在c语言中realloc
There could be many situations while programming in C or C++ language when you need to dynamically allocate memory. Normally, the compiler during compilation allocates memory for a program.
当需要动态分配内存时,在使用C或C ++语言进行编程时可能会有很多情况。 通常,编译器在编译过程中为程序分配内存。
In situations where the computer does not know how much memory to allocate, like in the case of array initialization (without predefined array length) both C and C++ programming languages give the user the power to dynamically allocate memory at run-time.
在计算机不知道要分配多少内存的情况下,例如在数组初始化的情况下(没有预定义的数组长度),C和C ++编程语言都为用户提供了在运行时动态分配内存的能力。
The four functions used for dynamic allocation of memory are,
用于动态分配内存的四个功能是:
malloc()
malloc()
calloc()
calloc()
realloc()
realloc()
free()
free()
In C and C++, all the above functions are defined inside the stdlib
and cstdlib
header files. Learn more about malloc() and calloc()
在C和C ++中 ,上述所有函数均在stdlib
和cstdlib
头文件中定义。 了解有关malloc()和calloc()的更多信息
In this tutorial, let’s go over the realloc() function in both languages.
在本教程中,让我们介绍两种语言的realloc()函数。
realloc()函数 (The realloc() function)
The realloc()
function dynamically reallocates previously allocated memory(un-freed) as well as resizes it.
realloc()
函数动态地重新分配先前分配的内存(未释放)并调整其大小。
Syntax:
语法:
ptr = realloc( ptr , new_size );
ptr
– It is the pointer to the first byte of the previously allocated memory. After reallocation, it serves as the pointer to the first byte of the newly allocated memory,ptr
–它是指向先前分配的内存的第一个字节的指针。 重新分配后,它用作指向新分配的内存的第一个字节的指针,new_size
– It is the new size of the memory block for reallocation in bytes.new_size
–它是用于重新分配的内存块的新大小(以字节为单位)。
The realloc()
function returns a void pointer on a successful reallocation of memory. Whereas, returns a Null pointer on failure.
realloc()
函数在成功重新分配内存时返回空指针 。 而在失败时返回Null指针 。
The realloc() function automatically allocates more memory to a pointer as and when required within the program. If a pointer is allocated with 4 bytes by definition and a data of size 6 bytes is passed to it, the realloc() function in C or C++ can help allocate more memory on the fly.
当程序需要时,realloc()函数会自动将更多内存分配给指针。 如果根据定义为指针分配了4个字节并将大小为6个字节的数据传递给指针,则C或C ++中的realloc()函数可以帮助动态分配更多内存。
在C中重新分配 (realloc in C)
The following code illustrates the use of realloc()
in C.
以下代码说明了C中realloc()
的用法。
#include<stdio.h>
#include<stdlib.h> //required for using realloc() in C
int main()
{
int *ptr;
int i;
// typecasting pointer to integer
ptr= (int *)calloc(4,sizeof(int));
if(ptr!=NULL)
{
for(i=0;i<4;i++)
{
printf("Enter number number %d: ", i+1);
scanf("%d",(ptr+i));
}
}
//reallocation of 6 elements
ptr= (int *)realloc(ptr,6*sizeof(int));
if(ptr!=NULL)
{
printf("\nNew memory allocated!\n");
for(;i<6;i++)
{
printf("Enter new number %d: ",i);
scanf("%d",(ptr+i));
}
}
printf("\n\nThe numbers are:\n");
for(i=0;i<6;i++)
{
printf("%d \n",ptr[i]);
}
free(ptr);
return 0;
}
Output:
输出:
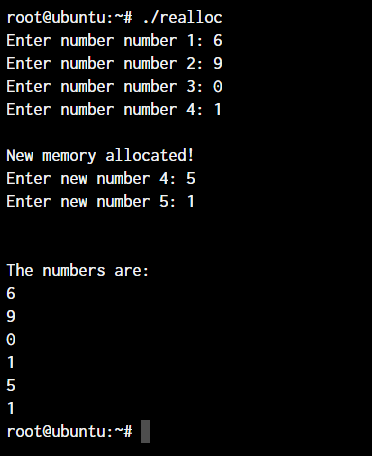
Understanding the code: In the above code, we have tried to solve a simulated real-world situation using the realloc()
function in C.
理解代码:在上面的代码中,我们尝试使用C中的realloc()
函数来解决模拟的现实情况。
As you can see, after the declaration of an integer pointer, ‘ptr’ and an integer iterator ‘i’, we have dynamically allocated memory using calloc()
for 4 sets of integer type data.
如您所见,在声明了整数指针'ptr'和整数迭代器'i'之后 ,我们使用calloc()
为4组整数类型数据动态分配了内存。
With ptr pointing to the first memory location. Then we have checked for successful memory allocation using the condition ptr!=NULL
.
使用ptr指向第一个内存位置。 然后,我们使用条件ptr!=NULL
检查了内存分配是否成功。
After the successful allocation of memory, we took user input for the number of their choice. These numbers are the specific values or data stored inside the memory spaces allocated by the calloc()
function.
成功分配内存后,我们接受了用户输入的选择数量。 这些数字是在calloc()
函数分配的内存空间中存储的特定值或数据。
But after this, we get another 2 numbers to store. In that case, we use the realloc()
function and reallocate memory space for a total of 6 sets of data. After successful reallocation, the previous 4 data stored stay intact and untouched. So, accordingly, we take user input for the 2 new data.
但是之后,我们又要存储两个数字。 在那种情况下,我们使用realloc()
函数并为总共6组数据重新分配内存空间。 成功重新分配后,先前存储的4个数据保持不变并且保持不变。 因此,因此,我们接受用户输入的2个新数据。
Certainly, to check whether all our data have been stored, we try to print all of them using the pointer, ptr
. After that, we yet again use realloc(ptr,0)
to free all the memory locations that we have used. Simply, realloc(ptr,0)
does the same task free(ptr)
does.
当然,要检查是否已存储所有数据,我们尝试使用指针ptr
打印所有数据。 之后,我们再次使用realloc(ptr,0)
释放我们已使用的所有内存位置。 简而言之, realloc(ptr,0)
完成了free(ptr)
相同的任务。
In the next section, we have followed the same logic using C++ programming.
在下一节中,我们使用C ++编程遵循相同的逻辑。
C ++中的realloc() (realloc() in C++)
The below-given code illustrates the use of realloc()
in C++.
下面给出的代码说明了C ++中realloc()
的用法。
#include<iostream>
#include<cstdlib> // for using realloc() in C++
using namespace std;
int main()
{
int *ptr;
int i;
//allocation of memory for 4 elements
ptr= (int *)calloc(4,sizeof(int));
if(ptr!=NULL)
{
for(i=0;i<4;i++)
{
cout<<"Enter number "<<i<<":";
cin>>ptr[i];
}
}
//reallocation of memory
ptr= (int *)realloc(ptr,6*sizeof(int));
if(ptr!=NULL)
{
cout<<"\nNew memory allocated!\n";
for(;i<6;i++)
{
cout<<"Enter new number "<<i<<":";
cin>>ptr[i];
}
}
cout<<"\n\nThe numbers are:\n";
for(i=0;i<6;i++)
{
cout<<ptr[i]<<endl;
}
free(ptr);
return 0;
}
The output will be the same as the image above. The only difference is in the code which is now written in C++.
输出将与上面的图像相同。 唯一的区别是现在用C ++编写的代码。
结论 (Conclusion)
So in this article, we learned how to use the realloc() function in C as well as in C++. We also discussed the respective code for better understanding.
因此,在本文中,我们学习了如何在C和C ++中使用realloc()函数。 为了更好的理解,我们还讨论了各自的代码。
参考资料 (References)
- https://stackoverflow.com/questions/3482941/how-do-you-realloc-in-c https://stackoverflow.com/questions/3482941/how-do-you-realloc-in-c
- https://stackoverflow.com/questions/21672912/freeing-allocated-memory-realloc-vs-free/21673118#21673118 https://stackoverflow.com/questions/21672912/freeing-allocated-memory-realloc-vs-free/21673118#21673118
翻译自: https://www.journaldev.com/35532/realloc-in-c-and-c-plus-plus
在c语言中realloc