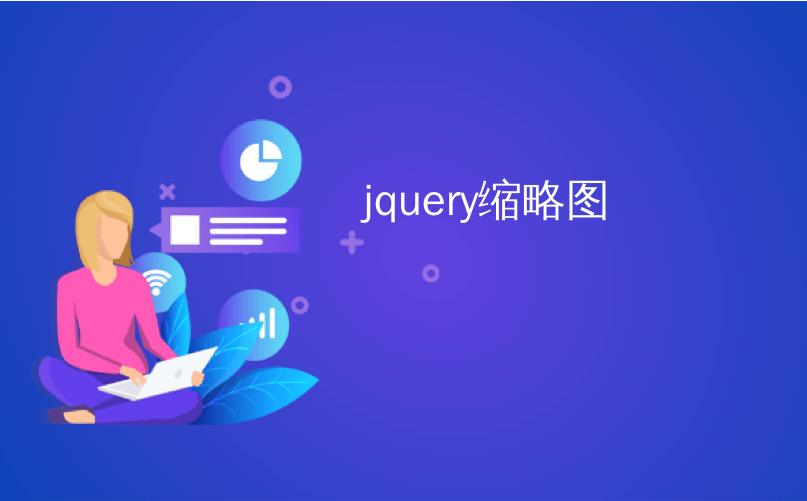
jquery缩略图
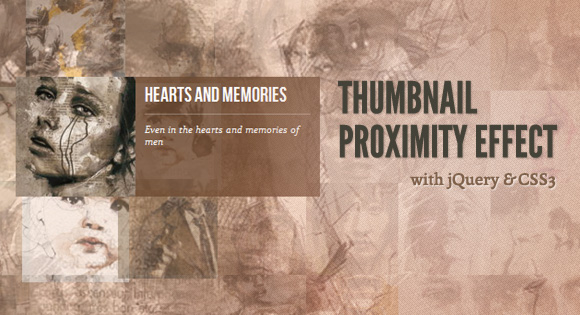
Today we want to show you how to create a neat thumbnail proximity effect with jQuery. The idea is to scale thumbnails when hovering over them and also scale their neighbouring thumbnails proportionally to their distance. We’ll also make a description appear.
今天,我们想向您展示如何使用jQuery创建简洁的缩略图接近效果。 想法是在将鼠标悬停在缩略图上时缩放缩略图,并根据其距离按比例缩放相邻的缩略图。 我们还将显示一个描述。
This is based on a request we once got from a reader who asked if the effect on http://porscheeveryday.com/ could be achieved with jQuery. This is our attempt to recreate the basics of that effect.
这是基于我们曾经从一位读者那里得到的一个请求,该请求者询问使用jQuery是否可以实现http://porscheeveryday.com/上的效果。 这是我们尝试重新创建该效果的基础。
We will be using the jQuery Proximity plugin by James Padolsey.
我们将使用James Padolsey的jQuery Proximity插件。
The illustations in the demos are by Florian Nicolle and they are licensed under the Creative Commons Attribution-NonCommercial 3.0 Unported License.
演示中的插图是Florian Nicolle的作品,它们是根据Creative Commons Attribution-NonCommercial 3.0 Unported License许可的。
标记 (The Markup)
We’ll use an unordered list for the thumbnails and add a division for the description of each image (for this tutorial, we will be going through demo 2):
我们将为缩略图使用无序列表,并为每个图像的描述添加一个划分(对于本教程,我们将进行演示2 ):
<ul id="pe-thumbs" class="pe-thumbs">
<li>
<a href="#">
<img src="images/thumbs/1.jpg" />
<div class="pe-description">
<h3>Time</h3>
<p>Since time, and his predestinated end</p>
</div></a>
</li>
<li><!-- ... --></li>
</ul>
Let’s style it!
让我们来造型吧!
CSS (The CSS)
The unordered list will be centered on the page and we’ll add a background image to “shine through” the thumbnails that will have a low opacity:
无序列表将位于页面的中心,我们将添加背景图像以“照亮”具有低不透明度的缩略图:
.pe-thumbs{
width: 900px;
height: 400px;
margin: 20px auto;
position: relative;
background: transparent url(../images/3.jpg) top center;
border: 5px solid #fff;
box-shadow: 0 1px 2px rgba(0,0,0,0.2);
}
We’ll also add a bit of tint to the background image by adding a pseudo element with a rgba background color:
我们还将通过添加具有rgba背景色的伪元素来为背景图像添加一些色彩:
.pe-thumbs:before {
content: "";
display: block;
position: absolute;
top: 0px;
left: 0px;
width: 100%;
height: 100%;
background: rgba(255,102,0,0.2);
}
The list items will float left and we’ll set the position of the anchors and images to relative:
列表项将向左浮动,我们将锚点和图像的位置设置为相对:
.pe-thumbs li{
float: left;
position: relative;
}
.pe-thumbs li a,
.pe-thumbs li a img{
display: block;
position: relative;
}
Each thumbnail will have an initial width of 100 pixels and an opacity of 0.2:
每个缩略图的初始宽度为100像素,不透明度为0.2:
.pe-thumbs li a img{
width: 100px;
opacity: 0.2;
}
The discription will be hidden by default and then we will make it appear using JavaScript:
默认情况下将隐藏该说明,然后使用JavaScript使它显示:
.pe-thumbs li a div.pe-description{
width: 200px;
height: 100px;
background: rgba(0,0,0,0.8);
position: absolute;
top: 0px;
left: -200px;
color: white;
display: none;
z-index: 1001;
text-align: left;
}
And finally, let’s style the content of the description division:
最后,让我们对描述部门的内容进行样式设置:
.pe-description h3{
padding: 10px 10px 0px 10px;
line-height: 20px;
font-family: 'BebasNeueRegular', 'Arial Narrow', Arial, sans-serif;
font-size: 22px;
margin: 0px;
}
.pe-description p{
padding: 10px 0px;
margin: 10px;
font-size: 11px;
font-style: italic;
border-top: 1px solid rgba(255,255,255,0.3);
}
And that’s all the style! Let’s move on to the JavaScript!
这就是所有样式! 让我们继续学习JavaScript!
JavaScript (The JavaScript)
The main idea is to first of all calculate the description container size and position when we hover over a thumbnail. This depends of course on the maximum scale of the thumbnail and where the thumbnail is located in the main wrapper. For example, when the thumbnail is close to the right edge, we’ll want to make the description box appear on the left side of the thumbnail.
主要思想是,当我们将鼠标悬停在缩略图上时,首先计算描述容器的大小和位置。 当然,这取决于缩略图的最大比例以及缩略图在主包装中的位置。 例如,当缩略图靠近右边缘时,我们将使描述框显示在缩略图的左侧。
Then we’ll need to bind the proximity event to the images. The idea is to scale the images up or down according to the position of the mouse. Once the image scales to the maximum value, we set its z-index very high, so that it stays on top, and we show the respective description:
然后,我们需要将接近事件绑定到图像。 这个想法是根据鼠标的位置放大或缩小图像。 图像缩放到最大值后,我们将其z-index设置得很高,使其停留在顶部,然后显示相应的描述:
// list of thumbs
var $list = $('#pe-thumbs'),
// list's width and offset left.
// this will be used to know the position of the description container
listW = $list.width(),
listL = $list.offset().left,
// the images
$elems = $list.find('img'),
// the description containers
$descrp = $list.find('div.pe-description'),
// maxScale : maximum scale value the image will have
// minOpacity / maxOpacity : minimum (set in the CSS) and maximum values for the image's opacity
settings = {
maxScale : 1.3,
maxOpacity : 0.9,
minOpacity : Number( $elems.css('opacity') )
},
init = function() {
// minScale will be set in the CSS
settings.minScale = _getScaleVal() || 1;
// preload the images (thumbs)
_loadImages( function() {
_calcDescrp();
_initEvents();
});
},
// Get Value of CSS Scale through JavaScript:
// http://css-tricks.com/get-value-of-css-rotation-through-javascript/
_getScaleVal= function() {
var st = window.getComputedStyle($elems.get(0), null),
tr = st.getPropertyValue("-webkit-transform") ||
st.getPropertyValue("-moz-transform") ||
st.getPropertyValue("-ms-transform") ||
st.getPropertyValue("-o-transform") ||
st.getPropertyValue("transform") ||
"fail...";
if( tr !== 'none' ) {
var values = tr.split('(')[1].split(')')[0].split(','),
a = values[0],
b = values[1],
c = values[2],
d = values[3];
return Math.sqrt( a * a + b * b );
}
},
// calculates the style values for the description containers,
// based on the settings variable
_calcDescrp = function() {
$descrp.each( function(i) {
var $el = $(this),
$img = $el.prev(),
img_w = $img.width(),
img_h = $img.height(),
img_n_w = settings.maxScale * img_w,
img_n_h = settings.maxScale * img_h,
space_t = ( img_n_h - img_h ) / 2,
space_l = ( img_n_w - img_w ) / 2;
$el.data( 'space_l', space_l ).css({
height : settings.maxScale * $el.height(),
top : -space_t,
left : img_n_w - space_l
});
});
},
_initEvents = function() {
$elems.on('proximity.Photo', { max: 80, throttle: 10, fireOutOfBounds : true }, function(event, proximity, distance) {
var $el = $(this),
$li = $el.closest('li'),
$desc = $el.next(),
scaleVal = proximity * ( settings.maxScale - settings.minScale ) + settings.minScale,
scaleExp = 'scale(' + scaleVal + ')';
// change the z-index of the element once
// it reaches the maximum scale value
// also, show the description container
if( scaleVal === settings.maxScale ) {
$li.css( 'z-index', 1000 );
if( $desc.offset().left + $desc.width() > listL + listW ) {
$desc.css( 'left', -$desc.width() - $desc.data( 'space_l' ) );
}
$desc.fadeIn( 800 );
}
else {
$li.css( 'z-index', 1 );
$desc.stop(true,true).hide();
}
$el.css({
'-webkit-transform' : scaleExp,
'-moz-transform' : scaleExp,
'-o-transform' : scaleExp,
'-ms-transform' : scaleExp,
'transform' : scaleExp,
'opacity' : ( proximity * ( settings.maxOpacity - settings.minOpacity ) + settings.minOpacity )
});
});
},
_loadImages = function( callback ) {
var loaded = 0,
total = $elems.length;
$elems.each( function(i) {
var $el = $(this);
$('
').load( function() {
++loaded;
if( loaded === total )
callback.call();
}).attr( 'src', $el.attr('src') );
});
};
return {
init : init
};
演示版(Demos)
Check out all three examples:
查看所有三个示例:
翻译自: https://tympanus.net/codrops/2012/01/04/thumbnail-proximity-effect/
jquery缩略图