原生dom实现导航栏
We know that whenever a web browser loads a web page, it renders an HTML DOM which is a tree-like data structure that is manipulated using JavaScript. This tree is generic and is usually called the node tree where each HTML DOM element represents a node. Starting from any random node in the node tree you can traverse the tree and reach any other node using the tree node relationships. These relationships define the relations between various HTML elements on the web page.
我们知道,每当网络浏览器加载网页时,它都会呈现HTML DOM,这是一种使用JavaScript进行操作的树状数据结构。 该树是通用树,通常称为节点树,其中每个HTML DOM元素代表一个节点。 从节点树中的任何随机节点开始,您可以遍历树并使用树节点关系到达任何其他节点。 这些关系定义了网页上各种HTML元素之间的关系。
In this article, we'll look at how we can navigate the DOM by utilizing the relationship between various nodes in the node tree.
在本文中,我们将研究如何利用节点树中各个节点之间的关系来导航DOM。
Let's familiarise ourselves with some DOM standards set by W3C. The entire document that the browser loads is itself a node and is called the document node. Inside this document or under this document node, various branches point towards other nodes which are the HTML element nodes. Any text or content embedded in these HTML tags is the text nodes.
让我们熟悉W3C设置的一些DOM标准。 浏览器加载的整个文档本身就是一个节点,称为文档节点。 在此文档内或该文档节点下,各种分支指向其他节点,这些节点是HTML元素节点。 这些HTML标记中嵌入的任何文本或内容都是文本节点。
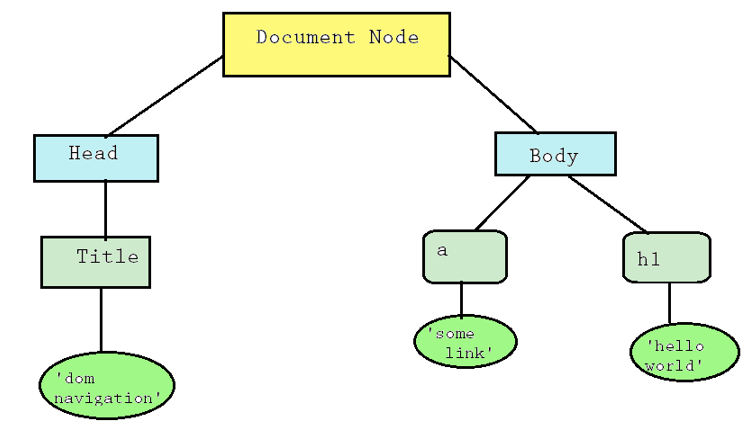
Regarding this simple DOM in the example, go ahead and create a similar index.html file with the following HTML,
关于示例中的简单DOM,请继续使用以下HTML创建类似的index.html文件,
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="styles.css">
<title>Events in DOM</title>
</head>
<body>
<a href="">some link</a>
<h1>Hello world</h1>
</body>
<script>
</script>
</html>
Output
输出量
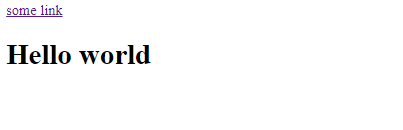
We know that the nodes in the tree have a hierarchical relationship so we can easily deduce the node tree relationships which will eventually help in navigating the DOM. The most common tree node relationships are parent, child, and sibling.
我们知道树中的节点具有层次关系,因此我们可以轻松推断出节点树关系,这最终将有助于导航DOM。 最常见的树节点关系是父级,子级和同级。
Our document node is the root node and it has two children, the <head> and the <body>. This document node is the root node as well as the parent node for its children <head> and <body>. The <head> has a <title> which is again a child node for the <head>, being the parent node for its child text node.
我们的文档节点是根节点,它有两个子节点,分别是<head>和<body> 。 该文档节点是其子节点<head>和<body>的根节点以及父节点。 <head>具有<title> ,它又是<head>的子节点,是其子文本节点的父节点。
The <body> is the parent node for it's two children <a> and <h1>. These children nodes themselves are siblings to one another and are parent nodes for their children's content or text nodes.
<body>是其两个子节点<a>和<h1>的父节点。 这些子节点本身是彼此的兄弟姐妹,并且是其子节点内容或文本节点的父节点。
Alright now let's navigate the DOM using these relationships that we have defined.
现在,让我们使用我们定义的这些关系导航DOM。
Let's get a reference to the <head> and <body> first.
首先让我们引用<head>和<body> 。
<script>
const head = document.querySelector('head');
const body = document.querySelector('body');
console.log('head: ', head);
console.log('body: ', body);
</script>
Output
输出量
head: <head>…</head>
<meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><meta http-equiv="X-UA-Compatible" content="ie=edge"><link rel="stylesheet" href="styles.css"><title>Events in DOM</title></head>
body: <body cz-shortcut-listen="true">…</body>
<a href>some link</a><h1>Hello world</h1><!-- Code injected by live-server --><script type="text/javascript">…</script><script>…</script></body>
We get <head> along with all it's descendants and <body> along with all its descendants.
我们得到<head>及其所有后代,以及<body>及其所有后代。
If we want to access the children of <head>.
如果要访问<head>的子级 。
console.log('Children of head:',head.children);
Output
输出量
Child of head: HTMLCollection(5) [meta, meta, meta, link, title, viewport: meta]
Alternately we can get to the <head> from <title> by getting a reference to <title> and asking for it's parent element.
或者,我们可以通过获取对<title>的引用并请求其父元素来从<title>进入<head> 。
const title=document.querySelector('title');
console.log('parent of title: ',title.parentElement);
Output
输出量
parent of title: <head> ... </head>
We know that both <a> and <h1> are children of <body> and have a sibling relationship with each other. Let's get a reference to <a> and navigate to <h1> using their sibling relationship.
我们知道<a>和<h1>都是<body>的子代,并且彼此具有同级关系。 让我们获得对<a>的引用,并使用它们的同级关系导航到<h1> 。
const link=document.querySelector('a');
console.log('Link: ',link);
console.log('Sibling of <a> :',link.nextElementSibling);
Output
输出量
Link: <a>some link</a>
Sibling of <a> : <h1>Hello world</h1>
Similarly we can get a reference to <h1> and navigate to <a>.
同样,我们可以引用<h1>并导航到<a> 。
const heading=document.querySelector('h1');
console.log('Heading: ',heading);
console.log('Sibling of <h1> :',heading.previousElementSibling);
Output
输出量
Heading: <h1>Hello world</h1>
Sibling of <h1> : <a>some link</a>
We can also chain these relationships together to navigate the DOM. Let's try to navigate to <h1> from the <title>.
我们还可以将这些关系链接在一起以导航DOM。 让我们尝试从<title>导航到<h1 > 。
We first get the parent element of <title> which will give us <head>, go to it's next sibling which is <body>, go to it's children which will return us an array of children having <a> and <h1> and simply access the <h1> using the it's index in the children array.
我们首先得到<title>的父元素,该元素将给我们<head> ,转到下一个兄弟姐妹<body> ,转到子元素,该子元素将返回具有<a>和<h1>的子元素数组,并且只需使用children数组中的索引访问<h1> 。
console.log('From <title> to <h1>: ',title.parentElement.nextElementSibling.children[1])
Output
输出量
From <title> to <h1>: <h1>Hello world</h1>
Thus, we can easily navigate the DOM using the DOM tree and create nodes, delete nodes, add children to existing nodes and do all kinds of stuff with JavaScript.
因此,我们可以轻松地使用DOM树导航DOM并创建节点,删除节点,将子代添加到现有节点并使用JavaScript进行各种处理。
翻译自: https://www.includehelp.com/code-snippets/the-dom-navigation.aspx
原生dom实现导航栏