do_wp_page
In any development process, errors are something that you cannot avoid, no matter how competent and thorough you are when writing your code.
在任何开发过程中,无论编写代码时多么熟练和全面,都无法避免错误。
As a WordPress developer, it is your job to make sure any of your code errors are being handled correctly without affecting the end user. WordPress is shipped with a basic error handling class, namely WP_Error
that can be used and integrated into your code for basic error handling.
作为WordPress开发人员,确保正确处理任何代码错误而不影响最终用户是您的工作。 WordPress附带了一个基本的错误处理类,即WP_Error
,可以使用该类并将其集成到您的代码中以进行基本的错误处理。
In this tutorial, we will take a look at the basic anatomy of the WP_Error
class, how it works and most importantly, we will cover how to integrate the WP_Error
class within our application.
在本教程中,我们将研究WP_Error
类的基本结构,如何工作,最重要的是,我们将介绍如何在应用程序中集成WP_Error
类。
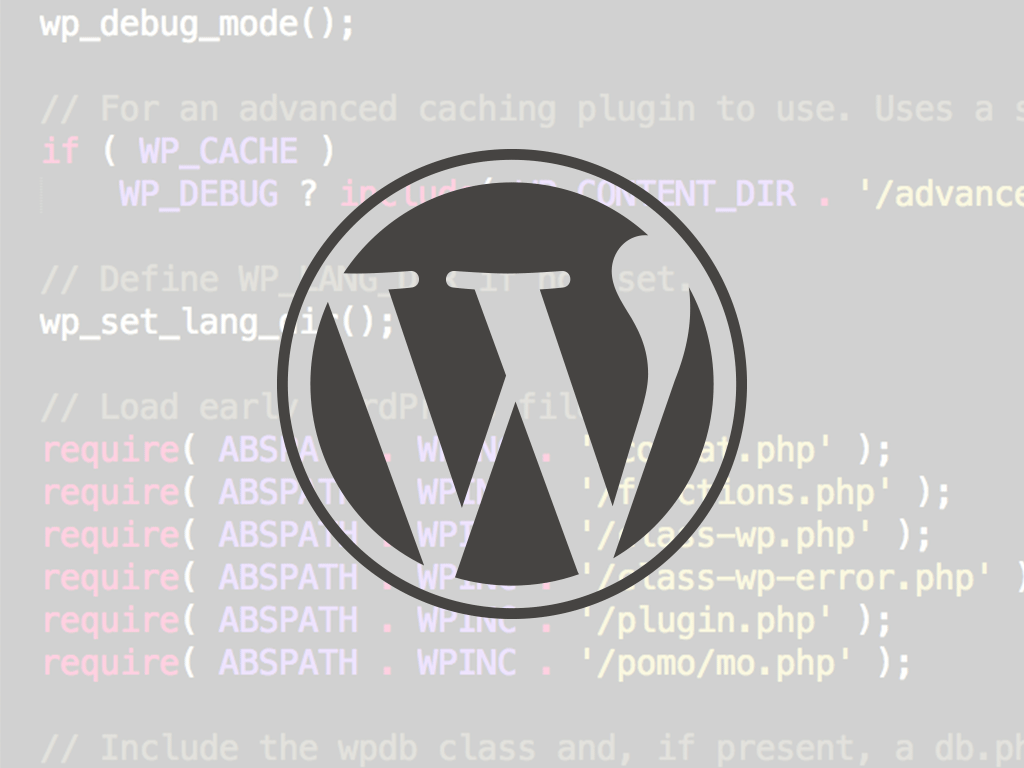
里面有什么 (What’s Inside)
The structure of the WP_Error
class is actually really simple and yet powerful enough to be used as an error handling mechanism for your plugin.
WP_Error
类的结构实际上非常简单,但功能强大到足以用作插件的错误处理机制。
The whole source code for the WP_Error
class can be found in the wp-includes/class-wp-error.php
file. Let’s take a look at its properties and the methods available.
WP_Error
类的整个源代码可以在wp-includes/class-wp-error.php
文件中找到。 让我们看一下它的属性和可用的方法。
物产 (Properties)
WP_Error
only has two private properties namely $errors
and $error_data
. $errors
is used to store related error messages while $error_data
can be used optionally to store related data that you want to access later on. WP_Error
uses a simple key-value pair to store the related errors and data into the object, so the key defined in WP_Error
must be unique to avoid it overwriting the previously defined key.
WP_Error
只有两个私有属性,即$errors
和$error_data
。 $errors
用于存储相关的错误消息,而$error_data
可以用于存储以后要访问的相关数据。 WP_Error
使用简单的键值对将相关的错误和数据存储到对象中,因此WP_Error
定义的键必须唯一,以避免覆盖先前定义的键。
方法 (Methods)
WP_Error
comes with various methods that are ultimately modifying the two properties it contains. Let’s take a look at a few of them.
WP_Error
带有各种方法,这些方法最终都会修改它包含的两个属性。 让我们看看其中的一些。
get_error_codes
– Returns all the available error codes for that specificWP_Error
instance. If you only need the first error code, there is another separate function calledget_error_code
.get_error_codes
–返回该特定WP_Error
实例的所有可用错误代码。 如果只需要第一个错误代码,则还有另一个单独的函数,称为get_error_code
。get_error_messages( $code )
– If$code
is not supplied, the function will simply return all the stored messages in that specificWP_Error
instance. Again, if you only need to return a message for a specific error code, simply useget_error_message( $code )
.get_error_messages( $code )
–如果未提供$code
,该函数将仅返回该特定WP_Error
实例中的所有已存储消息。 同样,如果只需要为特定的错误代码返回一条消息,则只需使用get_error_message( $code )
。add( $code, $message, $data )
– This function is particularly useful when you want to modify the errors stored in the instantiated.WP_Error
object. Note that even$message
and$data
are not compulsory, the$errors
variable will still be populated.add( $code, $message, $data )
–当您要修改实例化中存储的错误时,此功能特别有用。WP_Error
对象。 请注意,即使$message
和$data
不是强制性的,$errors
变量仍将被填充。add_data( $data, $code)
– If you intend to only modify the$error_data
property, this function can be used. Note that the$code
argument comes second, opposite of what we have with theadd
method. If no$code
is supplied, the error data will be added to the first error code instead.add_data( $data, $code)
–如果您只想修改$error_data
属性,则可以使用此函数。 请注意,$code
参数排在第二位,与add
方法相反。 如果未提供$code
,则会将错误数据添加到第一个错误代码中。remove( $code )
– This is a rather new method added recently in WordPress 4.1 that removes all the associated error messages and data from the specific key.remove( $code )
–这是最近在WordPress 4.1中添加的一个相当新的方法,该方法从特定密钥中删除所有相关的错误消息和数据。
功能 (Function)
How can you know if a specific variable or data returned from a function is an instance of the WP_Error
? Well, there is a utility function that you can simply check that, called is_wp_error
which return true or false depending on the variable given.
您如何知道某个特定变量或从函数返回的数据是否是WP_Error
的实例? 好吧,有一个实用程序函数可以简单地检查它,即is_wp_error
,它根据给定的变量返回true或false。
is_wp_error( $thing )
– Return true if$thing
is aWP_Error
instance, false otherwhise.is_wp_error( $thing )
–如果$thing
是WP_Error
实例,则返回true,否则返回false。
实施自己的 (Implement Your Own)
It is not enough to just to know how the WP_Error
internally works, you also need to learn how to implement it well within your own application. Let’s walk through a few examples to get a better idea on how it works.
仅仅了解WP_Error
内部如何工作还不够,还需要学习如何在自己的应用程序中很好地实现它。 让我们来看一些示例,以更好地了解其工作原理。
处理WordPress核心函数返回的错误 (Handling Errors Returned by WordPress Core Function)
WordPress offers a lot of utility functions that can be used to speed up our development process. Most of the functions are also well equipped with basic error handling capabilities that we can use.
WordPress提供了许多实用程序功能,可用于加快我们的开发过程。 大多数功能还配备了我们可以使用的基本错误处理功能。
As an example, wp_remote_post
is a very helpful function that we can use to make a remote POST request to a specific URL. However, we cannot expect that the remote URL is always accessible or our request is always successful. What we do know from the codex page is that this function will return the WP_Error
on failure. This knowledge will help us along the way to implement error handling correctly in our application.
例如, wp_remote_post
是一个非常有用的函数,我们可以使用wp_remote_post
特定URL发出远程POST请求。 但是,我们不能期望远程URL总是可访问的,或者我们的请求总是成功的。 我们从法典页面知道的是,此函数将在失败时返回WP_Error
。 这些知识将帮助我们在应用程序中正确实现错误处理。
Take a look at this snippet.
看看这个片段。
// Make a responseo to remote url $url
$response = wp_remote_post( $url, array(
'timeout' => 30,
'body' => array( 'foo' => 'bar' )
)
);
if ( is_wp_error( $response ) ) {
echo 'ERROR: ' . $response->get_error_message();
} else {
// do something
}
As you can see, we are executing a remote POST request to a $url
. However, we do not simply take the $response
data as it is, but instead, we do a little checking with our handy is_wp_error
function that we covered earlier. If everything is fine, then only we can proceed with what we want to do with our $response
.
如您所见,我们正在执行对$url
的远程POST请求。 但是,我们不只是按原样获取$response
数据,而是对我们前面介绍的方便的is_wp_error
函数进行一些检查。 如果一切都很好,那么只有我们可以继续进行$response
想要做的事情。
在您自己的应用程序中返回自定义错误 (Returning a Custom Error in Your Own Application)
Let’s say you have a custom function that handles a contact form submission called handle_form_submission
. Assuming, we have a custom form setup somewhere, let’s see how we can make the function better by implementing our own error handling functionality.
假设您有一个自定义函数,用于处理联系表单提交,称为handle_form_submission
。 假设我们在某个地方有一个自定义表单设置,让我们看看如何通过实现自己的错误处理功能来使功能更好。
function handle_form_submission() {
// do your validation, nonce and stuffs here
// Instantiate the WP_Error object
$error = new WP_Error();
// Make sure user supplies the first name
if ( empty( $_POST['first_name'] ) ) {
$error->add( 'empty', 'First name is required' );
}
// And last name too
if ( empty( $_POST['last_name'] ) ) {
$error->add( 'empty', 'Last name is required' );
}
// Check for email address
if ( empty( $_POST['email'] ) ) {
$error->add( 'empty', 'Last name is required' );
} elseif ( ! is_email( $_POST['email'] ) ) {
$error->add( 'invalid', 'Email address must be valid' );
}
// Lastly, check for the message
if ( empty( $_POST['message'] ) ) {
$error->add( 'empty', 'Your message is required' );
}
// Send the result
if ( empty( $error->get_error_codes() ) ) {
return $error;
}
// Everything is fine
return true;
}
Of course, you will need to implement your own sanitization and validation in that functions as well, but that is out of scope for this tutorial. Now, knowing that we are correctly returning the WP_Error
instance on error, we can use it to supply a more meaningful error message to the end user.
当然,您还需要在该函数中实现自己的清理和验证,但这在本教程中不涉及。 现在,知道我们正确地返回了错误的WP_Error
实例,我们就可以使用它向最终用户提供更有意义的错误消息。
Assuming you have a specific part in your application to display the form submission error, you can do this:
假设您的应用程序中有特定部分显示表单提交错误,则可以执行以下操作:
$result = handle_form_submission();
if ( is_wp_error( $result ) ) {
echo '<p>Ops, something went wrong. Please rectify this errors</p>';
echo '<ul>';
echo '<li>' . implode( '</li><li>', $result->get_error_messages() ) . '</li>';
echo '</ul>';
} else {
// Everything went well
}
外部参考 (External References)
结论 (Conclusion)
Perfecting your craft in software development also means knowing what to do when your code fails to do what it is supposed to do, and making sure that your application can handle it gracefully.
完善软件开发技能还意味着知道在代码无法执行应做的事情时该怎么做,并确保您的应用程序可以正常处理它。
As far as WordPress goes, using the WP_Error
class that is shipped with it provides a rather simple yet powerful implementation of error handling that you can integrate it within your application.
就WordPress而言,使用它附带的WP_Error
类可以提供一种相当简单而强大的错误处理实现,您可以将其集成到应用程序中。
What are your thoughts? How are you using the WP_Error
in your daily development process? Please share it with us below.
你觉得呢?你有没有什么想法? 在日常开发过程中如何使用WP_Error
? 请在下面与我们分享。
翻译自: https://www.sitepoint.com/an-introduction-to-the-wp-error-class/
do_wp_page