A Python module is basically a file that contains ".py" extension and a Python package is any normal folder or directory that contains modules inside it.
Python模块基本上是一个包含“ .py”扩展名的文件,而Python包则是其中包含模块的任何普通文件夹或目录。
How import works?
导入如何工作?
To understand how import basically works in python, Let us import a module named "abc" in python.
要了解导入在python中的基本原理,让我们在python中导入名为“ abc”的模块。
import abc
After seeing the above statement the first thing that Python will do is to look up the name XYZ in sys.modules. This is basically a cache of all modules that have been imported previously.
看到上面的陈述后,Python要做的第一件事是在sys.modules中查找名称XYZ 。 这基本上是先前已导入的所有模块的缓存。
If this name is not found in the cache module then the python will proceed its searching through a list of the built-in module. This built-in module is basically pre-installed with python and can be seen through Python Standard Library. If the name still is not available at the built-in modules then the python will search for it in a list of directories that are basically defined by sys.path.
如果在缓存模块中找不到此名称,则python将继续通过内置模块列表进行搜索。 这个内置模块基本上预装了python,可以通过Python标准库查看。 如果该名称在内置模块上仍然不可用,则python将在基本上由sys.path定义的目录列表中进行搜索。
When Python finally finds the module it will bind it to a name in the local scope. This means that XYZ is now defined and it can be used in the current file without throwing an error called NameError.
当Python最终找到该模块时,它将把它绑定到本地范围内的名称。 这意味着XYZ现在已定义,并且可以在当前文件中使用它,而不会引发名为NameError的错误。
If the name or file is still not found then we'll get an error called ModuleNotFoundError.
如果仍未找到名称或文件,则将收到名为ModuleNotFoundError的错误。
Syntax:
句法:
In python we can write import statement in two ways:
在python中,我们可以通过两种方式编写import语句:
Import Resource Directly
直接导入资源
Ex:
例如:
import XYZ
导入XYZ
Import Resource from another package or module
从另一个包或模块导入资源
Ex:
例如:
from xyz import abd
从xyz import abd
Styling of Basic Import statement
基本导入语句的样式
The following points everyone should keep in mind while writing the import statement:
每个人在编写导入语句时应牢记以下几点:
These statements should always be written at the top of the file after any type of module comments.
在任何类型的模块注释之后,这些语句应始终写在文件顶部。
These statements should be divided based on how it is imported,these are basically divided into three groups as follows:
这些语句应根据其导入方式进行划分,基本上分为以下三组:
- Standard library import
- Third-party import
- Local application imports
1)绝对进口 (1) Absolute import)
An absolute import basically specifies that the resource to be imported must use its full path from the project’s root folder.
绝对导入基本上是指定要导入的资源必须使用项目根文件夹中的完整路径。
For example:
例如:
Let us consider a project directory that has two subdirectory named package 1 and package 2. Where package 1 further contains two python file named module1.py and module2.py. And package 2 contains three .py files and a directory called subpackage1 which contain a .py file.
让我们考虑一个项目目录,该目录具有两个名为package 1和package 2的子目录。其中package 1进一步包含两个名为module1.py和module2.py的 python文件。 程序包2包含三个.py文件和一个名为subpackage1的目录,该目录包含一个.py文件。
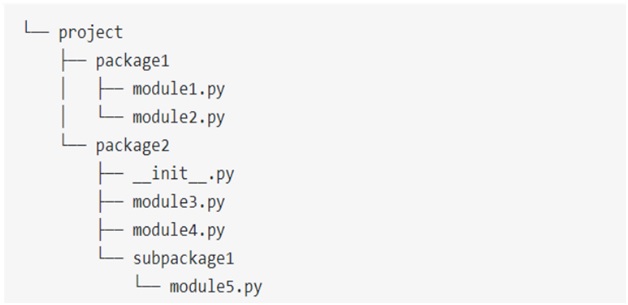
Let’s assume the following:
让我们假设以下内容:
package1 contains module2.py which contains a function named function2.
package1包含module2.py ,其中包含名为function2的函数 。
package2 contains __init__.py contains a class named class2.
package2包含__init__.py包含一个名为class2的类 。
package2 contains subpackage1 which basically contains module5.py which basically contains a function named function3.
package2包含subpackage1,它基本上包含module5.py ,它基本上包含一个名为function3的函数 。
Following are some of the practical example of absolute imports:
以下是一些绝对导入的实际示例:
from package1 import module1
from package2.subpackage1.module5 import function3
Here we have basically given a detailed path for each package or file basically from the top-level package folder. This is basically similar to that file path, but here we have uses a dot (.) instead of a slash (/) that are used in the file path.
在这里,我们基本上从顶层包文件夹中为每个包或文件给出了详细的路径。 这基本上类似于该文件路径,但是这里我们使用了一个点( 。 )而不是文件路径中使用的斜杠( / )。
Advantages and disadvantages of Absolute import
绝对进口的优点和缺点
These imports are preferred because of the following advantages:
这些导入是首选的,因为具有以下优点:
These imports are quite clear and straightforward.
这些导入非常清晰直接。
These are the easiest way to tell where the imported resource is stored.
这些是分辨导入资源存储位置的最简单方法。
These statements remain same even if the current location of imported statement changes.
即使导入的语句的当前位置发生更改,这些语句也将保持不变。
Because of these advantages PEP8 strongly recommends to use absolute import method.
由于这些优点,PEP8强烈建议使用绝对导入方法。
But every coin has two faces absolute import method posses some disadvantage to these are as follows.
但是每个硬币都有两个面的绝对导入方法对它们造成一些缺点如下。
These imports can get quite verbose too based on the complexity of the structure of the directory. let's imagine a statement like as follows:
基于目录结构的复杂性,这些导入也可能变得非常冗长。 让我们想象一个如下的语句:
from package1.subpackage2.subpackage3.subpackage4.subpackage5.module5 import function7
从package1.subpackage2.subpackage3.subpackage4.subpackage5.module5导入功能7
This seems somewhat ridiculous.
这似乎有些荒谬。
2)相对进口 (2) Relative Imports)
A relative import basically specifies that the resource that has been imported is relative to its current location—that is simply the location where the import statement is present.
相对导入基本上指定了已导入的资源是相对于其当前位置的,即相对于存在导入语句的位置。
Syntax:
句法:
Basically, the syntax of a relative import totally depends on the current location as well as the location where the module, package, or object is present which is needed to be imported.
基本上,相对导入的语法完全取决于当前位置以及需要导入的模块,包或对象所在的位置。
Following are some of the examples of Relative Import:
以下是相对导入的一些示例:
from .module import sclass
from ..package import function
from . import sclass
Here a single dot(.) symbol basically refers to the current location or the same directory. Double dot(..) basically refers to the parent directory. And three dot(...) basically means that we are in grandparent directory.
在这里,单个点( 。 )符号基本上是指当前位置或同一目录。 双点( .. )基本上是指父目录。 三个dot( ... )基本上意味着我们在祖父母目录中。
Advantages and disadvantages of relative import
相对导入的优点和缺点
One basic clear advantage of relative imports is that they are quite succinct. Based on the current location they can turn the ridiculously long import statement into something as simple as the following statement:
相对进口的一个基本的明显优势是它们非常简洁。 根据当前位置,他们可以将可笑的冗长的import语句转换为以下语句一样简单:
from ..subpackage4.module5 import function6
Relative Import too posses some disadvantages such as follows:
相对导入也具有一些缺点,例如:
These imports can be quite messy basically for shared projects where directory structure is likely to change to every time. Relative imports are also not as readable or understandable like absolute ones, and these import statements are not that easy to tell the location of the imported resources.
对于目录结构可能每次都更改的共享项目,这些导入基本上可能会非常混乱。 相对导入也不像绝对导入那样易读易懂,并且这些导入语句也不容易分辨出导入资源的位置。
翻译自: https://www.includehelp.com/python/absolute-vs-relative-imports.aspx