Here you will learn about Bellman-Ford Algorithm in C and C++.
在这里,您将了解C和C ++中的Bellman-Ford算法。
Dijkstra and Bellman-Ford Algorithms used to find out single source shortest paths. i.e. there is a source node, from that node we have to find shortest distance to every other node. Dijkstra algorithm fails when graph has negative weight cycle. But Bellman-Ford Algorithm won’t fail even, the graph has negative edge cycle. If there any negative edge cycle it will detect and say there is negative edge cycle. If not it will give answer to given problem.
Dijkstra和Bellman-Ford算法用于找出单源最短路径。 也就是说,有一个源节点,我们必须从该节点找到到其他每个节点的最短距离。 当图具有负权重循环时,Dijkstra算法失败。 但是Bellman-Ford算法甚至不会失败,因为图形具有负边沿周期。 如果有任何负边缘周期,它将检测并说有负边缘周期。 如果没有,它将给定问题的答案。
Bellman-Ford Algorithm will work on logic that, if graph has n nodes, then shortest path never contain more than n-1 edges. This is exactly what Bellman-Ford do. It is enough to relax each edge (v-1) times to find shortest path. But to find whether there is negative cycle or not we again do one more relaxation. If we get less distance in nth relaxation we can say that there is negative edge cycle. Reason for this is negative value added and distance get reduced.
Bellman-Ford算法将适用于以下逻辑: 如果图具有n个节点,则最短路径永远不会包含n-1个以上的边 。 这正是贝尔曼·福特所做的。 放松每个边缘(v-1)次以找到最短路径就足够了。 但是要发现是否存在负周期,我们再次放松一遍。 如果我们在第n次弛豫中得到的距离较小,则可以说存在负边缘周期。 原因是增加了负值,距离减小了。
Relaxing edge
松弛的边缘
In algorithm and code below we use this term Relaxing edge.
在下面的算法和代码中,我们使用此术语松弛边。
Relaxing edge is an operation performed on an edge (u, v) . when,
松弛边缘是在边缘(u,v)上执行的操作。 什么时候,
d(u) > d(v) + Cost(u,v)
d(u)> d(v)+成本(u,v)
Here d(u) means distance of u. If already known distance to “u” is greater than the path from “s” to “v” and “v” to “u” we update that d(u) value with d(v) + cost(u,v).
在此,d(u)表示u的距离。 如果已知的到“ u”的距离大于从“ s”到“ v”以及从“ v”到“ u”的距离,我们用d(v)+ cost(u,v)更新该d(u)值。
算法和时间复杂度 (Algorithm and Time Complexity)
Bellman-Ford (G,w,S){ //G is graph given, W is weight matrix, S is source vertex (starting vertex)
Initialize single source (G,S) //means initially distance to every node is infinity except to source. Source is 0 (zero). This will take O(v) time
For i=1 to |G.V| -1 //Runs (v-1) times
For each edge (G,V)€ G.E // E times
Relax(u,v,w) //O(1) time
For each edge (G,V) € G.E
If (v.d > u.d + w(u,v)) //The idea behind this is we are relaxing edges nth time if we found more shortest path than (n-1)th level, we can say that graph is having negative edge cycle and detected.
Return False
return true
}
Finally time complexity is (v-1) (E) O(1) = O(VE)
最后时间复杂度为(v-1)(E)O(1)= O(VE)
示例问题 (Example Problem)
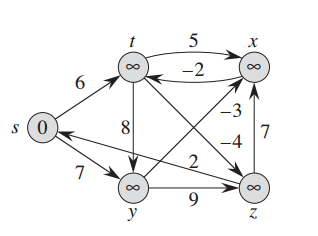
This is the given directed graph.
这是给定的有向图。
(s,t) = 6 (y,x) = -3
(s,t)= 6(y,x)= -3
(s,y)= 7 (y,z) = 9
(s,y)= 7(y,z)= 9
(t,y) = 8 (x,t) = -2
(t,y)= 8(x,t)= -2
(t,z) = -4 (z,x) = 7
(t,z)= -4(z,x)= 7
(t,x) = 5 (z,s) = 2
(t,x)= 5(z,s)= 2
Above we can see using vertex “S” as source (Setting distance as 0), we initialize all other distance as infinity.
在上方我们可以看到使用顶点“ S”作为源(将距离设置为0),我们将所有其他距离初始化为无穷大。
S | T | X | Y | Z | |
distance | 0 | ∞ | ∞ | ∞ | ∞ |
Path | – | – | – | – | – |
小号 | Ť | < |