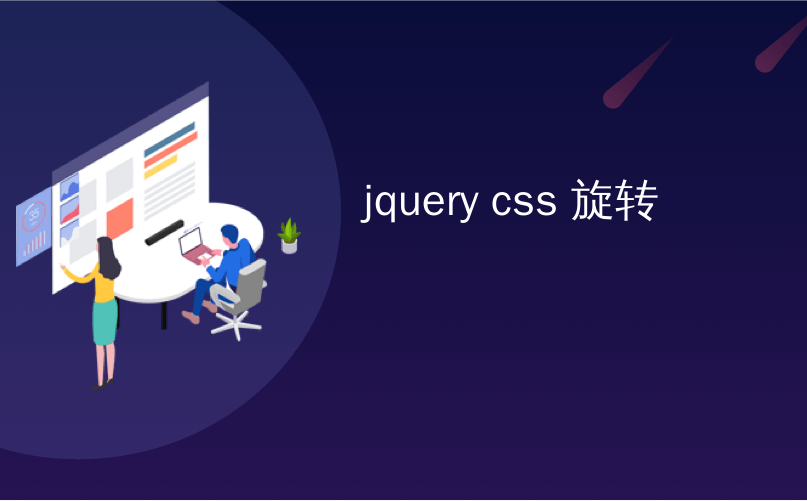
jquery css 旋转
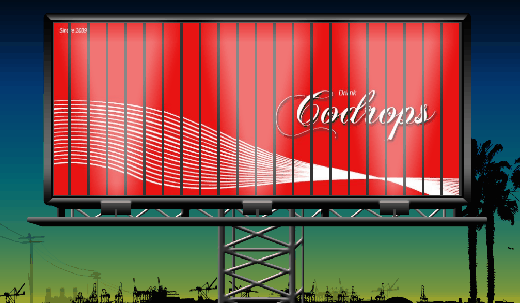
Currently we are in the “hey, let’s do that flash thing in jQuery”-mood and so we came up with another idea: a rotating billboard system.
目前,我们处于“嘿,让我们用jQuery来做那种闪光的东西”的心情,所以我们想到了另一个想法:旋转广告牌系统。
In this tutorial we will use some images, CSS and jQuery to create the effect of a rotating billboard with two ads. The idea is to make one set of image slices disappear while another one (the other ad) appear. We will decrease the width of each disappearing slice and increase the width of each appearing slice. This will give the effect of rotating slices, just like in a rotating billboard system.
在本教程中,我们将使用一些图像,CSS和jQuery来创建带有两个广告的旋转广告牌的效果。 这样做的目的是使一组图像切片消失而另一组图像(另一个广告)出现。 我们将减小每个消失的切片的宽度,并增加每个出现的切片的宽度。 就像在旋转广告牌系统中一样,这将产生旋转切片的效果。
Ok, let’s start coding!
好的,让我们开始编码!
1. HTML (1. The HTML)
We will have quite a lot of markup for the image slices – it will be 22 slices for each ad:
我们将对图像切片进行大量标记-每个广告将包含22个切片:
<div class="container">
<div class="ad">
<div id="ad_1" class="ad_1">
<img class="slice_1" src="ads/ad1_slice01.jpg"/>
<img class="slice_2" src="ads/ad1_slice02.jpg"/>
<img class="slice_3" src="ads/ad1_slice03.jpg"/>
<img class="slice_4" src="ads/ad1_slice04.jpg"/>
<img class="slice_5" src="ads/ad1_slice05.jpg"/>
<img class="slice_6" src="ads/ad1_slice06.jpg"/>
<img class="slice_7" src="ads/ad1_slice07.jpg"/>
<img class="slice_8" src="ads/ad1_slice08.jpg"/>
<img class="slice_9" src="ads/ad1_slice09.jpg"/>
<img class="slice_10" src="ads/ad1_slice10.jpg"/>
<img class="slice_11" src="ads/ad1_slice11.jpg"/>
<img class="slice_12" src="ads/ad1_slice12.jpg"/>
<img class="slice_13" src="ads/ad1_slice13.jpg"/>
<img class="slice_14" src="ads/ad1_slice14.jpg"/>
<img class="slice_15" src="ads/ad1_slice15.jpg"/>
<img class="slice_16" src="ads/ad1_slice16.jpg"/>
<img class="slice_17" src="ads/ad1_slice17.jpg"/>
<img class="slice_18" src="ads/ad1_slice18.jpg"/>
<img class="slice_19" src="ads/ad1_slice19.jpg"/>
<img class="slice_20" src="ads/ad1_slice20.jpg"/>
<img class="slice_21" src="ads/ad1_slice21.jpg"/>
<img class="slice_22" src="ads/ad1_slice22.jpg"/>
</div>
<div id="ad_2" class="ad_2">
<img class="slice_1" src="ads/ad2_slice01.jpg"/>
<img class="slice_2" src="ads/ad2_slice02.jpg"/>
<img class="slice_3" src="ads/ad2_slice03.jpg"/>
<img class="slice_4" src="ads/ad2_slice04.jpg"/>
<img class="slice_5" src="ads/ad2_slice05.jpg"/>
<img class="slice_6" src="ads/ad2_slice06.jpg"/>
<img class="slice_7" src="ads/ad2_slice07.jpg"/>
<img class="slice_8" src="ads/ad2_slice08.jpg"/>
<img class="slice_9" src="ads/ad2_slice09.jpg"/>
<img class="slice_10" src="ads/ad2_slice10.jpg"/>
<img class="slice_11" src="ads/ad2_slice11.jpg"/>
<img class="slice_12" src="ads/ad2_slice12.jpg"/>
<img class="slice_13" src="ads/ad2_slice13.jpg"/>
<img class="slice_14" src="ads/ad2_slice14.jpg"/>
<img class="slice_15" src="ads/ad2_slice15.jpg"/>
<img class="slice_16" src="ads/ad2_slice16.jpg"/>
<img class="slice_17" src="ads/ad2_slice17.jpg"/>
<img class="slice_18" src="ads/ad2_slice18.jpg"/>
<img class="slice_19" src="ads/ad2_slice19.jpg"/>
<img class="slice_20" src="ads/ad2_slice20.jpg"/>
<img class="slice_21" src="ads/ad2_slice21.jpg"/>
<img class="slice_22" src="ads/ad2_slice22.jpg"/>
</div>
</div>
</div>
<div class="billboard"></div>
For these images, 22 slices (each slice 35 pixels wide) of a 770 pixel wide and 340 pixel high image were made. One might think that this is a lot of work and that we could actually take one whole picture and create divs acting like the slices with that background image and the right background position. But then we would not be able to create the same effect of a rotating slice (at least not with the JavaScript we created for this showcase).
对于这些图像,制作了770像素宽和340像素高图像的22个切片(每个切片35像素宽)。 有人可能会认为这是一项繁重的工作,实际上我们可以拍摄一张完整的照片,然后创建具有像带有背景图像和正确背景位置的切片的div的div。 但是,那么我们将无法创建与旋转切片相同的效果(至少不能使用我们为此展示创建JavaScript)。
The billboard will be an absolute positioned div with the billboard image. Since the image has some transparent spotlights, we want to lay it over the ad container.
广告牌将是带有广告牌图片的绝对定位的div。 由于图像具有一些透明的聚光灯,因此我们希望将其放置在广告容器上方。
2. CSS (2. The CSS)
The style for the billboard frame will be the following:
广告牌框架的样式如下:
.billboard{
position:absolute;
bottom:0px;
left:50%;
margin-left:-447px;
width:916px;
height:599px;
background:transparent url(../images/billboard.png) no-repeat 0px 0px;
}
To position the element in the center of the page, we set the left value to 50% and the left margin negatively to half of the width of the element.
为了将元素放置在页面的中心,我们将左边的值设置为50%,将左边距设置为负值,宽度为元素宽度的一半。
The container for the ads will have the same style like the billboard, except the background-image. We do that, because we need to position the containing elements at the same place like the billboard. We don’t want to place the ads inside of the billboard because we need the billboard to be on top of them. So, we do this trick and create another element with the same positioning:
除了背景图片之外,广告的容器将具有与广告牌相同的样式。 我们这样做是因为我们需要将包含元素的位置放置在广告牌一样的位置。 我们不想将广告放置在广告牌内,因为我们需要广告牌在广告牌的顶部。 因此,我们执行此技巧并创建具有相同位置的另一个元素:
.container{
position:absolute;
bottom:0px;
left:50%;
margin-left:-447px;
width:916px;
height:599px;
}
.ad{
width:800px;
height:336px;
position:relative;
margin:35px 0px 0px 60px;
background-color:#333;
}
.ad_1 img{
width:35px;
height:336px;
position:absolute;
}
.ad_2 img{
width:0px;
height:336px;
margin-left:18px;
position:absolute;
}
The slices of the ad images will be 35 pixels wide. The slices of the second ad will have the same width, but initially we need to set it to 0. We also need to set the left margin of the second slices to 18 pixels since we want to create the rotating effect. I will explain more about this value after we define the CSS for the slices.
广告图像的切片将为35像素宽。 第二个广告的片段将具有相同的宽度,但首先我们需要将其设置为0。由于要创建旋转效果,我们还需要将第二个片段的左边界设置为18像素。 在为切片定义CSS之后,我将解释有关此值的更多信息。
The single slices need to be positioned:
单个切片需要放置:
.slice_1{left:0px;}
.slice_2{left:36px;}
.slice_3{left:72px;}
.slice_4{left:108px;}
.slice_5{left:144px;}
.slice_6{left:180px;}
.slice_7{left:216px;}
.slice_8{left:252px;}
.slice_9{left:288px;}
.slice_10{left:324px;}
.slice_11{left:360px;}
.slice_12{left:396px;}
.slice_13{left:432px;}
.slice_14{left:468px;}
.slice_15{left:504px;}
.slice_16{left:540px;}
.slice_17{left:576px;}
.slice_18{left:612px;}
.slice_19{left:648px;}
.slice_20{left:684px;}
.slice_21{left:720px;}
.slice_22{left:756px;}
By positioning the elements one more pixel to the left than they are actually wide, we create a little gap between the them. Now, the left margin has a value of 18 pixels because it is half of a slice plus its gap. We set this because we want the slices to appear (or disappear) from (or to) their center and not just from the side. If we simply set the width of a slice to 0 pixels the image will not seem to be rotating.
通过将元素的位置比实际宽度增加一个像素,我们在它们之间创建了一个小间隙。 现在,左边距的值为18像素,因为它是切片的一半加上其间隙。 设置此值是因为我们希望切片从其中心而不是仅从侧面出现(或消失)。 如果我们仅将切片的宽度设置为0像素,则图像似乎不会旋转。
And that is all the CSS. For the additional fancy background images you can check out the downloadable version below.
这就是所有CSS。 有关其他精美背景图片,您可以在下面查看可下载的版本。
Let’s create the rotating effect with jQuery.
让我们用jQuery创建旋转效果。
3. JavaScript (3. The JavaScript)
We will now create a function for rotating the slices. The function will make the first ad slices disappear by making their width 0 pixels. To achieve the rotating effect, we add a left margin of 18 pixels.
现在,我们将创建一个旋转切片的功能。 该功能将第一个广告片段的宽度设为0像素,从而使其消失。 为了实现旋转效果,我们添加了18个像素的左边距。
While the first ad slices disappear we make the others appear by removing the left margin of 18 pixels (that we initially set in the CSS) and giving them a width of 35 pixels. We call the rotate function like this:
当第一个广告片段消失时,我们通过删除18像素(我们最初在CSS中设置的)的左边距并为其设置35像素的宽度,使其他广告片段出现。 我们这样调用旋转函数:
$('#ad_1 > img').each(function(i,e){
rotate($(this),500,3000,i);
});
The whole script that we call will look like this:
我们调用的整个脚本如下所示:
$(function() {
$('#ad_1 > img').each(function(i,e){
rotate($(this),500,3000,i);
});
function rotate(elem1,speed,timeout,i){
elem1.animate({'marginLeft':'18px','width':'0px'},speed,function(){
var other;
if(elem1.parent().attr('id') == 'ad_1')
other = $('#ad_2').children('img').eq(i);
else
other = $('#ad_1').children('img').eq(i);
other.animate({'marginLeft':'0px','width':'35px'},
speed,function(){
var f = function() { rotate(other,speed,timeout,i) };
setTimeout(f,timeout);
});
});
}
});
So, the rotate function performs the hiding of the current element (that it was called upon) and then identifies which element it’s currently dealing with, so that another call of the rotate function can be performed on the other ad slices. The two times mentioned in the rotate function stand for the speed of the rotation effect (speed) and the duration between the swapping of the ads (timeout) in milliseconds.
因此,rotate函数执行当前元素(被调用)的隐藏,然后标识其当前正在处理的元素,以便可以在其他广告片段上执行对Rotate函数的另一次调用。 旋转功能中提到的两次代表旋转效果的速度(速度)和广告交换之间的持续时间(超时),以毫秒为单位。
And that’s it! I hope you like and enjoy it!
就是这样! 希望您喜欢并喜欢它!
翻译自: https://tympanus.net/codrops/2009/12/16/creating-a-rotating-billboard-system-with-jquery-and-css/
jquery css 旋转