blame git
When you are working with a huge code base, you may discover bugs in your code (or worse, in someone else’s code) that prevent you from proceeding any further in your development. You can checkout to an old commit to see if the bug was present there — but this is often the worst way of doing so. Imagine you have a hundred commits to check — how much time would be wasted?
当您使用庞大的代码库时,您可能会发现代码中的错误(或更糟糕的是,别人的代码中),这些错误会阻止您进一步进行开发。 您可以签出一个旧的提交,看看那里是否存在该错误-但这通常是最糟糕的方式。 想象一下,您要检查一百次提交-浪费了多少时间?
Thankfully, Git has two tools that help you with debugging. We will have a look at both and try to understand their use cases. Let us start by intentionally introducing a bug into our code:
幸运的是,Git有两个工具可以帮助您进行调试。 我们将对两者进行研究,并尝试了解它们的用例。 让我们首先有意将错误引入代码中:
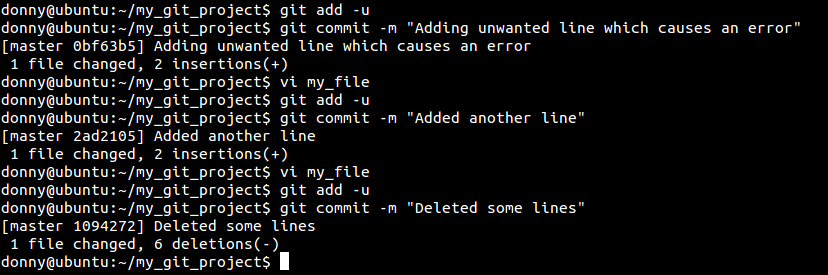
I’ve added a line to the file my_file
that is unwanted and assumed to cause the error. I also add a few commits after that to bury the faulty commit. Let us verify that the faulty line has been added to the file by running the following:
我在文件my_file
中添加了一行,该行是不必要的,并假定会导致该错误。 在此之后,我还添加了一些提交以掩盖错误的提交。 让我们通过运行以下命令来验证是否已将错误行添加到文件中:
cat my_file
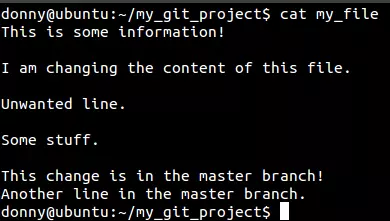
Notice the “Unwanted line” that is supposedly causing the error.
注意可能导致错误的“不必要的行”。
用Blame进行调试 (Debugging with Blame)
Once you have discovered a bug, you may or may not know the location of the faulty code. Let’s say that you do. In our case, let’s say that you know my_file
is causing the trouble. In that case, we can run the following command to get more information about the lines in the files, and the commits those lines belong to.
发现错误后,您可能会或可能不会知道错误代码的位置。 假设您这样做。 在我们的例子中,假设您知道my_file
引起了麻烦。 在这种情况下,我们可以运行以下命令以获取有关文件中的行以及这些行所属的提交的更多信息。
git blame my_file
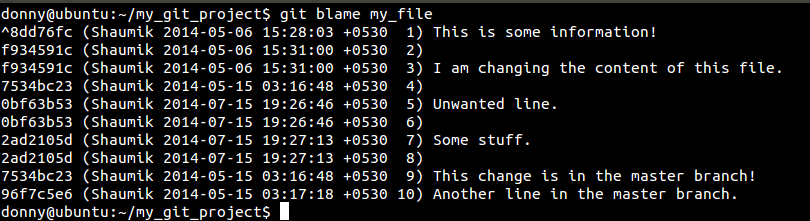
If you look at the output of git blame
, you can see that commit 0bf63b53
is what introduced the bug (“Unwanted line”). If you want to check what else was changed in that commit, or want more information about the same, you can run the following:
如果查看git blame
的输出,则可以看到commit 0bf63b53
是引入该错误的原因(“有害行”)。 如果要检查该提交中的其他更改,或者需要有关该更改的更多信息,可以运行以下命令:
git show 0bf63b53
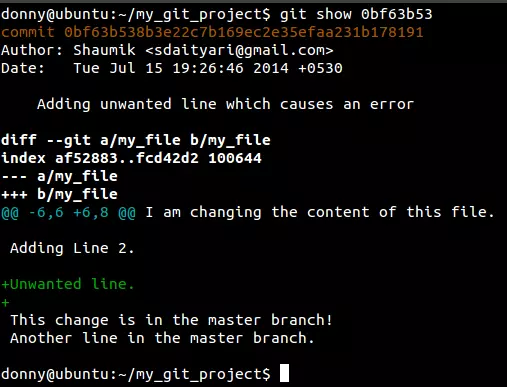
There you go — we now know which commit caused the error and what else was changed in that commit. We can proceed to fixing the error.
到此为止-我们现在知道是哪个提交导致了错误,以及该提交中还有哪些更改。 我们可以继续修复错误。
用Bisect进行调试 (Debugging with Bisect)
git blame
helps you when you have some idea about what is causing the problem. What if you had no idea what is causing the error and there are hundreds of commits before you can go back to a working state? This is where git bisect
comes into play.
当您对导致问题的原因有一些了解时, git blame
会为您提供帮助。 如果您不知道是什么导致了错误,并且有数百次提交,然后又回到工作状态,该怎么办? 这就是git bisect
发挥作用的地方。
I will mention it once again — git bisect
for a trivial situation like our case is overkill. However, I am going through the process for demonstration purposes only.
我会再次提到它git bisect
在一个微不足道的情况下,例如我们的案例是过大的。 但是,我仅出于演示目的而进行该过程。
Imagine git bisect
as a wizard that takes you through your commits to find out which commit brought in the error. It performs a binary search to look through the commits, before arriving at the one that introduced the bug.
想象一下git bisect
作为一个向导,它会带您完成所有提交,以找出导致错误的提交。 它会执行二进制搜索来查找提交,然后才发现引入错误的提交。
To start off, we need to select a “good” commit, where the bug is not present. We then need to select a “bad” commit where the bug was present (ideally the latest commit that contains the bug, so you can assign it as “bad”). Git then walks you through recent commits and asks you whether they are “good” or “bad”, until it finds the culprit. It’s essentially a binary search algorithm over the array of commits to find which commit was the first “bad” commit.
首先,我们需要选择一个“良好的”提交,其中不存在该错误。 然后,我们需要选择一个存在错误的“坏”提交(最好是包含该错误的最新提交,因此您可以将其指定为“坏”)。 然后,Git会引导您完成最近的提交,并询问您是“好”还是“坏”,直到找到罪魁祸首。 本质上,它是对提交数组进行二进制搜索的算法 ,用于查找哪个提交是第一个“不良”提交。
A very important thing to note here is that you should be searching for a single bug in this process. If you have multiple bugs, you need to perform a binary search for each of the bugs.
这里要注意的一个非常重要的事情是,您应该在此过程中寻找一个错误。 如果您有多个错误,则需要对每个错误执行二进制搜索。
Working with the same bug in the case of git blame
, we will assume that we don’t know what file has the error. To check if an error is present in a certain commit, we will run cat my_file
to see if the contents of the file contain the unwanted line.
在git blame
的情况下使用相同的错误,我们将假定我们不知道哪个文件有错误。 要检查某个提交中是否存在错误,我们将运行cat my_file
来查看文件内容是否包含不需要的行。
启动git bisect
向导 (Start the git bisect
Wizard)
We will run the following command to tell Git that we are going into binary search mode to find a bug:
我们将运行以下命令来告诉Git我们将进入二进制搜索模式以查找错误:
git bisect start
选择一个好的承诺 (Select a Good Commit)
After we start the wizard, we need to inform Git about the commit where everything was working. Let’s examine the commit history to find the commit we want.
启动向导后,我们需要向Git通知一切工作正常的提交。 让我们检查提交历史以找到所需的提交。
git log --oneline
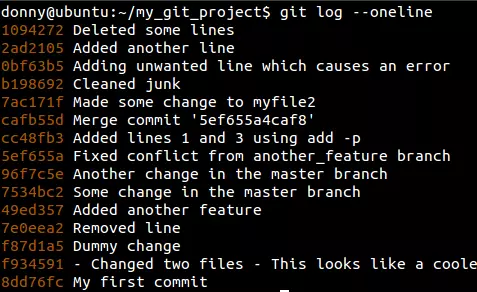
We go with 8dd76fc
, which is the oldest one:
我们使用8dd76fc
,这是最古老的一个:
git bisect good 8dd76fc
选择错误的提交 (Select a Bad Commit)
After we have assigned the “good” tag to a good commit, we need to find a bad commit so that Git can search in between those two and tell us where the bug was introduced. Since we know that the latest commit (1094272
) has the error, we go with that one:
在将“良好”标签分配给良好提交之后,我们需要找到一个错误提交,以便Git可以在这两个之间进行搜索,并告诉我们该错误的引入位置。 由于我们知道最新的提交( 1094272
)存在错误,因此我们继续进行以下操作:
git bisect bad 1094272
将提交分配为“良好”或“不良” (Assign Commits as “Good” or “Bad”)
Once we have assigned our good and bad commits (which serve as the initial and final pointers for our search) Git walks us through the commits and asks us whether each commit contains the bug.
一旦我们分配好提交和坏提交(用作搜索的初始和最终指针),Git就会引导我们完成提交,并询问我们每个提交是否都包含错误。

Notice in the screen shot that 7 revisions would be covered in roughly 3 steps. The number of steps grows logarithmically. Since 22 < 7 < 23, we need three steps. If there were a hundred revisions, we would need roughly 7 steps and if there were a thousand revisions, we would need about 10 steps.
注意,在屏幕截图中,大约3个步骤将涵盖7个修订版本。 步数对数增长。 由于2 2 <7 <2 3 ,我们需要三个步骤。 如果有一百个修订,则大约需要7个步骤,如果有一千个修订,则大约需要10个步骤。
Now we are presented with commit cc48fb
and we need to ascertain if it’s a good or a bad commit. In our case, we check the contents of the file and see if the unwanted line is present:
现在,我们看到了提交cc48fb
,我们需要确定它是好是坏。 在我们的例子中,我们检查文件的内容,看看是否存在不需要的行:
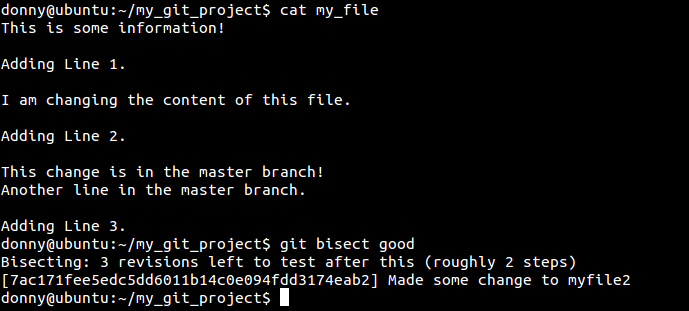
Since the line is not present, we designate it as a good commit.
由于该行不存在,因此我们将其指定为良好提交。
git bisect good
We continue this process for the next few steps until git bisect
finds the first bad commit:
我们继续进行以下步骤,直到git bisect
找到第一个错误的提交为止:
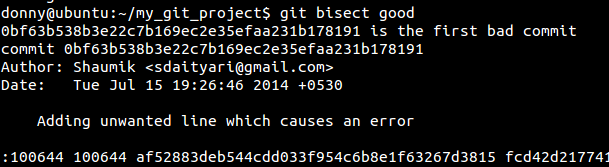
After we are done with the commit, we need to come out of the Git binary search mode:
完成提交后,我们需要退出Git二进制搜索模式:
git bisect reset
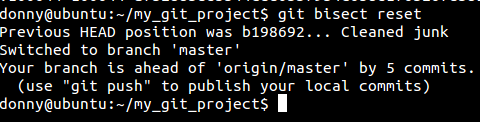
You may want to have a look at this nice screencast on Git Bisect, which takes you through the process that I have discussed.
您可能想看看Git Bisect上的这个不错的截屏视频 ,它带您完成了我所讨论的过程。
流程自动化 (Automating the Process)
We have gone through the process of debugging in Git interactively. If you are familiar with unit testing, you could write a unit test that identifies the bug. In case you want to run the tests automatically, you need to provide Git the test script that you have written.
我们已经交互式地完成了在Git中进行调试的过程。 如果您熟悉单元测试,则可以编写可识别错误的单元测试。 如果要自动运行测试,则需要向Git提供您编写的测试脚本。
git bisect start
git bisect run [location_to_script_file]
Replace location_to_script_file
with the actual location of the script file, removing the square brackets.
用脚本文件的实际位置替换location_to_script_file
,去掉方括号。
Here’s a tutorial on how to mechanize the process of debugging in Git in PHP.
这是有关如何在PHP的Git中机械化调试过程的教程。
结论 (Conclusion)
We took an overly simplified case to explain a very powerful concept. If you know what file has the bad code, you should proceed with git blame
without a second thought. However, if you have no idea what is causing the error, and your repository is considerably large, with an enormous history, git bisect
is definitely the way to go.
我们用一个过于简化的案例来解释一个非常强大的概念。 如果您知道哪个文件的代码错误,则应继续进行git blame
。 但是,如果您不知道是什么导致了错误,并且您的存储库非常大且具有悠久的历史,那么git bisect
绝对是正确的选择。
How do you debug your code? Do you like binary search in Git? Do you have a better way of doing the same thing? Let us know in the comments below.
您如何调试代码? 您喜欢在Git中进行二进制搜索吗? 您有做同一件事的更好方法吗? 在下面的评论中让我们知道。
blame git