javascript手风琴
In the modern web, people aren't satisfied just with the boring text on the screen. Images could help, but what if your content is only text? With modern JavaScript, you can spice up anything on your webpage. Enhance its looks, animate elements on the page or even keep mini games like effects on mouse movement. Those tricks for some other day, but today, we will look at how to create accordions with JavaScript?
在现代网络中,人们对屏幕上无聊的文字不满意。 图片可能会有所帮助,但是如果您的内容仅是文字呢? 使用现代JavaScript,您可以在网页上添加任何内容。 增强外观,为页面上的元素设置动画,甚至保留迷你游戏,例如对鼠标移动的效果。 这些技巧还有一天,但是今天,我们将研究如何使用JavaScript创建手风琴 ?
Accordions are elements that have a tab like structure, which hides the content under its header, sort of like category. When the user clicks on the header, the content element slides down and shows the content under that header.
手风琴是具有选项卡式结构的元素,该结构将内容隐藏在其标题下,类似于类别。 当用户单击标题时,内容元素将向下滑动并在该标题下显示内容。
Starting out first with barebones HTML structure, we need two things, one heading and another is the content below it that will be shown when the user clicks on this header.
首先从准系统HTML结构开始,我们需要两件事,一是标题,二是其下方的内容,当用户单击此标头时将显示该内容。
<div class="container">
<p>accordion Example</p>
<div class="accordion-container">
<div class="accordion">
<h2 class="accordion-header">Tab 1</h2>
<div class="content">
<p>Welcome to Tab 1</p>
</div>
</div>
<div class="accordion">
<h2 class="accordion-header">Tab 2</h2>
<div class="content">
<p>Welcome to Tab 2</p>
</div>
</div>
<div class="accordion">
<h2 class="accordion-header">Tab 3</h2>
<div class="content">
<p>Welcome to Tab 3</p>
</div>
</div>
</div>
</div>
Here we have a parent .container for our code. Inside this, we have a simple text showing accordion example.
在这里,我们为代码提供了一个父.container 。 在其中,我们有一个简单的文本显示了手风琴示例。
Below it, we have div wrapper for accordion named .accordion-container that will hold all the .accordion elements. Inside each accordion element we have a h2 element, that is our header with the class .accordion-header and below it we have .content element that wraps our content.
在它下面,我们有一个名为.accordion-container的手风琴的div包装器,它将容纳所有.accordion元素。 在每个手风琴元素中,我们都有一个h2元素,这是我们的类.accordion-header的标题 ,在其下方,我们具有.content元素,用于包装内容。
When user will click on the accordion header, then this content will be shown to the user. We have created three instances of this structure, because a normal accordion has multiple indvidual content elements.
当用户单击手风琴标题时,该内容将显示给用户。 我们创建了此结构的三个实例,因为普通的手风琴具有多个单独的内容元素。
Now, let's style this up a bit. Here's the CSS for styling the accordion.
现在,让我们对其进行一些样式化。 这是样式CSS 。
.accordion-header {
background: #e76;
color: #fff;
padding: 5px 10px;
margin: 0;
cursor: pointer;
}
.accordion {
border: 2px solid #e76;
}
.accordion .content {
height: 0px;
overflow-y: hidden;
padding: 0px 10px;
}
.full-height {
height: auto !important;
}
The main thing to note here is that we initially have provided 0px height to the content, since we don't want it to show it up. Next we have a secondary class, .full-height that has the styling, height: auto !important which will change the height to auto. Note the !important condition here, because we want it as the highest priority.
这里要注意的主要事情是,我们最初为内容提供了0px的高度 ,因为我们不希望它显示出来。 接下来,我们有一个二级类.full-height ,其样式为height:auto!important ,它将高度更改为auto。 注意此处的!important条件,因为我们希望将其作为最高优先级。
Now, comes the time to add the real magic to make it functional.
现在,是时候添加真正的魔力来使其发挥作用了。
//Get the elements based on their class name
var tabs = document.getElementsByClassName('accordion-header');
for(var i=0; i<tabs.length; i++) {
// Add event listener to each accordion element
tabs[i].addEventListener('click', function() {
var content = this.nextElementSibling;
content.classList.toggle('full-height');
});
}
In this code, first we have a tabs variable that holds the array of accordion-header elements, which is nothing but our headers.
在这段代码中,首先我们有一个tabs变量,它保存着手风琴式标题元素的数组,而这个数组只是标题。
Now, for each element of this array, we need it to respond on clicks. To make that happen, we need to add an event listener to register click, and inside that we need the next element of this header, which is our content element.
现在,对于该数组的每个元素,我们需要它来响应点击。 为了实现这一点,我们需要添加一个事件侦听器来注册点击,并且在其中我们需要此标头的下一个元素,即我们的内容元素。
Now using the this.nextElementSibling we get the next element corresponding to the current accordion-header element. Next we toggle the class full-height for this content element. We do toggle instead of add so that on second click, the toggle will remove the class full-height from the element and it will return to default 0px height, we set for .content element in CSS.
现在,使用this.nextElementSibling获取与当前手风琴标题元素相对应的下一个元素。 接下来,我们切换该内容元素的全高类。 我们进行切换而不是添加,以便在第二次单击时,切换将从元素中删除全高类,并且它将返回到默认的0px高度,这是我们在CSS中为.content元素设置的。
This is how you can easily create accordion content with JavaScript and CSS.
这是您可以轻松使用JavaScript和CSS创建手风琴内容的方法。
Checkout live demo here.
Output
输出量
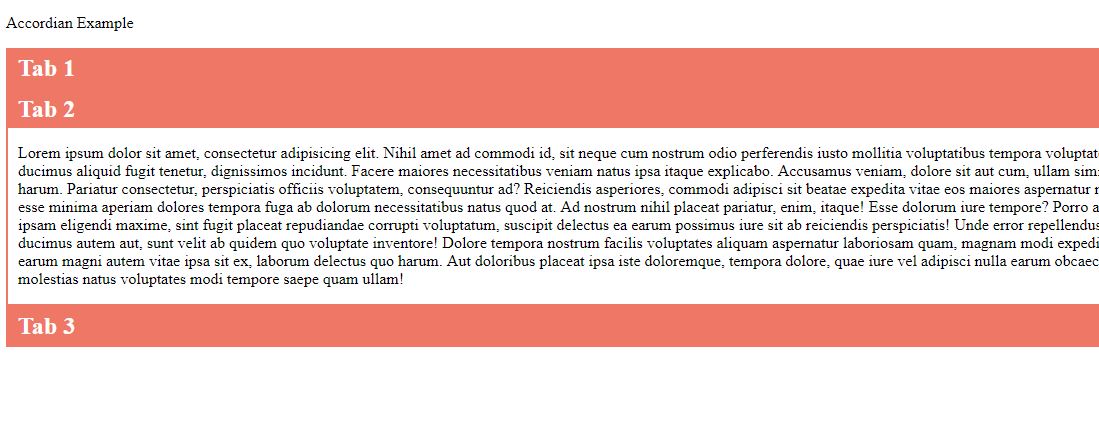
If you like this article, please comment and share your thoughts.
如果您喜欢这篇文章,请发表评论并分享您的想法。
翻译自: https://www.includehelp.com/code-snippets/create-accordions-with-javascript.aspx
javascript手风琴