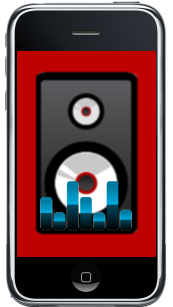
Here is a very useful code snippet for playing your own sound file continuously in your iPhone application. The example uses a .caf file and is based on the AVAudioPlayer Class. You can find the class reference of Apple here: http://developer.apple.com/iPhone/library/documentation/AVFoundation/Reference/AVAudioPlayerClassReference/Reference/Reference.html
这是一个非常有用的代码片段,可用于在iPhone应用程序中连续播放自己的声音文件。 该示例使用.caf文件,并且基于AVAudioPlayer类。 您可以在这里找到Apple的类参考: http : //developer.apple.com/iPhone/library/documentation/AVFoundation/Reference/AVAudioPlayerClassReference/Reference/Reference.html
Let’s assume that you have a view controller where you want to play some sound. I will first start by the header file of your view controller and then move on to the methods in your class MyViewController.m.
假设您有一个要在其中播放声音的视图控制器。 我将首先从视图控制器的头文件开始,然后再转到类MyViewController.m中的方法。
In the header file MyViewController.h we will define the audio player first:
在头文件MyViewController.h中,我们将首先定义音频播放器:
#import <UIKit/UIKit.h>
@interface MyViewController : UIViewController {
AVAudioPlayer *player;
}
@property (nonatomic, retain) AVAudioPlayer *player;
@end
In MyViewController.m we want to load the sound file from our project and allocate the AVAudioPlayer. Further, we will want to define the looping behavior and the volume. This is how we integrate all that in MyViewController.m:
在MyViewController.m中,我们要从项目中加载声音文件并分配AVAudioPlayer。 此外,我们将要定义循环行为和音量。 这就是我们将所有内容集成到MyViewController.m中的方式:
#import "MyViewController.h"
@interface MyViewController()
-(void)playSound;
@end
@implementation MyViewController
@synthesize player;
- (void)viewDidLoad {
[super viewDidLoad];
NSString *soundFilePath =
[[NSBundle mainBundle] pathForResource: @"mySound" ofType: @"caf"];
NSURL *fileURL =
[[NSURL alloc] initFileURLWithPath: soundFilePath];
AVAudioPlayer *newPlayer =
[[AVAudioPlayer alloc] initWithContentsOfURL: fileURL error: nil];
[fileURL release];
self.player = newPlayer;
[newPlayer release];
}
- (void)viewWillAppear:(BOOL)animated{
player.numberOfLoops = -1;
player.currentTime = 0;
player.volume = 1.0;
[self playSound];
}
- (void)viewWillDisappear:(BOOL)animated{
if (self.player.playing) {
[self.player stop];
}
}
- (void) playSound{
[self.player play];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
- (void)viewDidUnload {
}
- (void)dealloc {
[super dealloc];
[player release];
}
@end
Setting numberOfLoops to a negative integer will make your sound loop until you call stop to terminate the playing of the sound. The currentTime is the moment from when you want to play the sound in seconds. So zero means that you want to start from the beginning. The method playSound will call play to actually play the sound.
将numberOfLoops设置为负整数将使您的声音循环,直到您调用stop终止声音播放为止。 currentTime是从几秒钟开始播放声音的时刻。 因此,零表示您要从头开始。 playSound方法将调用play来实际播放声音。
Check out the class reference for more options, like pausing your sound or playing more sounds simultaneously. I hope it helped! Enjoy!
请查阅课程参考,以了解更多选项,例如暂停声音或同时播放更多声音。 希望对您有所帮助! 请享用!

翻译自: https://tympanus.net/codrops/2009/09/15/how-to-play-sound-in-your-iphone-app/