)。
Open rainbow.html
in your favorite browser and try clicking on the button a few times. If everything is working correctly, the background should change to every color of the rainbow, such as in the screenshot below.
在您喜欢的浏览器中打开rainbow.html
,然后尝试单击按钮几次。 如果一切正常,则背景应更改为彩虹的每种颜色,如下面的屏幕截图所示。
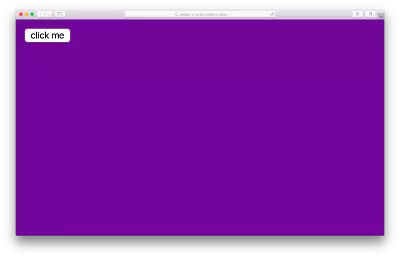
If you want to try this out quickly, you can checkout the code on CodePen. For the sake of getting some practice in though, I would recommend you also take the time to create these files, write up the code by hand and try running it in your browser as well.
如果您想快速尝试一下,可以在CodePen上签出代码。 为了进行一些练习,我建议您也花些时间创建这些文件,手动编写代码,并尝试在浏览器中运行它。
不要破坏网络 (Don’t Break the Web)
An important concept in the development of the JavaScript language is that it has to be backward compatible. That is, all old code must work the same way when interpreted by an engine running a new specification (it’s a bit like saying that PlayStation 4 must still be able to run games created for PlayStation 1, 2 and 3). This is to prevent JavaScript from “breaking the web” by making drastic changes that would mean legacy code on some websites not running as expected in modern browsers.
JavaScript语言开发中的一个重要概念是它必须向后兼容 。 也就是说,当运行新规范的引擎解释所有旧代码时,它们的工作方式必须相同(这有点像说PlayStation 4必须仍然能够运行为PlayStation 1、2和3创建的游戏)。 这是为了防止JavaScript通过进行重大更改而“破坏网络”,这将意味着某些网站上的旧代码无法在现代浏览器中按预期运行。
So new versions of JavaScript can’t do anything that isn’t already possible in previous versions of the language. All that changes is the notation used to implement a particular feature to make it easier to write. This is known as syntactic sugar, as it allows an existing piece of code to be written in a nicer and more succinct way.
因此,新版本JavaScript无法执行以前版本的语言所无法完成的任何工作。 所有这些更改都是用于实现特定功能以使其易于编写的符号。 这被称为语法糖 ,因为它允许以一种更好,更简洁的方式编写现有代码。
The fact that all versions of JavaScript are backwardly compatible means that we can use transpilers to convert code from one version of JavaScript into another. For example, you could write your code using the most up-to-date version of JavaScript and then transpile it into version 5 code, which would work in virtually any browser.
所有版本JavaScript都向后兼容,这意味着我们可以使用编译器将代码从一个JavaScript版本转换为另一个JavaScript版本。 例如,您可以使用最新版本JavaScript编写代码,然后将其转换为版本5代码,该代码几乎可以在任何浏览器中使用。
A new version of ECMAScript every year means it’s likely that browsers will always be slightly when it comes to implementing the latest features (they’re getting faster at doing this, but it’s still taken two years for most browsers to support ES6 modules). This means that if you want to use the most up-to-date coding techniques, you’ll probably have to rely on using a transpiler, such as Babel, at some point.
每年都会有一个新版本的ECMAScript,这意味着浏览器在实现最新功能方面总是会稍微有些慢(它们的执行速度越来越快,但是大多数浏览器支持ES6模块仍需要两年时间)。 这意味着,如果您想使用最新的编码技术,则有时可能不得不依靠使用诸如Babel的编译器。
If you find that some code isn’t working in your browser, you can add the following link into your HTML document:
如果发现浏览器中无法使用某些代码,则可以将以下链接添加到HTML文档中:
<script src='https://unpkg.com/babel-standalone@6/babel.min.js'></script>
Note that this link needs to go before any JavaScript that needs to be transpiled.
请注意,此链接需要在需要翻译的任何JavaScript 之前进行。
You also have to change the type
attribute to text/babel
in any links to JavaScript files. For example, the link to the JavaScript file in the example above would change to:
您还必须在任何指向JavaScript文件的链接中将type
属性更改为text/babel
。 例如,上例中指向JavaScript文件的链接将更改为:
<script type='text/babel' src='main.js'></script>
This isn’t the best long-term solution as it requires the browser to transpile all the code at run-time, although it’s fine for experimenting with code. A better solution is to transpile your code as part of a build process, which is covered in Chapter 15.
这不是最好的长期解决方案,因为它要求浏览器在运行时转换所有代码,尽管可以尝试使用代码。 更好的解决方案是在构建过程中编译代码,这在第15章中进行了介绍。
A number of online editors such as CodePen, Babel REPL and JS Fiddle allow you to transpile code in the browser. The ECMAScript 6 compatibility table also contains up-to-date information about which features have been implemented in various transpilers.
许多在线编辑器(例如CodePen , Babel REPL和JS Fiddle)使您可以在浏览器中转换代码。 ECMAScript 6兼容性表还包含有关在各种编译器中实现了哪些功能的最新信息。
翻译自: https://www.sitepoint.com/hello-world-your-first-javascript-programs/