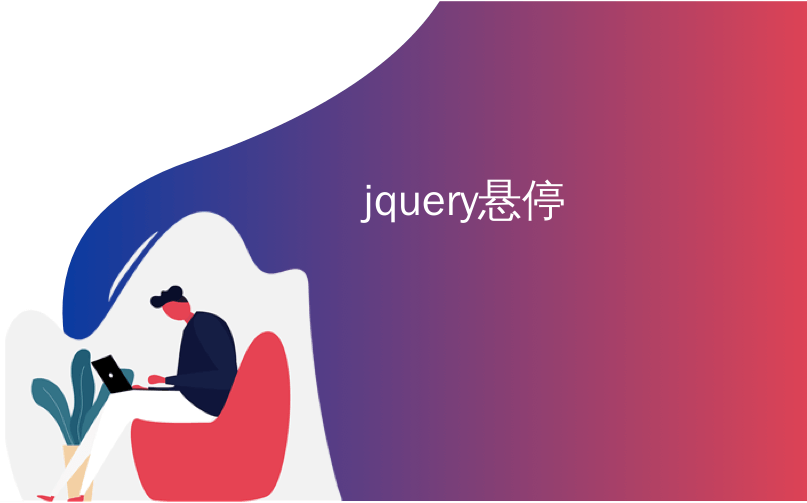
jquery悬停
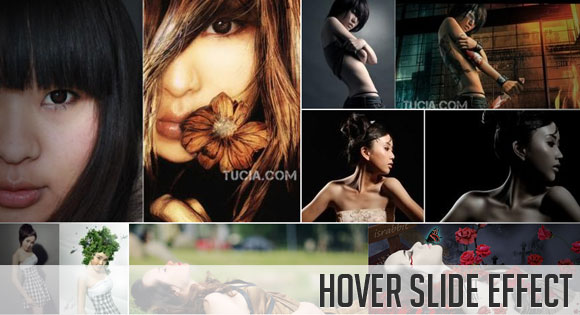
Today we will create a neat effect with some images using jQuery. The main idea is to have an image area with several images that slide out when we hover over them, revealing other images. The sliding effect will be random, i.e. the images will slide to the top or bottom, left or right, fading out or not. When we click on any area, all areas will slide their images out.
今天,我们将使用jQuery创建具有某些图像的整洁效果。 主要思想是要有一个图像区域,其中包含多个图像,当我们将鼠标悬停在这些区域上时,它们会露出其他图像。 滑动效果是随机的,即图像将滑动到顶部或底部,左侧或右侧,是否淡出。 当我们单击任何区域时,所有区域都会将其图像滑出。
The idea is based on the beautiful Flash based animation on the Yumaki website.
这个想法是基于Yumaki网站上基于Flash的漂亮动画。
So, let’s start.
所以,让我们开始吧。
标记 (The Markup)
For the HTML structure we will create a div element with the class and id “hs_container”. Inside we will place the different image areas with all possible images. The first image will have the class “hs_visible” which will make it being displayed on the top of all other images:
对于HTML结构,我们将创建一个类和ID为“ hs_container”的div元素。 在内部,我们将放置具有所有可能图像的不同图像区域。 第一张图片将具有“ hs_visible”类,这将使其显示在所有其他图片的顶部:
<div id="hs_container" class="hs_container">
<div class="hs_area hs_area1">
<img class="hs_visible" src="images/area1/1.jpg" alt=""/>
<img src="images/area1/2.jpg" alt=""/>
<img src="images/area1/3.jpg" alt=""/>
</div>
<div class="hs_area hs_area2">
<img class="hs_visible" src="images/area2/1.jpg" alt=""/>
<img src="images/area2/2.jpg" alt=""/>
<img src="images/area2/3.jpg" alt=""/>
</div>
<div class="hs_area hs_area3">
<img class="hs_visible" src="images/area3/1.jpg" alt=""/>
<img src="images/area3/2.jpg" alt=""/>
<img src="images/area3/3.jpg" alt=""/>
</div>
<div class="hs_area hs_area4">
<img sclass="hs_visible" src="images/area4/1.jpg" alt=""/>
<img src="images/area4/2.jpg" alt=""/>
<img src="images/area4/3.jpg" alt=""/>
</div>
<div class="hs_area hs_area5">
<img class="hs_visible" src="images/area5/1.jpg" alt=""/>
<img src="images/area5/2.jpg" alt=""/>
<img src="images/area5/3.jpg" alt=""/>
</div>
</div>
Let’s take a look at the style.
让我们看一下样式。
CSS (The CSS)
In our stylesheet, we will define all the areas and their dimensions. Since we will make them absolute, we will also define the positions for each area. Let’s start by defining the main container:
在样式表中,我们将定义所有区域及其尺寸。 由于我们将它们设为绝对,因此我们还将定义每个区域的位置。 让我们从定义主容器开始:
.hs_container{
position:relative;
width:902px;
height:471px;
overflow:hidden;
clear:both;
border:2px solid #fff;
cursor:pointer;
-moz-box-shadow:1px 1px 3px #222;
-webkit-box-shadow:1px 1px 3px #222;
box-shadow:1px 1px 3px #222;
}
It’s important that we define the overflow as hidden, since we don’t want the sliding images to be shown when they are out of this container.
重要的是,将溢出定义为隐藏,因为我们不希望滑动图像超出此容器时显示它们。
Each area will also have its overflow hidden and be of position absolute:
每个区域也将隐藏其溢出并处于绝对位置:
.hs_container .hs_area{
position:absolute;
overflow:hidden;
}
We position the images inside of the area and make them invisible:
我们将图像放置在该区域内并使它们不可见:
.hs_area img{
position:absolute;
top:0px;
left:0px;
display:none;
}
The first image will be visible, so we give it the following class:
第一张图片将可见,因此我们为它提供了以下类别:
.hs_area img.hs_visible{
display:block;
z-index:9999;
}
And now, we will define the borders and positions of each area:
现在,我们将定义每个区域的边界和位置:
.hs_area1{
border-right:2px solid #fff;
}
.hs_area4, .hs_area5{
border-top:2px solid #fff;
}
.hs_area4{
border-right:2px solid #fff;
}
.hs_area3{
border-top:2px solid #fff;
}
.hs_area1{
width:449px;
height:334px;
top:0px;
left:0px;
}
.hs_area2{
width:451px;
height:165px;
top:0px;
left:451px;
}
.hs_area3{
width:451px;
height:167px;
top:165px;
left:451px;
}
.hs_area4{
width:192px;
height:135px;
top:334px;
left:0px;
}
.hs_area5{
width:708px;
height:135px;
top:334px;
left:194px;
}
And that’s all the style! Let’s take a look at the JavaScript.
这就是所有样式! 让我们看一下JavaScript。
JavaScript (The JavaScript)
For the effects, we will be giving the option to use some easing, so don’t forget to include the jQuery Easing Plugin if you make use of that option. So, let’s first define some variables:
对于效果,我们将提供使用一些缓动的选项,因此如果您使用该选项,请不要忘记包括jQuery Easing Plugin 。 因此,让我们首先定义一些变量:
//custom animations to use
//in the transitions
var animations = ['right','left','top','bottom','rightFade','leftFade','topFade','bottomFade'];
var total_anim = animations.length;
//just change this one to one of your choice
var easeType = 'swing';
//the speed of each transition
var animSpeed = 450;
//caching
var $hs_container = $('#hs_container');
var $hs_areas = $hs_container.find('.hs_area');
When we move the mouse over one of the areas we will animate the current image with an animation from our array, so that the next image gets visible. We will use a flag “over” to know if we can animate a certain area since we don’t want two animations to happen at the same time in one area.
当我们将鼠标移到其中一个区域上时,我们将使用数组中的动画为当前图像设置动画,以便可以看到下一个图像。 因为我们不希望在一个区域中同时发生两个动画,所以我们将使用“ over”标记来知道是否可以对某个区域进行动画处理。
We will have a case for each animation and we will define how the animation will behave.
每个动画都有一个案例,我们将定义动画的行为方式。
//first preload all images
$hs_images = $hs_container.find('img');
var total_images = $hs_images.length;
var cnt = 0;
$hs_images.each(function(){
var $this = $(this);
$('
').load(function(){
++cnt;
if(cnt == total_images){
$hs_areas.each(function(){
var $area = $(this);
//when the mouse enters the area we animate the current
//image (random animation from array animations),
//so that the next one gets visible.
//"over" is a flag indicating if we can animate
//an area or not (we don't want 2 animations
//at the same time for each area)
$area.data('over',true).bind('mouseenter',function(){
if($area.data('over')){
$area.data('over',false);
//how many images in this area?
var total = $area.children().length;
//visible image
var $current = $area.find('img:visible');
//index of visible image
var idx_current = $current.index();
//the next image that's going to be displayed.
//either the next one, or the first one if the current is the last
var $next = (idx_current == total-1) ? $area.children(':first') : $current.next();
//show next one (not yet visible)
$next.show();
//get a random animation
var anim = animations[Math.floor(Math.random()*total_anim)];
switch(anim){
//current slides out from the right
case 'right':
$current.animate({
'left':$current.width()+'px'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'left' : '0px'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the left
case 'left':
$current.animate({
'left':-$current.width()+'px'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'left' : '0px'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the top
case 'top':
$current.animate({
'top':-$current.height()+'px'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'top' : '0px'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the bottom
case 'bottom':
$current.animate({
'top':$current.height()+'px'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'top' : '0px'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the right and fades out
case 'rightFade':
$current.animate({
'left':$current.width()+'px',
'opacity':'0'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'left' : '0px',
'opacity' : '1'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the left and fades out
case 'leftFade':
$current.animate({
'left':-$current.width()+'px','opacity':'0'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'left' : '0px',
'opacity' : '1'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the top and fades out
case 'topFade':
$current.animate({
'top':-$current.height()+'px',
'opacity':'0'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'top' : '0px',
'opacity' : '1'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
//current slides out from the bottom and fades out
case 'bottomFade':
$current.animate({
'top':$current.height()+'px',
'opacity':'0'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'top' : '0px',
'opacity' : '1'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
default:
$current.animate({
'left':-$current.width()+'px'
},
animSpeed,
easeType,
function(){
$current.hide().css({
'z-index' : '1',
'left' : '0px'
});
$next.css('z-index','9999');
$area.data('over',true);
});
break;
}
}
});
});
//when clicking the hs_container all areas get slided
//(just for fun...you would probably want to enter the site
//or something similar)
$hs_container.bind('click',function(){
$hs_areas.trigger('mouseenter');
});
}
}).attr('src',$this.attr('src'));
});
And that’s it! We hope you enjoyed the tutorial and find it useful!
就是这样! 我们希望您喜欢本教程并发现它有用!
翻译自: https://tympanus.net/codrops/2010/11/16/hover-slide-effect/
jquery悬停