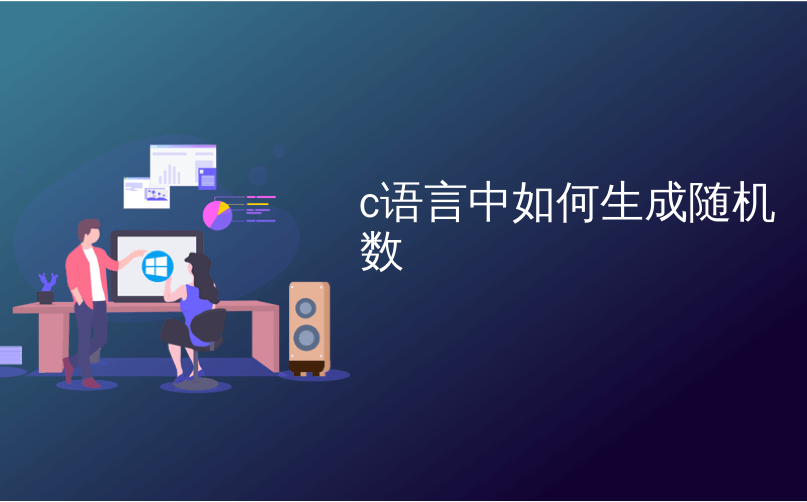
c语言中如何生成随机数
Here you will get program and learn how to generate random number in C and C++.
在这里,您将获得程序并学习如何在C和C ++中生成随机数 。
We can use rand() function to generate random number. It is defined in stdlib.h header file. It generates a random number between 0 and RAND_MAX (both inclusive). Here RAND_MAX is a constant whose value depends upon the compiler you are using.
我们可以使用rand()函数生成随机数。 它在stdlib.h头文件中定义。 它会生成一个介于0和RAND_MAX (包括两端)之间的随机数。 RAND_MAX是一个常量,其值取决于您使用的编译器。
The random value returned by rand() depends upon the initial value called as seed. The value of seed is same each time you run the program. Due to this the sequence of random numbers generated in each program run are same.
rand()返回的随机值取决于称为种子的初始值。 每次您运行该程序时,seed的值都相同。 因此,在每次程序运行中生成的随机数序列都是相同的。
To solve this problem we provide new seed value on each program run. You can give seed value to rand() function algorithm using srand() function. We give current time in seconds as an argument of srand() to give a unique seed value for each program run.
为了解决这个问题,我们在每次运行程序时提供新的种子值。 您可以使用srand()函数为rand()函数算法提供种子值。 我们将当前时间(以秒为单位)作为srand()的参数,以为每个程序运行提供唯一的种子值。
Note: Using rand() is not a secure way of generating random number if you are developing a real world application. To generate a secure random number read this.
注意:如果要开发实际应用程序,则使用rand()并不是生成随机数的安全方法。 要生成一个安全的随机数,请阅读this 。
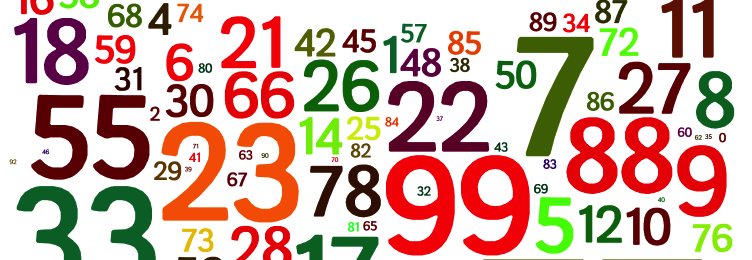
在C和C ++中生成随机数的程序 (Program to Generate Random Number in C and C++)
C Program
C程序
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
int main(){
srand(time(NULL));
printf("%d", rand());
return 0;
}
C++ Program
C ++程序
#include<iostream>
#include<stdlib.h>
#include<time.h>
using namespace std;
int main(){
srand(time(NULL));
cout<<rand();
return 0;
}
如何生成一个范围内的随机数? (How to generate random number in a range?)
Suppose there are two numbers a and b. Then result of a%b will be always in between 0 and b-1 (both inclusive). So we will use this concept to generate random number in a range.
假设有两个数字a和b 。 那么a%b的结果将始终在0到b-1之间 (包括两者)。 因此,我们将使用此概念来生成一定范围内的随机数。
Lets say you want to generate number in between 1 and 10 then it can be done by rand()%10+1.
假设您要生成1到10之间的数字,然后可以通过rand()%10 + 1完成 。
Comment below if you have doubts or found anything incorrect in above tutorial for random number in C and C++.
如果您对上面的教程中的C和C ++中的随机数有疑问或发现不正确之处,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2016/12/generate-random-number-c.html
c语言中如何生成随机数