In the first part, we introduced the YouTube API and built a small demo to list the most popular videos on YouTube. In this part, we will extend our application to have search functionality, and we’ll also list the available categories on YouTube to let the user narrow down their area of interest. Let’s get started.
在第一部分中 ,我们介绍了YouTube API,并构建了一个小型演示以列出YouTube上最受欢迎的视频。 在这一部分中,我们将扩展应用程序以具有搜索功能,并且还将列出YouTube上的可用类别,以使用户缩小他们的关注范围。 让我们开始吧。
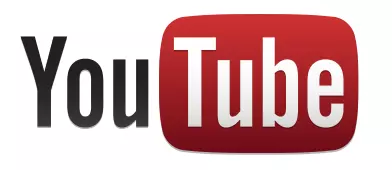
清单类别 (Listing Categories)
YouTube videos are grouped by categories. We can retrieve the list of categories for a certain country using their ISO code. The result can also be localized using an optional parameter like en_US
, fr_FR
, etc. You can read more in the documentation.
YouTube视频按类别分组。 我们可以使用其ISO代码检索特定国家/地区的类别列表。 还可以使用可选参数(例如en_US
, fr_FR
等)对结果进行本地化。您可以在文档中阅读更多内容 。
// app/Http/routes.php
Route::get('/categories', ['uses' => 'YouTubeAPIController@categories']);
// app/Http/Controllers/YouTubeAPIController.php
public function categories()
{
$youtube = \App::make('youtube');
$categories = $youtube->videoCategories->listVideoCategories('snippet', ['regionCode' => 'MA']);
dump($categories);
}
As before, the first parameter is the part
, and I used my country code as a filter. The result looks like the following.
和以前一样,第一个参数是part
,我使用了国家代码作为过滤器。 结果如下所示。
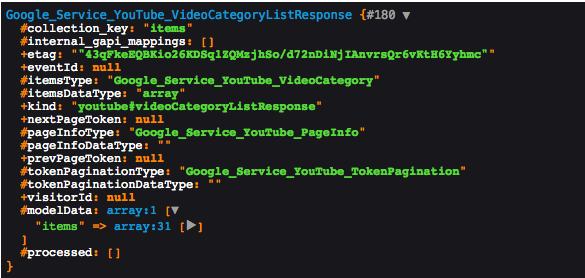
Back to our demo, we need to use the list of categories and give the user the ability to filter the list of videos.
回到我们的演示,我们需要使用类别列表,并使用户能够过滤视频列表。
// app/Http/Controllers/YouTubeAPIController.php
public function videos()
{
$options = ['chart' => 'mostPopular', 'maxResults' => 16];
$regionCode = 'MA';
$selectedCategory = 0;
if (\Input::has('page')) {
$options['pageToken'] = \Input::get('page');
}
if (\Input::has('category')) {
$selectedCategory = \Input::get('category');
$options['videoCategoryId'] = $selectedCategory;
}
$youtube = \App::make('youtube');
$categories = $youtube->videoCategories->listVideoCategories('snippet', ['regionCode' => $regionCode])->getItems();
$videos = $youtube->videos->listVideos('id, snippet', $options);
return view('videos', ['videos' => $videos, 'categories' => $categories, 'selectedCategory' => $selectedCategory]);
}
When the user selects a category, we pass the category ID as a parameter to the videos method. This should work fine with the pagination, so let’s see our view markup changes.
当用户选择类别时,我们会将类别ID作为参数传递给videos方法。 这在分页中应该可以正常工作,所以让我们看一下我们的视图标记更改。
// resources/views/videos.blade.php
{!! Form::open(['route' => 'videos', 'name' => 'formCategory', 'class' => 'form-inline']) !!}
<label for="category">Filter by category: </label>
<select name="category" id="category" class="form-control" onchange="this.form.submit()">
<option value="0">All</option>
@foreach($categories as $category)
<option value="{{ $category->getId() }}" @if($selectedCategory == $category->getId()) selected @endif>{{ $category['snippet']['title'] }}</option>
@endforeach
</select>
{!! Form::close() !!}
<!-- Pagination -->
<li @if($videos->getPrevPageToken() == null) class="disabled" @endif>
<a href="/videos?page={{$videos->getPrevPageToken()}}&category={{ $selectedCategory }}" aria-label="Previous">
<span aria-hidden="true">Previous «</span>
</a>
</li>
<li @if($videos->getNextPageToken() == null) class="disabled" @endif>
<a href="/videos?page={{$videos->getNextPageToken()}}&category={{ $selectedCategory }}" aria-label="Next">
<span aria-hidden="true">Next »</span>
</a>
</li>
The form is submitted on change event of the list of categories, and we make sure to pass the selected category along with the page token on the navigation. The zero category resets our filter.
该表单是在类别列表的change事件上提交的,并且我们确保在导航中传递所选类别以及页面标记。 零类别将重置我们的过滤器。
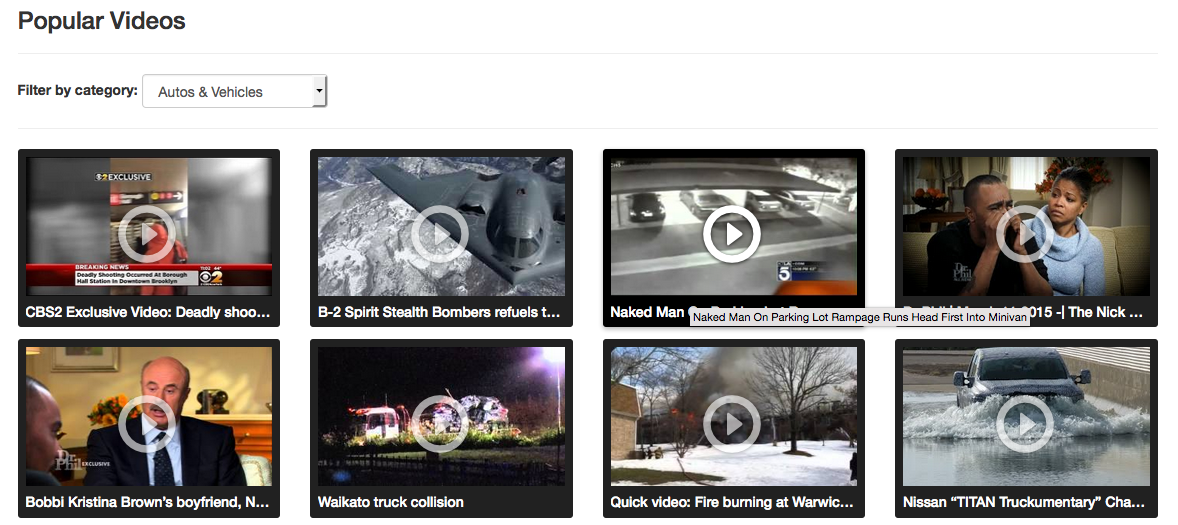
影片搜寻 (Video Search)
Searching is an important aspect of the YouTube platform, and we need to have this functionality in our demo. The user can search and paginate through the list of results.
搜索是YouTube平台的重要方面,我们需要在演示中使用此功能。 用户可以搜索结果列表并进行分页。
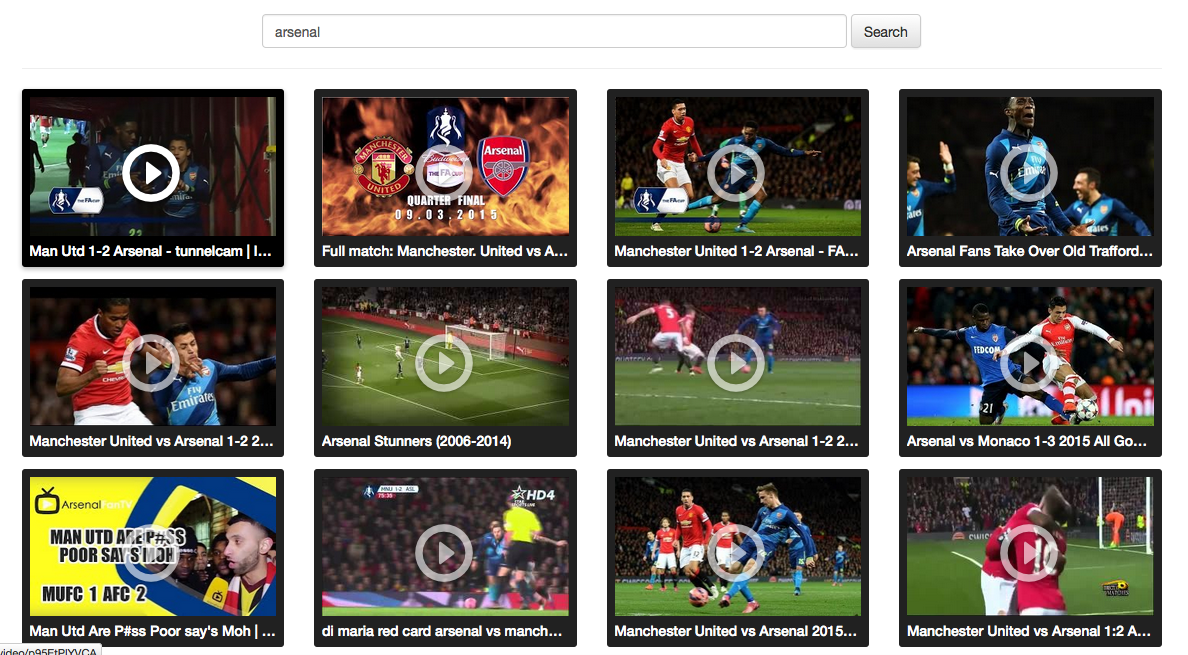
// app/Http/routes.php
Route::any('/search', ['as' => 'search', 'uses' => 'YouTubeAPIController@search']);
// app/Http/Controllers/YouTubeAPIController.php
public function search()
{
if (!\Input::has('query')) {
return view("search");
}
$options = ['maxResults' => 16, 'q' => \Input::get("query")];
if (\Input::has('page')) {
$options['pageToken'] = \Input::get('page');
}
$youtube = \App::make('youtube');
$videos = $youtube->search->listSearch("snippet", $options);
return view("search", ['videos' => $videos, 'query' => \Input::get('query')]);
}
If the query
parameter is not present, that means we need just to render an empty page with the search input, otherwise, we add the query parameter to the list of options and we see if we have a pagination parameter. The view is almost identical to the videos one.
如果query
参数不存在,则意味着我们只需要使用搜索输入来呈现一个空白页,否则,我们将查询参数添加到选项列表中,然后查看是否有分页参数。 视图几乎与视频相同。
// resources/views/search.blade.php
{!! Form::open(['route' => 'search', 'class' => 'form-inline']) !!}
<input type="text" name="query" class="form-control" style="width: 50%;" autofocus="true" value="{{ $query }}"/>
<input type="submit" class="btn btn-default" value="Search"/>
{!! Form::close() !!}
@if(isset($videos))
<ul class="list-unstyled video-list-thumbs row">
@foreach($videos as $video)
<li class="col-lg-3 col-sm-4 col-xs-6">
<a href="{{ URL::route('video', ['id' => $video->getId()->getVideoId()]) }}" title="{{ $video['snippet']['title'] }}" target="_blank">
<img src="{{ $video['snippet']['thumbnails']['medium']['url'] }}" alt="{{ $video['snippet']['title'] }}" class="img-responsive" height="130px" />
<h2 class="truncate">{{ $video['snippet']['title'] }}</h2>
<span class="glyphicon glyphicon-play-circle"></span>
</a>
</li>
@endforeach
</ul>
<nav class="text-center">
<ul class="pagination pagination-lg">
<li @if($videos->getPrevPageToken() == null) class="disabled" @endif>
<a href="/search?page={{$videos->getPrevPageToken()}}&query={{ $query }}" aria-label="Previous">
<span aria-hidden="true">Previous «</span>
</a>
</li>
<li @if($videos->getNextPageToken() == null) class="disabled" @endif>
<a href="/search?page={{$videos->getNextPageToken()}}&query={{ $query }}" aria-label="Next">
<span aria-hidden="true">Next »</span>
</a>
</li>
</ul>
</nav>
@else
<h2>No result.</h2>
@endif
You see that the videos result is identical to the video page, except that the $video->getId()
method returns a resource, and you need to get the video ID using a chained call. YouTube API results have array access, so you can work with them as arrays instead of calling getter methods. The response also contains information about the video channel, creation date, quality, etc. You may use them to enrich your page.
您会看到视频结果与视频页面相同,只是$video->getId()
方法返回资源,并且您需要使用链接调用来获取视频ID。 YouTube API结果具有数组访问权限,因此您可以将它们作为数组使用,而不用调用getter方法。 该响应还包含有关视频频道,创建日期,质量等的信息。您可以使用它们来丰富页面。
When you visit a single video page on YouTube, you see a list of suggestions based on your current video. The search endpoint offers this possibility through some options:
当您访问YouTube上的单个视频页面时,您会看到基于当前视频的建议列表。 搜索端点通过一些选项提供了这种可能性:
// app/Http/Controllers/YouTubeAPIController.php
public function video($id)
{
$options = ['maxResults' => 1, 'id' => $id];
$youtube = \App::make('youtube');
$videos = $youtube->videos->listVideos('id, snippet, player, contentDetails, statistics, status', $options);
$relatedVideos = $youtube->search->listSearch("snippet", ['maxResults' => 16, 'type' => 'video', 'relatedToVideoId' => $id]);
if (count($videos->getItems()) == 0) {
return redirect('404');
}
return view('video', ['video' => $videos[0], 'relatedVideos' => $relatedVideos]);
}
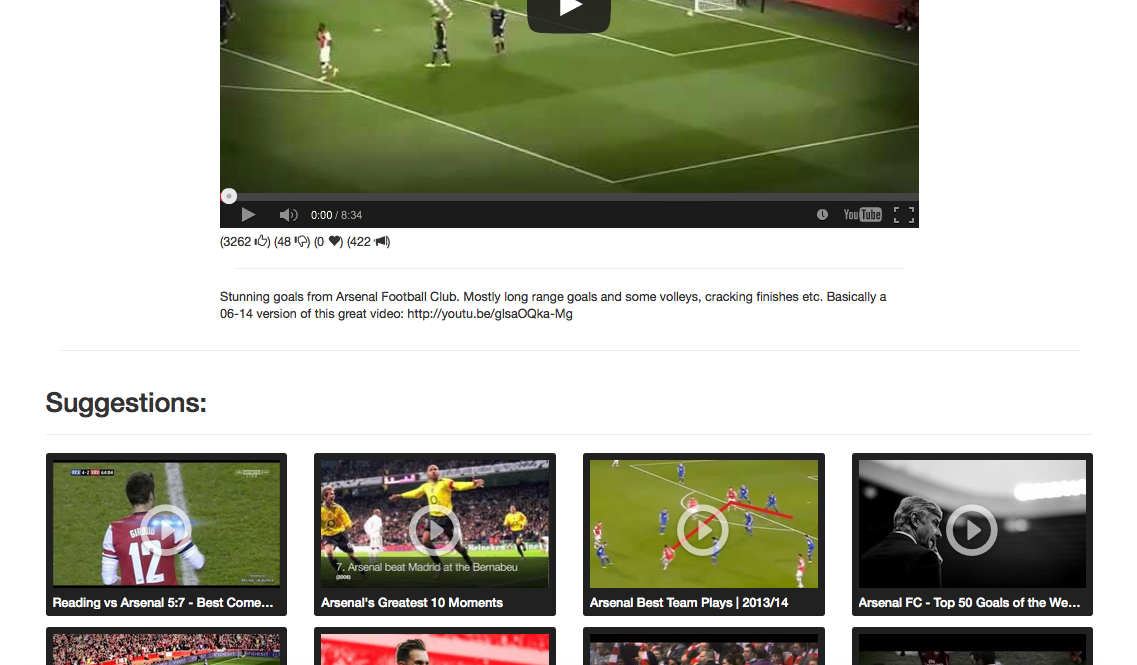
According to the documentation, you need to set the type
parameter to video
when you want to do a related video search. The search endpoint has a lot of options like restricting the search to a certain quality, a certain category or a certain channel, etc. Be sure to check the documentation for the list of available options.
根据文档 ,要进行相关的视频搜索时,需要将type
参数设置为video
。 搜索端点有很多选项,例如将搜索限制为某种质量,某种类别或某种渠道等。请务必查看文档以获取可用选项列表。
扩展演示 (Extending the Demo)
Because we can’t cover everything in this tutorial, you can take the demo from here and start extending it with some new functionality. You can start by displaying video channel names and links under every video element, and when the user clicks on the link, redirect them to the /channel/channel_id
route and only display the channel videos; you can group the video by playlists, etc.
因为我们不能涵盖本教程中的所有内容,所以您可以从此处开始进行演示,并开始使用一些新功能对其进行扩展。 您可以从显示每个视频元素下方的视频频道名称和链接开始,然后当用户单击链接时,将其重定向到/channel/channel_id
路由,仅显示频道视频。 您可以按播放列表等对视频进行分组
配额和缓存 (Quotas & Caching)
An important part of working with APIs are quotas. YouTube gives you 50,000,000 units/day, and as we said earlier, units are calculated per request and can be increased when you ask for more information. Some API calls cost more than others. For example, calls to the search endpoint cost 100 units. Apart from that, you are limited to 3000 requests/second/user.
使用API的重要部分是配额。 YouTube每天为您提供50,000,000个单位,正如我们之前所说,单位是根据请求计算的,可以在您要求更多信息时增加。 一些API调用比其他API花费更多。 例如,调用搜索端点的成本为100个单位。 除此之外,您最多只能将3000个请求/秒/用户。
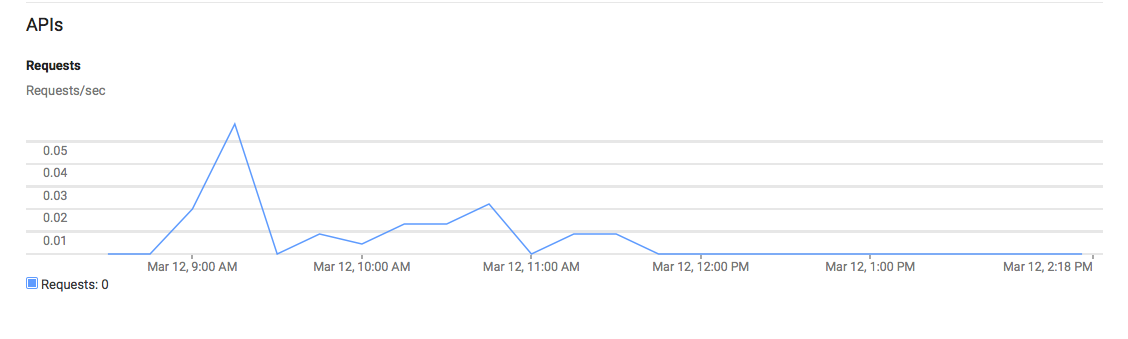
Etags缓存 (Etags Caching)
If you’ve noticed in the previous screenshots, we have an Etag attribute which stamps the current state of the entity result. This attribute can be sent inside the HTTP headers as described on the HTTP spec page and if the entity has changed you’ll get a refreshed version, otherwise you get a 304 (not modified) response. You can read more about optimization and performance in the documentation.
如果您在之前的屏幕截图中已经注意到,我们有一个Etag属性,用于标记实体结果的当前状态。 可以按照HTTP规范页面上的说明在HTTP标头内发送此属性,如果实体已更改,您将获得刷新的版本,否则,您将获得304(未修改)响应。 您可以在文档中阅读有关优化和性能的更多信息。
结论 (Conclusion)
In this article, we introduced the YouTube API v3 and built a demo along the way. The API offers a lot of features, like working with user activities, playlists, channels, subscriptions, etc. Be sure to check the getting started guide to familiarize yourself with the limits and possibilities.
在本文中,我们介绍了YouTube API v3,并在此过程中构建了一个演示。 该API提供了许多功能,例如处理用户活动,播放列表,频道,订阅等。请务必查看入门指南,以了解限制和可能性。
You can check the final demo on Github, it includes an installation guide to get you started. If you have any comments or questions, don’t hesitate to post them below and I will do my best to answer them.
您可以在Github上查看最终的演示,其中包括安装指南以帮助您入门。 如果您有任何意见或疑问,请随时在下面发布,我会尽力回答。
翻译自: https://www.sitepoint.com/youtube-videos-php-categories-search-suggestions/