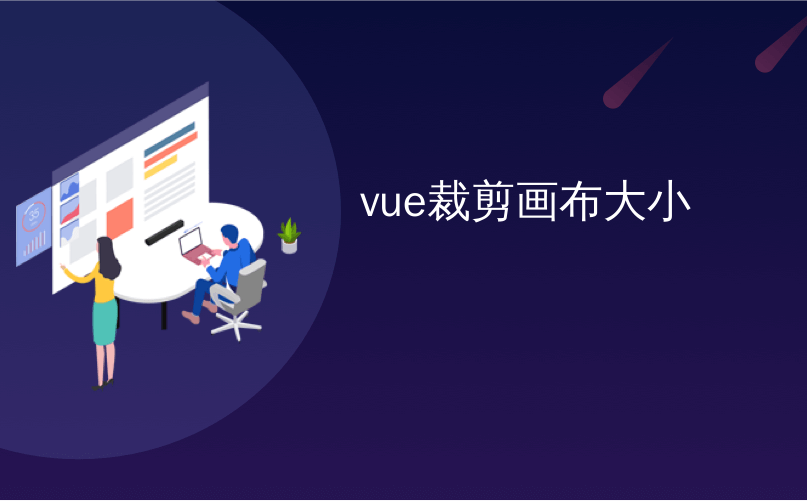
vue裁剪画布大小
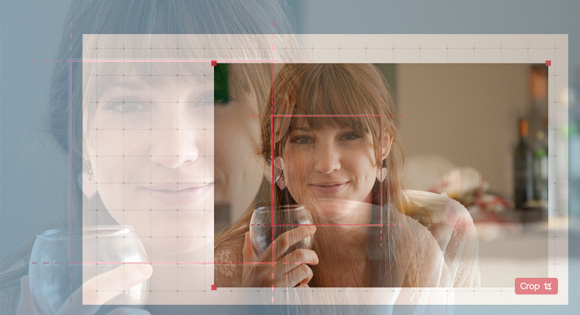
In this tutorial we’re going to learn how to resize and crop an image using the HTML5 <canvas>
element, and while we’re at it, let’s create some fancy controls for resizing, commonly seen in photo editing applications.
在本教程中,我们将学习如何使用HTML5 <canvas>
元素调整图像大小和裁剪图像,而在此过程中,我们将创建一些精美的控件来调整大小,这在照片编辑应用程序中很常见。
In a real world example a website or app might use a technique like this to resize and frame a profile picture before uploading. Whilst we could do this on the server, it would require the transfer of a potentially large file, which is slow. Instead we can resize the image on the client side before uploading it, which is fast.
在真实的示例中,网站或应用可能会使用类似的技术在上传之前调整个人资料图片的大小并对其进行构图。 虽然我们可以在服务器上执行此操作,但它需要传输可能很大的文件,这很慢。 取而代之的是,我们可以在上传之前在客户端上调整图像的大小,这是很快的。
We do this by creating an HTML5 <canvas>
element and drawing the image to the canvas at a particular size, then extracting the new image data from the canvas as a data URI. Most browsers have good support for these methods, so you can probably use this technique right now, however just be aware of some limitations unrelated to browser support such as quality and performance.
为此,我们创建一个HTML5 <canvas>
元素,然后以特定大小将图像绘制到画布上,然后从画布中提取新的图像数据作为数据URI。 大多数浏览器都对这些方法提供了良好的支持,因此您可能现在可以使用此技术,但是请注意与浏览器支持无关的一些限制,例如质量和性能。
Resizing very large images can cause the browser to slow down or in some cases, even crash. It makes sense to set reasonable limits on the file size just as you would when uploading a file. If quality is important you may find the resized image looks undesirable due to how the browser resampled it. There are some techniques to improve the quality of images downscaled with canvas, but they are not covered in this tutorial.
调整非常大的图像大小可能会导致浏览器速度降低,甚至在某些情况下甚至崩溃。 就像上载文件一样,对文件大小设置合理的限制很有意义。 如果质量很重要,则由于浏览器如何对其进行重新采样,可能会发现调整大小后的图像看起来不理想。 有一些技术可以改善使用canvas缩小的图像的质量,但本教程未介绍它们。
Take a look at the final result in this demo or download the ZIP file.
With that in mind, let’s get started!
考虑到这一点,让我们开始吧!
标记 (The Markup)
In our demo we’re going to start with an existing image:
在我们的演示中,我们将从现有图像开始:
<img class="resize-image" src="image.jpg" alt="Image" />
That’s it! That’s all the HTML we need for this demo.
而已! 这就是本演示所需的所有HTML。
CSS (The CSS)
The CSS is also very minimal. First, define the styles for the resize-container and the image.
CSS也非常小。 首先,定义调整大小容器和图像的样式。
.resize-container {
position: relative;
display: inline-block;
cursor: move;
margin: 0 auto;
}
.resize-container img {
display: block
}
.resize-container:hover img,
.resize-container:active img {
outline: 2px dashed rgba(222,60,80,.9);
}
Next, define the position and style for each of the ‘resize handles’. These are the little squares at each corner of the image that we drag to resize.
接下来,为每个“调整大小手柄”定义位置和样式。 这些是我们拖动以调整大小的图像每个角上的小方块。
.resize-handle-ne,
.resize-handle-ne,
.resize-handle-se,
.resize-handle-nw,
.resize-handle-sw {
position: absolute;
display: block;
width: 10px;
height: 10px;
background: rgba(222,60,80,.9);
z-index: 999;
}
.resize-handle-nw {
top: -5px;
left: -5px;
cursor: nw-resize;
}
.resize-handle-sw {
bottom: -5px;
left: -5px;
cursor: sw-resize;
}
.resize-handle-ne {
top: -5px;
right: -5px;
cursor: ne-resize;
}
.resize-handle-se {
bottom: -5px;
right: -5px;
cursor: se-resize;
}
JavaScript(The JavaScript)
With the JavaScript start by defining some of the variables and initializing the Canvas and the target image.
使用JavaScript首先定义一些变量并初始化Canvas和目标图像。
var resizeableImage = function(image_target) {
var $container,
orig_src = new Image(),
image_target = $(image_target).get(0),
event_state = {},
constrain = false,
min_width = 60,
min_height = 60,
max_width = 800,
max_height = 900,
resize_canvas = document.createElement('canvas');
});
resizeableImage($('.resize-image'));
Next, we create the init
function that will be called immediately. This function wraps the image with a container, creates resize handles and makes a copy of the original image used for resizing. We also assign the jQuery object for the container element to a variable so we can refer to it later and add a mousedown
event listener to detect when someone begins dragging one of the handles