php变量
A variable is used in PHP scripts to represent a value. As the name variable suggests, the value of a variable can change (or vary) throughout the program. Variables are one of the features that distinguish a programming language like PHP from markup languages such as HTML.
PHP脚本中使用变量表示值。 顾名思义,变量的值可以在整个程序中变化(或变化)。 变量是区分诸如PHP之类的编程语言与诸如HTML之类的标记语言的功能之一。
Variables allow you write your code in a generic manner. To highlight this, consider a web form which asks users to input their name and favorite color.
变量允许您以通用方式编写代码。 为了突出这一点,请考虑一个网络表单,该表单要求用户输入其名称和喜欢的颜色。
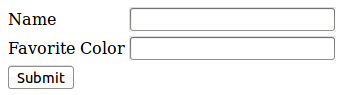
Every time the form is completed, the data will be different. One user may say his name is John and his favorite color is blue. Another may say her name is Susan and her favorite color is yellow. We need a way of working with the values a user enters. The way to do this is by using variables.
每次填写表格时,数据都会不同。 一个用户可能说他的名字是John,而他最喜欢的颜色是蓝色。 另一个人可能会说她的名字叫苏珊,她最喜欢的颜色是黄色。 我们需要一种使用用户输入的值的方法。 做到这一点的方法是使用变量。
There are a few standard variables that PHP creates automatically, but most of the time variables are created (or declared) by you, the programmer. By creating two variables called $name
and $color
, you could create generic code which can handle any input values. For John, this code:
PHP会自动创建一些标准变量,但是大多数情况下,变量是由您(程序员)创建(或声明 )的。 通过创建两个名为$name
和$color
变量,您可以创建可以处理任何输入值的通用代码。 对于约翰来说,这段代码:
<?php
echo "Hello, $name. Your favorite color is $color.";
would display:
将显示:
Hello, John. Your favorite color is blue.
On the other hand, Susan would see:
另一方面,Susan会看到:
Hello, Susan. Your favorite color is yellow.
We will look into variable names and displaying the value of variables in the rest of this article, but the important point now is to understand how the use of generic variables can make processing data easy.
在本文的其余部分中,我们将研究变量名称并显示变量的值,但现在的重点是要了解使用通用变量如何使数据处理变得容易。
创建变量 (Creating Variables)
In PHP, simply writing the name of a variable for the first time in a script will create it. There’s nothing extra you need to do. The variable name has to follow some standard rules, though:
在PHP中,只需在脚本中首次编写变量名即可创建它。 您无需执行任何其他操作。 但是,变量名必须遵循一些标准规则:
The name starts with a
$
sign名称以
$
符号开头The first character after the
$
must be a letter or underscore$
之后的第一个字符必须是字母或下划线- All subsequent characters can be a combination of letters, numbers and underscores 所有后续字符可以是字母,数字和下划线的组合
$customerName
is a valid variable name because it observes all three rules above.
$customerName
是有效的变量名,因为它遵守上述所有三个规则。
$123customer
is not valid because it violates the second rule, the first character after the $
sign must be a letter or underscore.
$123customer
无效,因为它违反了第二条规则, $
符号后的第一个字符必须是字母或下划线。
It’s a good idea to give your variable a meaningful name. If the data you will be storing is a customer’s name, a sensible name might be $customerName
. You could also call it $abc123
, but I hope you agree that the former suggestion is better.
给您的变量起一个有意义的名字是个好主意。 如果您要存储的数据是客户的姓名,那么明智的名称可能是$customerName
。 您也可以将其称为$abc123
,但我希望您同意前者的建议更好。
There are different conventions you can follow when writing variable names. Regardless of what you choose, it is important to be consistent and follow the convention throughout your script. For example, you can use an underscore to separate words (e.g. $customer_name
), or use capital letters to differentiate words, a style called Camel Case (e.g. $customerName
).
编写变量名称时可以遵循不同的约定。 无论您选择什么,在整个脚本中保持一致并遵循约定很重要。 例如,您可以使用下划线来分隔单词(例如$customer_name
),也可以使用大写字母来区分单词(一种称为Camel Case的样式)(例如$customerName
)。
You are allowed to use both upper and lower case letters when naming a variable, but be aware that $CustomerName
is not the same as $customerName
. PHP will treat the two as different variables! This reinforces the need to stick to a naming convention.
命名变量时,可以同时使用大写和小写字母,但是请注意, $CustomerName
与$customerName
。 PHP将把这两个当作不同的变量! 这加强了遵守命名约定的需要。
分配变量 (Assigning Variables)
Now that you know you can have PHP create a new variable anytime you need it just by writing a new name, let’s look at another example to learn about assigning values to them.
既然您已经知道可以在PHP中随时通过编写一个新名称来创建一个新变量,那么让我们看另一个示例,以了解有关为它们分配值的信息。
<?php
$customerName = "Fred";
$customerID;
$customerID = 346646;
$customerName = $customerID;
First, the variable $customerName
is given the value “Fred”. This is referred to as assigning a value. And because this is the first time $customerName
is used, the variable is created automatically. Anytime you write $customerName
after that, PHP will know to use the value “Fred” instead.
首先,变量$customerName
被赋予值“ Fred”。 这称为分配值。 并且由于这是第一次使用$customerName
,因此将自动创建变量。 此后任何时候编写$customerName
,PHP就会知道使用值“ Fred”来代替。
Then, $customerID
is written. A variable can be created without assigning a value to it, though this is generally not considered to be good practice. It is better to assign a default value just so you know it has a value. Remember, variables are variable, so you can always change the value later. Afterwards, the variable $customerID
is assigned the value 346646.
然后,写入$customerID
。 可以创建一个变量而不给它赋值,尽管通常认为这不是一个好习惯。 最好分配一个默认值,以便您知道它具有一个值。 请记住,变量是可变的,因此您以后随时可以更改值。 之后,变量$customerID
被分配值为346646。
Finally, the value of $customerID
is assigned to $customerName
. You can assign the value of one variable to another; in this case the value of $customerID
(346646) overwrites the value in $customerName
(“Fred”) so that both variables now represent 346646!
最后,将$customerID
的值分配给$customerName
。 您可以将一个变量的值分配给另一个。 在这种情况下, $customerID
(346646)的值将覆盖$customerName
(“ Fred”)中的值,以便两个变量现在都代表346646!
Notice how the types of data referenced by a variable have different “types.” This property is called the data’s data type. “Fred” is given with quotation marks, so it is a string (string is just a fancy name for text). 346646 is obviously a number (more specifically, an integer).
请注意,变量引用的数据类型如何具有不同的“类型”。 此属性称为数据的数据类型 。 “ Fred”用引号引起来,因此它是一个字符串 (字符串只是文本的奇特名称)。 346646显然是一个数字(更具体地说是一个整数 )。
Here are some examples of assigning values with different data types:
以下是一些使用不同数据类型分配值的示例:
<?php
$total = 0; // Integer
$total = "Year to Date"; // String
$total = true; // Boolean
$total = 100.25; // Double
$total = array(250, 300, 325, 475); // Array
Now that you understand the basics of naming variables and assigning values, let’s look at this example and see if you can work out the answer:
现在您已经了解了命名变量和分配值的基础,让我们看一下这个示例,看看是否可以得出答案:
<?php
$firstNumber = 4;
$secondNumber = 6;
$result = $firstNumber + $secondNumber;
The examples in the previous section showed that values to the right of the =
sign are assigned to the variable name on the left of the =
sign, so the value 4 is assigned to $firstNumber
.
上一节中的示例显示,将=
符号右侧的值分配给=
符号左侧的变量名称,因此将值4分配给$firstNumber
。
Have a close look at the last line. Though I haven’t explained it previously, the +
sign is an operator, in this case performing addition. So what do you think the value will be in $result
?
仔细看看最后一行。 尽管我之前没有解释,但是+
号是一个运算符,在这种情况下执行加法。 那么您认为$result
的价值是多少?
If your answer is 10 then well done, that’s correct! If not, have a look at the example again and read the explanation carefully.
如果您的答案是10,那么做得好,那是正确的! 如果不是,请再次查看示例并仔细阅读说明。
显示变量的值 (Displaying the Value of Variables)
As you saw at the beginning, you can display the value represented by a variable using echo
. You can also use print
if you’d like, as there is little difference between the two at this point besides less typing with echo
.
如开始时所看到的,您可以使用echo
显示变量代表的值。 如果愿意,您还可以使用print
,因为这两者之间除了几乎没有用echo
键入之外,几乎没有什么区别。
<?php
echo $customerName;
Perhaps you would like to make the example more meaningful by adding some text in quotation marks before the variable contents:
也许您想通过在变量内容之前在引号中添加一些文本来使示例更有意义:
<?php
echo "Customer name = " . $customerName;
The dot between the text in quotation marks and the variable name is the concatenation operator. It joins the string and the value of the variable together.
引号中的文本与变量名之间的点是连接运算符。 它将字符串和变量的值连接在一起。
You could avoid using concatenation and make use of interpolation instead. Interpolation is when the variable name appears in a string and is replaced by its value instead. Taking advantage of this can sometimes make your code easier to read.
您可以避免使用串联,而改为使用插值 。 插值是指变量名出现在字符串中,并用其值代替。 利用此优势有时会使您的代码更易于阅读。
<?php
echo "Customer name = $customerName";
PHP automatically performs interpolation on strings that are enclosed by double-quotation marks. If you wish to display the name of the variable with your text, you can use a backslash immediately before the variable name:
PHP自动对用双引号引起来的字符串执行插值。 如果希望在文本中显示变量的名称,则可以在变量名称之前使用反斜杠:
<?php
echo "$customerName has the value: $customerName";
Alternatively, PHP will not perform interpolation on strings that are enclosed by single-quotation marks. So this is an equally effective statement:
另外,PHP不会对用单引号引起来的字符串执行插值。 因此,这是同样有效的声明:
<?php
echo '$customerName has the value: ' . $customerName;
For more information about variables, check out the PHP documentation. You’ll review everything you’ve learned here, and learn about what special variables PHP will automatically define and make available to your scripts, how a variable is bound to the context in which it was declared, and even how variables can be used as the names for other variables!
有关变量的更多信息,请查看PHP文档 。 您将在此处查看所学内容,并了解PHP将自动定义哪些特殊变量并将其提供给脚本,如何将变量绑定到声明该变量的上下文中,以及如何将变量用作变量。其他变量的名称!
Image via Kachan Eduard / Shutterstock
图片来自Kachan Eduard / Shutterstock
php变量