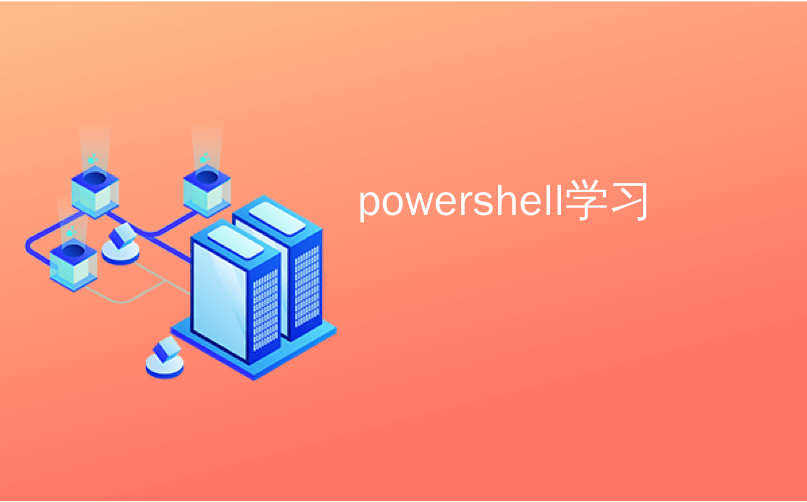
powershell学习
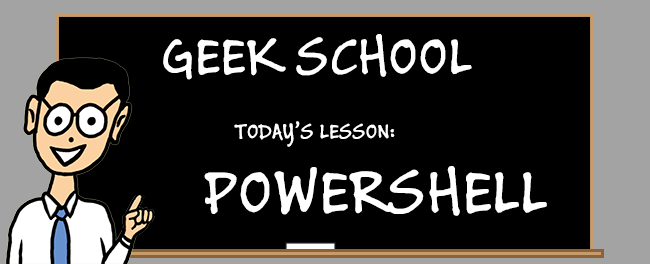
Understanding objects is one of the fundamental concepts to “getting” PowerShell. Join us as we explore objects and how they make PowerShell better than any other shell out there today.
了解对象是“获取” PowerShell的基本概念之一。 与我们一起探索对象,以及它们如何使PowerShell优于当今的任何其他Shell。
Be sure to read the previous articles in the series:
确保阅读本系列中的先前文章:
And stay tuned for the rest of the series all week.
并继续关注本系列的其余部分。
对象 (Objects)
Have you ever wondered what sets PowerShell apart from a traditional Linux shell like Bash, or even the legacy command prompt? The answer is really simple: traditional shells output text, which makes it difficult to do things like formatting and filtering. Of course, there are tools to help you get the job done (sed and grep come to mind), but at the end of the day, if you want to do any kind of heavy text parsing, you need to know regular expressions like the back of your hand.
您是否曾经想过,PowerShell与Bash甚至传统命令提示符等传统Linux Shell有何不同? 答案很简单:传统的shell输出文本,这使得很难进行格式化和过滤之类的操作。 当然,有一些工具可以帮助您完成工作(想到sed和grep),但是最终,如果您想进行任何种类的繁重文本解析,则需要了解正则表达式,例如手背
PowerShell takes advantage of the underlying .Net framework and takes a different approach, using objects instead of text. Objects are just a representation of something. They are a collection of parts and actions to use them. Let’s take a look at the parts of a bicycle and how we might use them.
PowerShell利用底层的.Net框架并采用不同的方法,即使用对象而不是文本。 对象只是某种事物的表示。 它们是使用它们的部分和动作的集合。 让我们看一下自行车的各个部分以及如何使用它们。
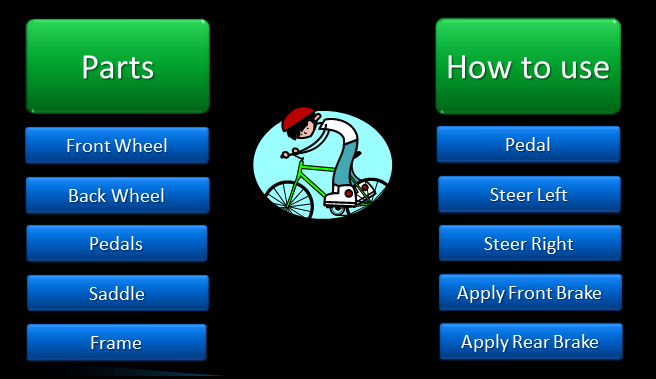
Objects in .Net are much the same except for two small differences: the “Parts” are called properties and the “Instructions” are called methods. If we wanted to represent a Windows Service as an object, we might decide that it is appropriate to describe it using three properties: Service Name, State and Description. We also need to interact with the service, so we might give the object a Start, a Stop and a Pause method.
.Net中的对象几乎相同,除了两个小区别:“部件”称为属性 ,“指令”称为方法 。 如果我们想将Windows服务表示为对象,则可以决定使用三个属性来描述它是适当的:服务名称,状态和描述。 我们还需要与服务进行交互,因此我们可以为对象提供Start,Stop和Pause方法。
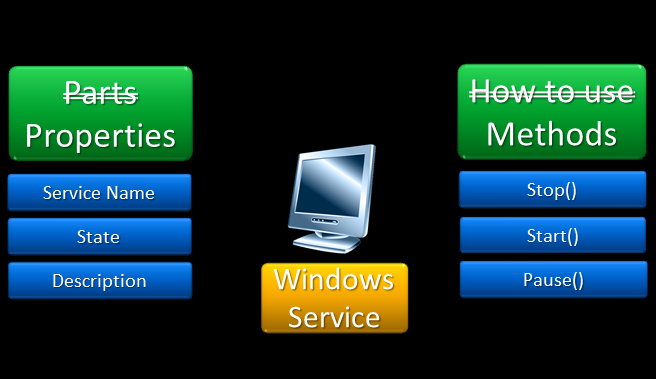
You can see an object’s properties and methods by passing it to the Get-Member cmdlet. The objects that a PowerShell cmdlet outputs are largely underlying types from the .Net framework, but you can create your own objects if you need to use a language like C# or use the PSObject type.
通过将对象传递给Get-Member cmdlet,可以查看其属性和方法。 PowerShell cmdlet输出的对象主要是.Net框架中的基础类型,但是如果需要使用C#之类的语言或使用PSObject类型,则可以创建自己的对象。
管道 (The Pipeline)
There are plenty of Linux shells with a pipeline, allowing you to send the text that one command outputs as input to the next command in the pipeline. PowerShell takes this to the next level by allowing you to take the objects that one cmdlet outputs and pass them as input to the next cmdlet in the pipeline. The trick is knowing what type of object a cmdlet returns, which is really easy when using the Get-Member cmdlet.
有很多带有管道Linux Shell,使您可以将一个命令输出的文本作为输入发送给管道中的下一个命令。 PowerShell通过允许您获取一个cmdlet输出的对象并将它们作为输入传递到管道中的下一个cmdlet,将其提升到一个新的水平。 诀窍是知道cmdlet返回什么类型的对象,这在使用Get-Member cmdlet时确实很容易。
Get-Service | Get-Member
服务获取| 获得会员
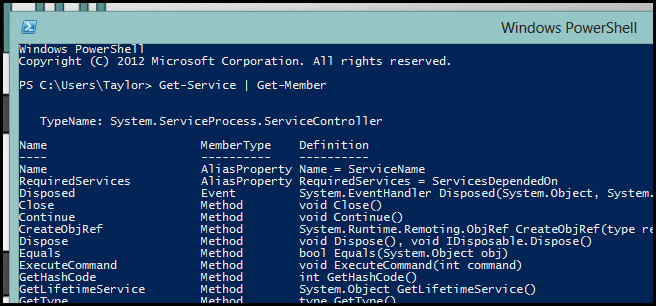
For reasons beyond the scope of this article, properties and methods are jointly called class members, which explains why you use the Get-Member cmdlet to get a list of all the methods and properties an object has. However, the Get-Member cmdlet also returns another important piece of information, the underlying object type. In the above screenshot, we can see that Get-Service returns objects of the type:
由于超出了本文范围的原因,属性和方法被统称为类成员,这说明了为什么使用Get-Member cmdlet来获取对象具有的所有方法和属性的列表。 但是,Get-Member cmdlet还返回另一条重要信息,即基础对象类型。 在上面的屏幕截图中,我们可以看到Get-Service返回了以下类型的对象:
System.ServiceProcess.ServiceController
System.ServiceProcess.ServiceController
Since PowerShell deals with objects and not text, not all cmdlets can be linked together using the pipeline[1]. That means we need to find a cmdlet that’s looking to accept a System.ServiceProcess.ServiceController object from the pipeline.
由于PowerShell处理对象而不是文本,因此不能使用管道将所有cmdlet链接在一起[1]。 这意味着我们需要找到一个cmdlet,该cmdlet希望从管道中接受System.ServiceProcess.ServiceController对象。
Get-Command -ParameterType System.ServiceProcess.ServiceController
Get-Command -ParameterType System.ServiceProcess.ServiceController
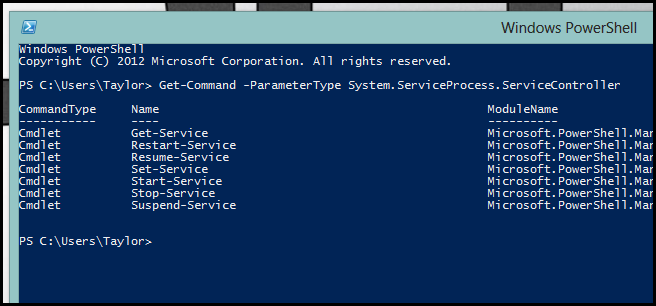
Notice that there is a cmdlet called Stop-Service; let’s take a look at the help for it.
注意,有一个名为Stop-Service的cmdlet。 让我们来看看它的帮助。
Get-Help –Name Stop-Service
获取帮助–名称停止服务
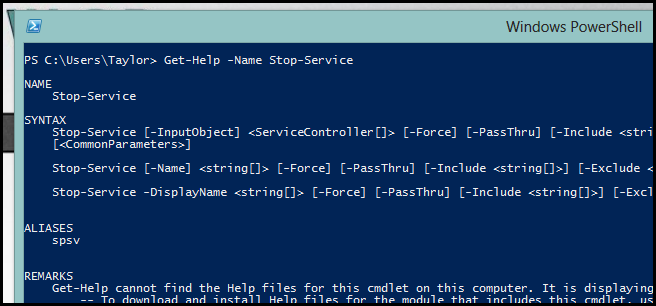
It looks like the InputObject parameter takes an array of ServiceController objects as input. Usually, if you see a parameter called InputObject, it will accept input from the Pipeline, but just to be sure let’s take a look at the full help for that parameter.
看起来InputObject参数采用ServiceController对象的数组作为输入。 通常,如果看到一个名为InputObject的参数,它将接受来自管道的输入,但是为了确保让我们看一下该参数的完整帮助。
Get-Help -Name Stop-Service –Full
获取帮助-名称停止服务–完整
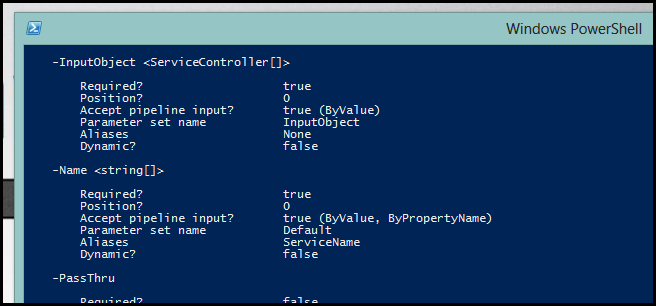
Our suspicions were correct. So at this point we know the following:
我们的猜想是正确的。 因此,在这一点上,我们知道以下几点:
- Get-Service returns ServiceController objects Get-Service返回ServiceController对象
- Stop-Service has a parameter called InputObject that accepts one or more ServiceControllers as input. Stop-Service具有一个名为InputObject的参数,该参数接受一个或多个ServiceController作为输入。
- The InputObject parameter accepts pipeline input. InputObject参数接受管道输入。
Using this information we could do the following:
使用此信息,我们可以执行以下操作:
Get-Service -Name ‘Apple Mobile Device’ | Stop-Service
Get-Service-名称“ Apple Mobile Device” | 停止服务
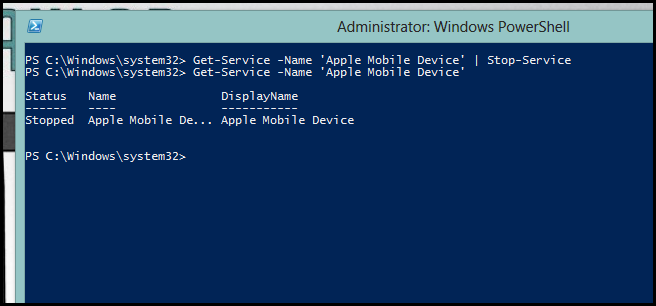
That’s all for this time folks. Next time we look at how we can format, filter and compare objects in the Pipeline.
这一次所有的人。 下次,我们将研究如何格式化,过滤和比较管道中的对象。
家庭作业 (Homework)
If you have any questions you can tweet me @taybgibb, or just leave a comment.
如果您有任何疑问,可以发给我@taybgibb ,或发表评论。
翻译自: https://www.howtogeek.com/138121/geek-school-learning-powershell-objects/
powershell学习