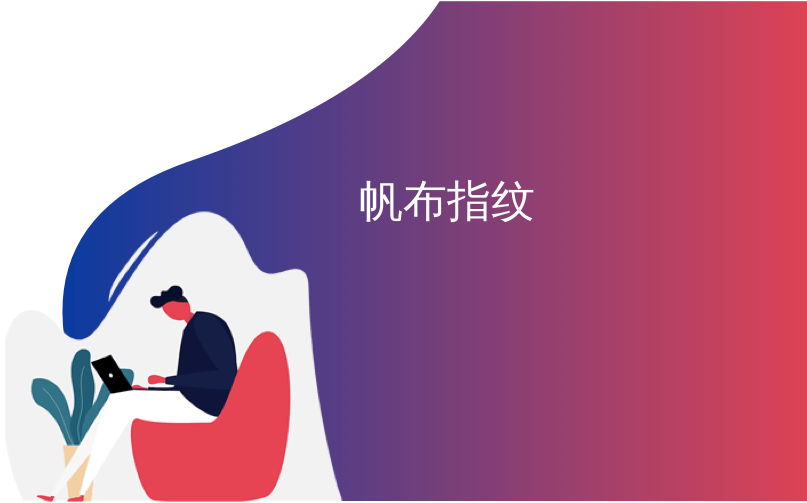
帆布指纹
UPDATE: Translation in Brazilian Portuguese here, thanks Maujor!
更新:这里有巴西葡萄牙语的翻译,谢谢Maujor!
OK, so you have an HTML table. Let's turn it into a pie chart with a bit of javascript.
OK,所以您有一个HTML表。 让我们将其转换为带有一些javascript的饼图。
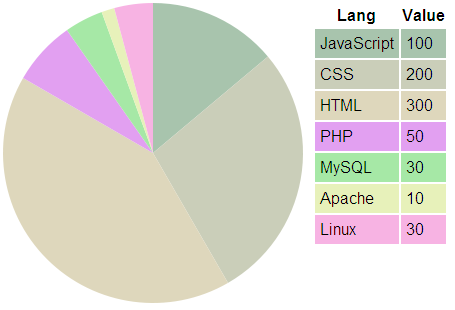
We'll be using the canvas tag, so the browser has to support it. For IE - well, you still have the table. That's why we'll call it progressive enhancement. Unobtrusive too. Here's a screenshot:
我们将使用canvas标记,因此浏览器必须支持它。 对于IE-好的,您仍然可以使用表格。 这就是为什么我们将其称为渐进增强。 也不引人注目。 这是屏幕截图:
» The demo is here (refresh for new colors)
»演示在这里(刷新新颜色)
Here are the ingredients to the recipe:
这是食谱的成分:
One
<canvas>
tag一个
<canvas>
标签One
<table>
full of data一个
<table>
充满数据- javascript to get the data from the tablejavascript从表中获取数据
- javascript to plot the data on the canvasJavaScript在画布上绘制数据
一个画布标签(One canvas tag)
<canvas id="canvas" width="300" height="300"></canvas>
一张表中的数据 (One table full of data)
This is a bare bone unstyled old school table.
这是一副没有样式的老式学校桌。
<table id="mydata">
<tr> <th>Lang</th><th>Value</th> </tr>
<tr><td>JavaScript</td> <td>100</td> </tr>
<tr><td> CSS</td> <td>200</td> </tr>
<tr><td> HTML</td> <td>300</td> </tr>
<tr><td> PHP</td> <td> 50</td> </tr>
<tr><td> MySQL</td> <td> 30</td> </tr>
<tr><td> Apache</td> <td> 10</td> </tr>
<tr><td> Linux</td> <td> 30</td> </tr>
</table>
javascript从表中获取数据 (javascript to get the data from the table)
First, some setup. Let's tell the script which is the ID of the data table, the ID of the canvas tag and which column contains the data:
首先,进行一些设置。 让我们告诉脚本哪个是数据表的ID,canvas标签的ID和哪个列包含数据:
// source data table and canvas tag
var data_table = document.getElementById('mydata');
var canvas = document.getElementById('canvas');
var td_index = 1; // which TD contains the data
Next, select all table rows, then loop through the rows, selecting all TDs. Add the data we need to a data
array. While at it, run a total
of the data in the column and also create an array of random colors
. Paint each row with the selected color. (we'll see the actual getColor() in a bit.)
接下来,选择所有表行,然后循环浏览各行,选择所有TD。 将我们需要的data
添加到data
数组中。 而在它,运行一个total
的数据的列中,并且还创建的随机阵列colors
。 用所选颜色绘制每一行。 (稍后将看到实际的getColor()。)
var tds, data = [], color, colors = [], value = 0, total = 0;
var trs = data_table.getElementsByTagName('tr'); // all TRs
for (var i = 0; i < trs.length; i++) {
tds = trs[i].getElementsByTagName('td'); // all TDs
if (tds.length === 0) continue; // no TDs here, move on
// get the value, update total
value = parseFloat(tds[td_index].innerHTML);
data[data.length] = value;
total += value;
// random color
color = getColor();
colors[colors.length] = color; // save for later
trs[i].style.backgroundColor = color; // color this TR
}
JavaScript在画布上绘制数据 (javascript to plot the data on the canvas)
Time for the fun part, the drawing! First, we need to create a context object. Then figure out the raduis of the pie and the center, all based on the width/height pf the canvas tag:
时间是有趣的部分,绘画! 首先,我们需要创建一个上下文对象。 然后根据canvas标签的宽度/高度计算出饼图和中心的半径:
// get canvas context, determine radius and center
var ctx = canvas.getContext('2d');
var canvas_size = [canvas.width, canvas.height];
var radius = Math.min(canvas_size[0], canvas_size[1]) / 2;
var center = [canvas_size[0]/2, canvas_size[1]/2];
Next, let's loop through data
and paint pieces of the pie. To draw a piece of pie, you basically need to call these methods of the context object:
接下来,让我们遍历data
并绘制饼图。 要画一块,基本上,您需要调用上下文对象的以下方法:
beginPath()
- to start the piece of the piebeginPath()
-开始一块馅饼moveTo()
- to set the pencil in the centermoveTo()
-将铅笔设置在中心arc()
- draw a piece of a circlearc()
-画一个圆lineTo()
- finish the circle piece with a line back to the centerlineTo()
-用一条圆心回到圆心来完成圆片closePath()
andfill()
but set the fill color first.closePath()
和fill()
但先设置填充颜色。
Here's the actual code for this part, hopefully the comments help:
这是这部分的实际代码,希望注释会有所帮助:
var sofar = 0; // keep track of progress
// loop the data[]
for (var piece in data) {
var thisvalue = data[piece] / total;
ctx.beginPath();
ctx.moveTo(center[0], center[1]); // center of the pie
ctx.arc( // draw next arc
center[0],
center[1],
radius,
Math.PI * (- 0.5 + 2 * sofar), // -0.5 sets set the start to be top
Math.PI * (- 0.5 + 2 * (sofar + thisvalue)),
false
);
ctx.lineTo(center[0], center[1]); // line back to the center
ctx.closePath();
ctx.fillStyle = colors[piece]; // color
ctx.fill();
sofar += thisvalue; // increment progress tracker
}
效用 (utility)
Here's the function that gives a random color:
这是给出随机颜色的函数:
// utility - generates random color
function getColor() {
var rgb = [];
for (var i = 0; i < 3; i++) {
rgb[i] = Math.round(100 * Math.random() + 155) ; // [155-255] = lighter colors
}
return 'rgb(' + rgb.join(',') + ')';
}
C'est tout! Enjoy your pie 😀
祝你好运! 享受你的馅饼😀
UPDATE: Comment by Zoltan below, if you use Explorer Canvas, you can make this work in IE with only this: <!--[if IE]><script type="text/javascript" src="/path/to/excanvas.js"></script><![endif]-->
更新:以下是Zoltan的评论,如果您使用Explorer Canvas ,则只能通过以下方式使它在IE中起作用: <!--[if IE]><script type="text/javascript" src="/path/to/excanvas.js"></script><![endif]-->
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
帆布指纹